Understanding and Fixing Hydration Errors in Next.js

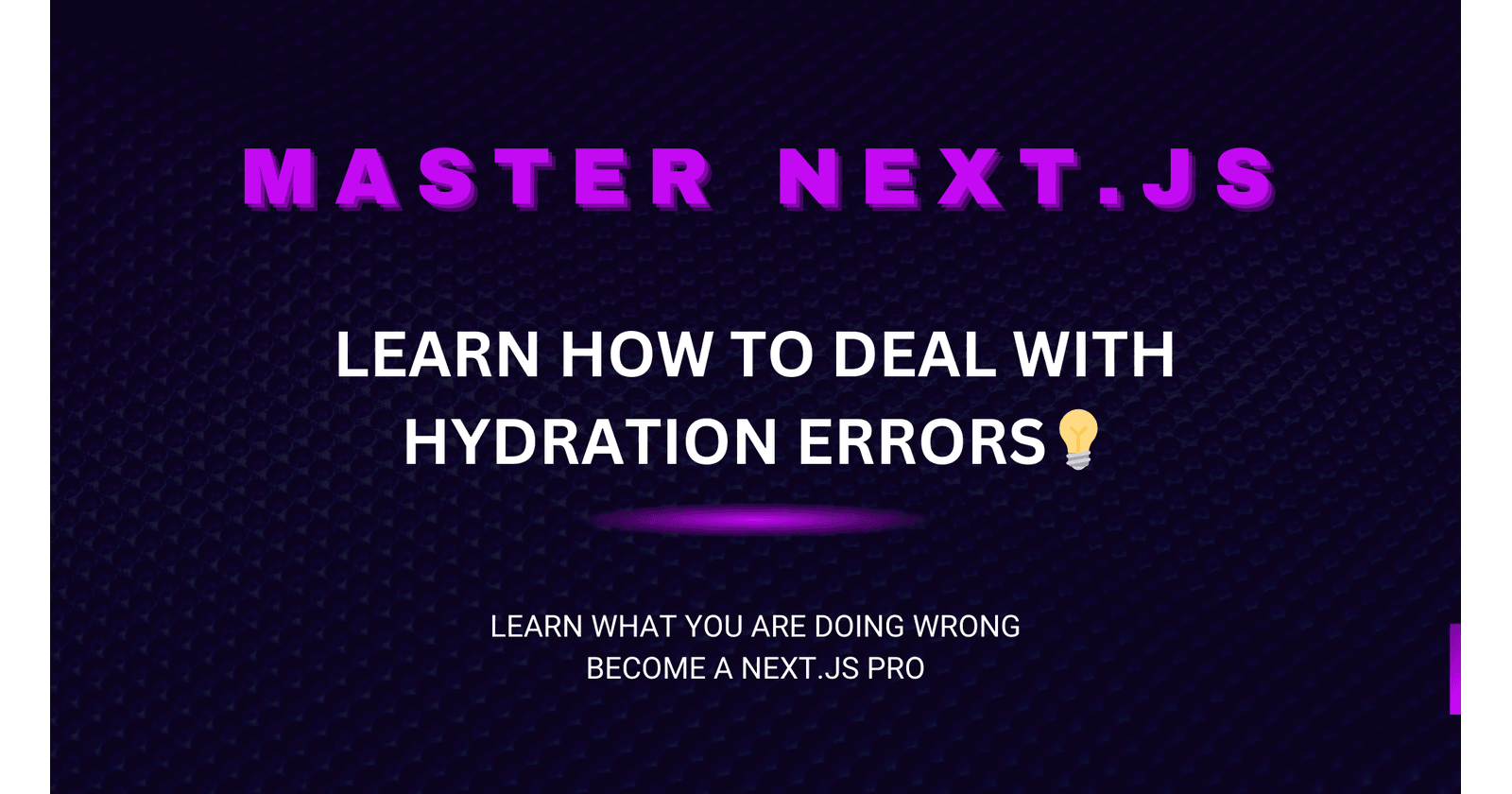
Hydration errors are a common pitfall for developers new to Next.js, especially when dealing with client components that rely on browser APIs like localStorage
. In this article, we’ll explore what hydration errors are, how they occur, and how to fix them effectively, with intuitive code examples to illustrate the concepts.
What Are Hydration Errors?
Hydration errors occur when there’s a mismatch between the HTML rendered on the server and the HTML that React generates on the client after hydration. This can lead to inconsistencies in the UI, resulting in unexpected behavior.
Example Scenario: Using localStorage
Let's say we have a simple client component that checks whether an item is favorited using localStorage
. Here's a basic example:
"use-client"
import { useEffect, useState } from 'react';
const FavoriteButton = () => {
const [isFavorited, setIsFavorited] = useState(false);
// Check localStorage on the client side
useEffect(() => {
if (typeof window !== 'undefined') {
const favorite = localStorage.getItem('favorite');
setIsFavorited(favorite === 'true');
}
}, []);
return (
<button>
{isFavorited ? 'Unfavorite' : 'Favorite'}
</button>
);
};
export default FavoriteButton;
How It Works
Server-Side Rendering: During the initial server render, the component executes, but since
localStorage
is not accessible,isFavorited
remainsfalse
. Thus, the server sends the following HTML to the client:<button>Favorite</button>
Client-Side Hydration: When the component mounts on the client side,
useEffect
runs, and it checkslocalStorage
. If the value istrue
, it updates the state to reflect this, leading to a re-render with:<button>Unfavorite</button>
This mismatch between the server-rendered HTML and the client-rendered HTML results in a hydration error.
Fixing Hydration Errors
Method 1: Suppress Hydration Warnings
If you are okay with knowing that there might be a hydration mismatch but want to suppress the warning, you can use the suppressHydrationWarning
attribute. Here’s how to implement it:
import { useEffect, useState } from 'react';
const FavoriteButton = () => {
const [isFavorited, setIsFavorited] = useState(false);
useEffect(() => {
if (typeof window !== 'undefined') {
const favorite = localStorage.getItem('favorite');
setIsFavorited(favorite === 'true');
}
}, []);
return (
<button suppressHydrationWarning>
{isFavorited ? 'Unfavorite' : 'Favorite'}
</button>
);
};
export default FavoriteButton;
Method 2: Correct HTML Structure
Hydration errors can also occur due to incorrect HTML structure, such as placing a <div>
inside a <p>
tag. If the HTML structure is incorrect, browsers may try to correct it when rendering on the client side, which can result in a mismatch between the server-rendered HTML and the client-rendered HTML. Ensure your HTML follows proper semantic structure. For example:
<!-- Incorrect -->
<p>
<div>This is a div inside a paragraph.</div>
</p>
<!-- Correct -->
<div>
<p>This is a paragraph.</p>
</div>
Conclusion
Hydration errors can be a frustrating issue when developing with Next.js, especially when using client components that interact with browser-specific APIs. By understanding how these errors arise and applying the methods outlined in this article, you can ensure smoother hydration and a better user experience in your applications. Always validate your initial state, maintain correct HTML structure, and feel free to suppress warnings when necessary to keep your development process streamlined.
Subscribe to my newsletter
Read articles from Rishi Bakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rishi Bakshi
Rishi Bakshi
Full Stack Developer with experience in building end-to-end encrypted chat services. Currently dedicated in improving my DSA skills to become a better problem solver and deliver more efficient, scalable solutions.