Why You Should Avoid Reflection in C# Unit Testing: Best Practices for Robust and Maintainable Code
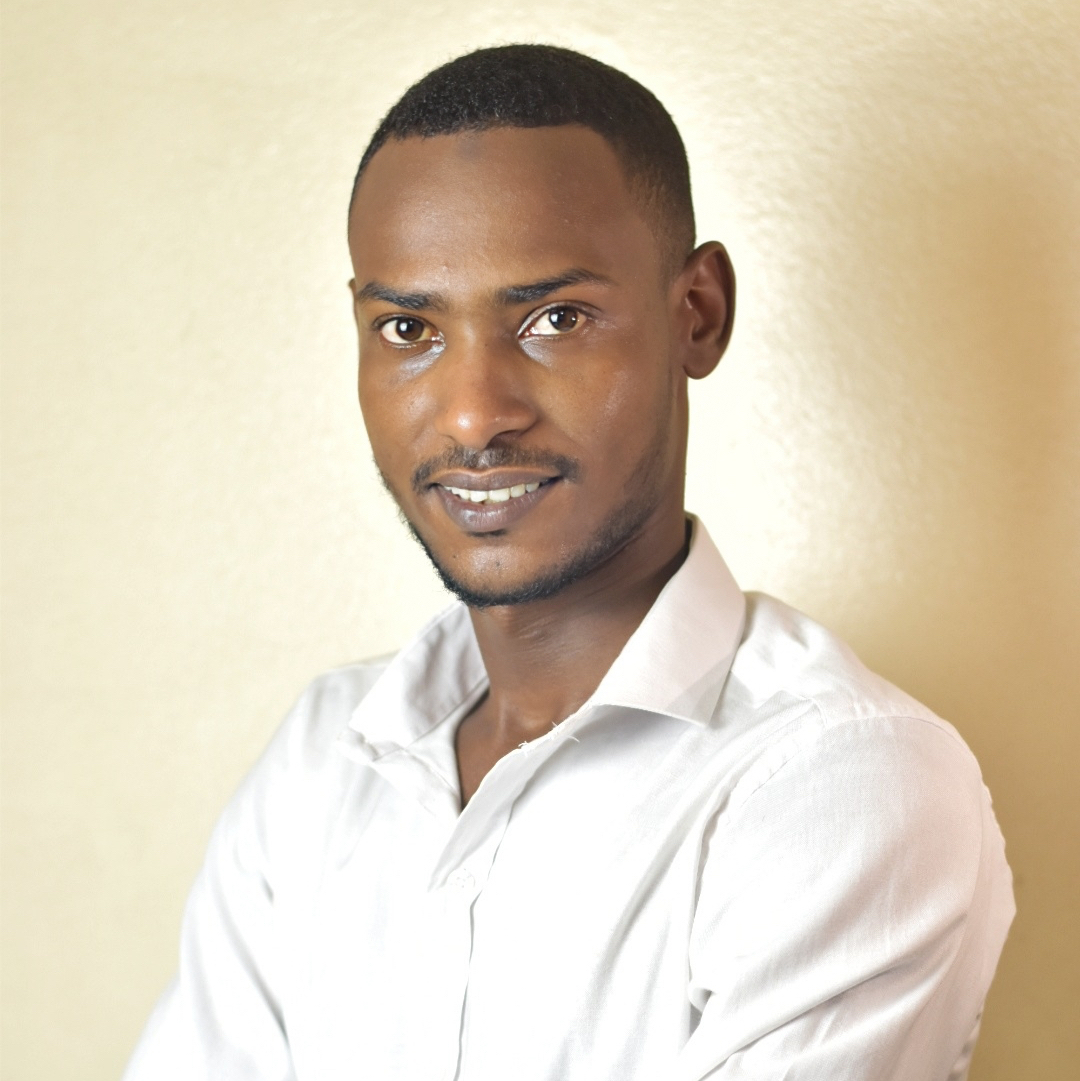
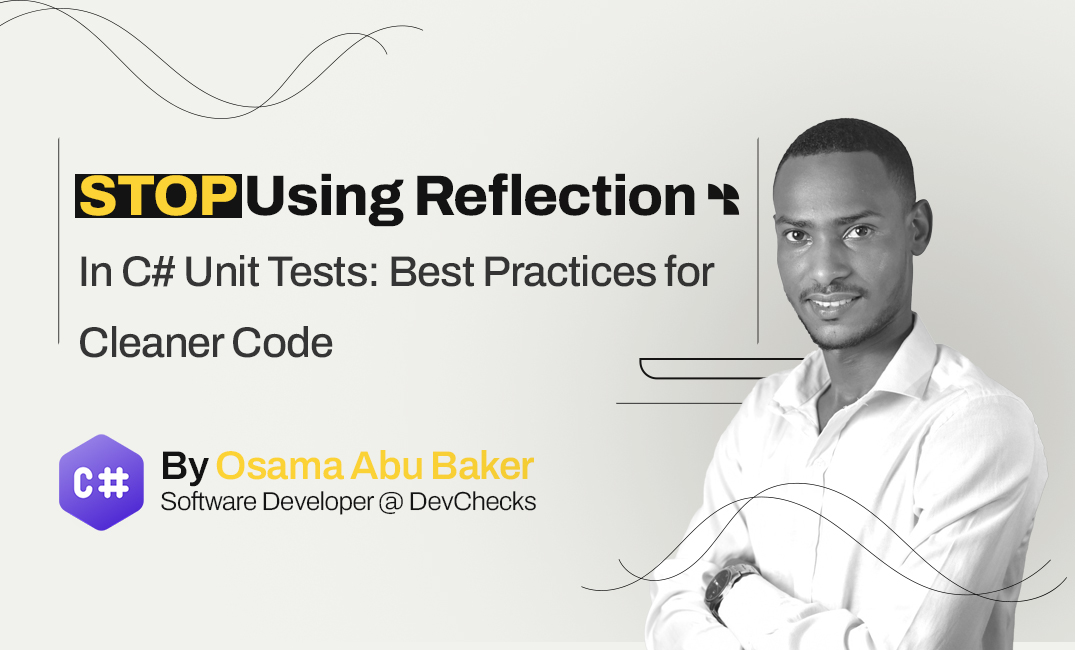
Reflection is a powerful feature in C# that allows you to inspect and interact with types at runtime. While it can be a lifesaver in specific scenarios, especially in dynamic programming, using reflection in unit tests is generally discouraged. In this article, we’ll dive into why reflection should be avoided in unit testing, what makes it problematic, and what alternatives exist for C# developers to create more robust, maintainable, and efficient tests.
What is Reflection in C#?
Reflection is a whole area of computing provides the ability to inspect metadata about classes, methods, properties, and fields at runtime. other folks call it late-binding
. Whether the typical approach to inspecting classes, methods, fields and properties in other DLL’s at compile time by adding the DLL as a reference to the project and importing it via using
declarations and it called early-binding
.
The reflection allows you to access all members that would normally be hidden from external access. While this is useful in some scenarios, such as framework development or debugging, its application in unit testing comes with significant downgrades.
The scenario that we are experiencing here is that we have a private methods within a class and it appears as uncovered code in our test measurements tool (Sonarqube) which it prevents us from going live as per our company guideline’s and all test files within other project.
We have a ClientGenerator
