Preparing for a Fast-Paced Startup Interview: A Backend Developer Guide

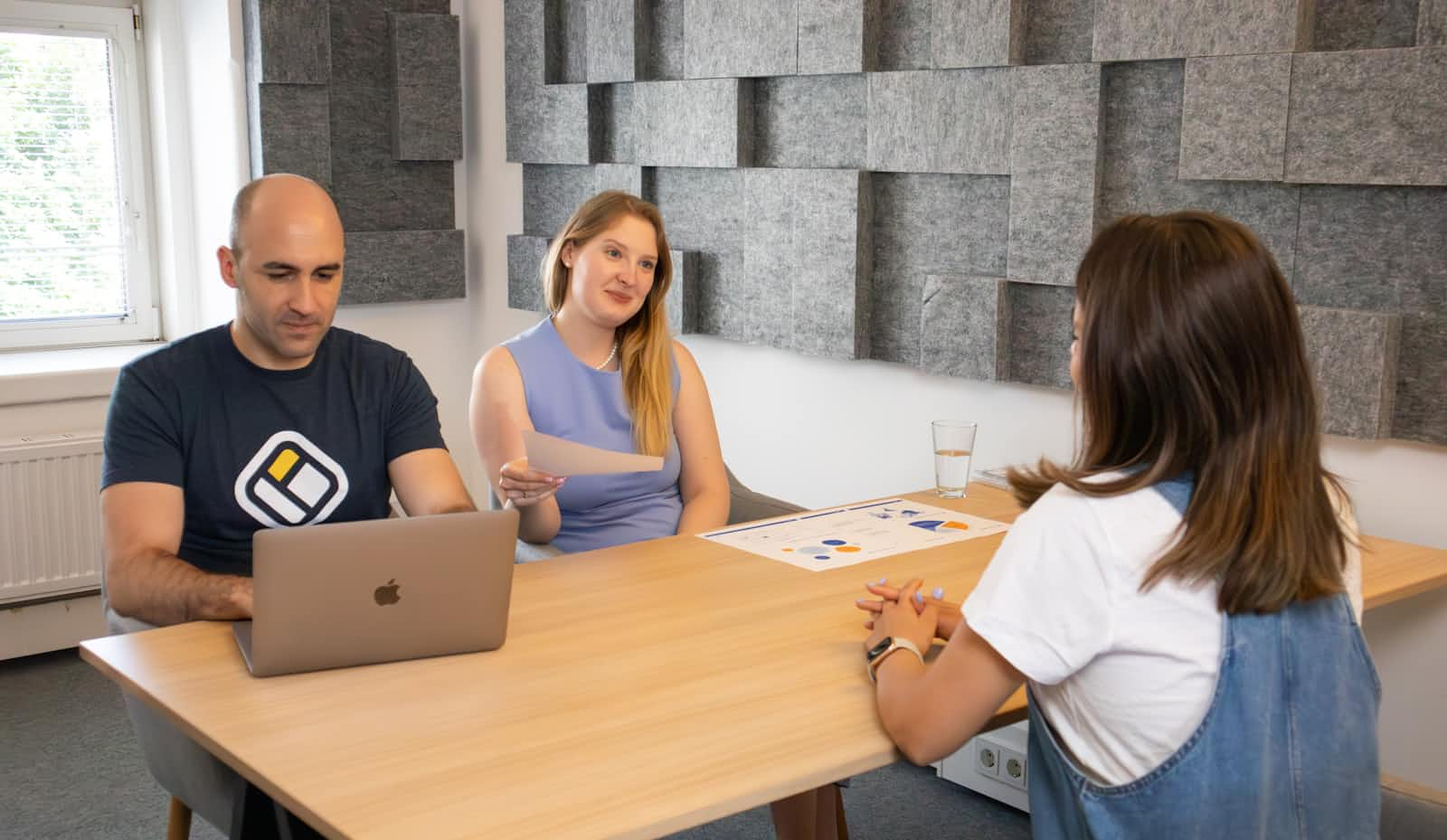
Introduction
Preparing for a developer job interview can feel like a challenging process, but with the right approach, it becomes manageable. Whether you're a senior backend developer or just looking to sharpen your skills, being well-prepared will make all the difference.
