#4.0 What XPath: Examples
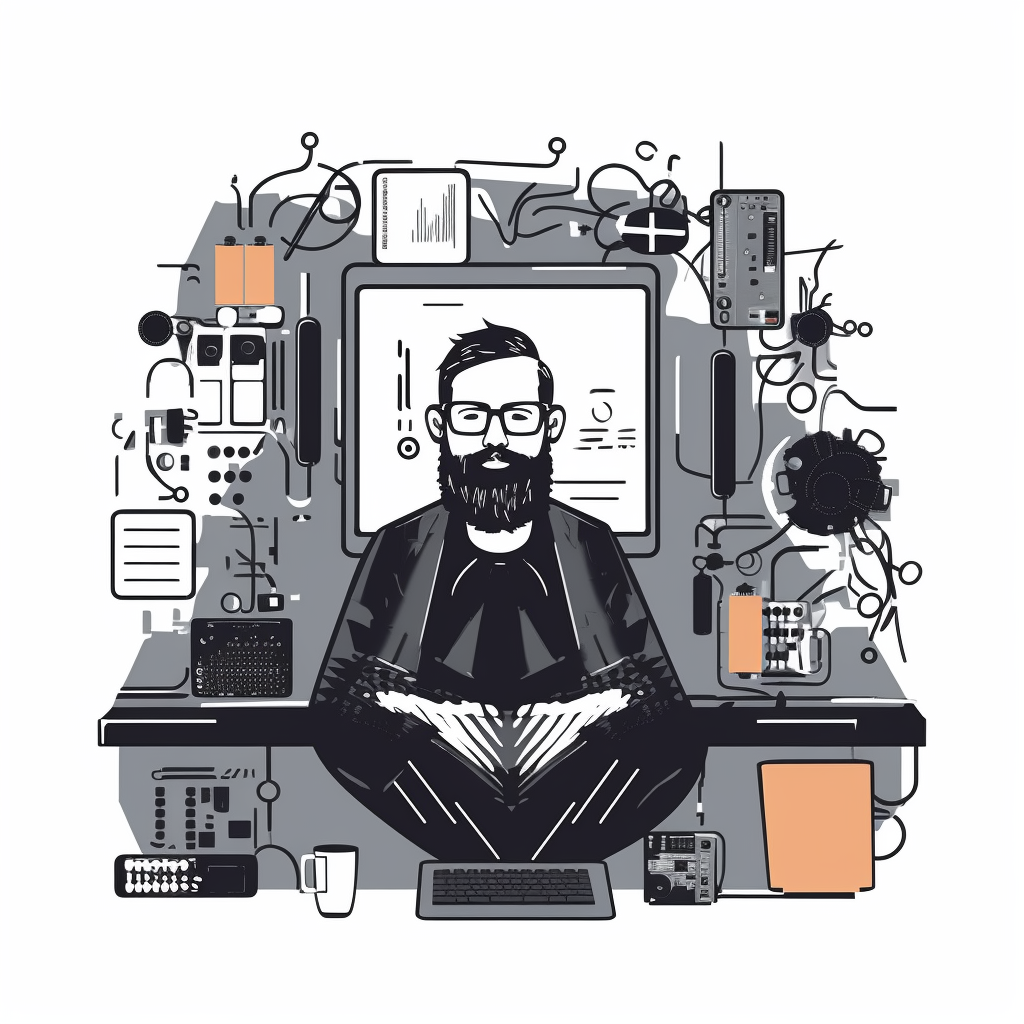
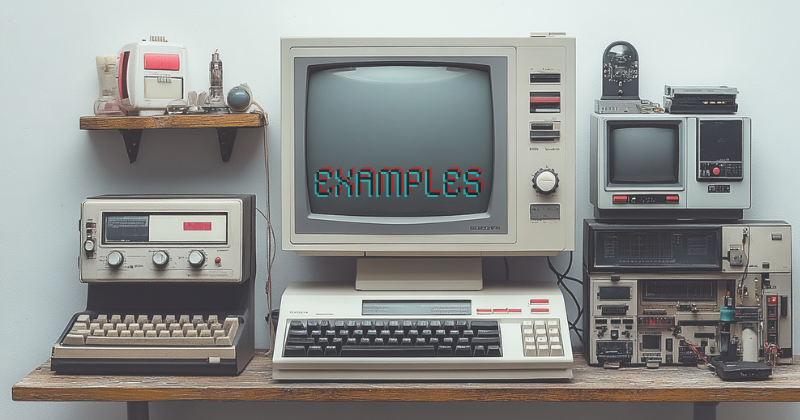
In this section, we'll explore practical examples of using XPath with Selenium WebDriver. We'll use Python for our examples, but the concepts can be applied to other programming languages supported by Selenium.
