π Jetpack Compose Tip: Flexible Layouts with FlowRow & FlowColumn πΌοΈ
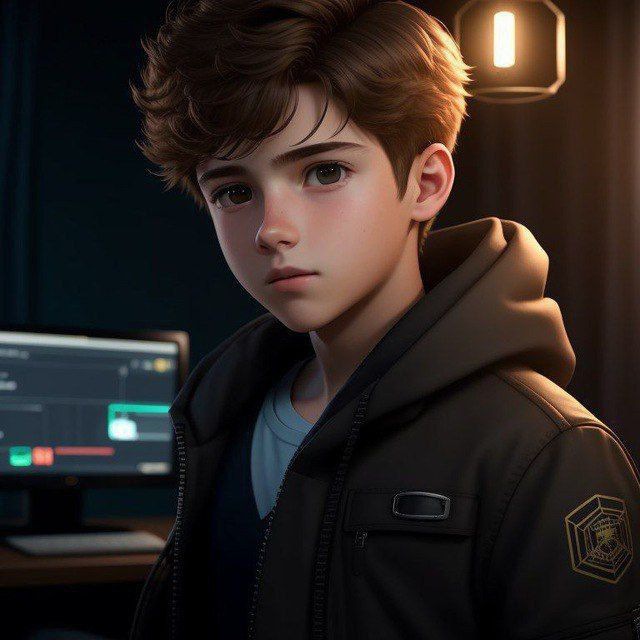
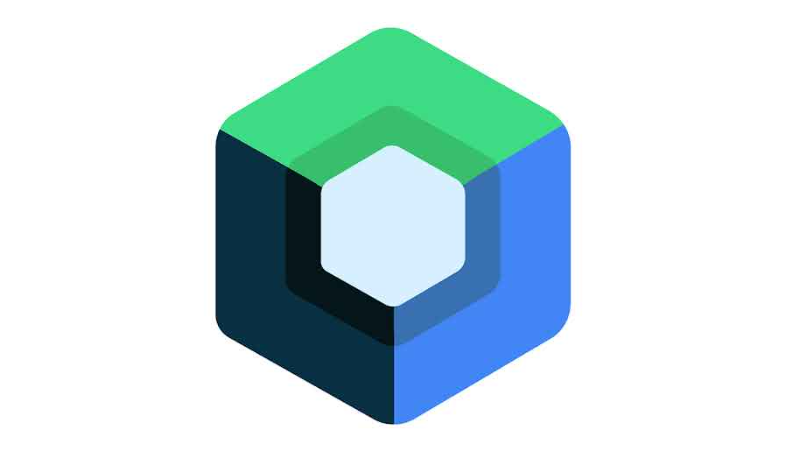
If you're building Android apps with Jetpack Compose, you may often need to arrange UI elements in flexible ways. That's where FlowRow and FlowColumn from the Accompanist library come to the rescue! π¦ΈββοΈ
π¨βπ» What do they do?
1οΈβ£ FlowRow: Arranges items in a horizontal row. When space runs out, they automatically "flow" to the next line, perfect for creating tag lists or chips.
2οΈβ£ FlowColumn: Similar, but vertical! Items are arranged in a column, and when the height runs out, they wrap into the next column.
π Use Cases:
Dynamic Content Layouts: Perfect for lists like tags, chips, or variable-sized items.
Responsive Design: Automatically adjusts based on available space.
Adaptive UI: Useful when supporting various screen sizes and orientations.
πͺ Advantages:
β¨ Automatic Wrapping: No need for manual calculation of wrapping positions. π Simpler Code: Reduces complex layout logic and calculations for arranging content. π± Responsive: Works great with dynamic or user-generated content. π Reusable: You can easily use FlowRow
and FlowColumn
across different screens or layouts.
β οΈ Disadvantages:
β‘οΈ Performance: Handling very large numbers of items may impact performance, so watch out for heavy use in large lists.
π Limited Customization: Compared to more complex layouts, fine-grained control over spacing, alignment, and item positioning can be somewhat limited.
πΌ Dependency: Since this is part of the Accompanist library, you'll need to add a third-party dependency to your project.
π‘ Here's how you can use them:
FlowRow example to create a wrapping row
import com.google.accompanist.flowlayout.FlowRow
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.size
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
@Composable
fun FlowRowExample() {
FlowRow(
modifier = Modifier.background(Color.LightGray),
mainAxisSpacing = 8.dp, // space between items in the row
crossAxisSpacing = 8.dp // space between rows
) {
for (i in 1..10) {
Box(
modifier = Modifier
.size(80.dp)
.background(Color.Cyan)
) {
Text(text = "Item $i")
}
}
}
}
// FlowColumn example to create a wrapping column
import com.google.accompanist.flowlayout.FlowColumn
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.size
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
@Composable
fun FlowColumnExample() {
FlowColumn(
modifier = Modifier.background(Color.LightGray),
mainAxisSpacing = 8.dp, // space between items in the column
crossAxisSpacing = 8.dp // space between columns
) {
for (i in 1..10) {
Box(
modifier = Modifier
.size(80.dp)
.background(Color.Cyan)
) {
Text(text = "Item $i")
}
}
}
}
π§ Make sure to add the Accompanist library to your project:
implementation ("com.google.accompanist:accompanist-flowlayout:<version>")
Flow layouts are incredibly helpful when dealing with dynamic content or items of variable size! π¨ You get complete flexibility without worrying about manually wrapping items.
Subscribe to my newsletter
Read articles from Hariom OP directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
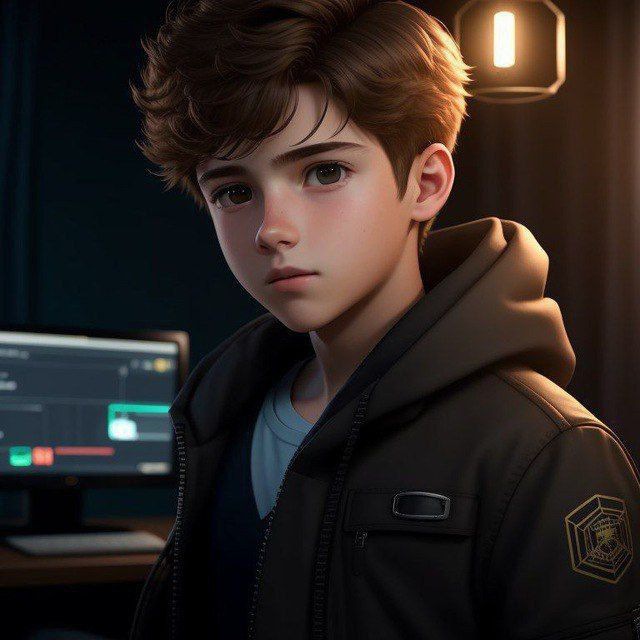
Hariom OP
Hariom OP
Passionate Android developer π