Essential Bash Commands for DevOps Professionals: A Comprehensive Guide

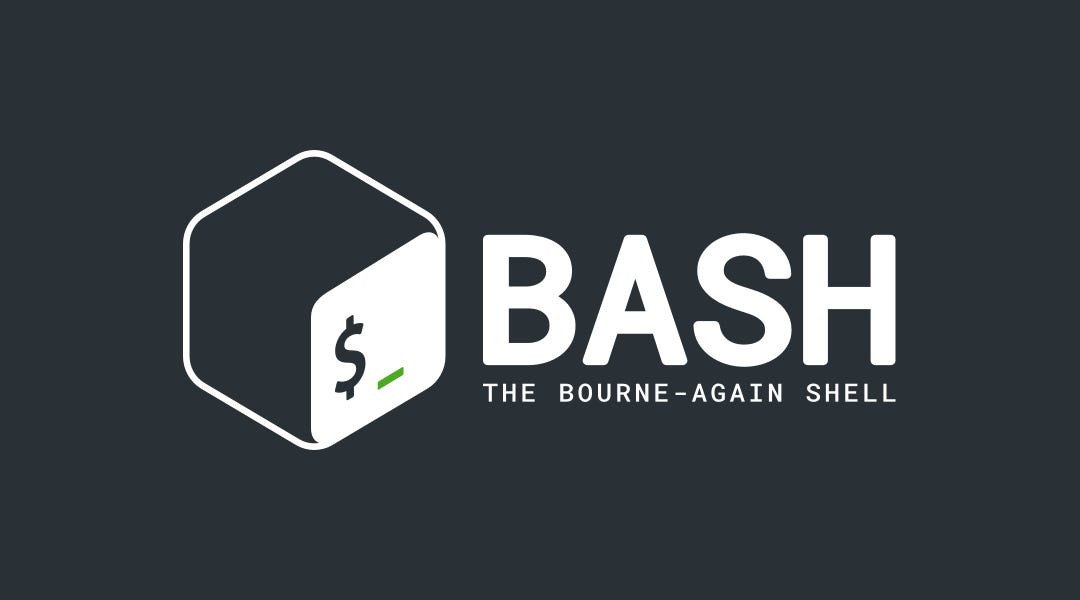
In the fast-paced world of DevOps, efficiency and automation are paramount. At the heart of many DevOps workflows lies Bash (Bourne Again Shell), a powerful command-line interface that enables developers and system administrators to perform tasks swiftly and reliably. Mastering basic Bash commands is essential for anyone looking to excel in DevOps, as these commands form the foundation for scripting, automation, and system management.
In this blog post, we'll explore some of the most essential Bash commands that every DevOps professional should know. Whether you're automating deployments, managing infrastructure, or troubleshooting systems, these commands will enhance your productivity and streamline your workflows.
1. Introduction to Bash
Bash is a Unix shell and command language that serves as the default shell on many Linux distributions and macOS. It allows users to interact with the operating system by executing commands, running scripts, and automating tasks. For DevOps professionals, Bash is an indispensable tool for managing servers, deploying applications, and orchestrating complex workflows.
Why Bash?
Automation: Automate repetitive tasks with scripts.
Flexibility: Combine multiple commands to perform complex operations.
Integration: Seamlessly integrate with other tools and services.
Accessibility: Available on most Unix-like systems.
2. Navigating the File System
Understanding how to navigate the file system is fundamental to using Bash effectively.
pwd
- Print Working Directory
Displays the current directory you are in.
pwd
# Output: /home/user/projects
cd
- Change Directory
Navigate between directories.
cd /var/www/html
Go up one directory:
cd ..
