@ConfigurationProperties: A Powerful Tool for Spring Boot Configuration
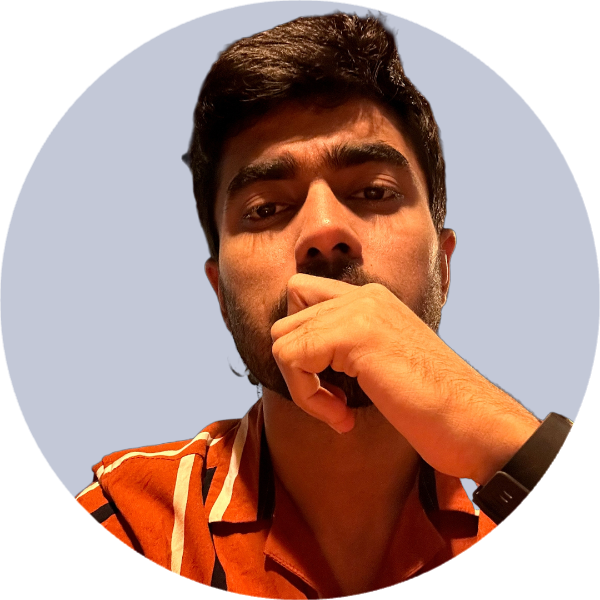
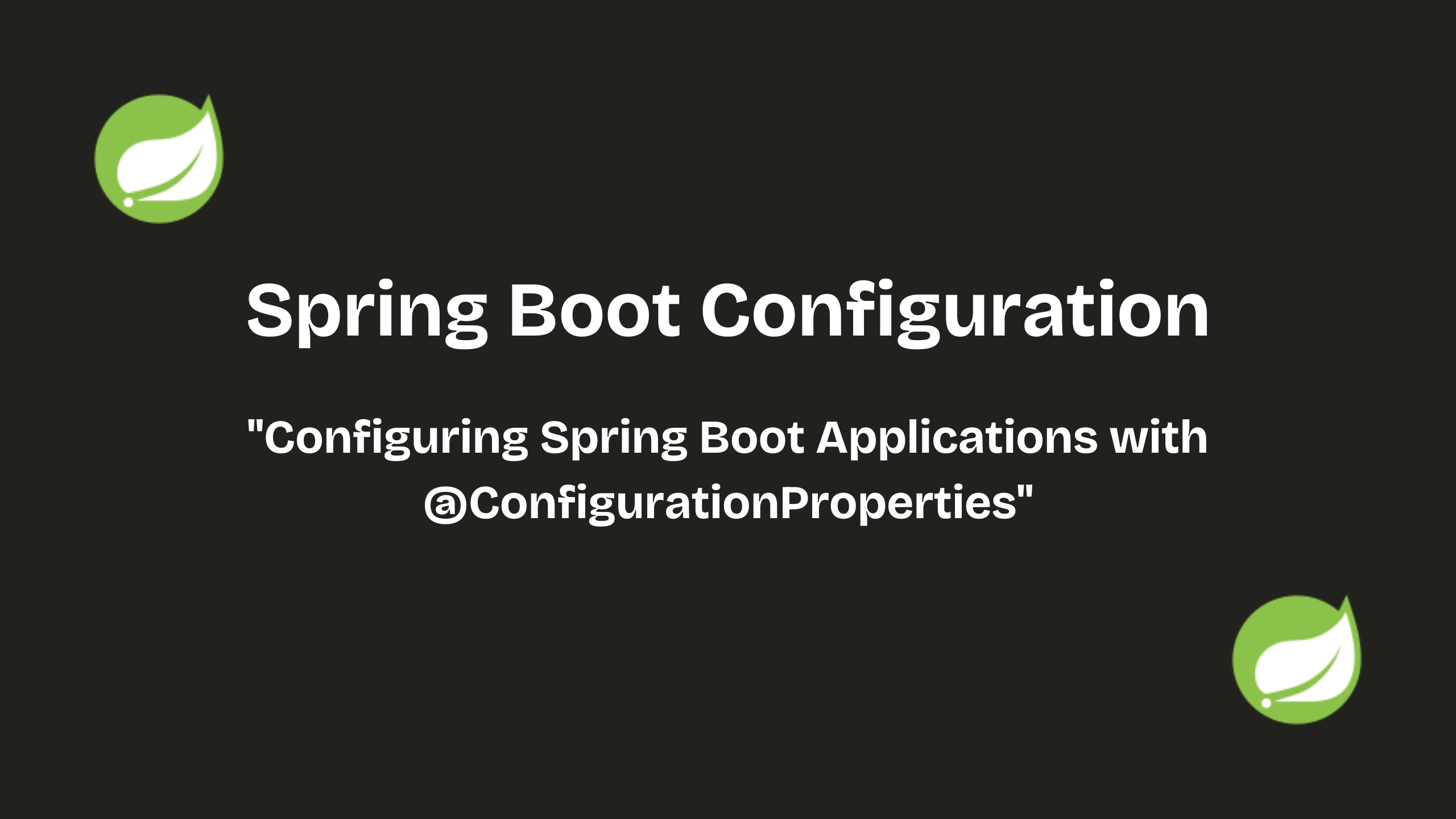
Introduction:
While working with Spring Boot applications, most of the times we need to externalize configurations into properties files or environment variables. This makes our application more flexible and easier to maintain in production.
In Spring Boot, one of the best ways to handle these externalized configurations is by using the @ConfigurationProperties
annotation.