In this guide, I’ll share how I believe a developer interview should look and how you can best prepare for it. We'll walk through the typical stages of an interview—from technical assessments to system design and behavioral questions—so you can understand what to expect without feeling unprepared.
This section will provide a broad overview, keeping things simple and focused. We'll explore the core topics to prepare, key skills to brush up on, and how to present yourself confidently throughout the process.
Understanding the Interview Stages
The developer interview process may vary between companies, but it generally follows a familiar structure. Understanding these stages will help you stay ahead and tailor your preparation. Here’s a breakdown of the common stages you’ll likely encounter:
Phone Screen / Initial Interview
This is usually your first interaction with the company, often led by a recruiter or hiring manager. They’ll ask about your experience, why you’re interested in the role, and possibly some light technical questions. Their goal is to ensure your background aligns with the role and to get a sense of your enthusiasm for the job. It’s also your chance to learn more about the company, so don’t hesitate to ask about the team, day-to-day responsibilities, or company culture.
Preparation Tip: Be ready to explain your experience and highlight key projects. Show curiosity about the role and the company. Ask thoughtful questions about the team’s challenges, what success looks like, and the technology stack they use. Let them know you’re excited to contribute and continuously learn on the job.
Technical Assessment
After the phone screen, you’ll usually move on to a coding challenge or technical exercise. These might involve algorithmic problems, real-world coding tasks, or a take-home project. The aim is to assess your problem-solving skills, technical fluency, and approach to tackling tasks relevant to the job.
Preparation Tip: Focus on problem-solving and practical application of your coding skills. Make sure to brush up on fundamental algorithms and data structures, but also think about how you’d approach real-world challenges. During the interview, explain your thought process clearly and show how you adapt to new problems. Companies appreciate candidates who are self-learners and can get the job done.
System Design Interview
For senior roles, particularly backend developers, the system design interview is crucial. You’ll be asked to design a scalable, efficient system while discussing trade-offs, performance, and reliability. This stage tests not only your technical expertise but also your ability to break down complex problems and design systems that solve real-world needs.
Preparation Tip: Approach the system design interview with a practical mindset. Think about systems you’ve worked on and how you’d improve them. Focus on key areas like scalability, database management, and fault tolerance. Don’t forget to ask questions—understanding the context and specific requirements for the system shows you’re engaged and thoughtful about the design process.
Behavioral Interview
While technical skills are vital, companies also want to know how you work with others and handle challenges. In the behavioral interview, you’ll be asked about how you approach problem-solving, leadership, and teamwork. It’s a chance to demonstrate how your experience aligns with the company’s values and culture.
Preparation Tip: Use the STAR method (Situation, Task, Action, Result) to structure your answers. Focus on examples that showcase your ability to collaborate, adapt, and learn quickly. Highlight times when you took initiative, learned something new, or solved a tough problem. This is also an opportunity to show you’re proactive and a self-learner who can adapt to the evolving demands of a role.
Culture Fit / Soft Skills Assessment
This is often the final stage, where the focus shifts to whether you’ll be a good fit for the team and the company culture. The interviewers will gauge how you communicate, collaborate, and align with their values. They’ll also want to see if you’re genuinely interested in contributing to their mission.
Preparation Tip: Be authentic and open about your working style. Show an interest in how the team operates day-to-day and how you could fit into that environment. Emphasize your ability to learn on the job, your problem-solving mindset, and how you approach getting things done efficiently. Ask questions that show you're thinking long-term, like the company’s growth plans or how they tackle new challenges.
Core Topics to Prepare
When preparing for a senior backend developer interview, having a solid grasp of the core concepts and technologies is essential. This isn’t just about solving coding problems—it’s about demonstrating your ability to build, maintain, and scale complex systems. Here are the key topics you should focus on:
Scalability
One of the most important areas you’ll be assessed on is your understanding of scalability. As systems grow, they need to handle increasing loads efficiently without sacrificing performance. Expect questions around designing systems that scale both horizontally and vertically, and how you would handle challenges like load balancing and redundancy.