4.1 Setting Up Selenium with Python
First, ensure you have Selenium and a WebDriver installed. For these examples, we'll use Chrome WebDriver.
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Set up the WebDriver
driver = webdriver.Chrome()
4.2 Basic XPath Usage
Let's start with some basic XPath examples:
# Navigate to a website
driver.get("https://example.com")
# Find an element by its ID
element = driver.find_element(By.XPATH, "//input[@id='username']")
# Find an element by its class
element = driver.find_element(By.XPATH, "//div[@class='error-message']")
# Find an element by its name attribute
element = driver.find_element(By.XPATH, "//input[@name='password']")
# Find an element by its text content
element = driver.find_element(By.XPATH, "//button[text()='Log In']")
4.3 Working with Forms
XPath is particularly useful when working with forms:
# Fill out a login form
driver.find_element(By.XPATH, "//input[@id='username']").send_keys("myusername")
driver.find_element(By.XPATH, "//input[@id='password']").send_keys("mypassword")
driver.find_element(By.XPATH, "//button[contains(text(), 'Log In')]").click()
# Check if login was successful
success_message = driver.find_element(By.XPATH, "//div[contains(@class, 'success-message')]")
assert "Welcome" in success_message.text
4.4 Handling Dynamic Elements
XPath can be used to locate elements with dynamic attributes:
# Wait for a dynamic element to appear
wait = WebDriverWait(driver, 10)
dynamic_element = wait.until(EC.presence_of_element_located((By.XPATH, "//div[starts-with(@id, 'message-')]")))
# Find an element with a partially matching class
element = driver.find_element(By.XPATH, "//div[contains(@class, 'user-profile')]")
# Find an element based on its sibling
element = driver.find_element(By.XPATH, "//label[text()='Email']/following-sibling::input")
4.5 Navigating Complex Structures
XPath excels at navigating complex DOM structures:
# Find a specific cell in a table
cell = driver.find_element(By.XPATH, "//table[@id='data']//tr[3]/td[2]")
# Find all items in a dropdown
dropdown_items = driver.find_elements(By.XPATH, "//select[@id='country']/option")
# Find a parent element
parent = driver.find_element(By.XPATH, "//input[@id='email']/parent::div")
# Find an ancestor element
form = driver.find_element(By.XPATH, "//input[@id='email']/ancestor::form")