class which has a method within a private access modifier called GenerateSecretKey
and it returns a string secret key.
public class ClientGenerator
{
private string GenerateSecretKey(Environment environment)
{
string secretKey = string.Empty;
// Secret key generation logic
return secretKey;
}
}
We intend to test this method however we can’t access a private method, the solution here is using reflection in C# to invoke a private method:
For learning sake I have dismissed all conventions and best practices like Dependency Injection
.
[Fact]
public void TestPrivateMethodUsingReflection()
{
var clientGenerator = new ClientGenerator();
var methodInfo = typeof(ClientGenerator).GetMethod("GenerateSecretKey", BindingFlags.NonPublic | BindingFlags.Instance);
Environment env = new Environment();
var result = methodInfo.Invoke(clientGenerator, new object[] { env });
Assert.NotEqual(string.Empty, result);
}
While this test passes, it relies on reflection to access the GenerateSecretKey
method, which is private. Although this works, it introduces many risks and inefficiencies. Let’s explore why this practice is discouraged.
Why Reflection is Not Recommended in Unit Testing?
1- Breaks Encapsulation
One of the foundational principles of object-oriented programming is encapsulation. By hiding internal details, we ensure that objects manage their own state and behavior. When using reflection in unit tests, you bypass this encapsulation, accessing methods that are deliberately hidden.
Key Problem: Accessing private methods in tests defeats the purpose of encapsulation, making internal details available to the outside world. This can make tests more fragile and harder to maintain.