Preparation Tip: Review concepts like sharding, replication, and distributed systems. Be ready to explain how you’ve scaled systems in the past, or how you would approach scaling a new system. Demonstrating an understanding of both the theory and the practical application of scalability is key.
Database Management
Backend systems often revolve around data, so you’ll need to show strong knowledge of databases, both SQL and NoSQL. You’ll likely be asked about database design, optimization techniques, and how to handle large datasets. This might include topics like indexing, query optimization, and database scaling.
Preparation Tip: Brush up on database design best practices. Understand the trade-offs between SQL and NoSQL databases, and be prepared to talk through scenarios where one is more suitable than the other. Discuss any experience you have with replication, partitioning, and performance tuning.
API Design
A large part of a backend developer’s role is designing and maintaining APIs. You’ll need to show that you can create APIs that are both scalable and easy to use. Questions may focus on the differences between REST and GraphQL, API versioning, and ensuring backwards compatibility.
Preparation Tip: Make sure you’re comfortable with designing APIs that balance usability and performance. Know the pros and cons of different approaches (REST vs. GraphQL), and be able to explain how you’d handle versioning and backward compatibility in a real-world scenario. Companies value developers who can create well-thought-out APIs that other teams or developers will find intuitive and maintainable.
Microservices Architecture
Microservices have become a key architectural pattern for backend systems, so expect questions about how to design, build, and manage them. You may need to discuss service-to-service communication, data consistency, and strategies for fault tolerance.
Preparation Tip: Prepare to talk about the benefits and challenges of microservices compared to monolithic architecture. Highlight your experience in building services that are independently deployable and how you handle the complexity that comes with service-to-service communication. Show your understanding of patterns like circuit breakers, message queues, and event-driven architectures.
DevOps Knowledge
DevOps is an increasingly important part of a backend developer’s role, especially as teams move towards continuous integration and deployment (CI/CD). You should have a working understanding of how to build and maintain CI/CD pipelines, infrastructure as code, and containerization technologies like Docker and Kubernetes.
Preparation Tip: Review how you’ve integrated DevOps practices into your workflow. Talk about the tools you’ve used for CI/CD, any experience with containerization, and how you’ve ensured a smooth deployment process. Be prepared to discuss infrastructure as code (using tools like Terraform or Ansible), and how you’ve contributed to system reliability and scalability.
Security Best Practices
Security is a crucial area for any backend developer, and interviewers will likely test your knowledge of securing APIs, encrypting data, and implementing authentication and authorization systems. This includes topics like OAuth2, JWT, and general secure coding practices.
Preparation Tip: Be familiar with security best practices and know how to implement secure authentication and authorization mechanisms. Discuss how you’ve handled securing data in transit and at rest, and be ready to explain the steps you’ve taken to mitigate common vulnerabilities (e.g., SQL injection, cross-site scripting).
Event-Driven Architecture
With the rise of distributed systems, event-driven architecture has become more prevalent. You might be asked about technologies like Kafka or RabbitMQ, and how you handle asynchronous processing and event streams.
Preparation Tip: If you’ve worked with event-driven systems, highlight how you managed asynchronous communication, message brokering, and event sourcing. Be able to explain when an event-driven architecture is appropriate and how you ensure data consistency and fault tolerance in such systems.
Performance Optimization
As a senior developer, you’ll be expected to understand performance bottlenecks and how to address them. This could include profiling applications, optimizing database queries, caching, and reducing latency in network communication.
Preparation Tip: Be ready to talk through specific examples of how you’ve optimized the performance of an application. Discuss the tools you use to monitor and profile performance and explain strategies like caching, load balancing, or improving database query efficiency.
Cloud Platforms
Finally, cloud platforms like AWS, Azure, or GCP are integral to modern backend development. You’ll need to show you can work with cloud infrastructure, including services like serverless computing, databases, and networking.
Preparation Tip: Review the cloud services you’ve used and how they fit into the systems you’ve built. Focus on areas like auto-scaling, load balancing, and serverless architectures. Be ready to explain how you’d design a cloud-based solution from the ground up, considering cost, performance, and scalability.
