#5.0 What XPath: Advanced Techniques
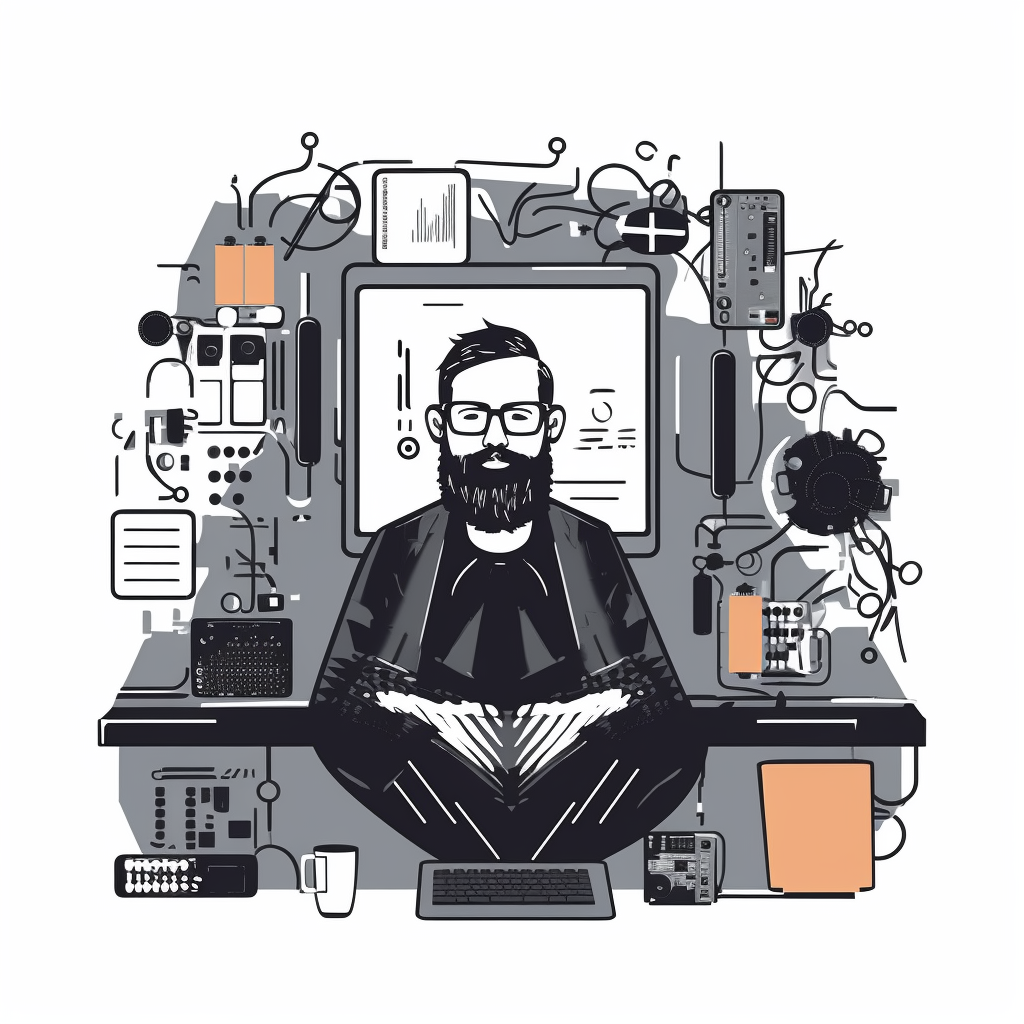
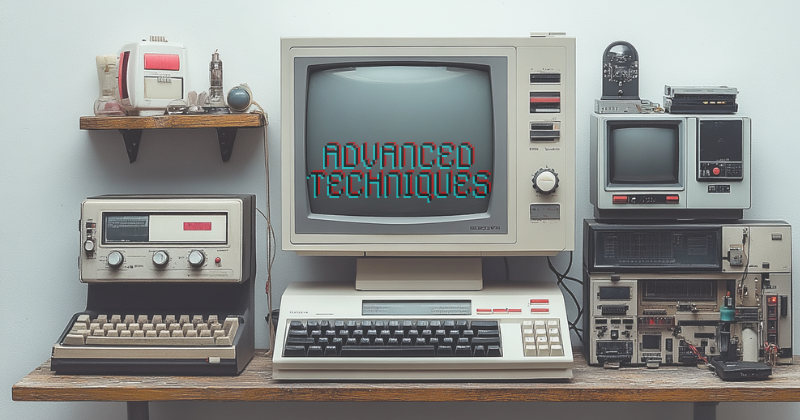
As web applications become more complex and dynamic, locating elements on a page can be challenging. This section covers advanced XPath techniques to handle these scenarios effectively.
