How to Initialize Paystack Payment in React Native Using react-native-paystack-webview
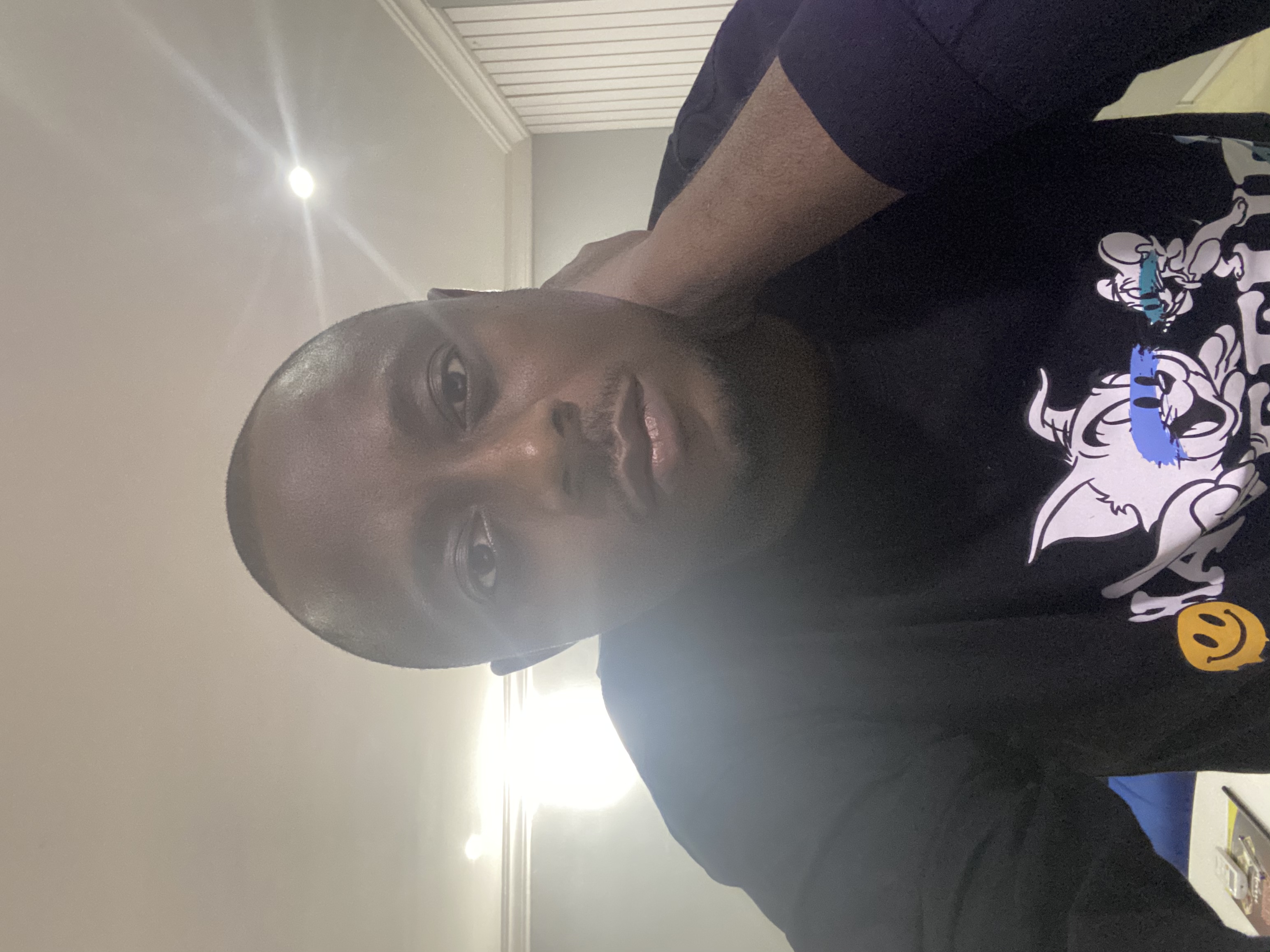
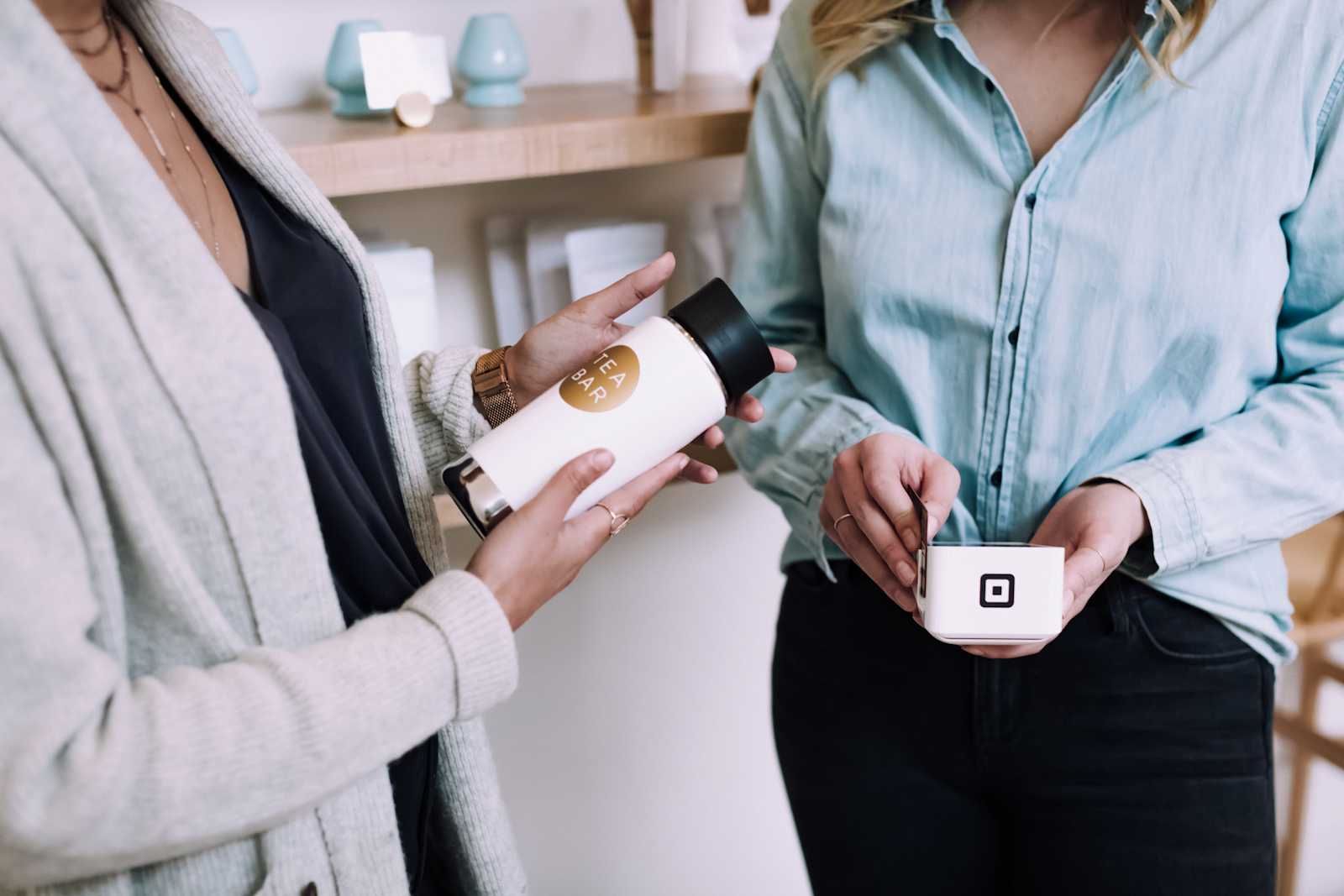
Introduction
Integrating seamless payment solutions into a mobile app is crucial for many applications. In this blog post, we will walk through how to integrate Paystack payment in a React Native app using the react-native-paystack-webview
package. Paystack is one of the most popular payment gateways in Africa, and this guide will help you handle payments efficiently within your React Native application.
