Code Guardians: Navigating Access Modifiers and Packages in Java

Table of contents
- Definition of a Package in Java :
- Creation of a Package :
- Why Use Packages?
- Introduction to the Import Statement
- How the Import Statement Works
- Why Use the Import Statement?
- Definition
- Introduction to Access Modifiers
- Let’s understand this by considering a scenario:
- The Four Access Modifiers
- Summary
- Table of Access Modifiers for Accessibility
- Implementing the Café System
- Explanation of the Menu Class
- Creating a Staff Menu
- Explanation of the Staff Menu Class
- Conclusion and Summary
- Overall Significance
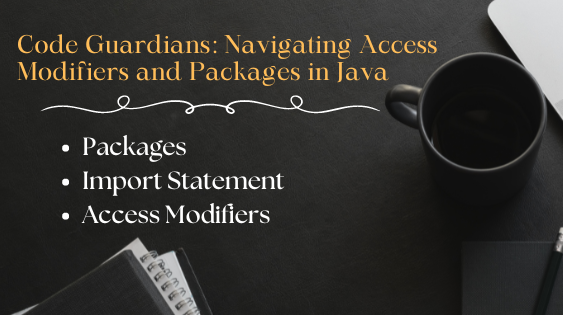
Imagine you have a vast music collection on your device. To keep it organized, you create a main folder labeled "Music" and sub-folders for different categories: one for your favorite songs, another for Bollywood hits, and others by singer. This system makes it easy to find and manage your music.
In Java, packages function in much the same way. A package is like a folder that groups related classes together, helping you organize your code and manage namespaces.
Just as folders keep your music tidy and accessible, packages in Java help maintain organized and manageable code. This not only enhances readability but also prevents naming conflicts and promotes code reuse. Embrace packages to make your Java projects as harmonious as your favorite playlists!
Definition of a Package in Java :
A package in Java is a namespace that organizes a set of related classes and interfaces. It helps manage code organization, avoid naming conflicts, and control access. By grouping similar classes, packages enhance code modularity and maintainability.
Syntax
package packageName;
package
is a keyword indicating that you are declaring a package and your class belongs to that package.packageName
is the name you choose for your package, usually following a hierarchical structure, often starting with the reverse of your domain name (e.g.,com.example
), followed by specific identifiers that represent the functionality or purpose of the classes contained in the package.
Creation of a Package :
Suppose you have a class named Dog.java
. To organize this class, you can create a package named animal
that will contain all animal-related classes. This way, you keep your code organized and easy to manage.
package animal;
class Dog {
String color;
int age;
String name;
// Method for the dog to bark
public void bark() {
System.out.println("vow vow");
}
// Method for the dog to eat
public void eat() {
System.out.println("Dog can eat");
}
}
Why Use Packages?
Imagine you and another student at your school or college share the same name. If someone calls out that name, it can cause confusion about who should respond. Now, let's say your parents visit the school and wait in the waiting room. The school peon comes to your class to call you.
To avoid confusion, the peon might use additional information, like your last name or class section, to ensure the right student responds. This way, even if there are multiple students with the same first name, the peon can accurately identify and call the correct person.
In Java, packages help avoid similar conflicts by organizing classes into distinct namespaces, ensuring that even if classes have the same name, they can be uniquely identified by their package names.
Benefits of Packages
Organization: Packages help you keep your code neat and organized, especially in large projects.
Namespace Management: They prevent naming conflicts. For instance, you can have two classes named
Dog
in different packages without issue.Access Control: Packages can also help manage access levels to classes and their members.
Introduction to the Import Statement
After learning how to create and organize packages in Java, it’s time to discover how to use the classes defined in those packages. Let’s explore this with an exciting scenario involving your beloved pet!
Imagine you’ve created a Dog class in an Animal package, which captures all the essential details about dogs—like their breed, age, and behaviors. Now, you want to use this Dog class in another package called YourPet, where you manage different pets and their care routines.
