Model Selection in Machine Learning: GridSearchCV, RandomizedSearchCV, and TPOT

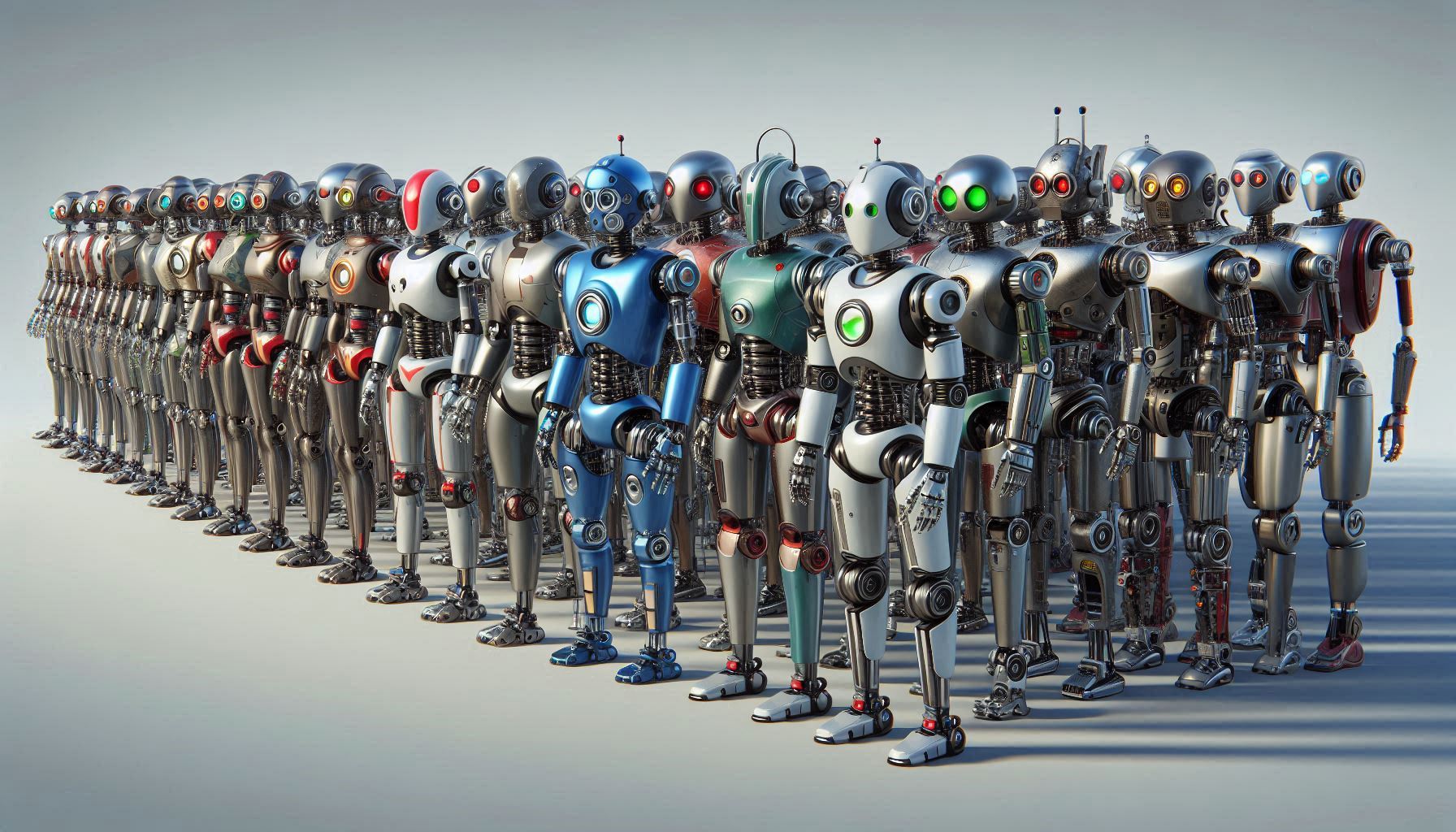
In machine learning, selecting the right model and tuning its hyperparameters is a critical step toward achieving optimal performance. Several techniques exist to assist in this process, ranging from exhaustive searches like GridSearchCV
to more randomized methods like RandomizedSearchCV
. Automated tools like TPOT
take it further by searching for the best models and pipelines using evolutionary algorithms. This article will cover the key aspects of these model selection techniques, their strengths, and practical implementations.
1. GridSearchCV
The GridSearchCV
method from Scikit-learn is a popular choice for finding the best model by searching through every possible combination of hyperparameters in a grid. Though computationally expensive, it guarantees that the best possible set of parameters is selected.
Key Features:
Exhaustive Search: Tests all combinations of hyperparameters.
