Node.js Interview Questions and Answers: Part 8

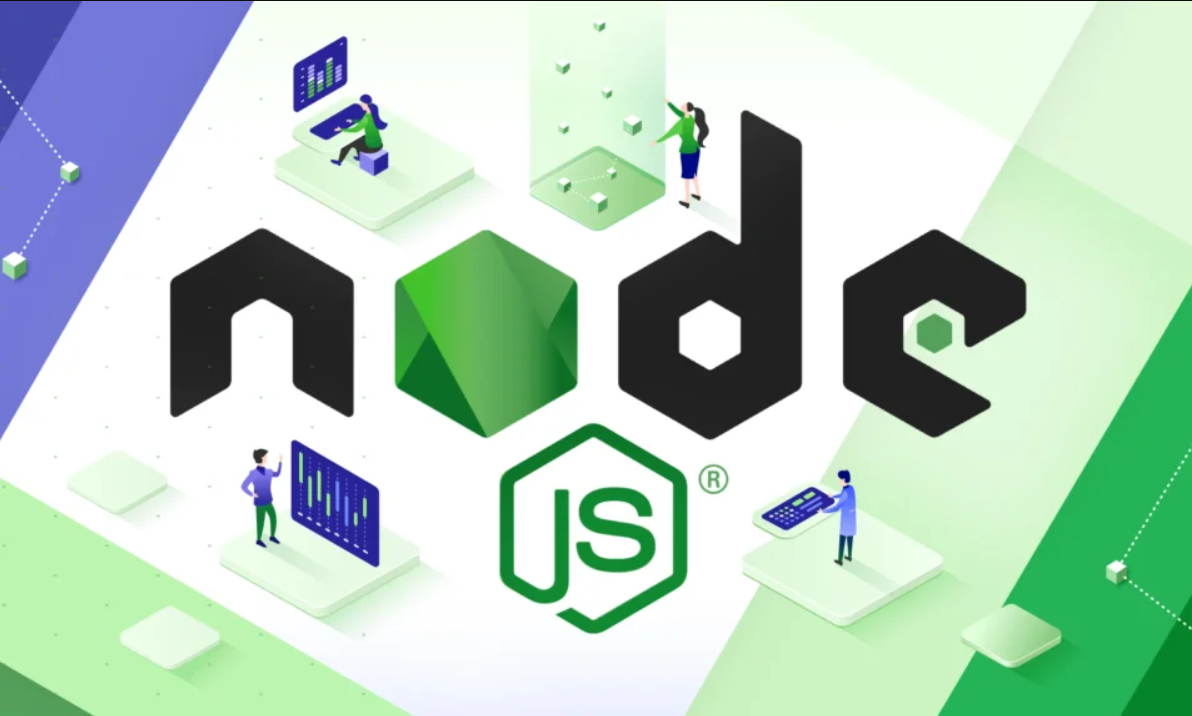
Table of Contents
Node.js Fundamentals: Exploring Key Concepts
Introduction
Node.js has revolutionized server-side programming, bringing JavaScript to the backend and enabling developers to build fast, scalable network applications. In this blog post, we'll dive deep into the fundamental concepts of Node.js through an interview-style Q&A format, complete with code examples and explanations.
Key Features of Node.js
Interviewer: Can you explain the key features that make Node.js stand out in the world of server-side programming?
Candidate: Absolutely! Node.js has several standout features that have contributed to its popularity:
Asynchronous and Event-Driven: Node.js uses an event-driven, non-blocking I/O model, making it lightweight and efficient.
JavaScript Everywhere: It allows developers to use JavaScript on both the front-end and back-end, promoting full-stack development with a single language.
NPM (Node Package Manager): One of the largest ecosystems of open-source libraries in the world, making it easy to add functionality to your projects.
Single-Threaded with Event Loop: Node.js can handle concurrent operations despite running in a single thread, thanks to its event loop.
Cross-Platform: It runs on various platforms like Windows, macOS, and Linux.