How to Present Yourself
Beyond technical skills, how you present yourself during the interview is just as important. Being able to communicate clearly, collaborate effectively, and show your curiosity and passion for the role makes a lasting impression. Here's how you can stand out during the interview process:
Demonstrate Curiosity
Interviewers appreciate candidates who are genuinely curious about the role, the team, and the company. Show that you're not just looking for any job, but that you’re interested in what the company does and how you can contribute. Ask thoughtful questions about the product, the technology stack, or the challenges the team is facing.
Tip: Prepare a few questions ahead of time based on the job description or research about the company. Focus on areas that are relevant to the role—such as their approach to scalability, their tech roadmap, or how they handle code reviews. This shows you’re actively thinking about how you can make an impact.
Communicate Clearly
Clear communication is critical for any developer role, especially when explaining technical concepts to non-technical stakeholders or collaborating with team members. During the interview, make sure to articulate your thoughts step-by-step, especially when walking through your problem-solving process or system design decisions.
Tip: When explaining your thought process, avoid jumping straight to the solution. Instead, break it down—start with the problem, explore the different approaches you considered, and explain why you made certain choices. This not only showcases your problem-solving skills but also demonstrates your ability to collaborate with others effectively.
Be a Self-Learner
One of the most valued traits in developers is the ability to learn on the job. Technology evolves quickly, and companies look for candidates who stay current and can quickly adapt to new tools and frameworks. During the interview, highlight any instances where you taught yourself something new, picked up a new technology, or found a solution on your own.
Tip: Talk about any side projects, new technologies you’ve explored recently, or courses you’ve taken. Showing that you’re a proactive learner tells the interviewer that you won’t need your handheld to pick up new skills.
Show Enthusiasm for Problem-Solving
At the core of any developer role is solving problems. Companies want developers who not only have the skills to write code but who enjoy diving into challenges and finding elegant solutions. Show that you love tackling problems, especially those relevant to the company or the role you’re applying for.
Tip: When discussing your previous work, focus on the problems you solved rather than just the technologies you used. Describe how you approached the challenge, what obstacles you faced, and how you ultimately delivered the solution. Enthusiasm for solving real-world problems goes a long way in making you a memorable candidate.
Focus on Getting the Job Done
Companies want to hire developers who are results-oriented and get things done. Talk about your ability to deliver, meet deadlines, and work under pressure. Senior backend roles often involve juggling multiple priorities, so demonstrating your ability to stay focused and complete tasks efficiently will make a strong impression.
Tip: Use specific examples from your past work to highlight your reliability. Mention how you prioritized tasks, managed your time, or how you handled challenges to ensure a project was completed successfully.
Highlight Collaboration and Leadership
As a senior developer, your role will often involve leading others or working closely with cross-functional teams. Show that you can collaborate effectively and mentor junior developers. Talk about how you’ve contributed to the success of your team, whether by leading projects, providing guidance, or improving processes.
Tip: If you’ve been a mentor or led a team, mention how you helped junior developers grow or how you drove initiatives to improve team performance. Even if you’ve worked more independently, focus on your ability to collaborate with other teams (e.g., product, design, or QA) to deliver high-quality outcomes.
Common Pitfalls to Avoid
Even with strong preparation, there are a few common mistakes that can trip up even experienced developers during interviews. Being aware of these pitfalls can help you stay on track and make the best impression possible:
Overcomplicating Solutions
One common mistake in technical interviews is over-engineering a solution. When faced with a problem, it’s tempting to dive into complex architectures or try to showcase advanced knowledge. However, interviewers are often looking for clear, concise, and efficient solutions, not just technical depth.
Tip: Keep it simple. Start with the most straightforward solution and explain your reasoning. If there’s room for optimization, mention it as a next step. This shows that you can prioritize simplicity while also being aware of potential improvements.
Ignoring the Problem's Scope
Sometimes candidates get so caught up in solving the problem that they miss out on understanding the scope of the task. Whether it’s a coding exercise or a system design question, you need to clarify the requirements before jumping into a solution. This ensures you’re solving the right problem and meeting the interviewer's expectations.
