Java Record
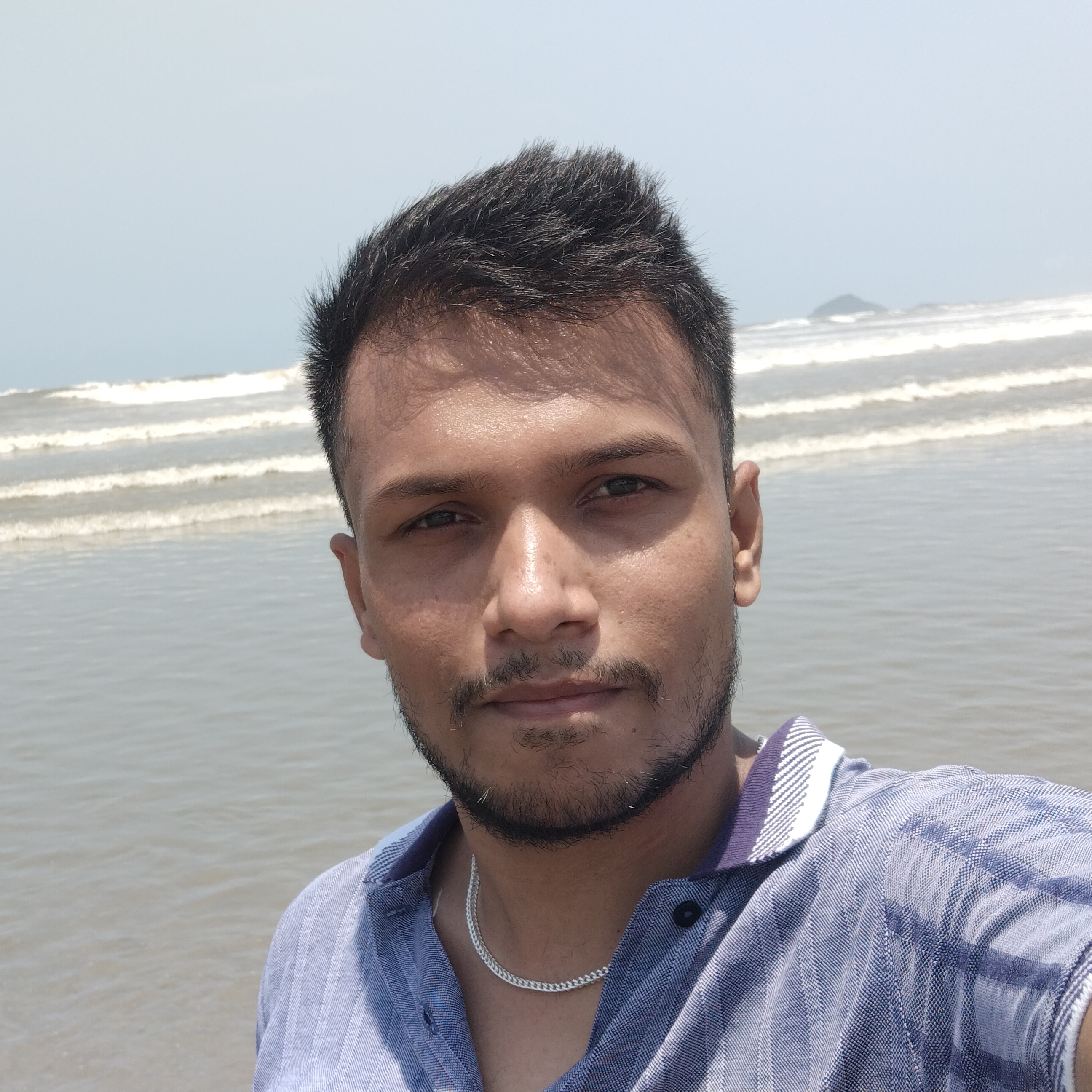
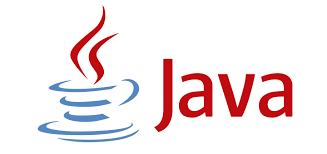
As an SDET, writing efficient and maintainable code is crucial, especially when dealing with data models in test automation frameworks. Traditionally, creating simple data classes in Java meant writing getters, setters, constructors, and implementing toString()
, equals()
, and hashCode()
methods. This can lead to a lot of boilerplate code.