This is where the import statement comes into play. Instead of duplicating your Dog class in the YourPet package, you can simply import it from the Animal package. This not only saves time and effort but also promotes better code organization and reuse.
Step 1: Creating the Animal Package
Let’s say you’ve already created a package called Animal, and within it, you have a Dog class:
// File: com/animal/Dog.java
package com.animal;
public class Dog {
private String breed;
private int age;
public Dog(String breed, int age) {
this.breed = breed;
this.age = age;
}
public String getDetails() {
return "Breed: " + breed + ", Age: " + age + " years";
}
public void bark() {
System.out.println("Woof! Woof!");
}
}
Step 2: Creating the YourPet Package
Now, you want to create a package called YourPet to manage your pet details and activities. Here’s how you can do that:
// File: com/yourpet/PetManager.java
package com.yourpet;
// Importing the Dog class from the Animal package
import com.animal.Dog;
public class PetManager {
public static void main(String[] args) {
// Creating a Dog object using the imported Dog class
Dog myDog = new Dog("Golden Retriever", 3);
System.out.println(myDog.getDetails());
myDog.bark(); // Calling the bark method
}
}
How the Import Statement Works
Importing the Class: At the top of the
PetManager
class, the lineimport com.animal.Dog;
tells the Java compiler that you want to use theDog
class from thecom.animal
package. This allows you to access theDog
class without writing its full package name every time.Creating Objects: Once imported, you can create instances of the
Dog
class directly usingDog myDog = new Dog("Golden Retriever", 3);
. You can now use all the methods defined in theDog
class, likegetDetails()
andbark()
.
Why Use the Import Statement?
Code Reusability: You don’t have to recreate the
Dog
class in the YourPet package. Instead, you can leverage existing code, making your program more efficient.Clarity and Organization: The import statement keeps your code clean and organized. It’s clear which classes you’re using and where they come from.
Avoids Duplication: Prevents redundant code and keeps your project easier to maintain. If you need to update the
Dog
class, you only do it in one place.
Definition
An import statement in Java is used to bring in classes and interfaces from other packages into the current file, allowing you to use them without needing to specify their fully qualified names. This helps in organizing code and avoiding redundancy by reusing existing classes and interfaces.
Now you are able to create your package and also use it anywhere. But while writing some logic in your package or some code, it should not be visible to the clients. Additionally, you don’t want everyone to use your code. How can you achieve that? This is where access modifiers come into the picture.
Introduction to Access Modifiers
Imagine you have a treasure chest filled with valuable items. You want to share some of these items with your friends, but you also want to keep certain items private and only accessible to you. To manage this, you use different types of locks and keys. Some keys are given to your friends, while others are kept only by you.
In Java, access modifiers act like these locks and keys. They control the visibility and accessibility of classes, methods, and variables. By using access modifiers, you can specify which parts of your code can be accessed by other classes and which parts should remain hidden.
Let’s understand this by considering a scenario:
Imagine you’ve opened a quirky café called "The Hidden Brew," where you serve exclusive blends of coffee and pastries. To keep things interesting and organized, you’ve created a unique menu system. In this café, the menu items have different levels of accessibility based on who can see or order them.
The Four Access Modifiers
Public:
Visibility: Accessible to everyone, just like the main menu at your café.
Use Case: You want all your customers to see and order popular items like Espresso or Cappuccino.
Example: Methods that let customers view or order these drinks.
Protected:
Visibility: Accessible only to your café staff and anyone who has worked at the café (subclasses).
Use Case: Special recipes that are reserved for employees but not available to the general public.
Example: A method that allows staff to modify a recipe for the Secret Blend.
Default (Package-Private):
Visibility: Accessible only within the café’s internal operations (same package).
Use Case: Behind-the-scenes items like the ingredient stock or special blends for events that shouldn’t be public knowledge.
Example: A method to manage inventory that is not exposed to customers.
Private:
Visibility: Accessible only within the class, just like your private notes on how to run the café.