Go to the home directory:
cd ~
ls
- List Directory Contents
Lists files and directories within the current directory.
ls
# Output: file1.txt file2.sh directory1
Detailed listing:
ls -la
List with human-readable file sizes:
ls -lh
3. File and Directory Operations
Managing files and directories is a daily task in DevOps.
mkdir
- Make Directory
Creates a new directory.
mkdir new_project
Create nested directories:
mkdir -p projects/devops/scripts
touch
- Create Empty File
Creates a new empty file or updates the timestamp of an existing file.
touch README.md
cp
- Copy Files and Directories
Copies files or directories from one location to another.
cp source.txt destination.txt
Copy a directory recursively:
cp -r src/ backup/src_backup/
mv
- Move or Rename Files and Directories
Moves or renames files and directories.
mv old_name.txt new_name.txt
Move a file to a different directory:
mv file.txt /var/www/html/
rm
- Remove Files or Directories
Deletes files or directories.
rm unwanted_file.txt
Remove a directory recursively:
rm -rf old_directory/
find
- Search for Files and Directories
Searches for files and directories based on various criteria.
find /var/log -name "*.log"
Find files larger than 100MB:
find / -type f -size +100M
4. Text Processing and Manipulation
Processing text files is common in configuration management, logging, and data analysis.
cat
- Concatenate and Display Files
Displays the contents of a file.
cat server.log
grep
- Search Text Using Patterns
Searches for patterns within files.
grep "ERROR" application.log
Case-insensitive search:
grep -i "warning" system.log
Search recursively in directories:
grep -r "timeout" /var/www/html/
awk
- Pattern Scanning and Processing
Processes and analyzes text files.
awk '{print $1, $3}' data.csv
Sum a column of numbers:
awk '{sum += $2} END {print sum}' numbers.txt
sed
- Stream Editor for Filtering and Transforming Text
Performs basic text transformations on an input stream.
sed 's/localhost/127.0.0.1/g' config.conf > updated_config.conf
Delete lines containing a pattern:
sed '/DEBUG/d' application.log > production.log
sort
and uniq
- Sort and Remove Duplicate Lines
Sorts lines in a file and removes duplicates.
sort access.log | uniq -c | sort -nr
Sort a file alphabetically:
sort names.txt
Remove duplicate lines:
uniq duplicates.txt unique.txt
5. Process Management
Managing processes ensures that applications run smoothly and resources are utilized efficiently.
ps
- Report a Snapshot of Current Processes
Displays information about active processes.
ps aux
Filter processes by name:
ps aux | grep nginx
top
and htop
- Dynamic Real-Time Process Viewer
Monitors system processes and resource usage in real-time.
top
Using
htop
(requires installation):htop
kill
- Terminate Processes
Sends signals to processes to terminate them.
kill -9 12345
Gracefully terminate a process:
kill -15 12345
nohup
and &
- Run Processes in the Background
Runs a command that continues executing after logging out.
nohup long_running_script.sh &
6. Networking Commands
Networking is integral to DevOps for managing servers, deploying applications, and ensuring connectivity.
ping
- Check Network Connectivity
Tests the reachability of a host.
ping google.com
curl
and wget
- Transfer Data from or to a Server
Downloads files or interacts with web APIs.
curl -O https://example.com/file.zip
wget https://example.com/file.zip
Send a POST request with JSON data:
curl -X POST -H "Content-Type: application/json" -d '{"key":"value"}' https://api.example.com/data
ssh
- Secure Shell for Remote Login
Connects to remote servers securely.
ssh user@server_ip
Execute a remote command:
ssh user@server_ip 'ls -la /var/www/html'
scp
and rsync
- Securely Copy Files Between Hosts
Transfers files securely over SSH.
scp local_file.txt user@remote_host:/path/to/destination/
sync -avz source/ user@remote_host:/path/to/destination/
Sync directories with
rsync
:rsync -avz /local/dir/ user@remote_host:/remote/dir/
netstat
and ss
- Network Statistics
Displays network connections, routing tables, and interface statistics.
netstat -tuln
ss -tuln
List all listening ports:
ss -tuln | grep LISTEN
7. Package Management
Managing software packages is essential for maintaining system stability and security.