Let's look at a simple example that demonstrates the asynchronous nature of Node.js:
console.log('Start');
setTimeout(() => {
console.log('This is asynchronous');
}, 0);
console.log('End');
// Output:
// Start
// End
// This is asynchronous
This code showcases how Node.js doesn't wait for the asynchronous operation (setTimeout) to complete before moving on to the next line.
Non-blocking I/O in Node.js
Interviewer: You mentioned non-blocking I/O. Could you elaborate on what this means in the context of Node.js?
Candidate: Certainly! Non-blocking I/O is a core feature of Node.js that allows it to handle multiple operations concurrently without waiting for each one to complete before moving on to the next.
In a blocking I/O model, when a request for an I/O operation (like reading a file or making a network request) is made, the execution of the entire process is paused until that operation completes. This can lead to significant performance issues, especially in high-traffic applications.
Node.js, on the other hand, uses non-blocking I/O. When an I/O request is made, Node.js continues to process other operations while waiting for the I/O to complete. Once the I/O operation finishes, a callback function is triggered to handle the result.
Here's an example to illustrate the difference:
const fs = require('fs');
// Blocking (synchronous) read
console.log('Start reading file synchronously...');
const dataSync = fs.readFileSync('example.txt', 'utf8');
console.log(dataSync);
console.log('Finished reading file synchronously');
// Non-blocking (asynchronous) read
console.log('Start reading file asynchronously...');
fs.readFile('example.txt', 'utf8', (err, dataAsync) => {
if (err) throw err;
console.log(dataAsync);
});
console.log('Finished starting async read');
// Output:
// Start reading file synchronously...
// [Contents of example.txt]
// Finished reading file synchronously
// Start reading file asynchronously...
// Finished starting async read
// [Contents of example.txt]
In this example, the synchronous read blocks the execution until the file is read, while the asynchronous read allows the program to continue executing while the file is being read in the background.
Concurrency Handling in Node.js
Interviewer: That's fascinating! But if Node.js is single-threaded, how does it handle concurrency?
Candidate: Great question! Node.js achieves concurrency through its event loop and the use of asynchronous programming patterns. Here's how it works:
Event Loop: The heart of Node.js concurrency model. It continuously checks for events and executes their associated callbacks.
Asynchronous APIs: Most I/O operations in Node.js are asynchronous and non-blocking.
Callback Queue: Completed asynchronous operations are placed in a queue to be processed by the event loop.
Worker Threads: For CPU-intensive tasks, Node.js provides a Worker Threads module to offload work to separate threads.
Here's a simplified visualization of how Node.js handles concurrent operations:
console.log('1. Script starts');
setTimeout(() => console.log('2. Timeout callback executed'), 0);
Promise.resolve().then(() => console.log('3. Promise resolved'));
process.nextTick(() => console.log('4. Next tick callback executed'));
console.log('5. Script ends');
// Output:
// 1. Script starts
// 5. Script ends
// 4. Next tick callback executed
// 3. Promise resolved
// 2. Timeout callback executed
This example demonstrates how Node.js handles different types of asynchronous operations, executing them in a specific order based on the event loop's phases.
V8 Engine and Node.js
Interviewer: You mentioned the V8 engine earlier. How exactly does Node.js utilize it?
Candidate: The V8 engine is a crucial component of Node.js. It's an open-source JavaScript engine developed by Google for the Chrome browser, and Node.js leverages it to execute JavaScript code outside the browser environment.
Here's how Node.js uses the V8 engine:
JavaScript Execution: V8 compiles and executes JavaScript code.
Just-In-Time (JIT) Compilation: V8 compiles JavaScript directly to native machine code before execution, improving performance.
Memory Management: V8 handles memory allocation and garbage collection for Node.js applications.
C++ Bindings: Node.js extends V8's capabilities by adding C++ bindings, allowing interaction with the operating system for tasks like file I/O and networking.