Tip: Ask questions before you start coding or designing. Clarify any assumptions and make sure you fully understand the problem. This not only demonstrates your attention to detail but also shows that you’re thoughtful about how you approach tasks.
Lack of Communication
Even if you can solve a problem perfectly, failing to communicate your thought process can hurt your chances. Interviewers are not just interested in your solution but in how you approach the problem, break it down, and consider alternatives. A lack of communication leaves interviewers guessing
Tips for Success
As you prepare for your developer interview, keep these final tips in mind to maximize your chances of success:
Practice, Practice, Practice
The best way to prepare for technical interviews is through consistent practice. Regularly solving coding challenges on platforms like LeetCode, HackerRank, or CodeSignal can sharpen your skills and help you become familiar with different types of problems.
Tip: Set aside dedicated time each week to practice coding challenges, focusing on different topics each session. This will help you build a strong foundation and improve your confidence as you approach your interview.
Mock Interviews
Participating in mock interviews can be incredibly beneficial. They simulate the real interview environment and help you practice articulating your thought process under pressure. It’s also an opportunity to receive constructive feedback from peers or mentors.
Tip: Use platforms like Pramp or Interviewing.io to schedule mock interviews. Alternatively, find a friend or colleague to practice with, allowing you to gain different perspectives and improve your approach.
Stay Calm and Collected
Interviews can be stressful, but it’s important to remain calm and composed. If you encounter a challenging question, take a moment to gather your thoughts before responding. It’s perfectly fine to think out loud or ask for clarification if needed.
Tip: Practice mindfulness or breathing exercises to help manage anxiety. Remind yourself that it’s a conversation, not an interrogation, and that the interviewers are interested in getting to know you as a candidate.
Learn from Every Experience
Whether you receive an offer or not, each interview is a valuable learning opportunity. Reflect on what went well and what could be improved. If you get feedback, take it to heart and use it to refine your approach for future interviews.
Tip: Keep a journal to track your interview experiences. Note down questions asked, your responses, and any feedback received. This can help you identify patterns and areas for improvement as you continue your job search.
A Practical Example
In this section, we’ll walk through a typical interview scenario, illustrating how a conversation might unfold as you demonstrate your technical knowledge and problem-solving skills. We’ll focus on a familiar product: a URL shortener for this example.
Introduction and Product Overview
After some initial guidelines, I introduce the concept of the URL shortener. Most candidates are familiar with this kind of application, making it a great starting point for discussion.
Step 1: Data Structure Planning
Next, I’ll ask you to plan the data structure for our URL shortener app. This is where you can showcase your understanding of how to model entities and their relationships.
What to Consider:
Clearly define the entities involved (e.g., URLs, users, click stats).
Discuss how you would store this data (e.g., relational database vs. NoSQL).
Explain the relationships between entities (e.g., one-to-many between users and URLs).
Tip: Be prepared to articulate your choices and the reasoning behind your decisions. This demonstrates your ability to think critically about data modeling.
Step 2: API Design
Once we’ve established a solid data structure, I’ll shift the focus to the API design. You’ll need to outline all the API routes our application will have, keeping it simple by just mentioning the URL and HTTP methods.
What to Consider:
Standard endpoints like
POST /urls
for creating a short URL,GET /urls/{id}
for retrieving a URL, etc.Discuss HTTP status codes (e.g., 200 OK, 404 Not Found) and their relevance.
Tip: Highlight any common practices you follow when designing APIs, such as versioning and error handling, and be prepared to discuss URL encoding/decoding and best practices for structuring URLs.
Step 3: Detailed API Specification
After verifying your understanding of API design, we’ll choose a few endpoints and ask you to provide a full specification. This includes all parameters, input/output formats, error handling, and tools like Swagger for API documentation.
What to Consider:
Discuss the types of data you expect in requests and responses (e.g., JSON format).
Define possible error responses (e.g., 404 Not Found, 400 Bad Request) and what they signify.
Tip: Incorporate examples to illustrate your points, as this can clarify your thought process.
Step 4: Implementing Controllers
Next, we’ll move on to implementation. I’ll ask you to write pseudo code for two entity controllers (e.g., URL and stats controllers) and one user/auth controller.
