How to implement Canary for Kubernetes apps using Istio

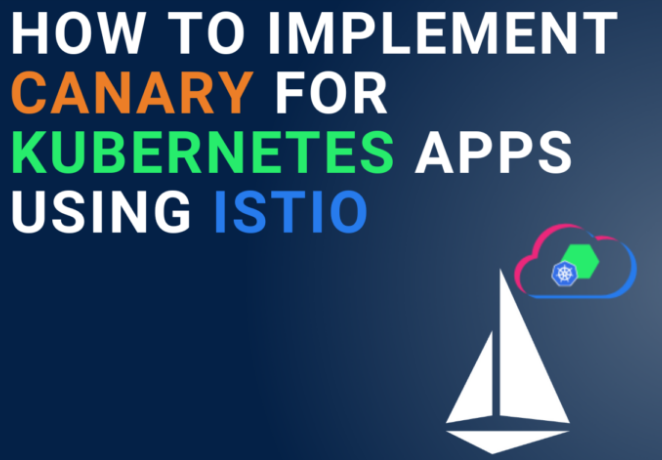
Let's break down the explanation of using Istio in Canary deployments into the four tasks as outlined below.
Task 1: Explain Service Mesh (Istio) Fundamentals
What is a Service Mesh?
A service mesh is a configurable infrastructure layer for microservices applications that makes it easy to manage service discovery, traffic management, and security without requiring changes to the application code. It provides a uniform way to manage the communication between microservices, allowing for features like traffic management, security, and observability.
Components of Istio
Envoy: Envoy is the data plane of Istio. It is a high-performance proxy that mediates all inbound and outbound traffic for all services in the mesh. Envoy is responsible for enforcing the rules and policies defined by Istio.
Pilot: Pilot is the control plane of Istio. It is responsible for managing the lifecycle of Envoy proxies, including configuration, deployment, and scaling. Pilot also provides service discovery and traffic management capabilities.
Citadel: Citadel is the identity and credential management component of Istio. It issues and manages certificates for the proxies and services in the mesh, ensuring secure communication between them.
Primary Features of Istio
Traffic Management: Istio provides features like traffic routing, load balancing, and circuit breaking, which allow for fine-grained control over how traffic is routed between services.
Security: Istio provides features like mutual TLS authentication, request and response encryption, and identity-based authentication, ensuring secure communication between services.
Observability: Istio provides features like tracing, logging, and monitoring, which allow for detailed insights into the behavior and performance of services in the mesh.
Task 2: Describe Canary Deployments
What are Canary Deployments?
Canary deployments are a deployment strategy where a new version of a service is deployed alongside the existing version, and a small percentage of traffic is routed to the new version. This allows for testing the new version in a production environment with real users, but with minimal risk. If the new version performs well, more traffic can be gradually routed to it, and if it performs poorly, the traffic can be routed back to the existing version.
Benefits of Canary Deployments
Reduced Risk: Canary deployments reduce the risk of deploying a new version of a service by limiting the impact of any potential issues.
Faster Feedback: Canary deployments allow for faster feedback on the performance of a new version, as it is tested with real users.
Gradual Rollout: Canary deployments enable a gradual rollout of a new version, allowing for a controlled increase in traffic to the new version.
How Canary Deployments Work
Traffic Routing: Traffic routing is a critical component of canary deployments. It involves directing a percentage of traffic to the new version of the service.
Gradual Rollout: The rollout of the new version is gradual, with traffic being incrementally routed to the new version.
Task 3: Integrate Istio with Canary Deployments
Setting Up Virtual Services
Virtual services in Istio define a set of rules that govern the behavior of requests routed to a service. To set up a virtual service for a canary deployment, you would define two versions of the service (e.g., v1
and v2
) and specify the traffic routing rules.
apiVersion: networking.istio.io/v1beta1
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service.default.svc.cluster.local
http:
- name: v1
route:
- destination:
host: my-service-v1
weight: 90
- name: v2
route:
- destination:
host: my-service-v2
weight: 10
In this example, 90% of the traffic is routed to my-service-v1
and 10% to my-service-v2
.
