How to add Font Awesome to an Angular project

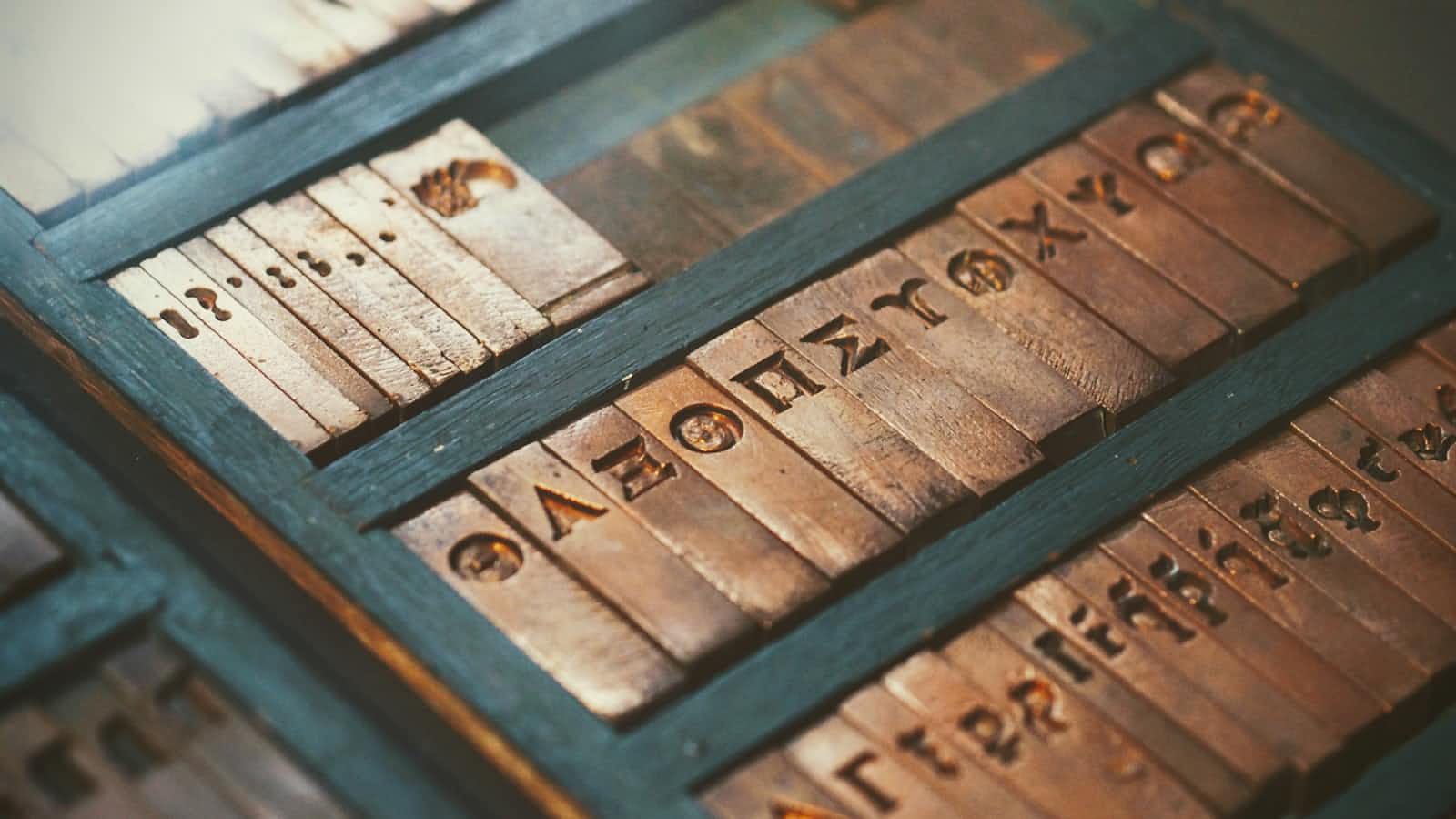
In this article, we will be working through how to use Font Awesome in an Angular app and how we can use Font Awesome’s icon animation and styling.
Before we move further, let’s talk about what Font Awesome is.
Font Awesome
Font Awesome is an icon toolkit with over 1,500 free icons that are incredibly easy to use. The icons were created using a scalable vector and inherit CSS sizes and color when applied to them. This makes them high-quality icons that work well on any screen size.
Before the release of Angular 5, developers had to install the Font Awesome package and reference its CSS in an Angular project before it could be used.
But the release of Angular 5 made it easy to implement Font Awesome in our project by the creation of the Angular component for Font Awesome. With this feature, Font Awesome can be integrated into our projects cleanly.
Font Awesome icons blend with text inline well due to their scalability. In this article, we are going to explore more on how to also use animation, coloring, and sizing for Font Awesome icons.
Creating a demo Angular app
Let’s create a demo Angular app for this tutorial. Open your terminal, CD to the project directory, and run the command below.
Before you run the command, make sure you have Node.js installed in your system and also have Angular CLI installed, too:
ng new angular-fontawesome
Installing Font Awesome dependencies
For those who have an existing project, we can follow up from here. Once the above command is done, CD to the project directory and install the below Font Awesome icon command:
npm install @fortawesome/angular-fontawesome
npm install @fortawesome/fontawesome-svg-core
npm install @fortawesome/free-brands-svg-icons
npm install @fortawesome/free-regular-svg-icons
npm install @fortawesome/free-solid-svg-icons
# or
yarn add @fortawesome/angular-fontawesome
yarn add @fortawesome/fontawesome-svg-core
yarn add @fortawesome/free-brands-svg-icons
yarn add @fortawesome/free-regular-svg-icons
yarn add @fortawesome/free-solid-svg-icons
Using Font Awesome icons in Angular applications
There are two steps to using Font Awesome in an Angular project. We’re going to look at both of these:
How to use Font Awesome icons at the components level
How to use the Font Awesome icons library
How to use Font Awesome icons at the components level
This step has to do with using Font Awesome icons at the component level, and it is not a good approach because it involves us importing icons into each of our components that needs an icon and also importing the same icons multiple times.
We are still going to take a look at how we can use icons in a component in case we are building an application that requires us to use an icon in just one component.
After installing Font Awesome, open the app.module.ts
and import the FontAwesomeModule
just like the one below:
import { FontAwesomeModule } from '@fortawesome/angular-fontawesome'
imports: [
BrowserModule,
AppRoutingModule,
FontAwesomeModule
],
After that, open app.component.ts
and import the icon name that you want to use. Let’s say we want to make use of faCoffee
:
import { faCoffee } from '@fortawesome/free-solid-svg-icons';
Next, we create a variable name called faCoffee
and assign the icon that we imported to the variable so can use it in the app.component.html
. If we don’t do that, we can’t use it:
faCoffee = faCoffee;
Now, in the app.component.html
, write the code below:
<div>
<fa-icon ="faCoffee"></fa-icon>
</div>
Run the command to serve our app and to check if our icon is showing:
ng serve
If we look at our webpage, we will see faCoffee
displayed on the screen. That shows that the icon was installed and imported successfully.
Subscribe to my newsletter
Read articles from himanshu ladva directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

himanshu ladva
himanshu ladva
Innovative Software Engineer with Strong Coding and Problem- Solving Skills. Passionate about Developing Cutting-Edge Solutions that Drive Business Success and Enhance User Experience.