@ConfigurationProperties
is an annotation in Spring Boot used to map application properties (defined in .properties
or .yaml
files) to Java objects(Java Beans). It allows you to bind hierarchical properties from a configuration file into a POJO (Plain Old Java Object), which can then be injected into your application's components.
In this blog, we’ll explore what @ConfigurationProperties
is, why it’s useful, how to use it, and why it is preferable over the @Value
annotation.
Why to use @ConfigurationProperties
?
Using @ConfigurationProperties
has several benefits:
Type Safety: It binds properties from the configuration files into strongly-typed POJOs, allowing for better validation and type safety.
Readability: Rather than using multiple
@Value
annotations, you can group related properties together in a single class, improving code organization and readability.Scalability: When your configuration grows, it becomes much easier to maintain and scale using
@ConfigurationProperties
than managing each property with individual@Value
annotations.
Getting-Started with @ConfigurationProperties
If you're using Spring Boot 2.2 or newer, there’s no need to add any additional dependencies, as @ConfigurationProperties
support is included out of the box.
Define Properties inside
application.yml
orapplication.properties
Create a Configuration Class
Next, create a POJO class to map the properties defined above. This class will use
@ConfigurationProperties
to bind the properties to its fields.In this example, we’re using the prefix
app.config
to map the properties (name
,version
,max-users
) from the properties file.Note: Getters and setters are mandatory for Spring to bind the properties correctly.
Run the Application and Access Configuration Properties
You can now inject
AppConfig
into any Spring-managed component (such as services or controllers) and access the configuration properties.Comparison:
@ConfigurationProperties
vs@Value
Hierarchical Configuration:
@ConfigurationProperties
allows for hierarchical configuration, meaning you can group related properties under a common prefix. In contrast,@Value
does not support this and each property must be handled individually.Type Safety:
@ConfigurationProperties
offers type safety by mapping properties to strongly-typed fields in a POJO. With@Value
, you can only inject simple types and there’s no validation of complex types at the configuration binding stage.Grouping Related Properties:
@ConfigurationProperties
makes it easy to group related properties in a single class, improving readability and organization.@Value
requires each property to be individually injected, which can lead to cluttered code as your configuration grows.Large Configuration Management: Managing large configurations with
@ConfigurationProperties
is more scalable and easier to maintain because you can organize properties into well-structured POJOs. With@Value
, as the number of properties increases, the code can become messy and difficult to manage.Getters/Setters Requirement: For
@ConfigurationProperties
to work, you must define getters and setters in the POJO to bind the properties. In contrast,@Value
does not require getters or setters, as it injects values directly.Default Value Support:
@Value
allows you to specify default values directly when the property is not present.@ConfigurationProperties
does not have built-in support for specifying default values in the same way, although you can handle defaults in other ways (like initializing fields directly in the class).
Advantages of @ConfigurationProperties
Grouping Related Properties: With
@ConfigurationProperties
, you can group related properties in a single class, improving clarity and organization.Type Safety: Spring validates the properties and maps them to the appropriate types. If there's a type mismatch, it fails at startup, making the application more robust.
Better for Complex Configurations:
@ConfigurationProperties
is more scalable and better suited for managing complex configurations, especially in large applications.Validation Support: You can also add validation annotations like
@NotNull
,@Min
, or@Max
to ensure that properties meet certain constraints, making it easier to catch invalid configurations at runtime.
Conclusion
The @ConfigurationProperties
annotation is a powerful tool in Spring Boot that offers type safety, improved readability, and better scalability compared to @Value
. It allows you to bind hierarchical properties to Java objects, making configuration handling more structured and maintainable.
Whether you're building a simple application or a complex microservice architecture, @ConfigurationProperties
provides a clean and flexible way to manage externalized configurations.
If you're still using @Value
in your Spring Boot applications, consider switching to @ConfigurationProperties
, especially as your configuration grows and becomes more complex.
Subscribe to my newsletter
Read articles from Sudarshan Doiphode directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
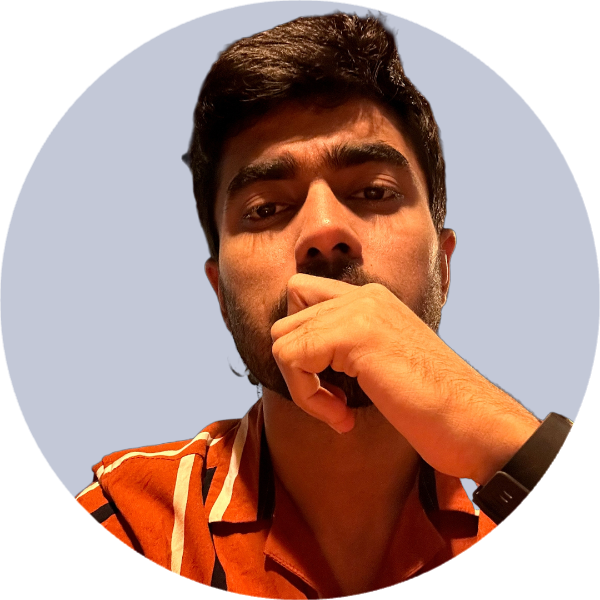
Sudarshan Doiphode
Sudarshan Doiphode
👋 Hey there! I'm Sudarshan, a tech enthusiast with a focus on: • 🚀 Spring Boot • 🌼 Spring • 🔒 Spring Security • 🌱 Hibernate • ☕ Java 8 & Java • 🚀 Apache Kafka • 🛢️ Apache Maven • 🐳 Docker • 🔗 Git • 🏗️ Design Patterns • And more tech adventures! Follow for insights and join the journey. 🌟👨💻 #TechEnthusiast