2- Leads to Fragile Tests
Private methods and internal logic are subject to frequent changes during refactoring or code improvement. If your tests depend on these private methods via reflection, you risk breaking your tests whenever internal logic changes, even if the overall behavior of the class remains the same.
Example:
[Fact]
public void TestPrivateMethodUsingReflection()
{
var clientGenerator = new ClientGenerator();
var methodInfo = typeof(ClientGenerator).GetMethod("GenerateSecretKey", BindingFlags.NonPublic | BindingFlags.Instance);
Environment env = new Environment();
// If the method name changes, this test breaks, even if the functionality is the same
var result = methodInfo.Invoke(clientGenerator, new object[] { env });
Assert.NotEqual(string.Empty, result);
}
In this case, if GenerateSecretKey
is renamed or refactored, the test will fail, even though the functionality might be correct when tested through public methods.
3- Slower Execution
Reflection is inherently slower than direct method calls because of the overhead of inspecting metadata and invoking methods dynamically. In large test suites, this added time can cause noticeable performance degradation and as a side effects it will increase the deployment duration.
4- Overcomplicates Test Code
Using reflection introduces unnecessary complexity in your test code. Instead of simple, readable assertions, you need to write verbose reflection code that’s harder to understand and maintain.
Example comparison:
Using reflection:
var methodInfo = typeof(ClientGenerator).GetMethod("GenerateSecretKey", BindingFlags.NonPublic | BindingFlags.Instance);
var result = methodInfo.Invoke(clientGenerator, new object[] { env });
Assert.NotEqual(string.Empty, result);
Versus normal test code:
var result = clientGenerator.PublicGenerateSecretKey(env);
Assert.NotEqual(string.Empty, result);
The latter is much cleaner and easier to understand, reducing the cognitive load for future developers working on the test suite.
5- Encourages Testing Implementation Rather than Behavior
In unit testing, the focus should always be on testing behavior rather than implementation details. Private methods are part of the implementation, while public methods define the behavior. By testing private methods, you risk coupling your tests to how the class achieves its functionality rather than what it does.
Best Practice: Test the public interface of your class and avoid testing private methods. The behavior of the class should be verified through the public methods, which may internally use private methods. This ensures your tests are more robust and aligned with the purpose of the class.
Alternatives to Testing Private Methods
1- Refactor Your Code
If a private method contains significant logic that you feel must be tested, consider refactoring it into a separate class or method that is public
or internal
. This allows you to test the logic directly without exposing unnecessary details.
Example:
public class SecretKeyGeneratorHelper
{
public string GenerateSecretKey(Environment environment)
{
string secretKey = string.Empty;
// Secret key generation logic.
return secretKey;
}
}
Now, instead of testing a private method in ClientGenerator
, you can test the GenerateSecretKey
method in SecretKeyGeneratorHelper
, which is a clean, reusable piece of code.
2- Test Through Public Methods
In most cases, private methods are invoked by public methods. You can test the public methods to ensure that the private methods are working as expected.
Example:
public class ClientGenerator
{
private string GenerateSecretKey(Environment environment)
{
string secretKey = string.Empty;
// Secre Key generation logic.
return secretKey;
}
public string GenerateSecretKeyForTest(Environment environment)
{
return GenerateSecretKey(environment);
}
}
[Fact]
public void TestPublicMethod()
{
var clientGenerator = new ClientGenerator();
Environment env = new Environment();
var result = clientGenerator.GenerateSecretKeyForTest(env);
Assert.NotEqual(string.Empty, result);
}
Here, the private GenerateSecretKey
is indirectly tested through the GenerateSecretKeyForTest
.
3- Use InternalsVisibleTo
Attribute
If making a method internal
is an option, you can use the InternalsVisibleTo
attribute to expose internal methods to your test project. This allows you to test internal methods without violating encapsulation.
Example:
Add this to AssemblyInfo.cs
:
[assembly: InternalsVisibleTo("YourTestProject")]
Then, change the private method to internal
, and it will be testable from your unit tests.
4- Use Protected Methods and Inheritance
If the private method is designed to be used in a class hierarchy, consider changing its visibility to protected
. You can then create a test subclass to verify its behavior.
Conclusion: Keep Your Tests Simple and Focused
Reflection may seem like a quick fix to test private methods, but in the long run, it makes your tests brittle, slow, and hard to maintain. Instead, focus on refactoring your code, testing behavior through public methods, and keeping your unit tests clean and efficient.