With the introduction of records
in Java 14, we can simplify this process significantly. Records
automatically generate these methods, allowing us to focus more on test logic rather than the mechanics of object creation.
public class Person {
private final String name;
private final int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
// toString(), equals(), hashCode() methods would also be here
}
Now, compare it to using a `record`:
public record Person(String name, int age) {}
In just one line, the record
handles everything, freeing up time to enhance test coverage and improve automation scripts. It’s a small change with a big impact on productivity and code quality.
Has anyone else started using records
in their test automation projects? I’d love to hear your experiences and how it has influenced your workflow.
Subscribe to my newsletter
Read articles from Karthik T directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
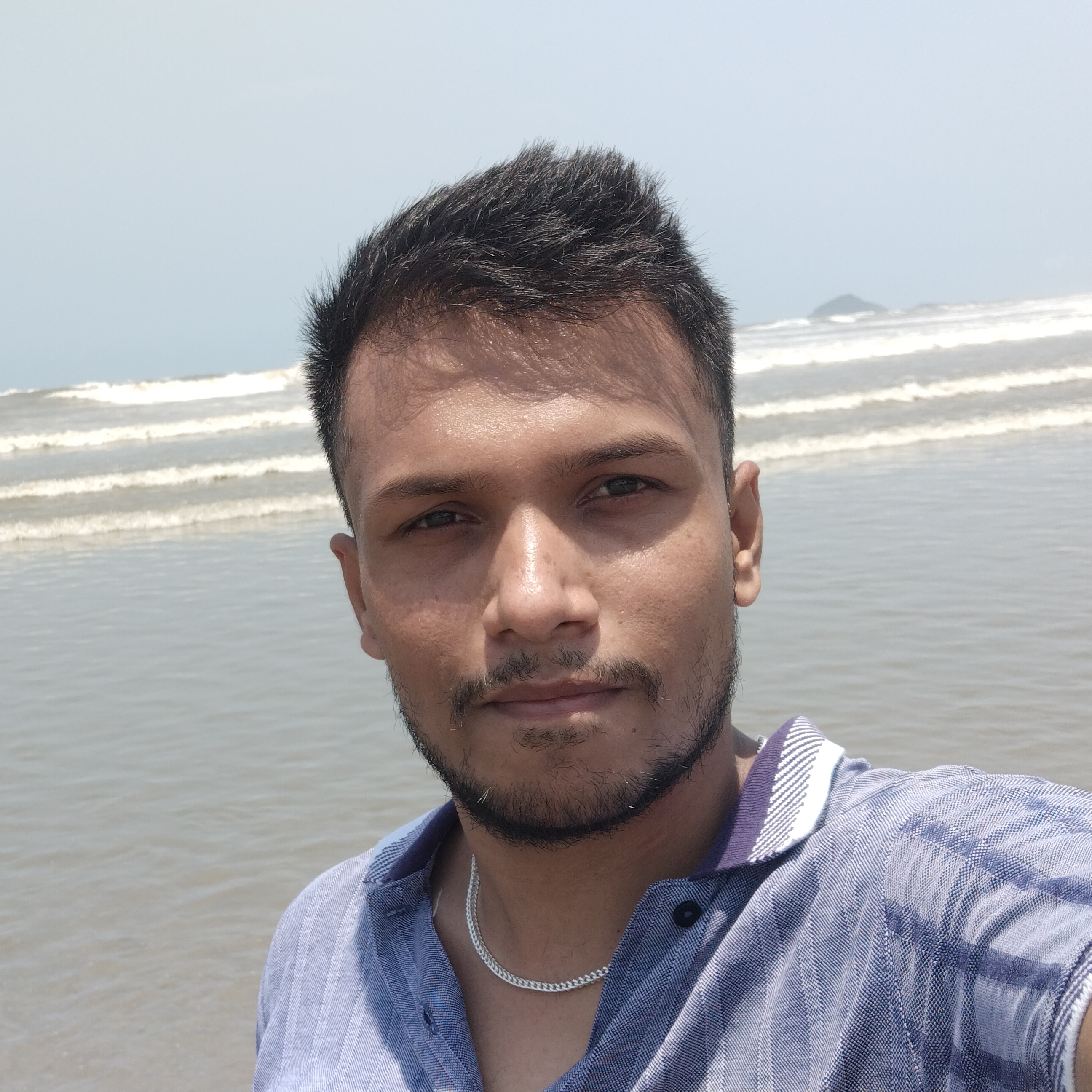
Karthik T
Karthik T
Experienced SDET with expertise in building hybrid UI & API automation frameworks using Playwright, Selenium, RestAssured and Docker ,Proficient in delivering scalable test solutions with comprehensive reporting