How Does the Null Coalescing Operator Improve JavaScript Readability?

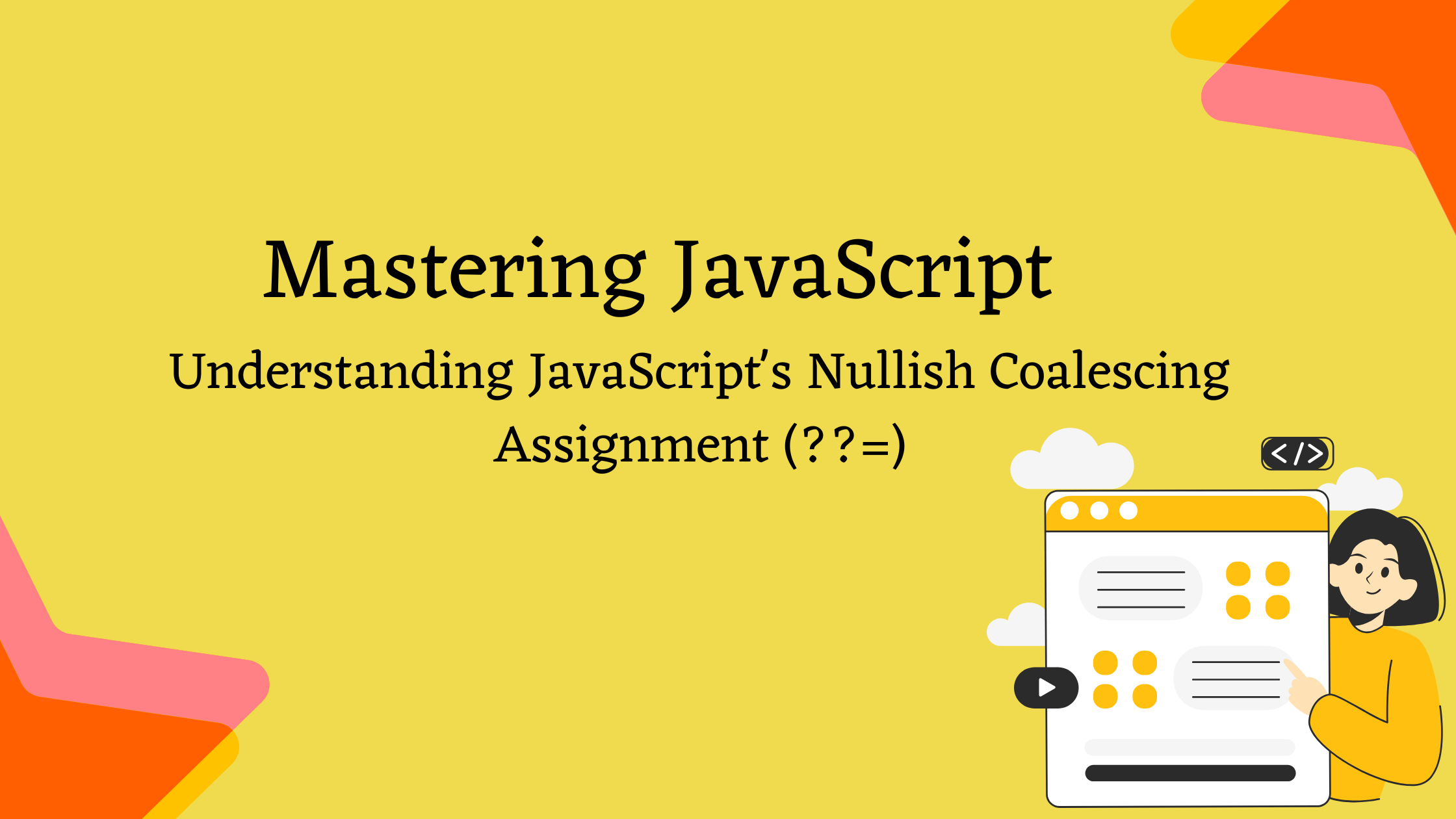
JavaScript continues to evolve, introducing new features that enhance its usability and make developers' lives easier. One of these recent additions is the Nullish Coalescing Assignment operator (??=), introduced in ECMAScript 2021. In this blog post, we’ll explore where it came from, why it’s useful, and how to implement it in real-world scenarios.
Where it came from?
The Nullish Coalescing Assignment operator is a combination of two important JavaScript features: the nullish coalescing operator (??) and the assignment operator (=).
Nullish Coalescing Operator (??): This operator returns the right-hand operand when the left-hand operand is
null
orundefined
, making it a useful tool for setting default values.For example:
let x = null; let y = x ?? 'default value'; // y will be 'default value'
The nullish coalescing assignment combines this functionality with assignment, allowing for a more concise way to set a variable only if it’s currently null or undefined.
Why use it?
Before the introduction of ??=, you might have seen code that used the logical OR operator (||) to set default values. However, the logical OR operator treats falsy values (like 0
, ""
, or false
) as needing a replacement, which can lead to unintended results. The nullish coalescing operator, on the other hand, is focused only on null
and undefined
, making it more precise.
The nullish coalescing assignment operator allows you to write cleaner, more readable code. It streamlines the process of assigning values, especially when dealing with optional configurations or defaults.
Use Cases in Production
Let's look at a practical example to understand how and when to use the ??= operator in a real-world scenario.
Example: Setting Default Configuration
Imagine you're building a function that accepts user settings, but you want to ensure that certain settings have default values if not provided:
function setupUserSettings(userSettings) {
// Default settings
userSettings.theme ??= 'light';
userSettings.notifications ??= true;
userSettings.language ??= 'en';
console.log(userSettings);
}
let settings1 = { theme: null, notifications: false };
setupUserSettings(settings1);
// Output: { theme: 'light', notifications: false, language: 'en' }
let settings2 = { notifications: undefined };
setupUserSettings(settings2);
// Output: { theme: 'light', notifications: true, language: 'en' }
In the setupUserSettings
function, we use ??= to assign default values for theme
, notifications
, and language
.
If
theme
isnull
, it gets assigned 'light'.If
notifications
isundefined
, it gets assignedtrue
.If
language
isn’t provided, it defaults to 'en'.
Notice how notifications
retains its value of false
, demonstrating the operator's precision. This ensures we only overwrite null
or undefined
values, making our code cleaner and more predictable.
Conclusion
The Nullish Coalescing Assignment operator (??=) is a valuable addition to JavaScript, allowing developers to write cleaner, more concise code when dealing with default values. By focusing solely on null
and undefined
, it avoids the pitfalls of treating all falsy values the same way. As you continue to develop in JavaScript, incorporating this operator can lead to more robust and maintainable code, especially when managing configurations and defaults.
Try it out in your next project and see how it can simplify your code!
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Darshit Anjaria
Darshit Anjaria
I’m a problem solver at heart, driven by the idea of building solutions that genuinely make a difference in people’s everyday lives. I’m always curious, always learning, and always looking for ways to improve the world around me through thoughtful, impactful work. Beyond building, I love giving back to the community — whether it’s by sharing what I’ve learned through blogs, tutorials, or helpful insights. My goal is simple: to make technology a little more accessible and useful for everyone. Let’s learn, build, and grow together.