FormGroup and FormControl in Angular

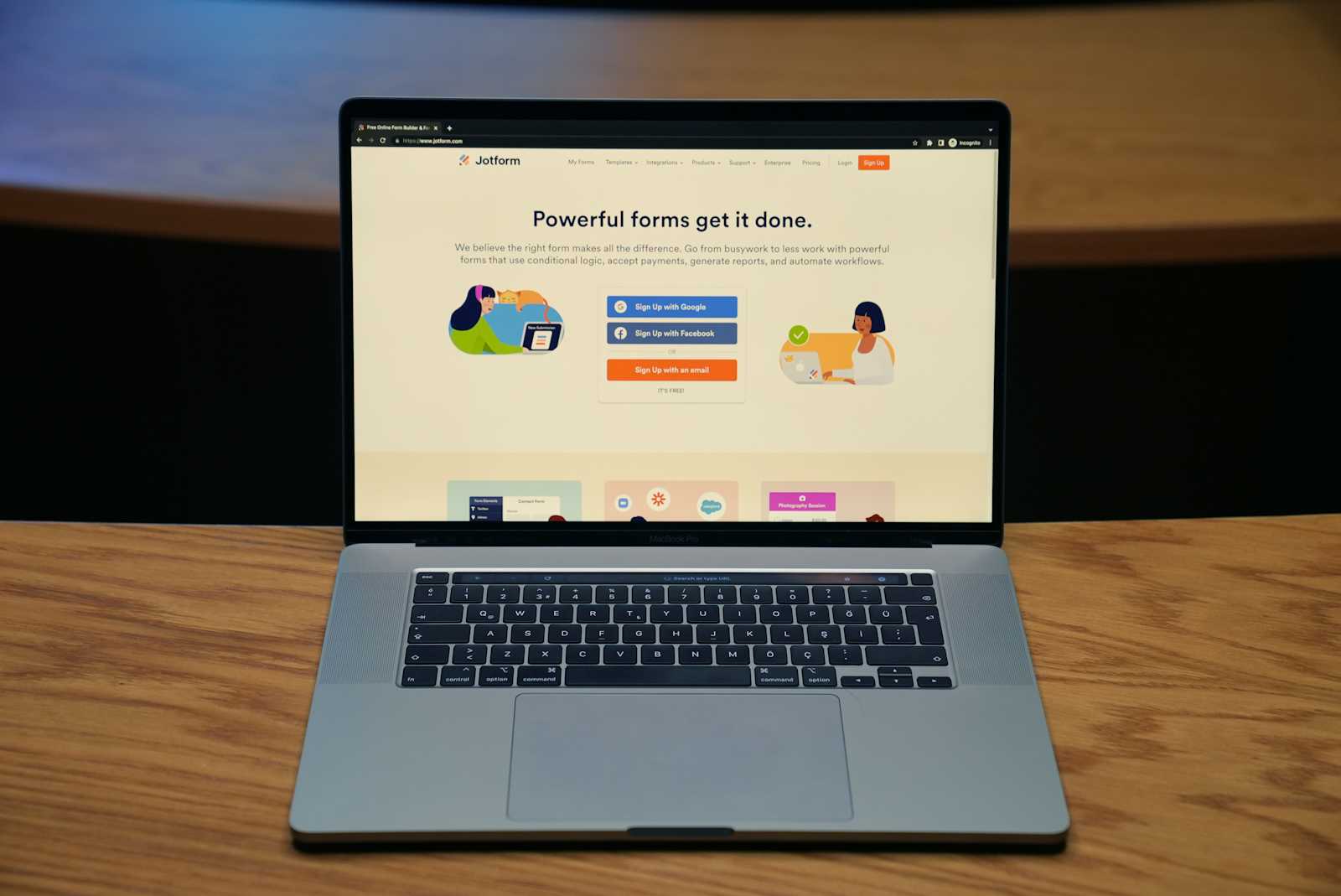
If you’re making a web app, you will need to capture information from your user sooner or later. Fortunately, reactive forms in Angular allow you to create clean forms without using too many directives. They also reduce the need for end-to-end testing as validating your forms is very easy.
Put simply, form controls in Angular give the developer all the control, and nothing is implicit anymore — every choice about inputs and controls must be made intentionally and explicitly. In this tutorial, we’ll show you how to divide form controls by form groups to create clusters that provide a platform to easily access the template element as groups. To illustrate the concept of form groups in Angular, we’ll walk through the process of building a reactive form so that you can fully understand how to set it up.
To follow along, download the starter project on GitHub and open it in VS Code. If you haven’t already, you’ll want to update to the most recent version at the time of writing, Angular v18.
What are form controls in Angular?
In Angular, form controls are fundamental building blocks. They hold both data values and validation rules for individual form elements. Every form input should be bound to a corresponding form control to enable data tracking and validation.
What is a form group in Angular?
Form groups wrap a collection of form controls. Just as the control gives you access to the state of an element, the group gives you the same access but to the state of the wrapped controls. Every form control in the form group is connected to the appropriate form control in the component code.
FormControl
and FormGroup
in Angular
FormControl
is a class in Angular that tracks the value and validation status of an individual form control. Along with FormGroup
and FormArray
, it is one of the three essential building blocks in Angular forms. As an extension of AbstractControl
, FormControl
provides access to the value, validation status, user interactions, and relevant events.
A FormGroup
in Angular is a container that aggregates multiple FormControl
instances. It tracks the values and validation states of the child from controls, organizing them into a single object using their respective names as keys. It calculates its status by reducing the status values of its children so that if one control in a group is invalid, the entire group is rendered invalid.
Strongly typed forms in Angular
Before Angular 14, you couldn’t specify a type for a FormControl
. This could lead to confusion at runtime over what type was expected to be in a form.
Since Angular 14, FormControl
is strongly typed by default. This allows you to specify the expected data type when creating a FormControl
. We’ll demonstrate this with a form example in a moment.
Registering form groups in Angular
To use FormGroup
in your Angular component, begin by importing it inside a component.
To see how this works, open the employee.component.ts
file and paste the code block below:
import { Component, OnInit } from '@angular/core';
import { FormControl, FormGroup } from '@angular/forms'
@Component({
selector: 'app-employee',
templateUrl: './employee.component.html',
styleUrls: ['./employee.component.css']
})
export class EmployeeComponent implements OnInit {
bioSection = new FormGroup({
firstName: new FormControl<string>(''),
lastName: new FormControl<string>(''),
age: new FormControl<number|null>(null)
});
constructor() { }
ngOnInit() {
}
}
Here, the form group was imported and initialized to group some form controls that compose the bio section of the form. To link the group to the view, we need to associate the model to the view with the form group name, like this:
<form [formGroup]="bioSection">
<label>
First Name:
<input type="text" [formControl]="bioSection.controls.firstName" >
</label>
<label>
Last Name:
<input type="text" [formControl]="bioSection.controls.lastName">
</label>
<label>
Age:
<input type="text" [formControl]="bioSection.controls.age">
</label>
<button type="submit">Submit Application</button>
</form>
We reference the FormGroup
created in code, and then directly specify the control to use in the FormControl
directive.
Your app.component.html
file should look like this:
<div style="text-align:center">
<h2>Angular Job Board </h2>
<app-employee></app-employee>
</div>
Now run your application in development with this command:
ng server
Subscribe to my newsletter
Read articles from himanshu ladva directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

himanshu ladva
himanshu ladva
Innovative Software Engineer with Strong Coding and Problem- Solving Skills. Passionate about Developing Cutting-Edge Solutions that Drive Business Success and Enhance User Experience.