Weekly JavaScript Insights: Sets and Maps Explored on My Coding Journey

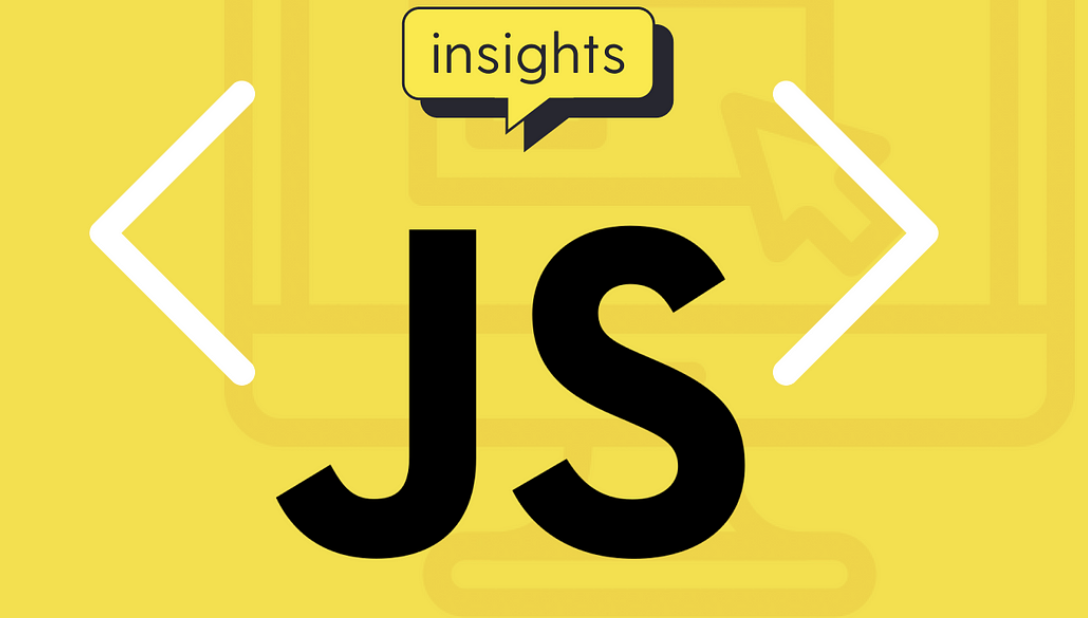
Hey guys! This week, I learned Sets and Maps in JavaScript. It was really interesting to learn the concepts and how JavaScript manages them. In my weekly post, I will share with you what I learned, problems I faced and how I managed to resolve those.
1)Sets
A Set is an unordered collection of unique objects; that is, it has no duplicated elements. It contains add, delete, and the existence operations. In this example, I learnt how to create a set and also how it does not accept duplicate values.
in addition to this i can also create a set directly my using its constructor
output :
To check if an element is present in a set, we have the Set.has()
method, which takes the element as input. This method returns a boolean value.
It can be accessed through the size property of Set.size
, which returns an integer value. In place of attaching the screenshot, I'll just write this code here to save your time.
const myArray=[1,2,3,4,52,1,3,7,78];
const uniqueElements=new Set(myArray);
console.log(uniqueElements.size);
2)Maps
A map can store key-value pairs. It can use any value, even objects or primitives as keys or values.
const person=new Map();
person.set('firstname','Aniket');
person.set("age",7);
person.set(1,"one");
console.log(person);
output:
Map(3) {'firstname' => 'Aniket', 'age' => 7, 1 => 'one'}
A big difference between keys in objects and keys in maps is that in objects, keys are strings whereas in maps, the keys can be objects.
console.log(typeof(person.keys()));
output is
object
Destructuring example with the Map
for(let [key,value] of person)
{
console.log(key," , ",value);
}
//output
firstname , Aniket
age , 7
1 ' , ' 'one'
Adding Object to an Map
This was quite interesting as we can directly add an object to a map and then access the properties of that object from the map.
const extraInfo=new Map();
extraInfo.set(personDetails,{age:24,gener:"male"});
console.log(extraInfo.get(personDetails).age); // output will be 24
Conclusion:
This week I toured the really exciting world of JavaScript sets and maps. Sets and maps have given me the ability to deal quite efficiently with data due to the unique values a collection has in sets and the flexible key-value pairs a map has. Well, I faced some difficulties, but with lots of practice and explorations, I was able to overcome them and get a good grip on things. Part of the learning journey is how I managed to find solutions in trying to teach myself. Hopefully, it will help many others too. Having learned more about my JavaScript basics, I look forward with a lot of eagerness to kick-start some new projects and see how I could use them to implement what I've learned so far. To stay updated, please do subscribe to my newsletter!
Subscribe to my newsletter
Read articles from Aniket Mogare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
