Storage of Web
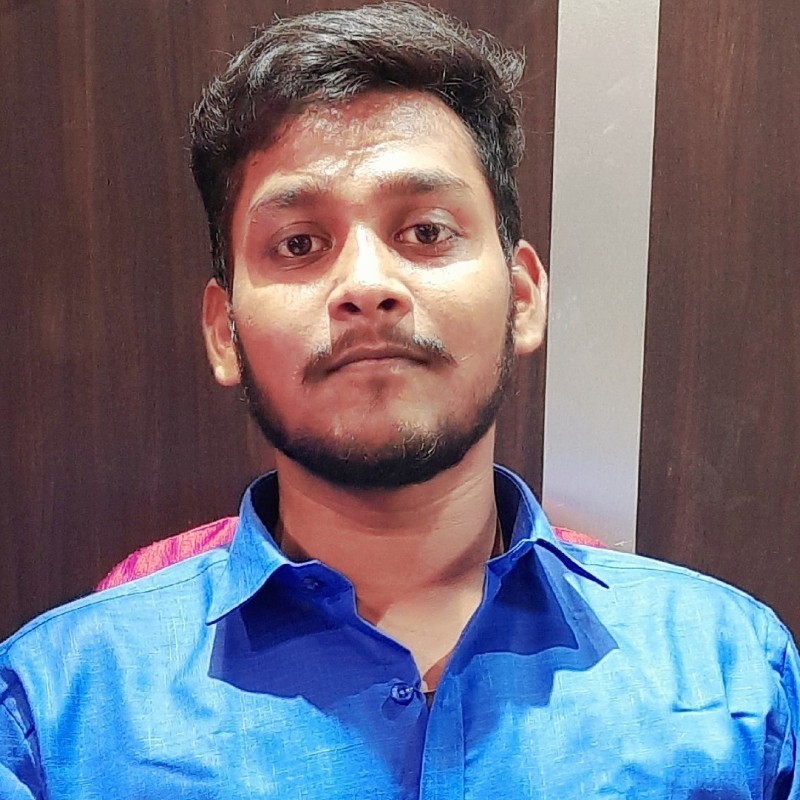
Table of contents
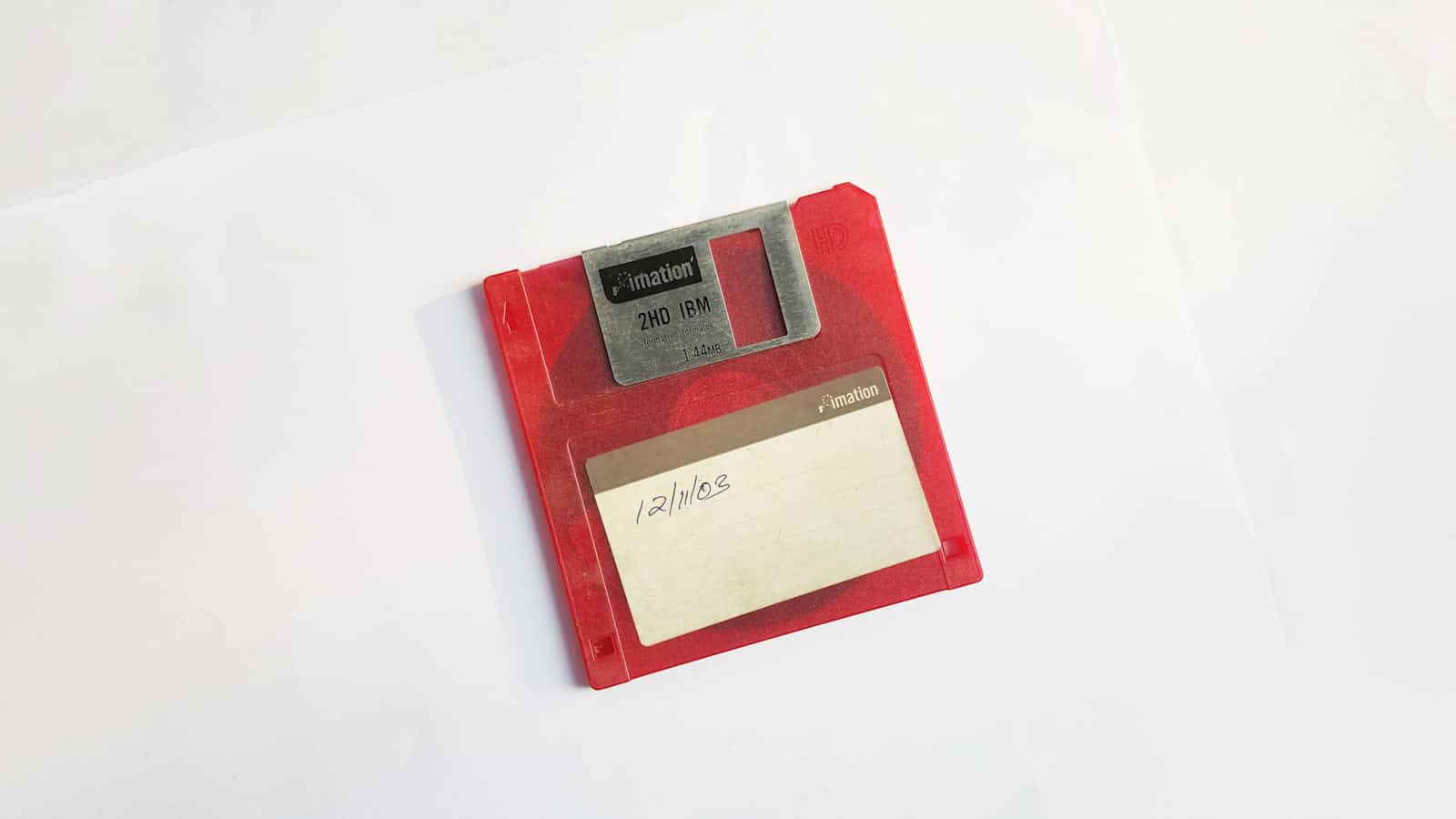
Web storage provides essential benefits to modern application to store data in browser resulting in improved user experience. Sometimes, internet connections can be unreliable or unavailable, which is why offline functionality and reliable performance are common.
Web storage allows data to persist across sessions and store locally without needing a server. Having storage available on the client side is crucial for many standard web features. Using browser cache and other storage techniques can substantially improve the user experience.
Session Storage
Session storage stores data in browser until page session ends i.e sessionStorage
is cleared when the window is closed.
Data can only be accessed from same source i.e its per origin/domain.
Session lifecycle only stays with browser tab and cleared when browser tab is closed.
The storage limit is larger than cookies, around 5MB
Only preferred for storing temporary data like user activities that are required in next actions like user choices, payment info, login sessions, cart choices etc.
Example
// Save data to sessionStorage
sessionStorage.setItem('rememberMe', 'true');
// Get saved data from sessionStorage
let name = sessionStorage.getItem('rememberMe');
Local Storage
Local storage is web storage API that allow sites and apps to store data in key - value pair format in the browser. This data lasts forever, meaning it never expires. Data is stored in browser. This storage is persistent and global.
Data can be only cleared by site functionality or deleted by browser’s settings.
Access to data can be done frequently without making network requests.
Locale storage can store up to 10 MB of data per origin (domain and protocol).
Preferred for storing larger data of apps like auth tokens, preferences or caching when user needs to persist data locally.
Example
// save data in local storage
const user = {
username: 'murali_saran',
remember: true,
darkMode: true,
lang: 'es'
};
localStorage.setItem('details', JSON.stringify(user));
// Fetch your data like:
const user = JSON.parse(localStorage.getItem('details'));
Cookies
Cookie is also type of web storage that is associated with the server i.e either sent by the server in header or set in Javascript. With every HTTP request they are sent server.
