š Boost Your Side Projects with These Free, Production-Level Databases:

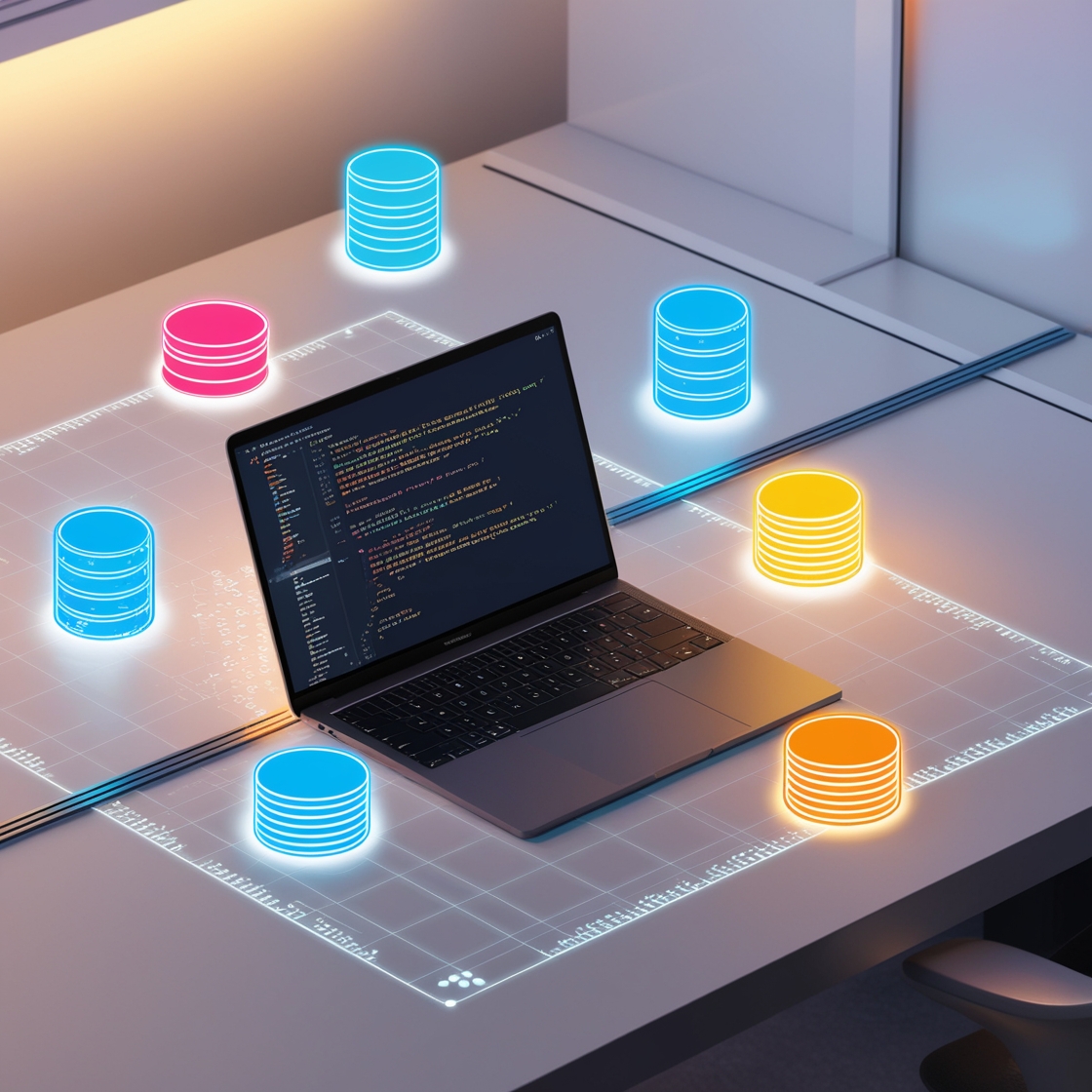
Whether you're building a hobby project or experimenting with new ideas, these free databases can provide the reliability and scalability you need:
Neon Database
Exploring Neon Database: Features, Usage, and Code Implementation
Introduction
Neon is a serverless, scalable implementation of PostgreSQL, designed to offer a modern, flexible, and efficient database solution. It separates storage and compute, allowing for autoscaling, database branching, and scaling to zero.