Setting Up Destination Rules
Destination rules in Istio define policies that apply to traffic intended for a service after routing has occurred. To set up a destination rule for a canary deployment, you would define the subsets of the service (e.g., v1
and v2
) and specify the traffic policies.
apiVersion: networking.istio.io/v1beta1
kind: DestinationRule
metadata:
name: my-service
spec:
host: my-service.default.svc.cluster.local
trafficPolicy:
tls:
mode: ISTIO_MUTUAL
subsets:
- name: v1
labels:
version: v1
- name: v2
labels:
version: v2
Setting Up Traffic Routing Rules
Traffic routing rules in Istio define how traffic is routed between services. To set up traffic routing rules for a canary deployment, you would define the routing rules based on the subsets defined in the destination rule.
apiVersion: networking.istio.io/v1beta1
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service.default.svc.cluster.local
http:
- name: v1
route:
- destination:
host: my-service-v1
subset: v1
weight: 90
- name: v2
route:
- destination:
host: my-service-v2
subset: v2
weight: 10
Task 4: Step-by-Step Example and Walkthrough
Step 1: Create a Simple Microservice
Let's create a simple microservice using Python and Flask. Save the following code in a file named app.py
.
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=5000)
Step 2: Deploy the Microservice with Istio
First, ensure you have Istio installed on your Kubernetes cluster. Then, deploy the microservice using the following YAML file.
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-service-v1
spec:
replicas: 1
selector:
matchLabels:
app: my-service
version: v1
template:
metadata:
labels:
app: my-service
version: v1
spec:
containers:
- name: my-service
image: my-service:v1
ports:
- containerPort: 5000
Step 3: Set Up Istio for Canary Deployment
Create the virtual service and destination rule as described in Task 3.
Step 4: Perform a Canary Rollout
To perform a canary rollout, you would update the virtual service to route more traffic to the new version. For example, you could update the virtual service to route 50% of the traffic to the new version.
apiVersion: networking.istio.io/v1beta1
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service.default.svc.cluster.local
http:
- name: v1
route:
- destination:
host: my-service-v1
weight: 50
- name: v2
route:
- destination:
host: my-service-v2
weight: 50
Step 5: Verify the Canary Deployment
Use tools like istioctl
to verify the canary deployment. For example, you can use istioctl analyze
to analyze the configuration and ensure it is correct.
istioctl analyze
This command will analyze the Istio configuration and report any errors or warnings.
Conclusion
Istio provides a powerful way to implement canary deployments in microservices environments. By defining virtual services, destination rules, and traffic routing rules, you can control the flow of traffic between different versions of a service, allowing for safe and controlled rollouts of new versions. The step-by-step example provided demonstrates how to set up a simple canary deployment using Istio, from creating a microservice to deploying it with Istio and performing a canary rollout.
Subscribe to my newsletter
Read articles from Mohammed Iliyas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Iliyas
Mohammed Iliyas
As a seasoned DevOps engineer, I bring a comprehensive set of skills to the table, encompassing version control with Git and GitOps using ArgoCD, containerization with Docker, and orchestration with Kubernetes, Helm, and Istio. I'm proficient in infrastructure provisioning and management using Terraform and Ansible, and have expertise in setting up and managing CI/CD pipelines with Jenkins, Azure Pipelines, and AWS CodePipeline. With extensive experience in cloud platforms, including Azure and AWS, I've deployed and managed applications on Azure Kubernetes Service (AKS) and AWS Elastic Container Service (ECS) and Elastic Container Service for Kubernetes (EKS). Additionally, I've integrated security practices into DevOps pipelines, ensuring secure and compliant software development and deployment. My technical prowess extends to Bash shell scripting, Linux system administration, and programming in Golang. Throughout my journey, I've developed a unique blend of skills that enable me to streamline development, deployment, and management of applications across multiple environments.