Bring Your UI to Life: Creating Micro Interactions with Figma, Lottie & React

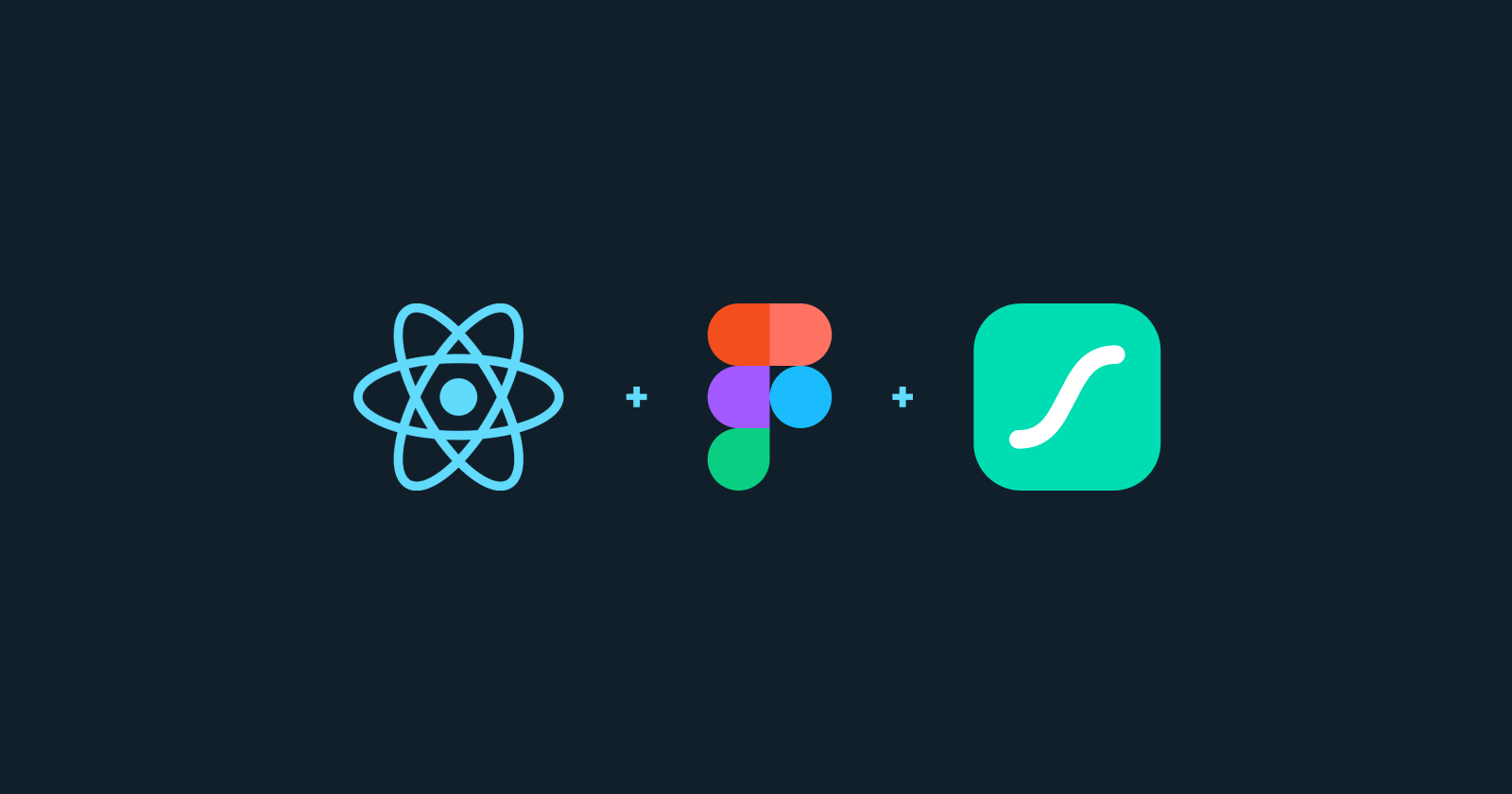
Micro interactions are common on most websites and mobile apps these days to enhance the user experience.
I tried creating a simple micro interaction for my portfolio web app. While this could be done with CSS alone, but it is really complex. I found it easier to create complex animations using Figma and Lottie (though this does involve additional JSON files and the lottie-react library). The best part is that you can achieve this with a little knowledge of Figma.
I wanted to create a simple animation for a color picker since I couldn’t find a free version of the same online.
Follow these steps to add a simple micro interaction to your web app-
Create Figma Design
Create Icon
- Create a frame of your desired size. Since it's an icon, you can use standard sizes like 24, 32, etc. I chose 96.
Do not add any fill to the frame so that your animation will have a transparent background.
I have created 3 elements on the frame -
- Now we will create two states (2 frames) for the animation: open state and closed state. We already created the open state. Let's create a closed state by duplicating the current frame. (Select the current frame, press Alt, and drag). Now, adjust the elements based on the animation you want to create.
Make sure the frame still has all the 3 elements i.e. top, mid, last in our scenario. This will help with the animation using the smart animate feature in Figma.
Create Prototype
Now lets add a prototype
We want our animation to go from the closed state to the open state. Our starting point will be the closed state frame.
Now add an interaction between the frames.
Thats it.
Export prototype to Lottie
- Add Lottie plugin to figma
Go to Menu > Plugins > Manage Plugins > search for Lottie and add the plugin. You will need to create a Lottie account as well.
Should open a small window like this -
- Select your starting frame and click on save to workspace.
This will export your prototype to lottie.
Download Lottie JSON file
Go to app.lottiefiles.com and select the uploaded animation file to open the editor. Then download the Lottie JSON file.
Add lottie animation to react component
Add the Lottie JSON file to your repository.
Add the
lottie-react
package to your repository.Create a React component to display the Lottie animation.
import { useLottie } from 'lottie-react'
import React from 'react'
import lottieData from './ColorSwatchIcon.json'
const ColorPicker = () => {
const { View, setSpeed, play, setDirection } = useLottie(
{
animationData: lottieData,
loop: false,
autoplay: false,
}
)
setSpeed(1)
return (
<div
className="color-picker"
onMouseEnter={() => {
setDirection(1)
play()
}}
onMouseLeave={() => {
setDirection(-1)
play()
}}
>
{View}
</div>
)
}
export default ColorPicker
And that's it, you have created a simple micro-interaction which plays the animation on hover.
Hope this helps. Thanks for reading.
Subscribe to my newsletter
Read articles from Anoop Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anoop Jadhav
Anoop Jadhav
Frontend Developer at Thoughtworks