#6.0 What XPath: Common Patterns
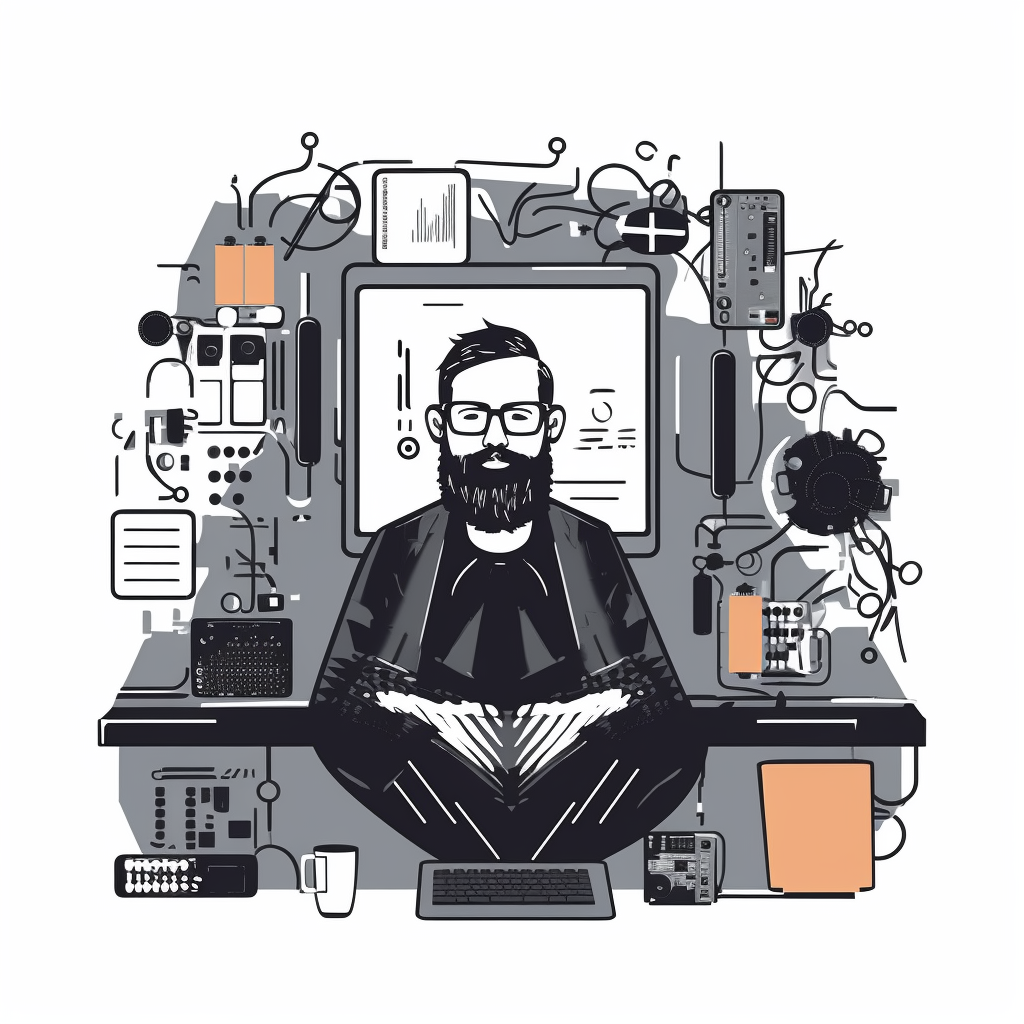
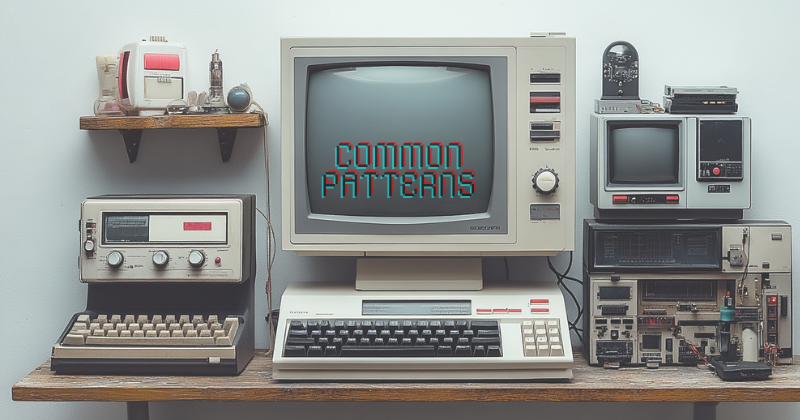
In web automation, certain XPath patterns are frequently used to interact with common web elements. This section will cover practical XPath techniques for locating and interacting with buttons, links, forms, input fields, tables, and lists.