Key Features of Neon Database
Serverless Architecture: Neon operates on a serverless model, meaning it automatically scales resources up or down based on demand. This ensures cost efficiency and optimal performance.
Separation of Storage and Compute: By decoupling storage and compute, Neon allows for independent scaling of these components. This separation enhances flexibility and resource management.
Database Branching: Similar to Git, Neon supports database branching. This feature enables developers to create isolated branches for testing, development, or backup purposes without affecting the main database.
Autoscaling: Neon can automatically adjust its resources to handle varying workloads, ensuring high availability and performance.
Scale to Zero: When there are no active connections, Neon can scale down to zero, reducing costs during idle periods.
Open Source: Neon is open-source, providing transparency and the ability to customize the database to meet specific needs.
Usage Scenarios
Development and Testing: Developers can use Neonās branching feature to create isolated environments for testing new features or running experiments without impacting the main database.
Microservices Architecture: Neonās ability to scale resources independently makes it ideal for microservices, where different services may have varying resource requirements.
Cost-Effective Solutions: For applications with fluctuating workloads, Neonās autoscaling and scale-to-zero capabilities ensure that resources are used efficiently, reducing costs.
Disaster Recovery: With database branching, creating backups and restoring data becomes straightforward, enhancing disaster recovery strategies.
Code Implementation
Hereās a simple example of how to connect to a Neon database and perform basic operations using Node.js:
// Import the Neon client
const { neon } = require('@neondatabase/serverless');
// Connect to the Neon database
const sql = neon('postgresql://username:password@project.us-east-2.aws.neon.tech/dbname');
// Function to fetch all posts from the database
async function fetchPosts() {
try {
const posts = await sql('SELECT * FROM posts');
console.log(posts);
} catch (error) {
console.error('Error fetching posts:', error);
}
}
// Function to insert a new post into the database
async function insertPost(title, content) {
try {
await sql('INSERT INTO posts (title, content) VALUES ($1, $2)', [title, content]);
console.log('Post inserted successfully');
} catch (error) {
console.error('Error inserting post:', error);
}
}
// Example usage
fetchPosts();
insertPost('My First Post', 'This is the content of my first post.');
Conclusion
Neon offers a robust and flexible solution for modern database needs, combining the power of PostgreSQL with the benefits of a serverless architecture. Its unique features like database branching and autoscaling make it a valuable tool for developers and businesses alike. Whether youāre building a new application or looking to optimize an existing one, Neon provides the tools and capabilities to help you succeed.
MongoDB
Exploring MongoDB: Features, Usage, and Code Implementation
Introduction
MongoDB is a popular NoSQL database known for its flexibility, scalability, and ease of use. Unlike traditional relational databases, MongoDB stores data in JSON-like documents, making it ideal for handling unstructured data.
Key Features of MongoDB
Document-Oriented Storage: MongoDB stores data in flexible, JSON-like documents, allowing for varied data structures within a single collection.
Schema Flexibility: Unlike relational databases, MongoDB does not require a predefined schema, making it easier to evolve data models as application requirements change.
Scalability: MongoDB supports horizontal scaling through sharding, distributing data across multiple servers to handle large volumes of data and high throughput.
Replication: MongoDB provides high availability with replica sets, which maintain multiple copies of data across different servers.
Indexing: MongoDB supports various types of indexes to improve query performance, including single field, compound, and geospatial indexes.
Aggregation Framework: MongoDBās powerful aggregation framework allows for complex data processing and transformation operations.
Open Source: MongoDB is open-source, providing transparency and the ability to customize the database to meet specific needs.
Usage Scenarios
Content Management Systems: MongoDBās flexible schema makes it ideal for content management systems where the structure of content can vary widely.