By adhering to these principles, you’ll write more reliable, maintainable tests that provide real value and protect your code from breaking changes.
Subscribe to my newsletter
Read articles from Osama Abu Baker directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
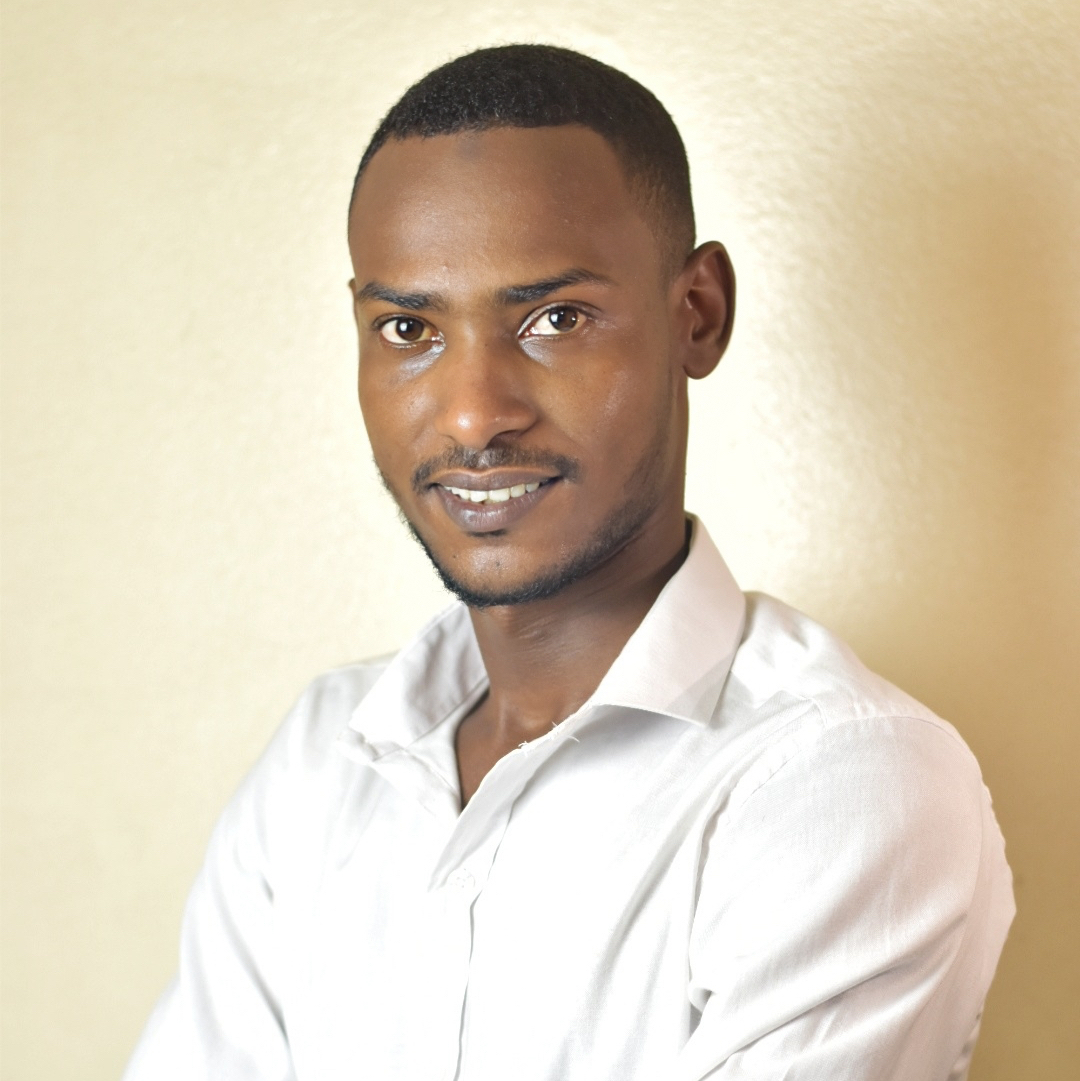
Osama Abu Baker
Osama Abu Baker
A versatile and dedicated developer with a keen eye for detail and a passion for creating efficient, high-quality software solutions. Always eager to learn and adopt new technologies, aiming to drive innovation and excellence in every project.