Automating Office Tasks (Excel, PDFs, Emails) Using Python: A Comprehensive Guide
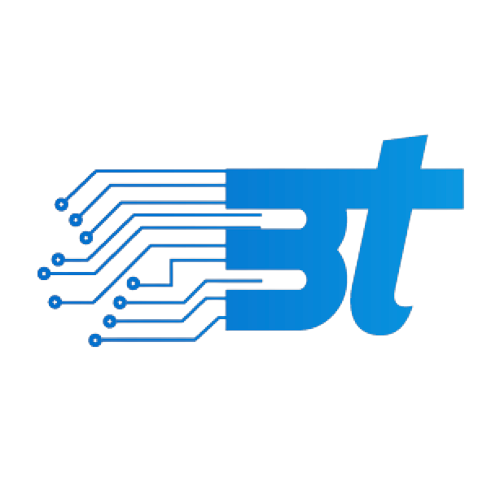

Automating office tasks is becoming increasingly important for businesses looking to improve efficiency and productivity. Python, with its versatile libraries and ease of use, is one of the most powerful tools for automating routine tasks. This extensive guide explores how Python can automate office tasks like manipulating Excel files, handling PDFs, and automating email workflows.
1. Introduction to Office Task Automation
Every day, businesses handle countless repetitive tasks like updating spreadsheets, generating reports, reading through documents, and responding to emails. These tasks, while crucial, can be time-consuming. Automating these office tasks can lead to significant time savings and reduce human errors.
Python is ideal for these tasks due to its ease of integration, extensive libraries, and large support community. By automating office workflows, you can free up time to focus on more strategic work, ultimately increasing productivity and efficiency.
2. Benefits of Automating Office Tasks
Automation in office environments provides a host of benefits, including:
Time Savings: Automation reduces the time spent on repetitive manual tasks, allowing employees to focus on higher-value work.
Improved Accuracy: Automating tasks like data entry and report generation reduces the likelihood of human error.
Increased Productivity: With mundane tasks automated, employees can work more efficiently and productively.
Scalability: Automated systems can handle growing amounts of data and tasks, unlike manual methods that require more resources as volume increases.
Cost Reduction: Automation reduces the need for labor-intensive processes, lowering operational costs.
3. Automating Excel Tasks with Python
Excel remains a dominant tool in business operations, from managing financial data to tracking project progress. However, manually handling Excel files can be tedious. Using Python, you can automate most tasks associated with Excel, such as reading, writing, processing data, and even generating reports.
Loading, Reading, and Writing Excel Files with openpyxl
The openpyxl
library allows Python to interact directly with Excel files. You can create, edit, and manage Excel spreadsheets programmatically.
Example: Writing Data to Excel
from openpyxl import Workbook
# Create a new workbook and select the active sheet
wb = Workbook()
ws = wb.active
# Write data to the sheet
ws['A1'] = "Automated"
ws['A2'] = "Data Entry"
ws['B1'] = 100
ws['B2'] = 200
# Save the workbook
wb.save("example.xlsx")
Example: Reading Data from Excel
from openpyxl import load_workbook
# Load an existing workbook
wb = load_workbook("example.xlsx")
ws = wb.active
# Read data from the sheet
print(ws['A1'].value) # Outputs: Automated
print(ws['B2'].value) # Outputs: 200
Automating Data Analysis with Pandas
For more advanced Excel automation, such as data analysis and manipulation, pandas
is an essential library. You can easily load data into DataFrames, manipulate it, and save it back to Excel.
Example: Automating Data Analysis
import pandas as pd
# Load an Excel file into a DataFrame
df = pd.read_excel("sales_data.xlsx")
# Perform calculations, such as adding a "Total Sales" column
df['Total Sales'] = df['Quantity'] * df['Price']
# Save the updated DataFrame back to Excel
df.to_excel("updated_sales_data.xlsx", index=False)
Automating Report Generation
You can also use Python to generate reports automatically from Excel data. This is particularly useful for monthly sales reports, financial statements, or any regular reporting requirements.
4. Automating PDF Management
Working with PDFs is another common office task, whether it’s extracting text, merging multiple documents, or creating reports. Python provides powerful libraries like PyPDF2
and pdfplumber
to automate these tasks.
Reading and Extracting Text from PDFs with PyPDF2
Example: Extracting Text from a PDF
import PyPDF2
# Open a PDF file
with open('document.pdf', 'rb') as pdf_file:
reader = PyPDF2.PdfReader(pdf_file)
page = reader.pages[0]
text = page.extract_text()
print(text)
Merging and Splitting PDFs
With Python, you can also automate the task of merging multiple PDFs into one or splitting a large document into several smaller ones.