Guaranteed Optimal Result: Provides the best model given the parameter grid.
Computational Cost: As the grid size increases, so does the computational cost, making it less efficient for large datasets or many parameters.
Example Code:
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
# Create a RandomForestClassifier
grid_rf_class = GridSearchCV(
estimator=RandomForestClassifier(criterion='gini'),
param_grid={'max_depth': [2, 4, 8, 15], 'max_features': ['auto', 'sqrt']},
scoring='roc_auc', # Metric to evaluate the model performance
n_jobs=4, # Number of cores to use for parallel processing
cv=5, # Number of folds in cross-validation
refit=True, # Refit the best model on the entire dataset
return_train_score=True # Get training score for analysis
)
# Fit the model on training data
grid_rf_class.fit(X_train, y_train)
# Retrieve the best parameters and estimator
best_params = grid_rf_class.best_params_
best_estimator = grid_rf_class.best_estimator_
Advantages of GridSearchCV
:
Thorough: Evaluates every combination of hyperparameters, ensuring the best one is selected.
Cross-validation: Ensures model performance is robust by using cross-validation.
Best for Smaller Grids: Works well when the hyperparameter space is small or computational resources are ample.
2. RandomizedSearchCV
Unlike GridSearchCV
, RandomizedSearchCV
selects hyperparameter combinations at random, which makes it less computationally expensive but does not guarantee the best result. However, it is effective when dealing with larger datasets or a vast hyperparameter space where an exhaustive search is infeasible.
Key Features:
Random Sampling: Samples a given number of parameter combinations.
Efficient: Less computationally intensive compared to
GridSearchCV
.Not Guaranteed: Does not always find the optimal model but balances between cost and performance.
Example Code:
from sklearn.model_selection import RandomizedSearchCV
from sklearn.ensemble import GradientBoostingClassifier
import numpy as np
# Define the model and hyperparameter space
random_GBM_class = RandomizedSearchCV(
estimator=GradientBoostingClassifier(),
param_distributions={'learning_rate': np.linspace(0.1, 2, 150),
'min_samples_leaf': list(range(20, 65))},
n_iter=10, # Number of random samples
scoring='accuracy', # Scoring metric
n_jobs=4, # Number of cores for parallel processing
cv=5, # Cross-validation folds
refit=True, # Refit the best model
return_train_score=True # Return training scores
)
# Fit the model on the training data
random_GBM_class.fit(X_train, y_train)
# Print specific results, like learning rates tested
print(random_GBM_class.cv_results_['param_learning_rate'])
Advantages of RandomizedSearchCV
:
Less Expensive: Reduces computational cost by randomly sampling hyperparameters.
Faster: Ideal for large datasets or when exploring a large hyperparameter space.
Adequate Performance: Often provides close-to-optimal results with fewer iterations.
3. TPOTClassifier
TPOTClassifier
from the tpot
library automates the model selection process using genetic algorithms. It not only tunes the hyperparameters but also searches for the best model pipeline, combining different preprocessing steps and model algorithms.
Key Features:
Automated ML: Automates the entire machine learning pipeline.
Evolutionary Algorithm: Utilizes genetic algorithms to evolve and improve the model pipeline.
Customizable: Allows you to specify the number of generations, population size, and scoring metric.
Example Code:
from tpot import TPOTClassifier
# Define a TPOT classifier
tpot_clf = TPOTClassifier(
generations=2, # Number of iterations in the search process
population_size=4, # Number of models to evaluate in each generation
offspring_size=3, # Number of models created through mutation and crossover
scoring='accuracy', # Evaluation metric
cv=2, # Number of cross-validation folds
verbosity=2, # Controls the amount of logging output
random_state=99 # Seed for reproducibility
)
# Fit the TPOT pipeline on the training data
tpot_clf.fit(X_train, y_train)
# Export the best pipeline as Python code
tpot_clf.export('best_pipeline.py')
Advantages of TPOTClassifier
:
Fully Automated: Finds the best machine learning pipeline without manual intervention.
Pipeline Search: Includes preprocessing steps and model tuning, providing a complete solution.
Reproducible: The generated Python code allows you to reproduce the best model.
Conclusion
Selecting the best model and hyperparameters is an essential task in machine learning, and various methods like GridSearchCV
, RandomizedSearchCV
, and TPOT
serve different needs.
Use GridSearchCV when you want an exhaustive search and can afford the computational cost.
Use RandomizedSearchCV for larger hyperparameter spaces where efficiency is more important than perfection.
Use TPOTClassifier if you're looking for a fully automated, evolutionary approach to find the best model pipeline.
Each of these methods helps improve the accuracy and robustness of your machine learning models by selecting the most appropriate parameters for your data.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.