5.1 Handling Dynamic Elements
Dynamic elements often have changing attributes or IDs, making them difficult to locate consistently. Here are some techniques to handle such elements:
5.1.1 Using Partial Matches
When IDs or classes change dynamically but contain a consistent part, use the contains()
function:
//div[contains(@id, 'product-')]
This XPath will match elements like <div id="product-123">
and <div id="product-456">
.
HTML snippet:
<div id="product-123">Product A</div>
<div id="product-456">Product B</div>
<div id="unrelated-789">Not a product</div>
5.1.2 Using Starts-with and Ends-with
For elements where the beginning or end of an attribute is consistent:
//input[starts-with(@name, 'user_')]
//a[ends-with(@href, '.pdf')]
Note: ends-with()
is only available in XPath 2.0 and above. For XPath 1.0, you can use this workaround:
//a[substring(@href, string-length(@href) - 3) = '.pdf']
HTML snippet:
<input name="user_firstname" type="text">
<input name="user_lastname" type="text">
<a href="document.pdf">PDF Document</a>
<a href="image.jpg">JPG Image</a>
5.1.3 Using Text Content
When attributes are unreliable, use the text content of the element:
//button[contains(text(), 'Submit')]
This is particularly useful for buttons or links with consistent text but changing IDs or classes.
HTML snippet:
<button id="btn-123">Submit Form</button>
<button id="btn-456">Cancel</button>
5.1.4 Using Parent-Child Relationships
If a parent element has a stable identifier, use it to locate dynamic child elements:
//div[@id='stable-parent']//input[@type='text']
This locates text inputs within a stable parent div, regardless of the input's changing attributes.
HTML snippet:
<div id="stable-parent">
<input type="text" id="dynamic-input-1">
<input type="text" id="dynamic-input-2">
</div>
5.2 Working with AJAX-Loaded Content
AJAX-loaded content poses a challenge because it may not be present in the initial DOM. Here are techniques to handle this:
5.2.1 Using WebDriverWait
Combine XPath with Selenium's WebDriverWait to wait for elements to appear:
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.XPATH, "//div[@class='ajax-loaded']"))
)
This waits up to 10 seconds for the element to appear before interacting with it.
HTML snippet (after AJAX load):
<div class="ajax-loaded">
<p>This content was loaded via AJAX</p>
</div>
5.2.2 Using Dynamic Properties
Look for properties that indicate the AJAX content has loaded:
//div[@class='content' and not(@data-loading)]
This XPath waits for a loading indicator to disappear.
HTML snippet (before and after AJAX load):
<!-- Before load -->
<div class="content" data-loading="true">Loading...</div>
<!-- After load -->
<div class="content">Content has loaded!</div>
5.3 XPath Axes and Their Applications
XPath axes allow you to select nodes based on their relationship to the context node. Here are some powerful axes and their uses:
5.3.1 Following and Preceding
Select elements that appear after or before the context node in document order:
//label[text()='Username']/following::input[1]
This selects the first input element that follows the 'Username' label.
HTML snippet:
<label>Username</label>
<input type="text" name="username">
<label>Password</label>
<input type="password" name="password">
5.3.2 Ancestor and Descendant
Navigate up or down the DOM tree:
//span[@class='error']/ancestor::form
//div[@id='content']//descendant::p
The first XPath finds the form containing an error message, while the second finds all paragraphs within a content div.
HTML snippet:
<form>
<div>
<input type="text">
<span class="error">Invalid input</span>
</div>
</form>
<div id="content">
<p>Paragraph 1</p>
<div>
<p>Nested paragraph</p>
</div>
</div>
5.3.3 Following-sibling and Preceding-sibling
Select siblings that appear after or before the context node:
//th[text()='Name']/following-sibling::th[1]
This selects the table header immediately following the 'Name' header.
HTML snippet:
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>Email</th>
</tr>
</table>
5.3.4 Self
Useful in complex expressions to refer back to the context node:
//div[@class='item'][count(self::*//*) > 5]
This selects 'item' divs that have more than 5 descendant elements.