4.6 Using XPath Functions
XPath functions can be powerful tools for locating elements:
# Find elements with text longer than 10 characters
long_text_elements = driver.find_elements(By.XPATH, "//p[string-length(text()) > 10]")
# Find elements with a specific text, ignoring case
case_insensitive = driver.find_element(By.XPATH, "//button[translate(text(), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ', 'abcdefghijklmnopqrstuvwxyz') = 'submit']")
# Find the last element in a list
last_item = driver.find_element(By.XPATH, "(//ul[@id='menu']/li)[last()]")
# Find elements based on numerical attribute values
expensive_items = driver.find_elements(By.XPATH, "//div[@class='product'][number(@data-price) > 100]")
4.7 Handling AJAX-loaded Content
When dealing with AJAX-loaded content, you often need to wait for elements to appear:
# Wait for an AJAX-loaded element
wait = WebDriverWait(driver, 10)
ajax_element = wait.until(EC.presence_of_element_located((By.XPATH, "//div[@id='ajax-content']//p")))
# Click a button to load more content, then wait for it
driver.find_element(By.XPATH, "//button[text()='Load More']").click()
wait.until(EC.presence_of_element_located((By.XPATH, "//div[@class='new-content']")))
4.8 Combining XPath with Other Selenium Features
XPath can be combined with other Selenium features for more complex interactions:
from selenium.webdriver.common.action_chains import ActionChains
# Hover over a menu item and click a submenu item
menu = driver.find_element(By.XPATH, "//li[@id='products-menu']")
submenu = driver.find_element(By.XPATH, "//li[@id='products-menu']//a[text()='Laptops']")
actions = ActionChains(driver)
actions.move_to_element(menu).click(submenu).perform()
# Handle an alert after clicking a button
driver.find_element(By.XPATH, "//button[text()='Delete Account']").click()
alert = wait.until(EC.alert_is_present())
alert.accept()
Remember to always close the WebDriver when you're done:
driver.quit()
These examples demonstrate the versatility and power of XPath in Selenium WebDriver. By mastering XPath, you can create robust and flexible web automation scripts capable of handling a wide variety of scenarios.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
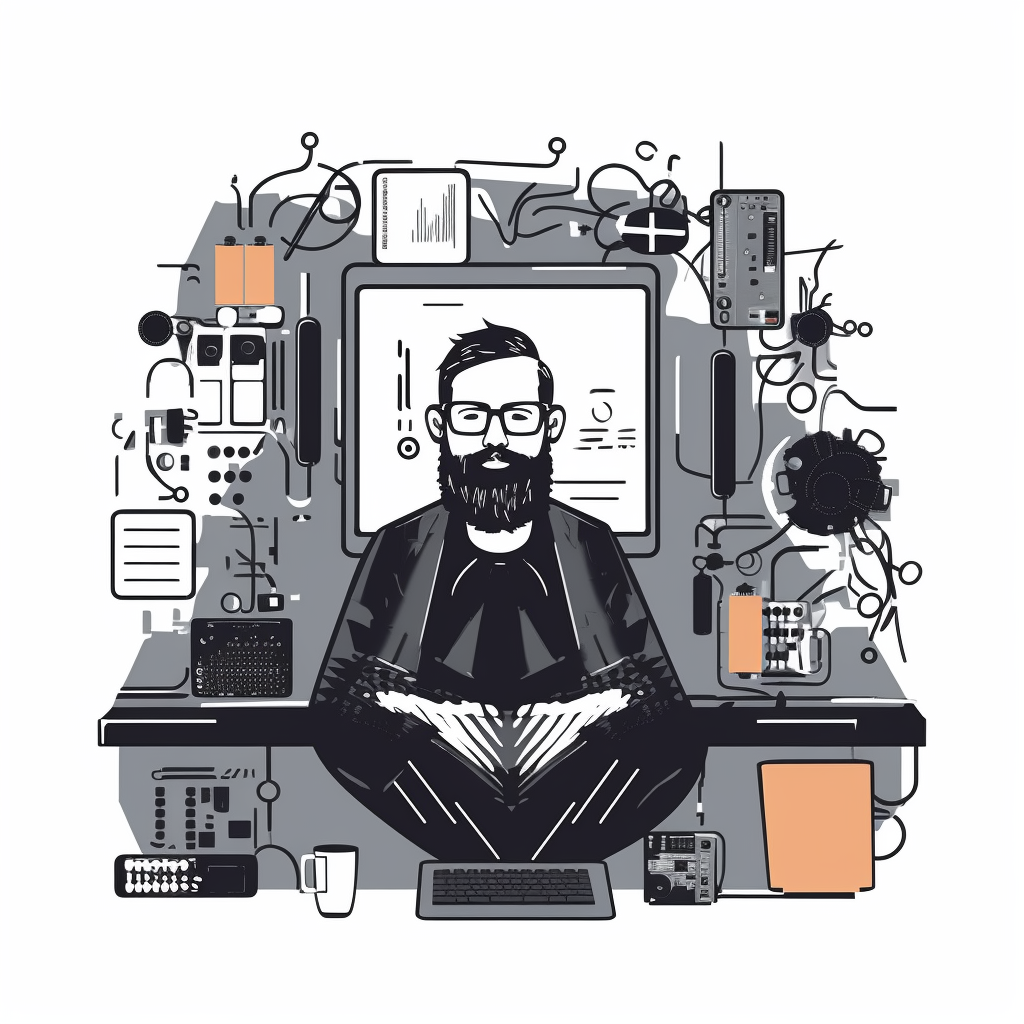
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.