MVVM Architecture in Android: A Comprehensive Guide

Table of contents
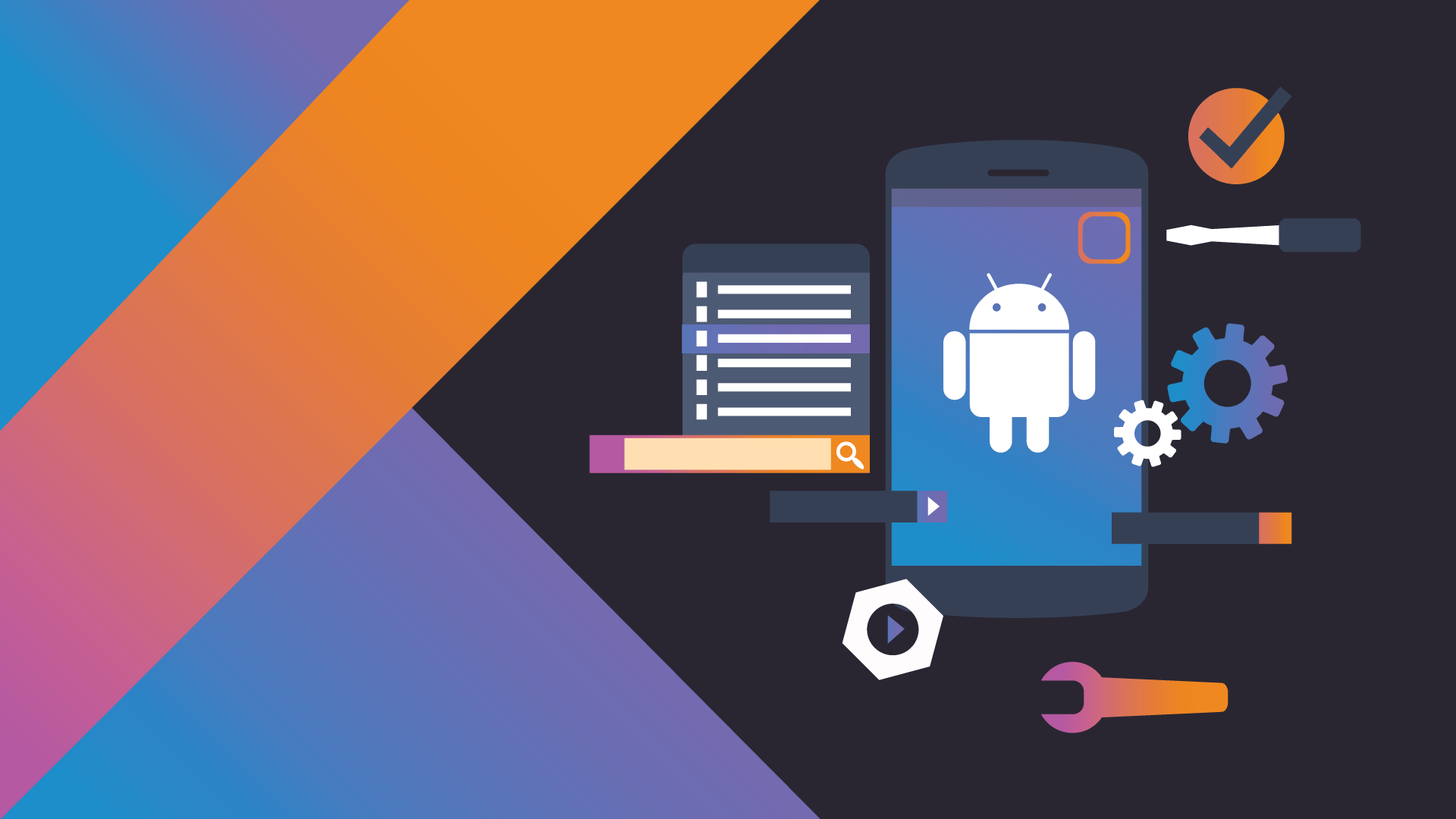
Welcome to our in-depth exploration of the Model-View-ViewModel (MVVM) architecture in Android development. In this blog post, we'll dive into the core concepts of MVVM, discuss its benefits, and provide practical examples to help you implement this powerful architectural pattern in your Android projects.
1. Understanding MVVM
MVVM is a design pattern that divides the codebase into three key components:
Model: Handles data and business logic.
View: Manages the UI of the application.