Real-Time Analytics: MongoDBās ability to handle large volumes of data and perform complex aggregations makes it suitable for real-time analytics applications.
Internet of Things (IoT): MongoDB can efficiently store and process the diverse and high-velocity data generated by IoT devices.
Mobile Applications: MongoDBās offline-first approach and synchronization capabilities make it a good fit for mobile applications.
Code Implementation
Hereās a simple example of how to connect to a MongoDB database and perform basic operations using Node.js:
// Import the MongoDB client
const { MongoClient } = require('mongodb');
// Connection URL
const url = 'mongodb://localhost:27017';
const client = new MongoClient(url);
// Database Name
const dbName = 'mydatabase';
async function main() {
// Connect to the MongoDB server
await client.connect();
console.log('Connected successfully to server');
const db = client.db(dbName);
// Collection Name
const collection = db.collection('documents');
// Insert a document
const insertResult = await collection.insertOne({ name: 'John Doe', age: 30 });
console.log('Inserted document:', insertResult.insertedId);
// Find all documents
const findResult = await collection.find({}).toArray();
console.log('Found documents:', findResult);
// Update a document
const updateResult = await collection.updateOne({ name: 'John Doe' }, { $set: { age: 31 } });
console.log('Updated document:', updateResult.modifiedCount);
// Delete a document
const deleteResult = await collection.deleteOne({ name: 'John Doe' });
console.log('Deleted document:', deleteResult.deletedCount);
// Close the connection
await client.close();
}
main().catch(console.error);
Conclusion
MongoDB offers a robust and flexible solution for modern database needs, particularly when dealing with unstructured data and requiring high scalability. Its document-oriented approach and powerful features make it a valuable tool for a wide range of applications. Whether youāre building a content management system, a real-time analytics platform, or an IoT solution, MongoDB provides the tools and capabilities to help you succeed.
Supabase Database
Exploring Supabase Database: Features, Usage, and Code Implementation
Introduction
Supabase is an open-source backend-as-a-service (BaaS) that provides developers with a suite of tools to build and scale applications quickly. It leverages PostgreSQL for its database, offering real-time capabilities, authentication, and storage solutions.
Key Features of Supabase
PostgreSQL Database: Supabase uses PostgreSQL, a powerful and reliable relational database, ensuring robust data management and querying capabilities.
Real-Time Capabilities: Supabase provides real-time updates, allowing applications to react to changes in the database instantly.
Authentication: Built-in authentication supports email, social logins, and magic links, making it easy to manage user identities.
Storage: Supabase offers scalable storage solutions for files, images, and other media, with a built-in CDN for fast delivery.
Auto-Generated APIs: RESTful and GraphQL APIs are auto-generated from your database schema, enabling easy data access and manipulation.
Edge Functions: Supabase supports serverless functions, allowing you to run custom business logic close to your users.
Database Branching: Similar to Git, Supabase allows you to create branches of your database for testing and development purposes.
Security: Supabase includes features like row-level security and SSL enforcement to ensure data protection and compliance.
Usage Scenarios
Real-Time Applications: Supabaseās real-time capabilities make it ideal for chat applications, live dashboards, and collaborative tools.
SaaS Products: With built-in authentication and storage, Supabase is well-suited for building software-as-a-service (SaaS) products.
E-Commerce: Supabaseās robust database and real-time features can power e-commerce platforms, handling everything from user authentication to inventory management.
Mobile and Web Apps: Supabaseās auto-generated APIs and storage solutions simplify the development of mobile and web applications.
