Binary Search
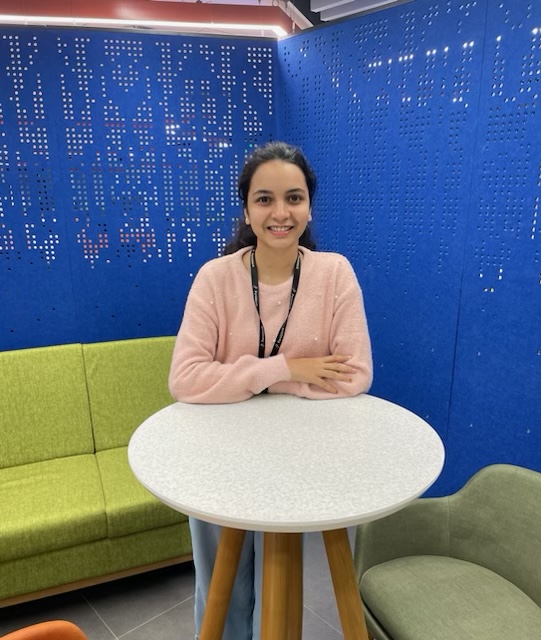
Table of contents
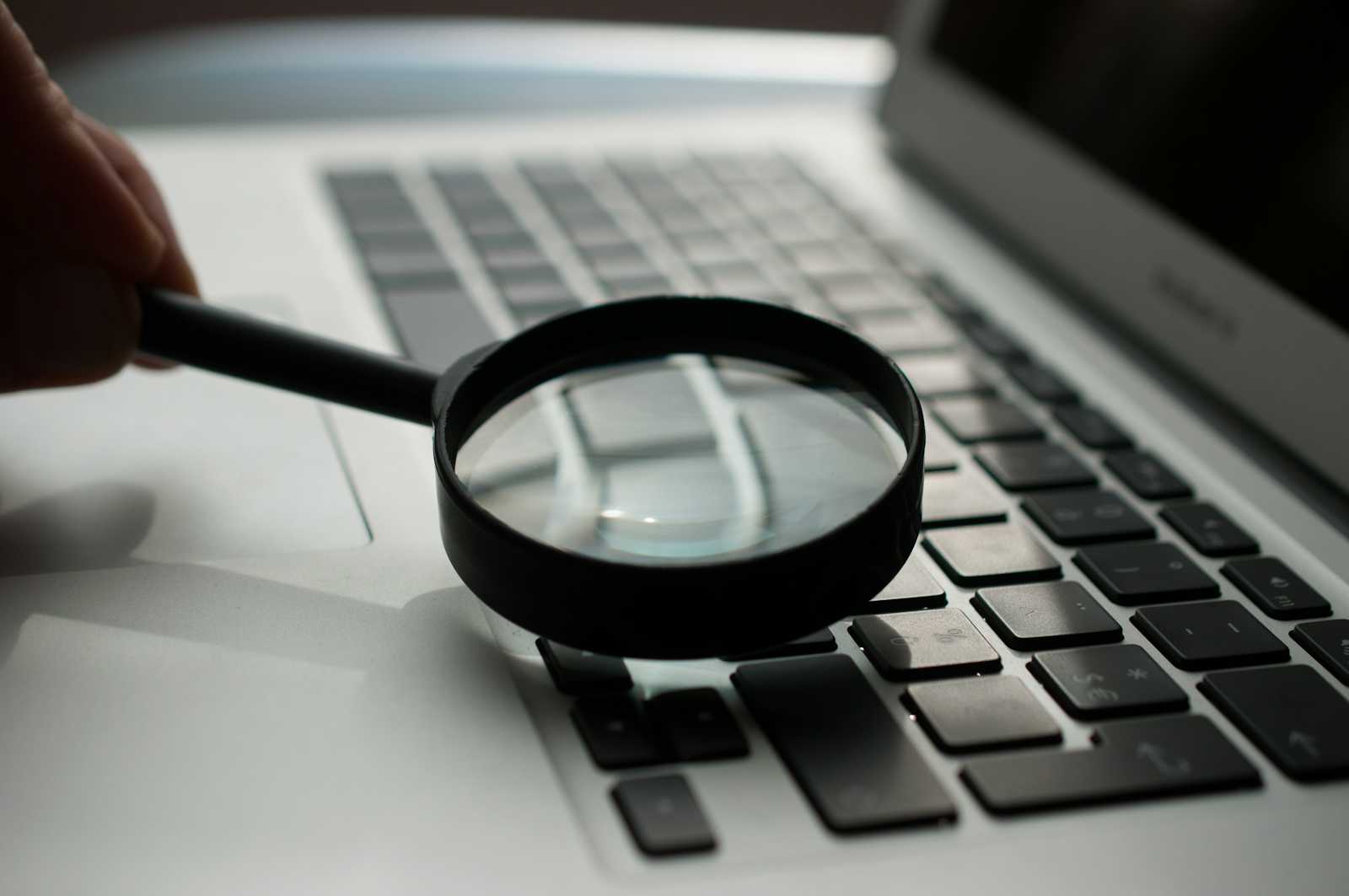
Repeatedly divide the array or list into halves to reduce the search space and locate the target value.
