How to Set Up a Database and Display Data on the Frontend Using Laravel and Vue.js

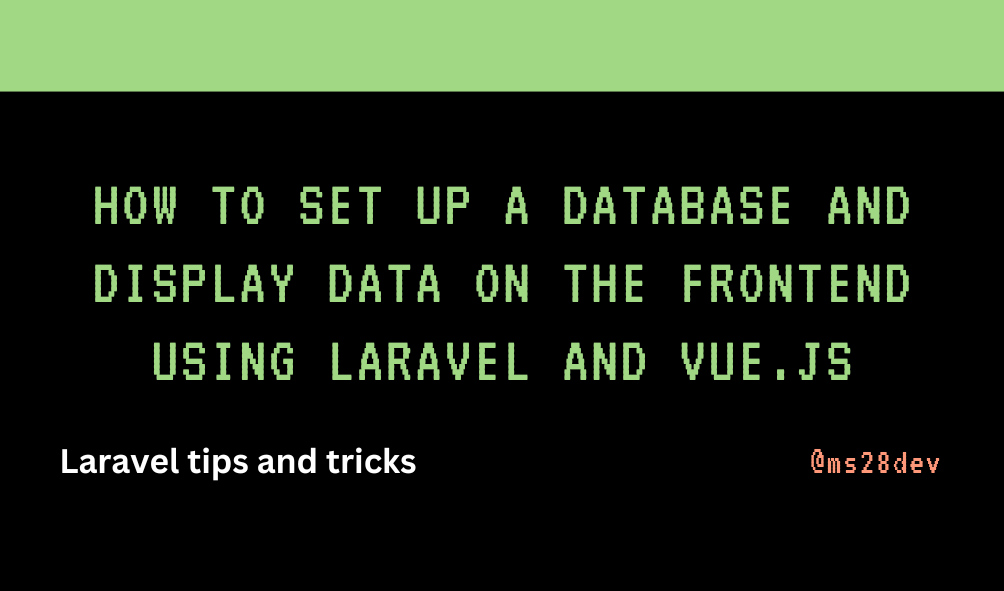
Introduction
Setting up a full-stack web application can seem challenging, but with the right combination of technologies, the process becomes much more manageable. One of the most popular stacks today is Laravel for the backend and Vue.js for the frontend. Laravel is a robust PHP framework that simplifies backend tasks like routing, database management, and authentication. Vue.js, on the other hand, is a flexible and lightweight JavaScript framework perfect for creating reactive user interfaces.
In this guide, we’ll walk through how to set up a database and display data on the frontend using Laravel for the backend and Vue.js for the frontend. We’ll demonstrate the process with an example of managing orders for an e-commerce application.
Prerequisites
Before we start, ensure you have the following tools installed on your machine:
PHP (version 7.4 or above)
Composer
Node.js and npm
Laravel (install it via Composer if you haven't)
Vue.js (Vue CLI or simply integrate it within Laravel)
A MySQL database (or another database like PostgreSQL)
Step 1: Set Up Laravel Project and Database
First, create a new Laravel project and configure your database connection:
Create a Laravel Project:
composer create-project --prefer-dist laravel/laravel orders-app
Configure the
.env
File: Open the.env
file in the root directory and update the database connection settings. If you’re using MySQL, the configuration will look like this:DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=orders_db DB_USERNAME=root DB_PASSWORD=
Ensure that you have created the database in MySQL (
orders_db
in this case) before running the migration.Run Migrations: Now that your database is set up, it’s time to run migrations. Laravel migrations help you manage database schema changes in a consistent and easy way.
Create a migration to manage orders using the following command:
php artisan make:migration create_manage_orders
Laravel will generate a migration file in the
database/migrations
folder. Open the migration file and define the table schema:public function up() { Schema::create('orders', function (Blueprint $table) { $table->id(); $table->string('product_name'); $table->integer('quantity'); $table->float('price'); $table->timestamps(); }); }
Run the Migration: Apply the migration to your database by running:
php artisan migrate
Step 2: Set Up the Laravel Model and Controller
Next, let’s create the Order model and a controller to handle the logic for fetching data from the database and sending it to the frontend.
Create the Order Model: Run the following command to generate the Order model:
php artisan make:model Order
This will create a model file at
app/Models/Order.php
. You can define relationships and attributes in this file.Create the Controller: Generate a controller for handling requests related to orders:
php artisan make:controller OrderController
Open the
OrderController.php
file inside theapp/Http/Controllers
directory. Add a method to fetch orders from the database:use App\Models\Order; class OrderController extends Controller { public function index() { // Fetch all orders $orders = Order::all(); return response()->json($orders); } }
Define the Route: In the
routes/web.php
file, define a route that calls the controller'sindex
method:Route::get('/orders', [OrderController::class, 'index']);
This route will return the order data in JSON format when accessed.
Step 3: Set Up Vue.js on the Frontend
Now, we need to set up Vue.js to display the fetched data on the frontend.
Install Vue: If you haven’t already set up Vue, you can install it within your Laravel project by running:
npm install vue@next
Edit the
resources/js/app.js
File: Import Vue and create a new Vue component for displaying the orders:import { createApp } from 'vue'; const app = createApp({ data() { return { orders: [] }; }, mounted() { // Fetch orders from the backend axios.get('/orders') .then(response => { this.orders = response.data; }) .catch(error => { console.error('Error fetching orders:', error); }); } }); app.mount('#app');
Create the Blade Template: Open the
resources/views/welcome.blade.php
file and add a root element for the Vue app:<div id="app"> <ul> <li v-for="order in orders" :key="order.id"> {{ order.product_name }} ({{ order.quantity }}) - ${{ order.price }} </li> </ul> </div>
Run the Application: Make sure your assets are built using
npm run dev
, and start the Laravel server:php artisan serve
Visit
http://127.0.0.1:8000
in your browser, and you should see the orders displayed.
Step 4: Conclusion
You now have a fully functional setup that displays data from your database on the frontend using Laravel and Vue.js. This basic example can be extended further to include more complex features like search functionality, pagination, and CRUD operations.
Further Reading:
Introduction to Laravel Eloquent ORM: Learn more about Laravel's Eloquent ORM to efficiently interact with your database.
Advanced Vue.js Components: Dive deeper into Vue.js and explore advanced component features.
Using Laravel API Resources: Learn how to structure and format your API responses using Laravel’s API resources.
State Management in Vue.js with Vuex: Explore how to manage state across your Vue.js application using Vuex.
Securing Your Laravel Application: Understand how to implement security features like authentication and authorization in Laravel.
By following these guides, you’ll be well on your way to building powerful full-stack applications with Laravel and Vue.js.
Subscribe to my newsletter
Read articles from Manvendra Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manvendra Singh
Manvendra Singh
R3F | Laravel | Babylon | Three.js | WordPress Performance Enthusiast