Code Implementation
Hereās a simple example of how to connect to a Supabase database and perform basic operations using JavaScript:
// Import the Supabase client
import { createClient } from '@supabase/supabase-js';
// Create a Supabase client
const supabaseUrl = 'https://your-supabase-url.supabase.co';
const supabaseKey = 'your-supabase-key';
const supabase = createClient(supabaseUrl, supabaseKey);
// Function to fetch all posts from the database
async function fetchPosts() {
const { data, error } = await supabase
.from('posts')
.select('*');
if (error) {
console.error('Error fetching posts:', error);
} else {
console.log('Posts:', data);
}
}
// Function to insert a new post into the database
async function insertPost(title, content) {
const { data, error } = await supabase
.from('posts')
.insert([{ title, content }]);
if (error) {
console.error('Error inserting post:', error);
} else {
console.log('Post inserted successfully:', data);
}
}
// Example usage
fetchPosts();
insertPost('My First Post', 'This is the content of my first post.');
Conclusion
Supabase offers a comprehensive and flexible backend solution for modern applications, combining the power of PostgreSQL with real-time capabilities, authentication, and storage. Its ease of use and robust features make it an excellent choice for developers looking to build scalable and responsive applications. Whether youāre developing a real-time chat app, a SaaS product, or an e-commerce platform, Supabase provides the tools and infrastructure to help you succeed.
PlanetScale
Exploring PlanetScale Database: Features, Usage, and Code Implementation
Introduction
PlanetScale is a MySQL-compatible database platform designed to offer scalability, performance, and reliability without compromising on developer experience. It leverages Vitess, an open-source database clustering system, to provide advanced features like horizontal sharding and non-blocking schema changes.
Key Features of PlanetScale
Horizontal Sharding: PlanetScale uses Vitess to shard data across multiple databases, allowing for unlimited scalability and high performance without the need for complex sharding logic in your application.
Non-Blocking Schema Changes: Schema changes in PlanetScale are applied without locking tables or causing downtime, ensuring continuous availability.
Database Branching: Similar to version control systems, PlanetScale allows you to create branches of your database schema for development, testing, or staging purposes.
Read-Only Regions: Support for globally distributed applications with read-only regions, enhancing performance and availability for read-heavy workloads.
Zero Downtime Imports: Migrate your existing databases to PlanetScale without any downtime, ensuring a seamless transition.
Built-In Connection Pooling: PlanetScale handles connection pooling automatically, eliminating connection limits and improving performance.
Enterprise-Grade Security: Features like daily backups, automatic failover, and robust security measures ensure data protection and reliability.
Usage Scenarios
High-Traffic Applications: PlanetScaleās horizontal sharding and non-blocking schema changes make it ideal for applications with high traffic and large datasets.
Global Applications: With read-only regions, PlanetScale supports globally distributed applications, ensuring low latency and high availability for users worldwide.
Continuous Deployment: The branching and non-blocking schema change features facilitate continuous deployment and integration, making it easier to manage database changes in a CI/CD pipeline.
E-Commerce Platforms: PlanetScaleās scalability and reliability are well-suited for e-commerce platforms that require high availability and performance.
Code Implementation
Hereās a simple example of how to connect to a PlanetScale database and perform basic operations using Node.js:
// Import the PlanetScale client
import { connect } from '@planetscale/database';
// Create a PlanetScale client
const config = {
host: 'your-planetscale-host',
username: 'your-username',
password: 'your-password'
};
const conn = connect(config);
// Function to fetch all users from the database
async function fetchUsers() {
try {
const results = await conn.execute('SELECT * FROM users');
console.log('Users:', results);
} catch (error) {
console.error('Error fetching users:', error);
}
}
// Function to insert a new user into the database
async function insertUser(name, email) {
try {
await conn.execute('INSERT INTO users (name, email) VALUES (?, ?)', [name, email]);
console.log('User inserted successfully');
} catch (error) {
console.error('Error inserting user:', error);
}
}
// Example usage
fetchUsers();
insertUser('Jane Doe', 'jane.doe@example.com');
Conclusion
PlanetScale offers a powerful and flexible database solution for modern applications, combining the reliability of MySQL with advanced features like horizontal sharding and non-blocking schema changes. Its ease of use and robust capabilities make it an excellent choice for developers looking to build scalable and high-performance applications. Whether youāre developing a high-traffic web app, a global service, or an e-commerce platform, PlanetScale provides the tools and infrastructure to help you succeed.
