Mastering Order Processing Systems: A Comprehensive Guide to Building Efficient Business Solutions with Python
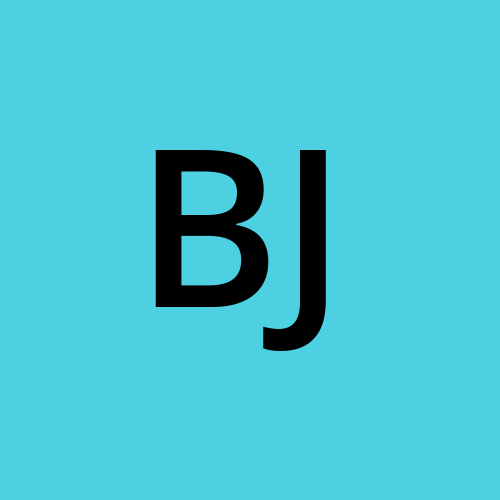
Part 3: Understanding the Basics of Order Processing Systems
Introduction
Order processing systems are the backbone of any business that deals with physical products or services. Whether you’re running an e-commerce platform or a small retail store, an efficient order processing system ensures smooth operations, satisfied customers, and a growing business. In this post, we will dive into the fundamental concepts of order processing systems, exploring how they work and why they are essential for business success.
Definition and Significance of Order Processing Systems
At its core, an order processing system is a series of steps and actions that occur when a customer places an order. The system ensures that the order is captured, validated, processed, and eventually fulfilled, ensuring the customer receives the product or service as expected.
Why Order Processing Systems Matter:
Efficiency and Speed: An automated order processing system reduces the manual effort required, ensuring orders are processed quickly.
Accuracy: It reduces human errors, such as incorrect product selections or quantity mismatches, by automating the verification and validation process.
Scalability: As businesses grow, manual order processing becomes inefficient. Automated systems handle large volumes of orders without any significant increase in workload.
Customer Satisfaction: A seamless order processing system ensures that customers receive their orders on time and in the right condition, improving customer satisfaction and trust.
Typical Workflow of an Order Processing System
The workflow of a typical order processing system can be broken down into several stages. Let’s explore the key stages in more detail:
1. Order Capture
The process starts when a customer places an order. The system captures the necessary details such as the product being ordered, the quantity, customer information, and payment details.
Here is a simplified example of what happens in code during order capture:
order_details = {
"customer_name": "John Doe",
"product_id": "P001",
"quantity": 2
}
The order details are stored in the system, either temporarily or directly in a database.
2. Order Validation
Once the order is captured, it needs to be validated. Validation ensures that:
The products are available in the inventory.
The requested quantity is in stock.
The customer details are correct and complete.
For example, in the system, validation might look like this:
def validate_order(order):
if not order['customer_name'] or not order['product_id'] or not order['quantity'].isdigit():
logging.warning("Validation failed for order: missing or invalid fields")
return False
if int(order['quantity']) <= 0:
logging.warning("Validation failed for order: quantity must be positive")
return False
return True
If the order fails validation, it is flagged, and corrective actions need to be taken before proceeding to the next step.
3. Inventory Management
Inventory management is a crucial component of order processing. The system checks whether the requested quantity is available in stock. If it is, the order moves forward; if not, the system may place the order on hold or send a notification to restock.
For example, once an order is placed, the stock level must be updated. Here’s a snippet showing how the system adjusts the inventory after order placement:
def reduce_stock(cursor, product_id, quantity):
cursor.execute("SELECT stock FROM products WHERE product_id = ?", (product_id,))
current_stock = cursor.fetchone()[0]
if current_stock >= int(quantity):
new_stock = current_stock - int(quantity)
cursor.execute("UPDATE products SET stock = ? WHERE product_id = ?", (new_stock, product_id))
logging.info(f"Updated stock for product {product_id}: {new_stock}")
else:
logging.warning(f"Not enough stock for product {product_id}.")
If the stock falls below a certain threshold, the system can trigger a low stock alert, notifying the admin to restock the inventory.
4. Order Fulfillment
Once the order is validated and inventory is adjusted, the next step is order fulfillment. In this stage:
The product is prepared for shipping or pickup.
The order status is updated in the system to reflect that it is "Shipped" or "Completed."
Here's an example of how the system might update the order status:
def update_order_status(order_id, new_status):
conn = sqlite3.connect('order_system.db')
cursor = conn.cursor()
cursor.execute("UPDATE orders SET order_status = ? WHERE order_id = ?", (new_status, order_id))
conn.commit()
logging.info(f"Order ID {order_id} status updated to {new_status}")
conn.close()
The order fulfillment process ends when the product reaches the customer, and the order status is marked as "Delivered" or "Completed."
Key Components of an Order Processing System
Now that we’ve walked through the workflow, let’s summarize the key components that make up the backbone of an order processing system.
Order Capture:
Responsible for capturing the customer’s order information, including product details, customer information, and payment status.
Forms the entry point for the order processing workflow.
Order Validation:
Ensures that all required order details are present and accurate.
Verifies the availability of products in stock.
Provides feedback if there are any issues with the order.
Inventory Management:
Monitors product stock levels in real-time.
Automatically adjusts inventory quantities when orders are processed.
Sends alerts for low stock or stock shortages.
Order Fulfillment:
Oversees the preparation, packaging, and shipping of orders.
Updates the order status and notifies the customer about their order’s progress.
Completes the order lifecycle once the product is delivered.
Conclusion
An efficient order processing system is crucial for the smooth operation of any business. By understanding the key stages of order capture, validation, inventory management, and fulfillment, businesses can automate and streamline their processes, ensuring timely and accurate order processing. In the upcoming parts of this series, we’ll start implementing these components in Python, beginning with building the user interface.
Stay tuned for the next part, where we will dive into creating the login interface using Tkinter, providing users with secure access to the system.
In this post, we explored the basics of order processing systems and their significance. We broke down the workflow into key stages and components, each of which plays a critical role in ensuring that customer orders are processed efficiently and accurately. With this foundational knowledge, you’re ready to start with Database Design and Initialization in the next part!
Link to My Source code : https://github.com/BryanSJamesDev/-Order-Processing-System-OPS-/tree/main
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
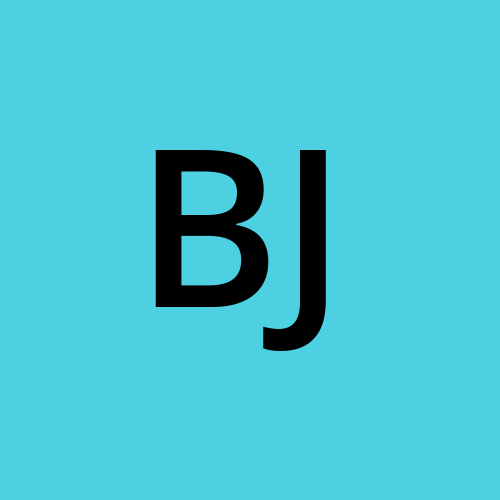