Storage limit is very small i.e around 4KB.
Can be triggered by Site functionality as well as server headers.
The Cookie header is optional and may be omitted if, for example, the browser's privacy settings block cookies.
Users shouldn’t always rely on cookies as these can be cleared, disabled or blocked from client side so should be considered using for session details or tracking analytics.
Example
// Set tracking and analytics
document.cookie = "trackingId=123456789#";
IndexedDB
IndexedDB allows you to store data permanently in a user's browser. It enables web applications with advanced query capabilities to function seamlessly, whether online or offline, without relying on network access.
IndexedDB is suitable for storing large amounts of structured data.
Saving large files or search indexes to boost speed and efficiency.
CRUD actions can be executed on the database.
Storing user-generated content and large files in structured data to improve performance.
// Open database
const db = await openDB('files', 1, {
upgrade(db) {
db.createObjectStore('cache');
}
});
// Add file to browser cache
const blob = await fetch('uploaded.jpg').then(r => r.blob());
const transact = db.transaction('cache', 'readwrite');
transact.objectStore('cache').add(blob, 'uploaded.jpg');
// Get cached file
const transact2 = db.transaction('cache');
const file = await transact2.objectStore('cache').get('uploaded.jpg');
For secure web storage, adhere to these guidelines:
Limit storage to non-sensitive data
Always use HTTPS
Enforce access controls
Encrypt any sensitive information
Sanitize input data
Protect against XSS attacks.
By following these practices, developers can safely utilize web storage and reduce potential security risks.
Reference - mdn web docs_
Subscribe to my newsletter
Read articles from ANSH VARUN directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
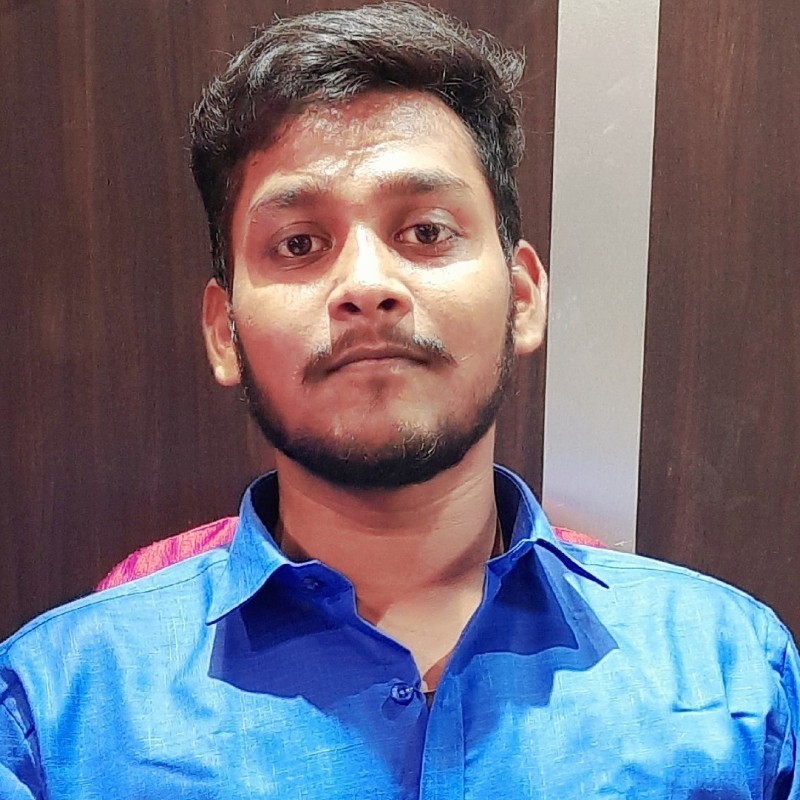
ANSH VARUN
ANSH VARUN
Developing multi platform applications and making process more efficient.