Example: Merging PDF Files
from PyPDF2 import PdfMerger
# Initialize a merger object
merger = PdfMerger()
# Append multiple PDF files
merger.append('file1.pdf')
merger.append('file2.pdf')
# Save the merged file
merger.write("merged_output.pdf")
merger.close()
Automating PDF Report Creation with reportlab
You can create dynamic PDF reports using the reportlab
library, which is especially useful for generating invoices, performance reports, or certificates.
Example: Creating a Simple PDF Report
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
# Create a PDF document
c = canvas.Canvas("report.pdf", pagesize=letter)
c.drawString(100, 750, "Monthly Sales Report")
c.drawString(100, 730, "Total Sales: $5000")
c.showPage()
c.save()
5. Automating Emails with Python
Email is a crucial part of everyday office communication, and automating email workflows can be a significant time saver. Python can help with tasks like sending bulk emails, generating email reports, and reading email inboxes.
Sending Emails Automatically with smtplib
You can send automated emails using the smtplib
library. This is particularly useful for sending reports, updates, or notifications.
Example: Sending an Email
import smtplib
from email.mime.text import MIMEText
# Define email parameters
sender = 'your_email@example.com'
recipient = 'recipient@example.com'
subject = 'Automated Report'
body = 'This is an automated email sent from Python.'
# Create the email message
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = sender
msg['To'] = recipient
# Send the email
with smtplib.SMTP('smtp.gmail.com', 587) as server:
server.starttls()
server.login(sender, 'your_password')
server.sendmail(sender, recipient, msg.as_string())
Reading Emails with imaplib
You can use imaplib
to read and process incoming emails automatically, such as retrieving attachments or responding to specific queries.
Example: Reading Emails
import imaplib
import email
# Log in to the email account
mail = imaplib.IMAP4_SSL('imap.gmail.com')
mail.login('your_email@example.com', 'your_password')
# Select the inbox
mail.select('inbox')
# Search for all emails
status, messages = mail.search(None, 'ALL')
# Fetch the latest email
email_ids = messages[0].split()
status, msg_data = mail.fetch(email_ids[-1], '(RFC822)')
msg = email.message_from_bytes(msg_data[0][1])
# Print the subject of the email
print(f"Subject: {msg['subject']}")
6. Integrating Workflows: Combining Excel, PDF, and Email Automation
Automation becomes even more powerful when you combine various tasks. For instance, you can automatically read data from an Excel file, generate a report in PDF format, and send it via email — all within the same Python script.
Example: Workflow Automation
import pandas as pd
from reportlab.pdfgen import canvas
import smtplib
from email.mime.text import MIMEText
# Step 1: Read data from Excel
df = pd.read_excel('sales_data.xlsx')
total_sales = df['Total Sales'].sum()
# Step 2: Generate a PDF report
c = canvas.Canvas("sales_report.pdf")
c.drawString(100, 750, f"Total Sales: ${total_sales}")
c.save()
# Step 3: Send the report via email
msg = MIMEText('Please find attached the sales report.')
msg['Subject'] = 'Sales Report'
msg['From'] = 'your_email@example.com'
msg['To'] = 'recipient@example.com'
# Send the email with the report attached
with smtplib.SMTP('smtp.gmail.com', 587) as server:
server.starttls()
server.login('your_email@example.com', 'your_password')
server.sendmail('your_email@example.com', 'recipient@example.com', msg.as_string())
Conclusion
openpyxl
, pandas
, PyPDF2
, and smtplib
, workflows can be integrated and executed seamlessly. By applying Python to everyday tasks, organizations can save time and enhance productivity significantly.Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
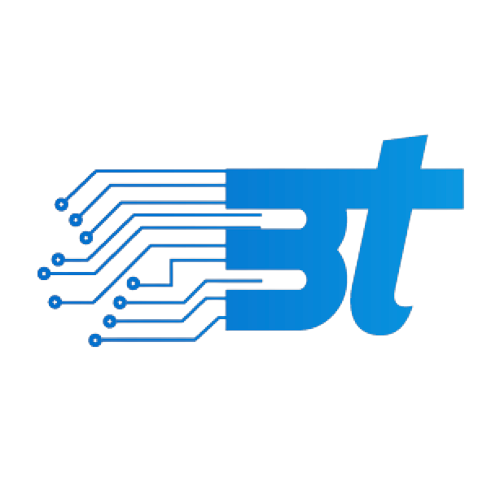
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.