Prerequisites
Before we begin, ensure that you have:
A working React Native app
Basic knowledge of React Native components and hooks
Your Paystack public key (you can get this by signing up for a Paystack account and creating a test or live API key)
Step 1: Installing Required Packages
Start by installing the required dependencies. You need both react-native-paystack-webview
and react-native-webview
(since Paystack transactions take place in a WebView).
Install the react-native-paystack-webview
package
npm install react-native-paystack-webview
Alternatively, if you are using Yarn:
yarn add react-native-paystack-webview
Install the react-native-webview
package
To ensure the WebView component works in React Native, install the react-native-webview
dependency as well.
npm install react-native-webview
After installation, always run npm install
(or yarn
) to sync up your dependencies.
Step 2: Setting Up the Paystack Component
Once the packages are installed, you can begin setting up the Paystack component in your app. Below is an example of how to use react-native-paystack-webview
with your Paystack public key to initiate a payment.
import React, { useRef } from 'react';
import { Paystack, paystackProps } from 'react-native-paystack-webview';
import { View, TouchableOpacity, Text } from 'react-native';
function Pay() {
// Create a ref for the Paystack WebView
const paystackWebViewRef = useRef(paystackProps.PayStackRef);
return (
<View style={{ flex: 1 }}>
{/* Paystack WebView */}
<Paystack
paystackKey="your-public-key-here" // Your Paystack public key
billingEmail="paystackwebview@something.com" // The email of the payer
amount={'25000.00'} // Amount to be charged (e.g. 25,000 NGN)
onCancel={(e) => {
// Handle payment cancellation
console.log('Payment cancelled', e);
}}
onSuccess={(res) => {
// Handle successful payment
console.log('Payment successful', res);
}}
ref={paystackWebViewRef}
channels={['card', 'ussd', 'qr', 'bank_transfer']} // Available payment channels
/>
{/* Button to trigger the payment */}
<TouchableOpacity onPress={() => paystackWebViewRef.current.startTransaction()}>
<Text>Pay Now</Text>
</TouchableOpacity>
</View>
);
}
export default Pay;
Step 3: Explaining the Code
Paystack Component: This is where the magic happens. The Paystack component provides the WebView that handles the payment flow.
paystackKey
: This is your Paystack public key. Replace the placeholder"your-public-key-here"
with your actual key.billingEmail
: The email of the payer, which is required by Paystack.amount
: The amount to be charged (in kobo for NGN).channels
: An array specifying the payment methods you want to enable (e.g., card, USSD, QR code, bank transfer).onCancel
andonSuccess
: Callback functions that are triggered when the payment is canceled or successful.
Step 4: Handling Transactions
onCancel: This function will be triggered if the user cancels the transaction. You can handle this by displaying a message to the user or logging the event for debugging purposes.
onSuccess: This function will be triggered if the payment is successful. Here, you can handle post-payment processes such as notifying the user, saving the transaction details to your database, or updating the user’s subscription status.
Step 5: Running the App
Now that everything is set up, you can run your app using:
npx react-native run-android
or
npx react-native run-ios
Make sure to test both success and cancellation scenarios to ensure that everything works smoothly.
Conclusion
In this post, we’ve walked through how to integrate Paystack payment functionality using the react-native-paystack-webview
package in your React Native app. This package allows you to securely process payments within your app by providing various payment options like card, USSD, bank transfer, and more.
By using Paystack’s powerful APIs, you can create a seamless and secure checkout experience for your users. You can extend this setup further by adding custom logic for transaction validation or integrating with your backend services.
