The Basics To CSS
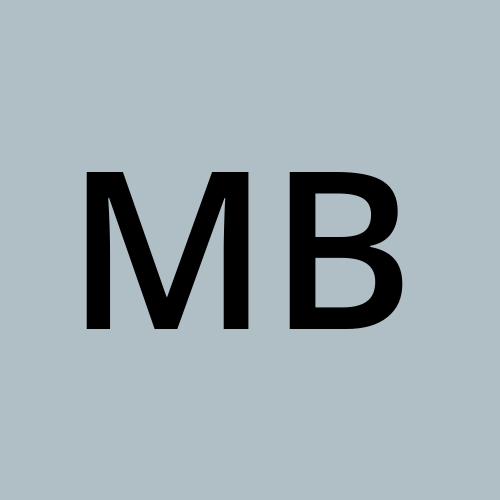

Introduction to CSS
Now that I've covered HTML, it's time to talk about CSS. Cascading Style Sheets (CSS) is a powerful language used to enhance the presentation of HTML documents. As I mentioned, CSS is used to design and style webpages. CSS dictates how elements should be rendered across various media types, including on-screen, print, and speech. The cascading aspect of CSS means that styles can be applied not only to a specific element but also inherited by its children, creating a hierarchy of styling. An easy way to explain this is to use code from the last article.
<body>
<p class="highlight">This is the first paragraph.</p>
<p class="highlight">This is the second paragraph.</p>
<p class="highlight">This is the third paragraph.</p>
</body>
In this example, the <body>
tag is the parent, and the <p>
tag is the child. Everything in the CSS code for the <body>
tag will affect all the <p>
tags as well. Some things you might want your CSS to control include the background color, font color, font size, positioning on the page, and much more. CSS saves a lot of work because it can control the layout of multiple web pages at once.
When tags like <font>
and color attributes were added to the HTML 3.2 specification, it created a nightmare for web developers. Building large websites, where fonts and color information were added to every single page, became a long and expensive process. To solve this problem, the World Wide Web Consortium (W3C) created CSS. CSS removed the style formatting from the HTML page. The style definitions are usually saved in external .css files (this can be done in Visual Studio Code). With an external stylesheet file, you can change the look of an entire website by changing just one file.
Developers now use HTML to describe the content of a web page and CSS for the styling, making things simpler. Need to add a new picture to your barber shop website? No problem. Just add the link to the picture in your HTML file, then go to your CSS file to change its size or position. In my previous article, in the HTML attribute section, I defined <style>
tags. I gave the example <p style="color: blue; font-size: 18px;">This is blue text.</p>
.
One of the things I love about coding is that there are many ways to do the same thing or achieve the same goal. If you need to make a small change to a webpage, you can use the <style>
tag, so you don't have to change two files; you can just add to the HTML file. Internal CSS is placed within the <style>
tags of an HTML document.
CSS SYNTAX
One CSS rule consists of a selector and a declaration block. The selector points to the HTML element you want to style. The declaration block contains one or more declarations separated by semicolons. Each declaration includes a CSS property name and a value, separated by a colon. More advanced multiple CSS declarations are separated with semicolons, and declaration blocks are surrounded by curly braces.
Example of CSS code:
button{ background-color: black; color: blue; }
In my example, button
is the selector, and inside the {}
curly braces is the declaration block. Now, background-color
and color
are the properties. Properties are what we are modifying about this button. Black and blue are the values or what we are modifying about the property. It would look black with blue text because the color
property changes the text color, while background-color
changes the background color.
We can divide CSS selectors into five categories:
Simple selectors (select elements based on name, id, class)
Combinator selectors (select elements based on a specific relationship between them)
Pseudo-class selectors (select elements based on a certain state)
Pseudo-elements selectors (select and style a part of an element)
Attribute selectors (select elements based on an attribute or attribute value)
The element selector selects HTML elements based on their name. The id selector uses the id attribute of an HTML element to select a specific element. Since an element's id is unique within a page, the id selector is used to select one unique element. To select an element with a specific id, write a hash (#) character followed by the id of the element. Example: <div id="header">This is a div with an id attribute.</div>
#header { color: red; }
The class selector selects HTML elements with a specific class attribute. To select elements with a specific class, write a period (.) character followed by the class name. For example, to target a class in an element like <p class="left">This paragraph.</p>
, the CSS code would look like this: p.left {color: red;}
.
The universal selector (*) selects all HTML elements on the page. That would look like * {text-align: center; color: blue;}
.
The grouping selector selects all the HTML elements with the same style definitions. Let's say you want to style the <h1>
, <title>
, and <p>
tags located in different parts of your HTML file. Remember, if the <p>
tag is inside the <h1>
tag, then the <p>
tag is a child of the <h1>
parent tag. Therefore, you would only need to style the <h1>
tag.