Appwrite
Exploring Appwrite Database: Features, Usage, and Code Implementation
Introduction
Appwrite is an open-source backend-as-a-service (BaaS) platform that provides a comprehensive suite of tools for building and scaling applications. It includes a powerful database service that supports structured data storage, querying, and real-time updates.
Key Features of Appwrite Database
Structured Data Storage: Appwrite allows you to create structured collections of documents, making it easy to organize and manage your data.
Real-Time Updates: Appwrite supports real-time data updates, enabling applications to react instantly to changes in the database.
Data Validation: You can define attributes for your collections to ensure that all user-submitted data is validated and stored according to the collection structure.
Indexing: Appwrite provides indexing capabilities to improve query performance, allowing you to efficiently search and filter your data.
Permissions and Access Control: Appwriteās permissions architecture lets you assign read or write access to each collection or document for specific users, teams, or roles.
Relationships: Appwrite supports relationships between collections, helping you model complex business logic.
Open Source: Being open-source, Appwrite offers transparency and the ability to customize the platform to meet specific needs.
Usage Scenarios
Real-Time Applications: Appwriteās real-time capabilities make it ideal for chat applications, live dashboards, and collaborative tools.
Content Management Systems: The structured data storage and validation features are perfect for content management systems where data integrity is crucial.
Mobile and Web Apps: Appwriteās comprehensive suite of tools, including authentication and storage, simplifies the development of mobile and web applications.
E-Commerce Platforms: With robust data management and real-time updates, Appwrite can power e-commerce platforms, handling everything from user authentication to inventory management.
Code Implementation
Hereās a simple example of how to connect to an Appwrite database and perform basic operations using JavaScript:
// Import the Appwrite client
import { Client, Databases } from 'appwrite';
// Create a new Appwrite client
const client = new Client()
.setEndpoint('https://[HOSTNAME_OR_IP]/v1') // Your API Endpoint
.setProject('your_project_id'); // Your project ID
// Initialize the Databases service
const databases = new Databases(client);
// Function to fetch all documents from a collection
async function fetchDocuments() {
try {
const result = await databases.listDocuments('your_database_id', 'your_collection_id');
console.log('Documents:', result.documents);
} catch (error) {
console.error('Error fetching documents:', error);
}
}
// Function to insert a new document into a collection
async function insertDocument(data) {
try {
const result = await databases.createDocument('your_database_id', 'your_collection_id', 'unique()', data);
console.log('Document inserted successfully:', result);
} catch (error) {
console.error('Error inserting document:', error);
}
}
// Example usage
fetchDocuments();
insertDocument({ title: 'My First Document', content: 'This is the content of my first document.' });
Conclusion
Appwrite offers a robust and flexible database solution for modern applications, combining structured data storage with real-time capabilities and comprehensive access control. Its ease of use and powerful features make it an excellent choice for developers looking to build scalable and responsive applications. Whether youāre developing a real-time chat app, a content management system, or an e-commerce platform, Appwrite provides the tools and infrastructure to help you succeed.
Firebase
Exploring Firebase: Features, Usage, and Code Implementation
Introduction
Firebase is a comprehensive app development platform by Google that provides a suite of tools and services to help developers build high-quality apps. It simplifies backend development, allowing you to focus more on creating engaging user experiences.
Key Features of Firebase
Realtime Database: Firebase Realtime Database is a NoSQL cloud database that allows you to store and sync data between your users in real-time.
Firestore: A flexible, scalable database for mobile, web, and server development from Firebase and Google Cloud Platform.
Authentication: Firebase Authentication provides backend services to easily authenticate users with passwords, phone numbers, and popular federated identity providers like Google, Facebook, and Twitter.
Cloud Functions: These are serverless functions that let you run backend code in response to events triggered by Firebase features and HTTPS requests.
Cloud Messaging: Firebase Cloud Messaging (FCM) allows you to send notifications and messages to users across platforms.
Hosting: Firebase Hosting provides fast and secure hosting for your web app, static and dynamic content, and microservices.