What to Consider:
Focus on structure and logic rather than syntax perfection.
Think about how you would validate input, handle errors, and ensure security.
Tip: While writing pseudo code, explain your approach to handling user authentication, including concepts like JWT, SSO, session management, and cookies.
Step 5: Coding Questions
Once you’ve laid out the pseudo code, we’ll delve deeper into the user/auth controller. I’ll ask questions about designing the login system and various authentication strategies.
What to Consider:
Discuss how to securely manage user credentials and sessions.
Explain your approach to implementing token-based authentication (JWT) and any relevant security measures.
Tip: This is a chance to demonstrate your understanding of both the technical and security aspects of user authentication.
Step 6: System Design Discussion
As we near the end of the interview, we’ll shift to system design. I’ll ask you about how you would scale the application and explore different scaling strategies (e.g., horizontal vs. vertical scaling).
What to Consider:
Discuss the use of load balancers, caching strategies, and security measures like WAF (Web Application Firewall).
Address potential performance issues, such as slow response times when users request short URLs, and how you would optimize those.
Tip: Be prepared to discuss trade-offs between different design choices and their implications for the system.
Step 7: CI/CD and Deployment Questions
Before wrapping up, we’ll discuss CI/CD practices and deployment strategies. This includes questions about techniques like blue-green deployments and ensuring zero downtime during releases.
What to Consider:
Discuss your experience with Git workflows and how they integrate into your deployment process.
Explain how you would manage rollbacks and monitor deployments for issues.
Explain how you would deploy this application to the cloud, the services you will use, and why.
Tip: Be prepared to share specific examples of CI/CD pipelines you’ve set up or worked with in the past.
Step 8: Experience and Project Discussion
Finally, I’ll invite you to discuss your previous experience and technical decisions. I might ask you to describe a complex project from your previous job, focusing on the technical challenges you faced and how you overcame them.
What to Consider:
Prepare to discuss specific technologies you used and the rationale behind your choices.
Be ready to reflect on your contributions to the project and any lessons learned.
Tip: Use this opportunity to connect your past experiences to the role you’re applying for, demonstrating how your background aligns with the company’s needs.
Sep 9: Closing
As we wrap up the interview, I’ll invite any final questions you have about the role or the company. This is your chance to clarify any details and show your enthusiasm for the position.
Final Tip: Tailor your questions to reflect your interest in the company’s culture, team dynamics, and future projects. A well-rounded closing reinforces your suitability for the fast-paced startup environment.
Additional Tips
Practice with Real-World Projects
To solidify your skills and demonstrate your capabilities to potential employers, consider building personal projects based on common products like URL shorteners, social networks, or other applications. This hands-on experience allows you to explore different technologies, architectures, and problem-solving approaches.
Focus on Key Areas
When building projects, concentrate on areas that align with your career goals and the types of roles you're targeting. For example, if you're interested in backend development, focus on building scalable APIs, efficient databases, and robust microservices architectures.
Create a Portfolio
Showcase your projects and skills by creating a portfolio. This can be a website, GitHub repository, or a combination of both. Include detailed descriptions of your projects, highlighting the technologies you used, challenges you overcame, and the outcomes you achieved.
Leverage Coding Challenges
Coding challenges platforms like LeetCode, HackerRank, and CodeSignal offer a structured way to practice problem-solving and coding skills. By regularly solving these challenges, you'll improve your ability to think critically, write efficient code, and approach technical problems confidently.
Continuously Learn and Grow
The field of software development is constantly evolving. Stay up-to-date with the latest trends, technologies, and best practices by reading blogs, attending conferences, and taking online courses. This commitment to lifelong learning will make you a more valuable asset to any company.
Remember: The key to success in developer interviews is a combination of technical knowledge, problem-solving skills, and effective communication. By following these guidelines and practicing consistently, you'll be well-prepared to impress potential employers and land your dream job.
Subscribe to my newsletter
Read articles from Nir Adler directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nir Adler
Nir Adler
HI there 👋 I'm Nir Adler, and I'm a Developer, Hacker and a Maker, you can start with me a conversation on any technical subject out there, you will find me interesting.