While we can't directly interact with V8 in our Node.js code, we can see its impact through the performance of our applications. Here's a simple benchmark to demonstrate V8's speed:
console.time('loop');
for (let i = 0; i < 1000000; i++) {
// Perform some operation
Math.sqrt(i);
}
console.timeEnd('loop');
// Output: loop: ~10ms
This code executes a million iterations of a mathematical operation in just a few milliseconds, showcasing V8's efficiency.
Performance Improvement for I/O-heavy Applications
Interviewer: Lastly, how does Node.js improve performance for I/O-heavy applications?
Candidate: Node.js is particularly well-suited for I/O-heavy applications due to its non-blocking, event-driven architecture. Here's how it enhances performance:
Asynchronous I/O: Node.js can handle many concurrent connections without the overhead of creating a new thread for each connection.
Event Loop: Efficiently manages I/O operations, ensuring the application remains responsive.
Streaming: Node.js can process data in chunks as it's received, rather than waiting for the entire payload.
Caching: Built-in modules like
fs
provide caching mechanisms to reduce unnecessary I/O operations.
Here's an example of how Node.js can efficiently handle multiple file read operations:
const fs = require('fs');
function readFiles(files) {
files.forEach(file => {
fs.readFile(file, 'utf8', (err, content) => {
if (err) {
console.error(`Error reading ${file}: ${err}`);
return;
}
console.log(`${file} content length: ${content.length}`);
});
});
}
const files = ['file1.txt', 'file2.txt', 'file3.txt'];
readFiles(files);
console.log('All file read operations initiated');
// Output:
// All file read operations initiated
// file2.txt content length: 1234
// file1.txt content length: 5678
// file3.txt content length: 9012
In this example, Node.js initiates all file read operations concurrently, improving overall performance compared to reading files sequentially.
Conclusion
We've covered several fundamental concepts of Node.js, including its key features, non-blocking I/O, concurrency model, use of the V8 engine, and performance benefits for I/O-heavy applications. These characteristics make Node.js a powerful platform for building scalable and efficient server-side applications.
In the next part of this series, we'll explore more advanced topics in Node.js, including REPL, global objects, module loading, and the differences between synchronous and asynchronous code. Stay tuned!
Subscribe to my newsletter
Read articles from Bodheesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bodheesh
Bodheesh
🚀 Bodheesh V C | Software Engineer | Tech Blogger | Vlogger | Learning Enthusiast | Node.js Developer | MERN Stack Advocate As a backend developer with over 2.5 years of experience, I’m passionate about building efficient, scalable systems that drive impactful results. Specializing in Node.js and the MERN stack, I’ve had the opportunity to work on a wide array of projects—from creating seamless APIs and real-time applications to implementing effective codes that enhance performance and scalability. I believe in simplicity and maintainability, striving to write clean, well-documented code that not only solves the problem at hand but also sets the foundation for future growth. My experience spans a variety of domains including backend architecture, database management, API development and cloud integrations. Beyond coding, I have a deep interest in sharing knowledge with the developer community. I actively contribute to blogs and social platforms, simplifying complex concepts around system design, DSA (Data Structures and Algorithms), and interview preparation, so that developers at all levels can benefit. My tech blogs, interview guides, and insights into best coding practices are geared toward helping others grow and succeed in their software development journey. I am also a vlogger, and I’ve created content around tech trends, tutorials, and tools to inspire others to pursue their passion for technology. As a continuous learner, I’m constantly exploring emerging technologies, such as AI-driven development, and finding ways to incorporate these advancements into real-world applications. My enthusiasm for coding, combined with my commitment to lifelong learning, keeps me motivated and excited for the future of software development. What I do: Backend development, specializing in Node.js and MERN stack Creating scalable, high-performance Applications Building RESTful APIs and microservices Writing tech blogs on Node.js, system design, and coding best practices Preparing developers for interviews with practical guidance on DSA and problem-solving Advocating for clean code and maintainable architectures Sharing knowledge through tutorials, blogs, and videos Whether it’s collaborating on an exciting project, learning from fellow developers, or simply discussing the latest in tech, I’m always eager to connect and grow together with the community. Let’s build something great!