6.1 Locating Buttons and Links
Buttons
By exact text:
//button[text()='Submit']
By partial text:
//button[contains(text(), 'Submit')]
By title attribute:
//button[@title='Submit Form']
By class:
//button[contains(@class, 'submit-btn')]
Image buttons:
//input[@type='image' and @alt='Submit']
Links
By exact text:
//a[text()='Home']
By partial text:
//a[contains(text(), 'Account')]
By href attribute:
//a[contains(@href, 'account/settings')]
By title attribute:
//a[@title='Go to Homepage']
Combining attributes:
//a[@href='/login' and contains(@class, 'nav-link')]
6.2 Working with Forms and Input Fields
Form Elements
Locating a form by ID:
//form[@id='login-form']
Finding inputs within a specific form:
//form[@id='login-form']//input
Input Fields
By name attribute:
//input[@name='username']
By placeholder text:
//input[@placeholder='Enter your email']
By label text (assuming proper HTML structure):
//label[text()='Email:']/following-sibling::input
Checkbox by label:
//label[contains(text(), 'Remember me')]/input[@type='checkbox']
Radio button by value:
//input[@type='radio' and @value='male']
Select Dropdowns
Locating a select element:
//select[@name='country']
Finding specific options:
//select[@name='country']/option[text()='United States']
Selecting by value attribute:
//select[@name='country']/option[@value='US']
6.3 Navigating Tables and Lists
Tables
Selecting a specific cell by row and column index:
//table[@id='data-table']/tbody/tr[2]/td[3]
Finding a row containing specific text:
//table[@id='data-table']//tr[contains(., 'Specific Text')]
Selecting a cell based on column header:
//table[@id='data-table']//th[text()='Name']/following-sibling::td
Selecting the last row of a table:
//table[@id='data-table']/tbody/tr[last()]
Finding a cell with specific text and getting its row index:
count(//table[@id='data-table']//td[text()='Target Text']/parent::tr/preceding-sibling::tr) + 1
Lists
Selecting all list items:
//ul[@class='menu-items']/li
Finding a specific list item by text:
//ul[@class='menu-items']/li[text()='About Us']
Selecting the first item of a list:
(//ul[@class='menu-items']/li)[1]
Selecting items after a specific item:
//li[text()='Target Item']/following-sibling::li
Selecting nested list items:
//ul[@class='parent-menu']//li
6.4 Handling Dynamic Content
Elements with dynamic IDs (using starts-with):
//div[starts-with(@id, 'message-')]
Finding elements with multiple possible classes:
//div[contains(@class, 'message') or contains(@class, 'notification')]
Locating elements that appear after AJAX calls (using wait conditions):
//div[@id='result' and contains(@style, 'display: block')]
6.5 Best Practices
Use specific attributes when possible (id, name, etc.) for faster and more reliable locators.
Avoid using indexes unless necessary, as they can break easily if the page structure changes.
Use contains() for classes when dealing with dynamic or multiple classes.
Combine multiple attributes or conditions to create more robust locators.