HTML snippet:
<div class="item">
<h3>Item Title</h3>
<p>Description</p>
<ul>
<li>Feature 1</li>
<li>Feature 2</li>
<li>Feature 3</li>
</ul>
</div>
5.4 Combining Multiple Techniques
For the most challenging scenarios, combine these techniques:
//div[contains(@class, 'product')]
[.//span[contains(@class, 'price')][number(translate(text(), '$,', '')) > 100]]
/following-sibling::div[1]
//button[contains(text(), 'Add to Cart')]
This complex XPath:
Finds product divs
Filters for products with a price over $100
Selects the next div after each matching product
Locates the 'Add to Cart' button within that div
HTML snippet:
<div class="product">
<h3>Expensive Product</h3>
<span class="price">$150.00</span>
</div>
<div class="actions">
<button>Add to Cart</button>
</div>
<div class="product">
<h3>Cheap Product</h3>
<span class="price">$50.00</span>
</div>
<div class="actions">
<button>Add to Cart</button>
</div>
By mastering these advanced techniques, you'll be equipped to handle even the most complex and dynamic web pages in your Selenium automation projects.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
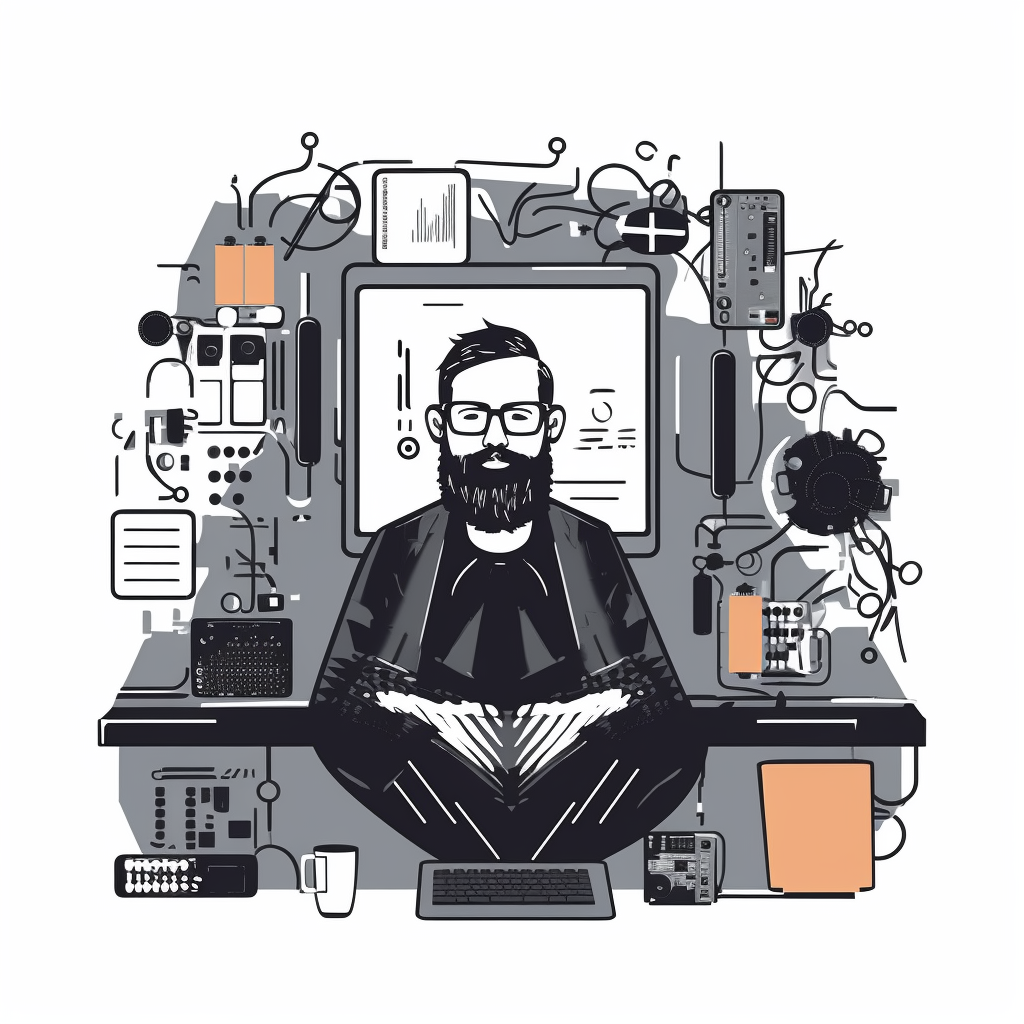
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.