How it works ?
Define start of array as index 0 initially.
Define end of array as index of array’s length -1 initially.
Run a loop until start is less than or equal to end because we want to reach the stage where there is only one element left in array.
Find mid each time as start + (end - start)/2.
If target is less than element at mid, search in left subarray and update end to mid - 1.
If target is greater than element at mid, search in right sub array and update start to mid + 1.
If target equals mid, return mid.
Update mid in each iteration of the loop.
If target is not found after running entire loop, return -1.
Code
class BinarySearch {
public static void main(String[] args) {
int[] array = {20, 40, 48, 50, 45};
binarySearch(array, 40);
}
static int binarySearch(int[] array, int target) {
int start = 0;
int end = array.length - 1;
while(start <= end) {
int mid = start + (end - start)/2;
if(target < array[mid])
end = mid -1;
else if(target > array[mid])
start = mid + 1;
else {
System.out.println("Number found at index: " + mid);
return mid;
}
}
System.out.println("Number not found");
return -1;
}
}
Output
java -cp /tmp/ujLsSYZLNs/BinarySearch
Number found at index: 1
=== Code Execution Successful ===
Characteristics
Time Complexity: O(log N) because in each iteration the size of array reduces by half. N → N/2 → N/4 → N/8 … N/2^k, at the end we are left with only one element to compare target with.
N/2^k = 1, taking log on both sides gives k = log N/ log 2.
Space Complexity: O(1)
Binary Search is best applicable when given array or list is sorted.
Subscribe to my newsletter
Read articles from Juhilee Nazare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
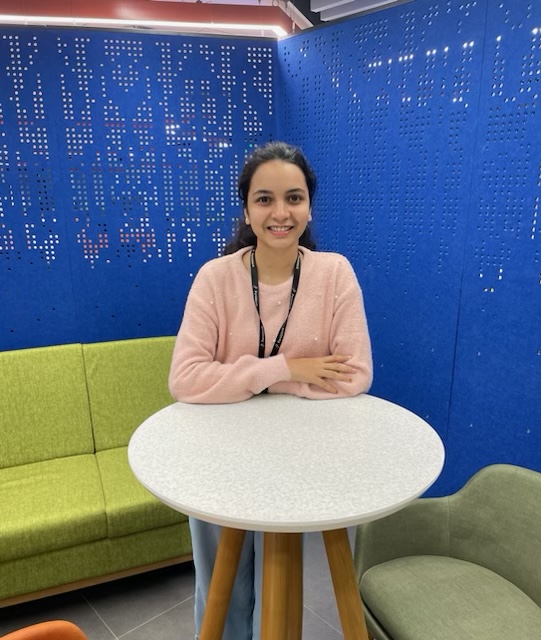
Juhilee Nazare
Juhilee Nazare
I've 3.5+ years of experience in software development and willing to share my knowledge while working on real-time IT solutions.