Automating MSI Uninstalls with PowerShell for .NET MAUI Fix
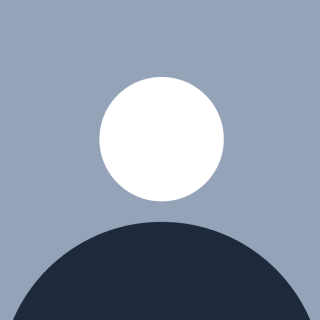
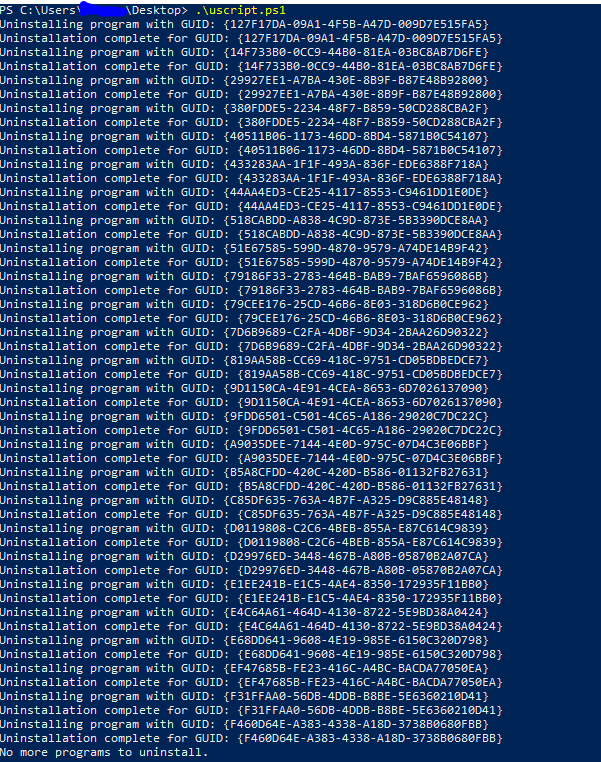
Recently, I found myself in a pickle trying to install .NET MAUI on my laptop, so I did a bit of searching and found out it was apparently a common issue.
Problem: Can't locate .NET MAUI Workloads
According to Microsoft's troubleshooting page, when dealing with the ‘Can’t locate the .NET MAUI workloads' problem:
Uninstall the .NET MAUI workloads through the CLI.
Uninstall any .NET SDKs in Control Panel.
Uninstall the .NET MAUI workloads in Visual Studio.
Automating the Process with PowerShell
After taking the steps above, I realized there were still leftover SDKs on my machine.
These leftover SDKs can conflict with newer installations, especially when installing workloads like .NET MAUI. Running the following reg query
command helps identify these 'hidden' SDKs that aren't visible in traditional uninstallation methods, allowing for a more thorough cleanup.
reg query HKLM\SOFTWARE\Microsoft\Windows\currentversion\uninstall\ -s -f manifest
Executing the command showed me this:
Which meant I had to uninstall each of these 52 SDKs with the msiexec
command below until the reg query
command returned 0 matches.
msiexec /x {GUID} /q IGNOREDEPENDENCIES=ALL
After uninstalling about 11 SDKs, I realized this manual approach wasn’t exactly productive as it was time consuming and I spelt ‘DEPENDENCIES’ wrong a couple of times. So, I wrote a PowerShell script to automate it with the help of this reddit ask and ChatGPT. By automating the process, the repetitive task of uninstalling each GUID is done without error or wasted time.
Here’s the script that saved me minutes:
PowerShell Script to Batch Uninstall MSI Files
# Function to run the reg query and return the result
function Get-InstalledPrograms {
# Run the reg query and return the output
$output = & reg query HKLM\SOFTWARE\Microsoft\Windows\currentversion\uninstall\ -s -f manifest
return $output
}
# Function to uninstall programs using msiexec
function Uninstall-Program {
param (
[string]$guid
)
# Uninstall using msiexec with the specific command and flags
Write-Host "Uninstalling program with GUID: $guid"
Start-Process "msiexec.exe" -ArgumentList "/x $guid /q IGNOREDEPENDENCIES=ALL" -Wait
Write-Host "Uninstallation complete for GUID: $guid"
}
# Loop to keep running reg query until no results are returned
do {
# Get the list of installed programs
$installedPrograms = Get-InstalledPrograms
# Define a list to store GUIDs for uninstallation
$msiGuids = @()
# Extract the GUIDs from the reg query output
foreach ($line in $installedPrograms) {
if ($line -match "({[A-Z0-9\-]+})") {
$msiGuids += $matches[1] # Extract the GUID from the match
}
}
# If there are no GUIDs left, stop the loop
if ($msiGuids.Count -eq 0) {
Write-Host "No more programs to uninstall."
break
}
# Loop through each extracted GUID and uninstall the program
foreach ($guid in $msiGuids) {
Uninstall-Program -guid $guid
}
} while ($msiGuids.Count -gt 0)
Steps to Use the Script:
Run the PowerShell script:
Save the script to a
.ps1
file.Open PowerShell as Administrator.
Run the script:
.\your_script.ps1
.
This is where I ran into an error due to the execution policy in PowerShell, which controls the level of script execution allowed on your system. PowerShell execution policies act as a security mechanism to prevent malicious scripts from running on your system.
Change the PowerShell Execution Policy (if needed)
I first checked the current execution policy by running this in PowerShell:
By default, it is set to the 'Restricted' policy which blocks all scripts. I then changed the execution policy to RemoteSigned
(allows you to run local scripts but still blocks scripts downloaded from the internet unless they are signed) using the following command in PowerShell as Administrator:
Then I ran the script again and this time….
It worked!
I was then finally able to reinstall .NET MAUI through Visual Studio (after uninstalling and reinstalling Visual Studio and Visual Studio Installer as a whole though 😅).
Note: I set my powershell execution policy back to restricted after running the script.
Thank you Microsoft Docs + ChatGPT + Reddit! (and sorry for the blurry screenshots).
If you read this till the end, I hope you have learned something useful! 😊✨
Subscribe to my newsletter
Read articles from emptycodes directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by