Day 65 - Working with Terraform Resources 🚀
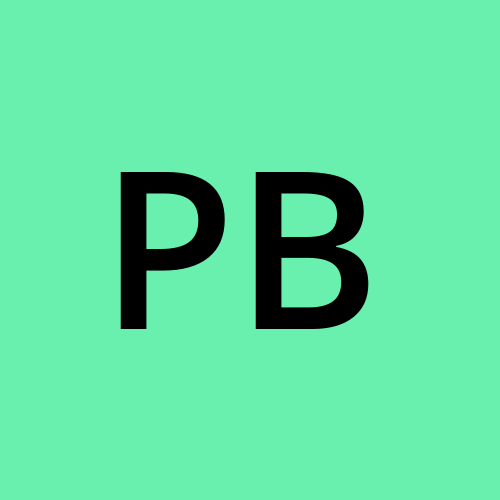
Understanding Terraform Resources
A resource in Terraform represents a component of your infrastructure, such as a physical server, a virtual machine, a DNS record, or an S3 bucket. Resources have attributes that define their properties and behaviors, such as the size and location of a virtual machine or the domain name of a DNS record.
When you define a resource in Terraform, you specify the type of resource, a unique name for the resource, and the attributes that define the resource. Terraform uses the resource block to define resources in your Terraform configuration.
Task 1: Create a security group
- Create a folder
terraform-webserver
and enter into it
mkdir terraform-webserver && cd terraform-webserver
- create a file
terraform.tf
and write the code
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 5.0"
}
}
}
- Now we should install
awscli
and setup aws configure to configure this you can use this script.
#!/bin/bash
sudo apt install unzip
curl "https://awscli.amazonaws.com/awscli-exe-linux-x86_64.zip" -o "awscliv2.zip"
unzip awscliv2.zip
sudo ./aws/install
aws --version
aws configure
AWS Access Key ID [None]: <your access key>
AWS Secret Access Key [None]: <your Secret Access Key>
- create a file
providers.tf
and write the code
provider "aws" {
region = "us-east-1"
}
To create a keypair we need to generate a key using this commands
# come back to your repository cd cd .ssh ssh-keygen <your keyname> ls pwd cd
create a file
keypair.tf
and wite the code
resource "aws_key_pair" "terrakey" {
key_name = "terrakey"
public_key = file("/home/ubuntu/.ssh/terrakey.pub")
}
- create a file
security-group.tf
and write this code
resource "aws_security_group" "web_server" {
name_prefix = "web-server-sg"
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 443
to_port = 443
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
ipv6_cidr_blocks = ["::/0"]
}
}
- Now create a file
main.tf
and write this code
resource "aws_instance" "web_server" {
ami = "<your ami-id>"
instance_type = "t2.micro"
key_name = "<your key name creted in the instance>"
subnet_id = "<your subnet-id>"
security_groups = [
aws_security_group.web_server.id
]
associate_public_ip_address = true
user_data = <<-EOF
#!/bin/bash
sudo apt update
sudo apt install -y apache2
sudo systemctl start apache2
sudo systemctl enable apache2
echo "<html><body><h1>Welcome to DevOpsParthu community!</h1></body></html>" > /var/www/html/index.html
EOF
tags = {
Name = "web_server"
}
}
- Run terraform init to initialize the Terraform project
terraform init
- Run terraform apply to create the security group.
terraform apply
Task 2: Create an EC2 instance
- Now your EC2 instance will create with Terraform.
Task 3: Access your website
Open a web browser and enter the public IP or DNS name of your EC2 instance.
You should see your website's content displayed in your browser.
Subscribe to my newsletter
Read articles from Pooja Bhavani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
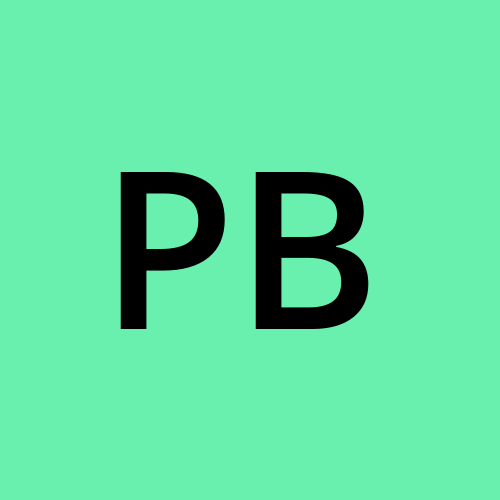
Pooja Bhavani
Pooja Bhavani
Hi, I am Pooja Bhavani, an enthusiastic DevOps Engineer with a focus on deploying production-ready applications, infrastructure automation, cloud-native technologies. With hands-on experience across DevOps Tools and AWS Cloud, I thrive on making infrastructure scalable, secure, and efficient. My journey into DevOps has been fueled by curiosity and a passion for solving real-world challenges through automation, cloud architecture, and seamless deployments. I enjoy working on projects that push boundaries whether it's building resilient systems, optimizing CI/CD pipelines, or exploring emerging technologies like Amazon Q and GenAI. I'm currently diving deeper into platform engineering and GitOps workflows, and I often share practical tutorials, insights, and use cases from my projects and experiences. ✨ Let’s connect, collaborate, and grow together in this ever-evolving DevOps world. Open to opportunities, ideas, and conversations that drive impactful tech!