Use Case: Sensitive information, such as your cost calculations or customer feedback, that should not be shared with anyone outside your planning team.
Example: Variables that store profit margins or customer details.
Summary
Access Modifier | Café Staff | Customers | Other Cafés |
Public | Yes | Yes | Yes |
Protected | Yes | No | No |
Default | Yes | No | No |
Private | Yes | No | No |
Table of Access Modifiers for Accessibility
Access Modifier | Same Class | Same Package | Sub-class (Different Package) | All Classes |
Public | YES | YES | YES | YES |
Protected | YES | YES | YES | NO |
Default | YES | YES | NO | NO |
Private | YES | NO | NO | NO |
Implementing the Café System
Let’s see how you could define a simple café menu system using access modifiers in Java.
// File: com/cafe/Menu.java
package com.cafe;
public class Menu {
private String secretRecipe; // Only accessible within this class
protected String specialBlend; // Accessible in subclasses
public Menu(String secretRecipe, String specialBlend) {
this.secretRecipe = secretRecipe;
this.specialBlend = specialBlend;
}
public void showMenu() { // Public method
System.out.println("Welcome to The Hidden Brew!");
System.out.println("1. Espresso");
System.out.println("2. Cappuccino");
System.out.println("3. Special Blend (Staff Only)");
}
}
Explanation of the Menu Class
Private Variable:
secretRecipe
isprivate
, which means only you (the café owner) can see the details of your secret recipe. No one else can access it directly, ensuring your special blend remains exclusive.
Protected Variable:
specialBlend
isprotected
. This means only staff (or subclasses) can access this information. So, if you have aStaffMenu
class, they can see it.
Public Method:
- The
showMenu()
method ispublic
, allowing everyone (customers) to see the available drinks. This way, all your customers know what they can order.
- The
Creating a Staff Menu
Now, let’s create a subclass for your staff:
// File: com/cafe/StaffMenu.java
package com.cafe;
public class StaffMenu extends Menu {
public StaffMenu(String secretRecipe, String specialBlend) {
super(secretRecipe, specialBlend);
}
public void revealSecret() {
System.out.println("Staff can access the secret recipe: " + specialBlend);
}
}
Explanation of the Staff Menu Class
Accessing Protected Variable:
- The
StaffMenu
class can access thespecialBlend
variable because it’sprotected
. Staff members can see the details of the special blend, enhancing their experience at the café.
- The
Separation of Responsibilities:
- The
StaffMenu
can have additional methods that are only relevant to staff, while theMenu
class keeps the customer-facing elements clean and straightforward.
- The
Conclusion and Summary
In this exploration of Java, we’ve covered three essential concepts: packages, import statements, and access modifiers. Understanding these topics is crucial for organizing your code effectively and ensuring that it remains maintainable and secure.
Packages
Definition: A package in Java is a namespace that groups related classes and interfaces, allowing for better organization of code.
Purpose: Packages help avoid naming conflicts, promote reusability, and enhance code readability. They are akin to folders in a file system, allowing you to structure your project logically.
Import Statements
Definition: The import statement in Java allows you to access classes and interfaces from other packages without needing to reference their fully qualified names.
Purpose: By using import statements, you simplify your code, improve readability, and avoid repetition. This makes it easier to manage and utilize existing code, much like bringing in tools or ingredients from different parts of your kitchen.
Access Modifiers
Definition: Access modifiers control the visibility and accessibility of classes, methods, and variables. The four main types are public, protected, default (package-private), and private.
Purpose: Access modifiers play a vital role in encapsulation, allowing you to protect sensitive information and control how different parts of your code interact. They help maintain a clean interface, ensuring that only necessary components are exposed to the outside world.
Overall Significance
Together, these concepts form the foundation for building well-structured, modular, and secure applications in Java. By leveraging packages, import statements, and access modifiers, you can create code that is not only functional but also maintainable and easy to understand. As you continue your Java journey, mastering these elements will enhance your programming skills and contribute to your success in software development. Happy coding!
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.