Analytics: Firebase Analytics offers free, unlimited reporting on up to 500 distinct events.
Crashlytics: A real-time crash reporter that helps you track, prioritize, and fix stability issues that erode your app quality.
Free Database in Firebase
Firebase offers a generous free tier, known as the Spark Plan, which is perfect for small applications and personal projects. Here are some of the free usage limits:
Realtime Database: 1 GB of storage and 50,000 reads/day, 20,000 writes/day, and 20,000 deletes/day1.
Firestore: 1 GB of storage and 50,000 reads/day, 20,000 writes/day, and 20,000 deletes/day1.
These limits make it easy to get started without incurring any costs, and you can scale up as your app grows.
Getting Started with Firebase
Step 1: Create a Firebase Project
Go to the Firebase Console.
Click on āAdd projectā and follow the setup instructions.
Step 2: Add Firebase to Your App
For example, to add Firebase to an Android app:
Register your app with Firebase.
Add the Firebase SDK to your app by including the necessary dependencies in your
build.gradle
file.
dependencies {
// Add the Firebase SDK for Google Analytics
implementation 'com.google.firebase:firebase-analytics:21.0.0'
// Add the Firebase SDK for Realtime Database
implementation 'com.google.firebase:firebase-database:20.0.0'
}
Step 3: Initialize Firebase in Your App
Initialize Firebase in your MainActivity.java
:
import com.google.firebase.database.FirebaseDatabase;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize Firebase
FirebaseDatabase database = FirebaseDatabase.getInstance();
DatabaseReference myRef = database.getReference("message");
myRef.setValue("Hello, Firebase!");
}
}
Using Firebase Features
Realtime Database
To read and write data to the Firebase Realtime Database:
// Write a message to the database
DatabaseReference myRef = FirebaseDatabase.getInstance().getReference("message");
myRef.setValue("Hello, Firebase!");
// Read from the database
myRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
String value = dataSnapshot.getValue(String.class);
Log.d("TAG", "Value is: " + value);
}
@Override
public void onCancelled(DatabaseError error) {
// Failed to read value
Log.w("TAG", "Failed to read value.", error.toException());
}
});
Authentication
To set up Firebase Authentication:
Enable the desired authentication method in the Firebase Console.
Add the Firebase Authentication SDK to your app.
implementation 'com.google.firebase:firebase-auth:21.0.0'
- Use the FirebaseAuth instance to sign in users.
FirebaseAuth mAuth = FirebaseAuth.getInstance();
// Sign in with email and password
mAuth.signInWithEmailAndPassword(email, password)
.addOnCompleteListener(this, new OnCompleteListener<AuthResult>() {
@Override
public void onComplete(@NonNull Task<AuthResult> task) {
if (task.isSuccessful()) {
// Sign in success
FirebaseUser user = mAuth.getCurrentUser();
Log.d("TAG", "signInWithEmail:success");
} else {
// If sign in fails, display a message to the user.
Log.w("TAG", "signInWithEmail:failure", task.getException());
}
}
});
Conclusion
Firebase offers a robust set of tools and services that can significantly streamline your app development process. Whether youāre building a simple app or a complex, scalable solution, Firebase has the features to support your needs. With its generous free tier, you can start exploring Firebase today without any cost and take your app development to the next level!
If you found this blog helpful, please like, share, and follow for more content like this. If you have any questions or need further assistance, feel free to leave a comment or reach out. Happy coding ššŖ!
Subscribe to my newsletter
Read articles from Prajwal Urkude directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prajwal Urkude
Prajwal Urkude
Tech enthusiast š» and software developer passionate about web development, game design š®, and cloud computing āļø. With a strong foundation in programming and a love for open-source technologies š ļø, I explore innovative tools and techniques to craft engaging digital experiences. On my blog, I share insights on web development, cloud solutions, and databases for hobbyists, aiming to empower developers at every stage of their journey š. This version highlights your focus on writing and tech while keeping an elegant tone!