Linear Search
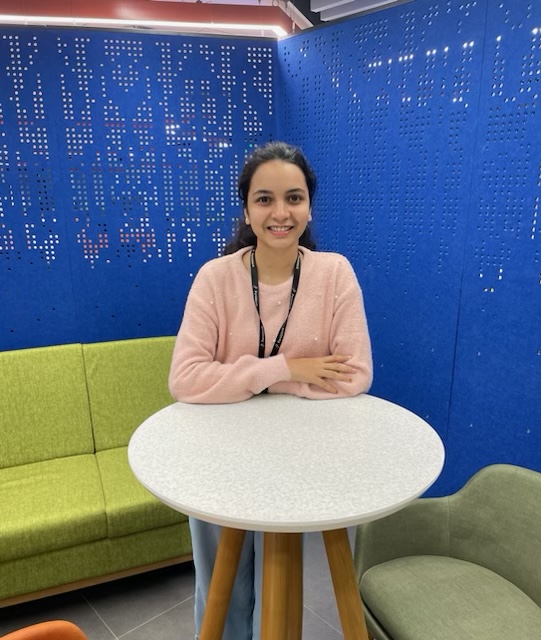
Table of contents
Linear search, also known as sequential search is used to find a specific value withing an array or a list. It checks each element one by one until desired element is found and end of the list is reached.
How it works ?
Start from first element in the array.
Compare the number to be found with each element in array by using for loop from index 0 to array length - 1.
If element is found return index.
If element is not found return -1.
Code
import java.util.Arrays;
class LinearSearch {
public static void main(String[] args) {
int[] array = {20, 40, 48, 50, 45};
linearSearch(array, 48);
}
static int linearSearch(int[] array, int numberToFind) {
for (int i = 0; i < array.length; i++) {
if(array[i] == numberToFind) {
System.out.println("Number found at index : " + i);
return i;
}
}
System.out.println("Number not found");
return -1;
}
}
Output
java -cp /tmp/mlKZBqbmts/LinearSearch
Number found at index : 2
=== Code Execution Successful ===
Characteristics:
Time Complexity : O(n) where n is the number of elements in array. In worst case, every element will be checked.
Space complexity : O(1) as it uses constant amount of additional space.
Linear search is most useful for small and unsorted arrays or lists.
Subscribe to my newsletter
Read articles from Juhilee Nazare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
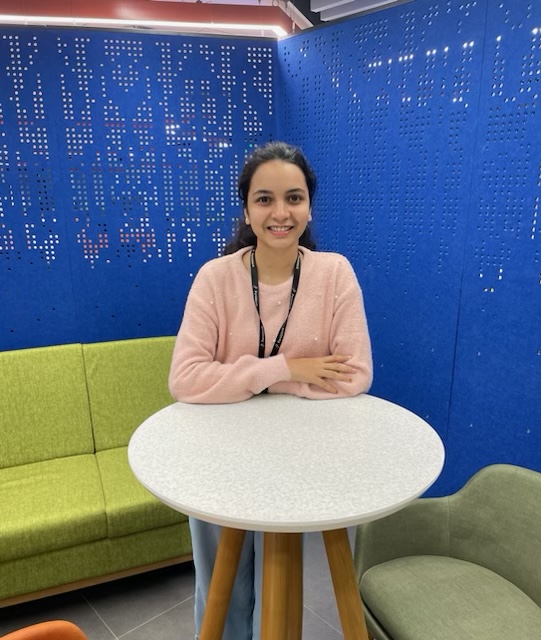
Juhilee Nazare
Juhilee Nazare
I've 3.5+ years of experience in software development and willing to share my knowledge while working on real-time IT solutions.