Further Reading
For more advanced use cases or to explore other features provided by Paystack, you can check out their official documentation:
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
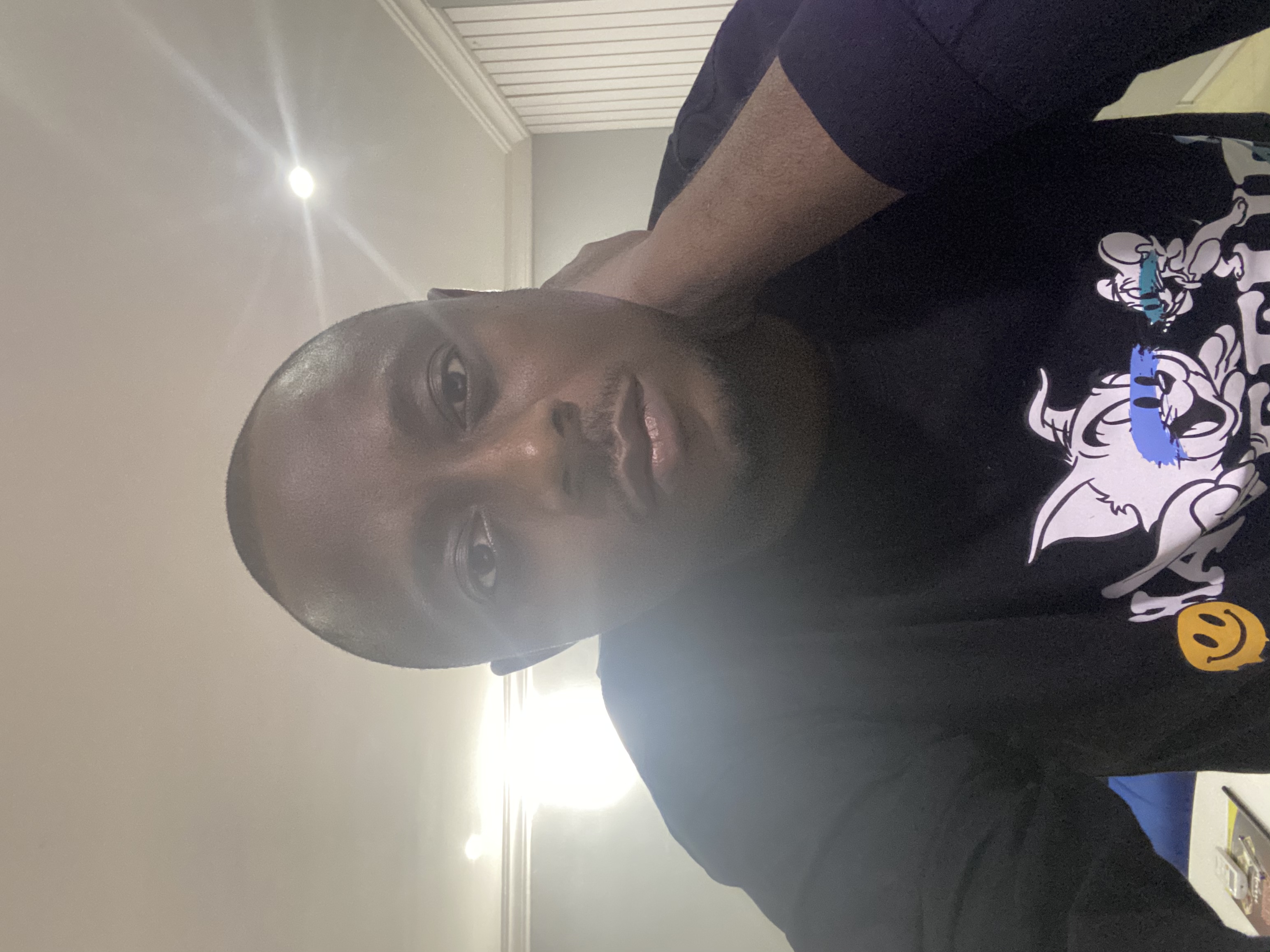
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.