Design Patterns — Facade Patterns

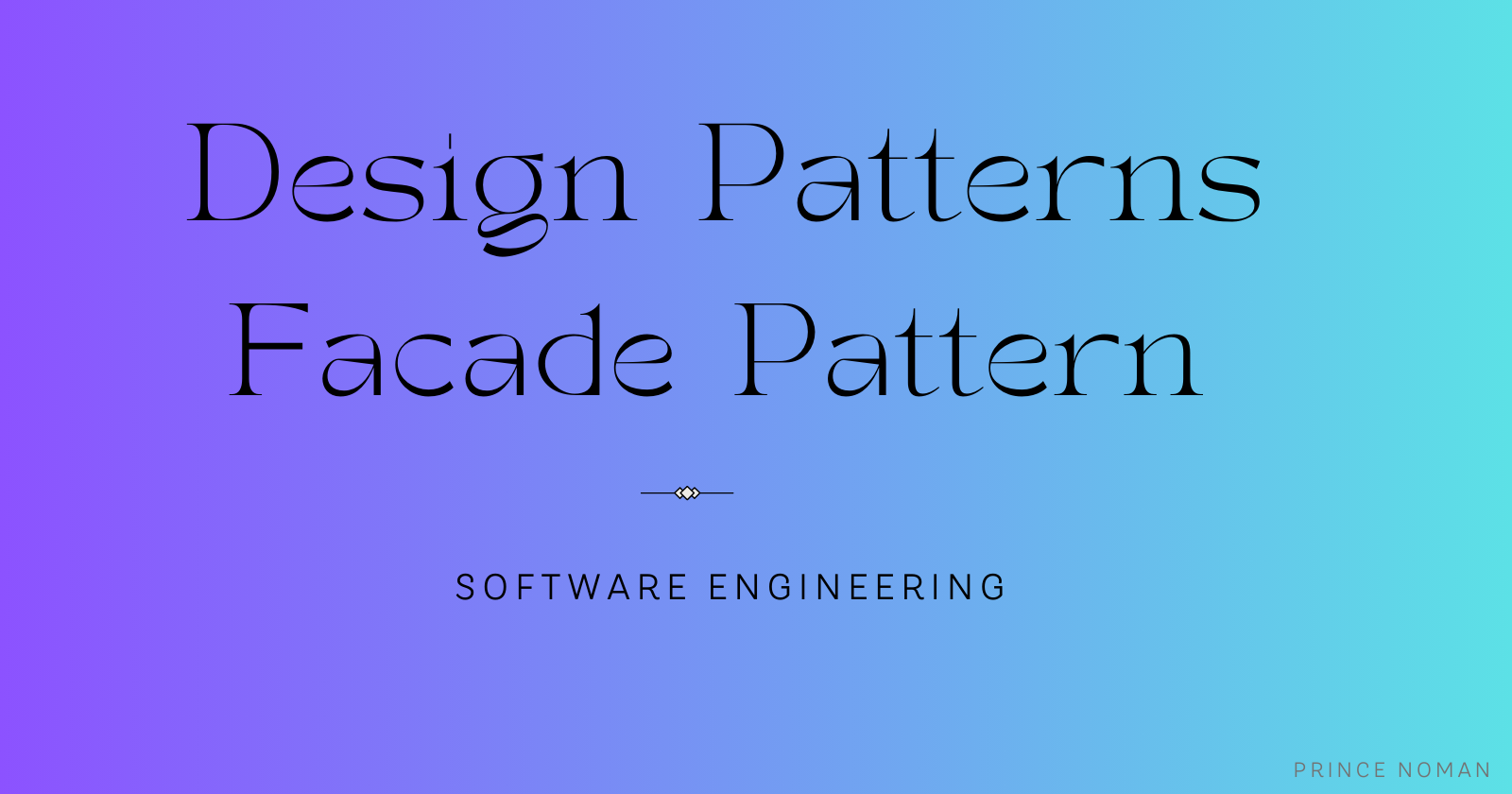
Why Facade Pattern?
The Facade Design Pattern is a structural pattern that provides a simple interface to a complex system or group of classes. This pattern is used when you want to control a complex subsystem through a simplified interface. Essentially, the Facade acts as a wrapper that helps simplify the execution of a particular task or multiple tasks.
This is typically used in systems where you are working with multiple classes or objects but want to handle these tasks in a straightforward manner.
Example of Facade Design Pattern:
Suppose you are creating an Order Processing System that involves different steps like Payment Gateway, Inventory Management, and Shipping Service. You want to handle these subsystems simply. Using the Facade, you can provide a simple interface to manage this complexity.
Step-by-Step Example (PHP):
PaymentGateway Class:
class PaymentGateway {
public function processPayment($amount) {
// Processing payment
echo "Processing payment of $$amount... \n";
}
}
InventoryManagement Class:
class InventoryManagement {
public function updateInventory($productId, $quantity) {
// Updating inventory
echo "Updating inventory for product ID: $productId, Quantity: $quantity \n";
}
}
ShippingService Class:
class ShippingService {
public function arrangeShipping($orderId) {
// Arranging shipping
echo "Arranging shipping for order ID: $orderId \n";
}
}
Now, instead of directly interacting with these three systems, we will create a Facade that manages these tasks together.
OrderFacade Class (Facade):
class OrderFacade {
protected $paymentGateway;
protected $inventoryManagement;
protected $shippingService;
public function __construct() {
$this->paymentGateway = new PaymentGateway();
$this->inventoryManagement = new InventoryManagement();
$this->shippingService = new ShippingService();
}
public function placeOrder($orderId, $productId, $quantity, $amount) {
// Simplifying complex tasks using Facade
$this->paymentGateway->processPayment($amount);
$this->inventoryManagement->updateInventory($productId, $quantity);
$this->shippingService->arrangeShipping($orderId);
echo "Order placed successfully! \n";
}
}
Using the Facade:
// Create an instance of OrderFacade
$orderFacade = new OrderFacade();
// Placing the order
$orderFacade->placeOrder(101, 202, 2, 150);
Output:
Processing payment of $150...
Updating inventory for product ID: 202, Quantity: 2
Arranging shipping for order ID: 101
Order placed successfully!
Analysis:
Here, the OrderFacade
class acts as a Facade. It manages the tasks of PaymentGateway
, InventoryManagement
, and ShippingService
in a simplified way. With the use of Facade, there is no need to directly interact with the individual complex classes.
The benefits of this pattern include:
Decoupling: It creates decoupling between the client and the complex system.
Simple Interface: Provides a simple interface for the user, allowing easy handling of complex tasks.
Code Maintainability: The code becomes easier to manage and modify because the logic is centralized within the Facade.
When to Use Facade:
When working with a complex system and you want to provide a simplified interface to the client.
When you want to reduce communication between various subsystems.
When you want your code to be maintainable and more readable.
Conclusion:
The Facade Design Pattern is very effective for simplifying the use of complex systems. If you need to manage multiple tasks or systems together in a straightforward manner, using a Facade is a good solution.
Subscribe to my newsletter
Read articles from Abdullah Al Noman Prince directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