ViewModel: Acts as a communication bridge between Model and View, preparing data for the UI and handling UI-related logic.
Let’s break down each component.
Model
Role: The Model is responsible for fetching and managing data, either from a database, a web service, or other sources. Example: Suppose you're building an app to display a list of movies. The Model retrieves the movie data.
data class Movie(val title: String, val director: String)
View
Role: The View is the UI that the user interacts with. It displays the data provided by the ViewModel. Example: In the movie app, the View is an XML layout or Jetpack Compose UI that presents the list of movies to the user.
<!-- activity_movie.xml -->
<TextView
android:id="@+id/movieTitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
ViewModel
Role: The ViewModel acts as an intermediary between the Model and the View. It prepares data for display and handles logic that affects the UI. Example: In the movie app, the ViewModel formats the movie data for display.
kotlinCopy codeclass MovieViewModel : ViewModel() {
fun getMovies(): List<Movie> {
return listOf(Movie("Inception", "Christopher Nolan"), Movie("Interstellar", "Christopher Nolan"))
}
}
View Example
kotlinCopy codeclass MovieActivity : AppCompatActivity() {
private lateinit var viewModel: MovieViewModel
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_movie)
viewModel = MovieViewModel()
val movies = viewModel.getMovies()
// Populate the UI with movie data
}
}
This simple architecture allows for a clean separation of concerns, making it easier to scale and maintain.
2. Understanding ViewModel Class
A ViewModel in Android is responsible for managing and holding UI-related data, while surviving configuration changes such as screen rotations.
Lifecycle Awareness
The ViewModel is lifecycle-aware, meaning it survives configuration changes, which prevents data loss when the screen is rotated or the configuration changes.
kotlinCopy codeimport androidx.lifecycle.ViewModel
class CounterViewModel : ViewModel() {
var count = 0
fun incrementCount() {
count++
}
}
3. Inheritance in Kotlin
Inheritance allows a class to inherit properties and methods from another class. It promotes code reuse and modularity.
Example of Inheritance
kotlinCopy codeopen class Vehicle {
fun start() {
println("Vehicle is starting.")
}
}
class Car : Vehicle() {
fun drive() {
println("Car is driving.")
}
}
In this example, the Car
class inherits from Vehicle
and can now use the start()
method in addition to its own drive()
method.
4. Open and Override Functions in Kotlin
By default, classes and functions in Kotlin are final and cannot be inherited or overridden. To enable inheritance, you must mark them with the open
keyword.
Example of Open and Override
kotlinCopy codeopen class Vehicle {
open fun start() {
println("Vehicle is starting.")
}
}
class SportsCar : Vehicle() {
override fun start() {
println("SportsCar is roaring to start!")
}
}
In this example, SportsCar
overrides the start()
method of Vehicle
to provide a specific implementation.
5. Interfaces in Kotlin
An interface defines a contract that classes must follow, but it doesn't provide implementation.
Example of Interface
kotlinCopy codeinterface Drivable {
fun drive()
}
class Bicycle : Drivable {
override fun drive() {
println("Bicycle is driving.")
}
}
Classes like Bicycle
that implement the Drivable
interface must provide an implementation for the drive()
function.
6. What are Repositories in Android?
In MVVM, a Repository is responsible for abstracting data sources, whether from a local database, cache, or a remote server. It serves as a clean API for data management, allowing the ViewModel to focus solely on preparing data for the View.
Example of Repository
kotlinCopy codeclass UserRepository(private val userDao: UserDao, private val apiService: ApiService) {
fun getUser(userId: String): LiveData<User> {
// Logic to fetch data from local database or network
}
}
The UserRepository abstracts the data retrieval process, whether from a database or a web service.
Conclusion
The MVVM architecture helps in keeping your Android app modular, maintainable, and scalable. Combined with concepts like inheritance, interfaces, and repositories, it lays a solid foundation for building robust applications that are easy to extend and maintain.
If you're new to MVVM, start by separating your app's UI logic, data handling, and business logic into distinct layers, and gradually integrate more advanced features like LiveData and repositories to optimize your app’s architecture. Happy coding!
Subscribe to my newsletter
Read articles from Aditya Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aditya Das
Aditya Das
B.Tech student in Information Technology with a passion for Android app development. Specializing in Kotlin, Java, and modern development practices. Exploring the latest trends in mobile and web technologies, and committed to sharing knowledge and insights through blogging. Follow along for tutorials, tips, and industry updates!