Here is a example of grouped selectors in code
h1, h2, p { text-align: center; color: blue; }
Simple CSS Selectors
#id | #firstname | Selects the element with id="firstname" |
.class | .intro | Selects all elements with class="intro" |
element.class | p.intro | Selects only <p> elements with class="intro" |
* | * | Selects all elements |
element | p | Selects all <p> elements |
element,element,.. | div, p | Selects all <div> elements and all <p> elements |
CSS Properties
Some common CSS properties I use:
button{
background-color: black;
Sets the background-color
has common values:
Color name value: black, blue ,red
Simply type the color name in the place of the value and u get that color.
rgb value: rbg(0, 127, 255);
(Red is the first value, Green is the seconded value, Blue is the third value) The Numbers defines the intensity of the color between 0 and 255. The code rbg(0, 0, 0);
would give you black no color intensity. The code rgb(255, 255, 255);
would give u white or full color intensity.
Hex value: #ff0000
; In CSS, colors can be specified using hexadecimal (hex) notation, which is a base-16 number system used to define colors on the web. Hex color codes start with a hash (#) followed by six hexadecimal values which represent the intensity of red, green, and blue in the color respectively. Now, to be honest, that is a very simple explanation, but that's as much as I want to cover in this article. If you want to know more, w3schools.com is a great place to look.
color: blue;
Color value: same common values as background-color
(color name, rgb, hex).
height: 40px;
Sets the height. common values: (Pixel value): 40px;
(Percentage): 75%;
(Auto): auto;
The browser calculates the height.
width: 110px;
Sets width. Refer to height common values.
cursor: pointer;
Change the mouse cursor's appearance when it hovers over an element. Pointer is the common value I use.
border-bottom: 2px solid white;
Common values I have use are: solid;
Single solid line. : double;
Double parallel lines. In CSS, the border property is used to define the outline of an element. It is a shorthand property that combines border-width, border-style, and border-color. You can specify the border for an element using these three sub-properties in any order. This is what a white solid border bottom would look like:
border-radius: 22px;
The border-radius property defines the radius of the element's corners. This property allows you to add rounded corners to elements. Four values - border-radius: 15px 50px 30px 5px;
(first value applies to top-left corner, second value applies to top-right corner, third value applies to bottom-right corner, and fourth value applies to bottom-left corner):
border-color: red;
Sets the border color.
border-style: solid;
Sets the border style.
border-width: 5px;
Sets the border width.
In Conclusion
I covered what CSS is and how it relates to HTML. I explained CSS syntax and how to write CSS in a code editor like Visual Studio Code. I went over some common properties, their values, and meanings. I provided example code as best as I could. Just like in my HTML Basics article, I barely scratched the surface of the vast world of CSS. In the next article I will cover more advanced CSS properties and features. Eventually also go into more HTML and CSS static web sites give examples of what it look’s like put together before I go into JavaScript.
Thoughts Feelings Story time !?!
As always, I love the learning process. Being so caught up on freeCodeCamp, I feel like I delayed this article a few more days than I would have liked. I understand that my journey to become the best self-taught programmer will never end because I can always be better tomorrow than I am today. There's always something new to learn, which is the nature of most great programmers from what I have learned. This week I also went to a meetup for freeCodeCamp on Dockers. I went to see it I could learn anything and to network. I did learn a little about Dockers which to my understanding is a application that would help you set up a container. Now, the purpose of this is when you write code on your computer, including libraries, databases, and services. Everything runs smoothly. When you go to your work computer to see it, it's buggy and won't render or function properly. With a docker container you would put everything in the container on you computer ( code, databases, libraries, and services). Once you have successfully set up the container on the Docker servers, you can go to your work computer or any other device your code will run on, and it will work as if you were on your home computer. Everyone up there was friendly most went to boot camps or got a degree. Some started the self-taught way and then pursued more formal education. It makes me a little nervous because they all seem smart but need help to make the pieces fit together when it comes to programing. A positive thing is that I think I was the youngest in the room, which made me feel good. I received offers to come back throughout the weeks to pick their brains and get help with interview questions for when I'm ready to apply for a job. I will be going up there next Friday to get advice and will start interviewing as soon as I feel ready. I love the community and all the help. This has been a long one. Thank you for taking the time to read. As always, see you next time.
Subscribe to my newsletter
Read articles from Michael Baisden directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
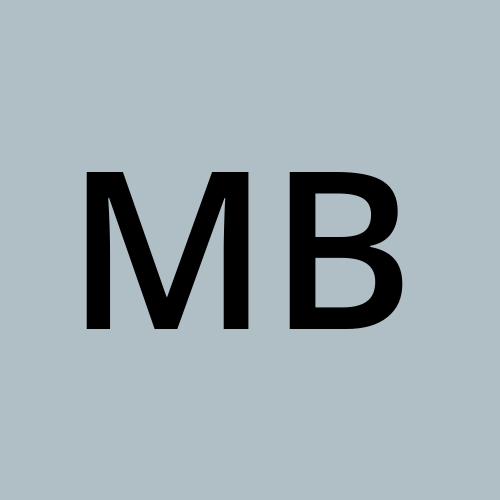
Michael Baisden
Michael Baisden
I am a developer from Columbus, Ohio, born and raised. I've always been into coding and working with computers. At the end of July 2024, I decided to teach myself how to program and write code. I love video games and music. I also love food—cooking, eating, and sharing. I am a God-loving man who keeps an open mind and loves to learn. I have a loving, caring girlfriend named Kerry and a cute dachshund named Kobe. When I'm not working or coding, I'm usually with them.