For Debian-Based Systems (apt
)
Update package lists:
sudo apt update
Upgrade installed packages:
sudo apt upgrade
Install a package:
sudo apt install nginx
Remove a package:
sudo apt remove nginx
For RPM-Based Systems (yum
or dnf
)
Update packages (using yum
):
sudo yum update
Update packages (using dnf
):
sudo dnf update
Install a package:
sudo yum install httpd
or
sudo dnf install httpd
Remove a package:
sudo yum remove httpd
or
sudo dnf remove httpd
Universal Package Managers
Snap:
sudo snap install node
Homebrew (Linuxbrew):
brew install terraform
8. Version Control with Git
Version control is crucial for managing code and configurations.
git clone
- Clone a Repository
Creates a local copy of a remote repository.
git clone https://github.com/user/repo.git
git status
- Check Repository Status
Displays the state of the working directory and staging area.
git status
git add
- Stage Changes
Adds changes to the staging area.
git add .
git commit
- Commit Changes
Records staged changes to the repository.
git commit -m "Add new feature"
git push
- Push Changes to Remote
Uploads local commits to the remote repository.
git push origin main
git pull
- Fetch and Merge Changes
Fetches and integrates changes from the remote repository.
git pull origin main
9. Scripting Basics
Automation is at the core of DevOps, and Bash scripting is a powerful tool for automating tasks.
Creating a Simple Bash Script
Create a script file:
nano deploy.sh
Add the following content:
#!/bin/bash echo "Starting deployment..." git pull origin main sudo systemctl restart nginx echo "Deployment completed."
Make the script executable:
chmod +x deploy.sh
Run the script:
./deploy.sh
Variables and Control Structures
#!/bin/bash
ENVIRONMENT=$1
if [ "$ENVIRONMENT" == "production" ]; then
echo "Deploying to production..."
# Production deployment commands
elif [ "$ENVIRONMENT" == "staging" ]; then
echo "Deploying to staging..."
# Staging deployment commands
else
echo "Usage: $0 {production|staging}"
exit 1
fi
Looping Through Files
#!/bin/bash
for file in /var/www/html/*.html
do
echo "Processing $file"
# Process the file
done
Functions
#!/bin/bash
backup_database() {
mysqldump -u user -p database > backup.sql
echo "Database backed up successfully."
}
deploy_application() {
git pull origin main
sudo systemctl restart app.service
echo "Application deployed successfully."
}
backup_database
deploy_application
10. Best Practices and Tips
To maximize the effectiveness of Bash in DevOps, consider the following best practices:
1. Use Meaningful Variable Names
Enhance readability by using descriptive variable names.
backup_directory="/var/backups"
2. Handle Errors Gracefully
Check for command success and handle failures.
#!/bin/bash
mkdir /new_directory
if [ $? -ne 0 ]; then
echo "Failed to create directory."
exit 1
fi
Alternatively, use set -e
to exit on any error.
#!/bin/bash
set -e
mkdir /new_directory
cp source.txt /new_directory/
3. Comment Your Scripts
Provide explanations for complex logic to aid future maintenance.
#!/bin/bash
# Update package lists
sudo apt update
# Upgrade all packages
sudo apt upgrade -y
4. Use Functions for Reusability
Organize scripts into functions to promote reusability and clarity.
deploy_application() {
git pull origin main
sudo systemctl restart app.service
}
deploy_application
5. Secure Your Scripts
Avoid hardcoding sensitive information. Use environment variables or secure storage solutions.
#!/bin/bash
DB_PASSWORD=${DB_PASSWORD}
mysqldump -u user -p$DB_PASSWORD database > backup.sql
6. Leverage Shellcheck
Use tools like Shellcheck to analyze scripts for potential errors and improvements.
shellcheck deploy.sh
11. Conclusion
Bash remains an essential tool in the DevOps toolkit, enabling professionals to automate tasks, manage systems, and streamline workflows efficiently. By mastering these basic Bash commands and incorporating them into your daily operations, you'll enhance your ability to deliver robust, scalable, and reliable solutions.
Remember, while Bash provides a powerful foundation, continuous learning and practice are key to unlocking its full potential. Explore advanced scripting techniques, integrate Bash with other tools, and stay updated with the latest best practices to excel in your DevOps journey.
Embrace the power of Bash, automate with confidence, and elevate your DevOps workflows to new heights!
Subscribe to my newsletter
Read articles from Prasant Jakhar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