When working with tables, try to use relationships between elements (like headers to data cells) rather than hard-coded indexes.
For forms, utilize the relationship between labels and input fields when possible.
Always consider the possibility of dynamic content and use appropriate wait strategies in your automation scripts.
Remember, while these XPath patterns are common and useful, the best XPath for your specific use case will depend on the structure of the website you're automating. Always analyze the HTML structure and choose the most reliable and maintainable locator strategy.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
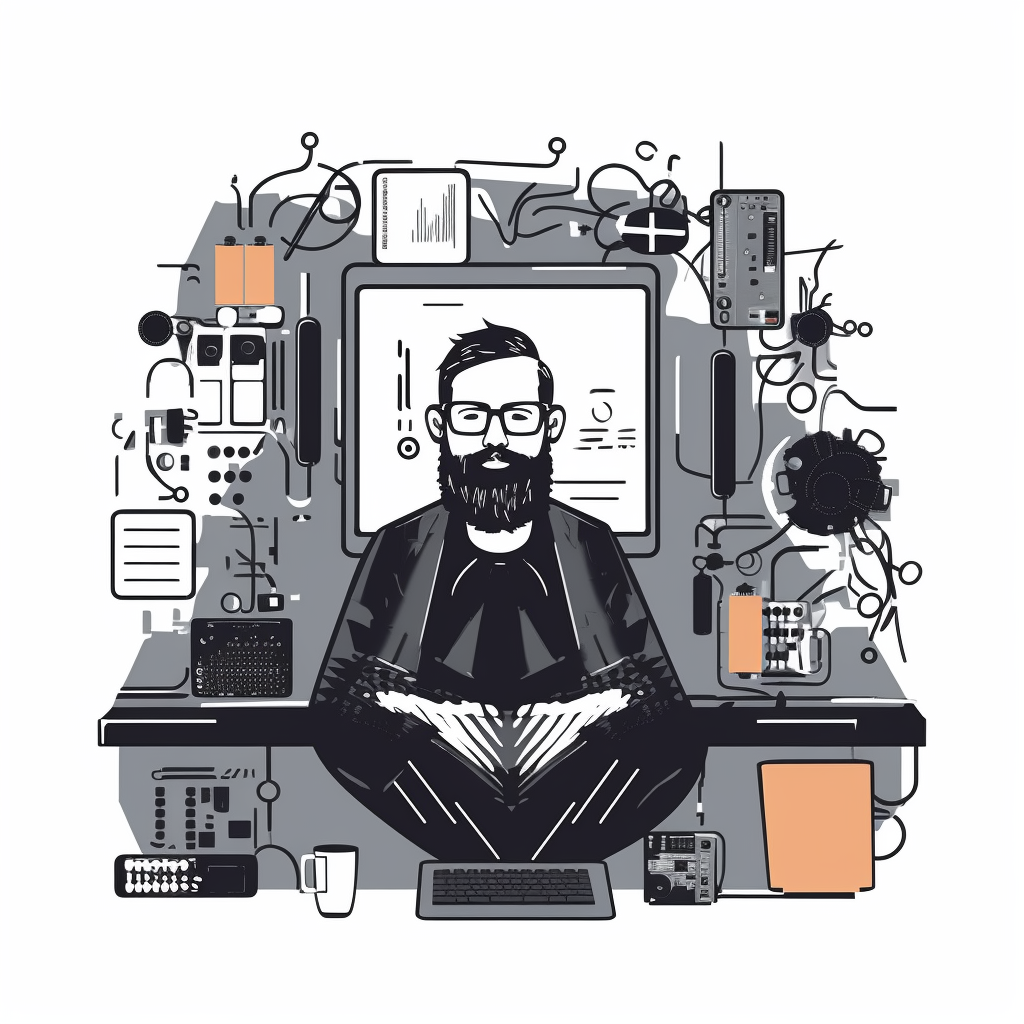
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.