#7.0 What XPath: Best Practices | Optimization
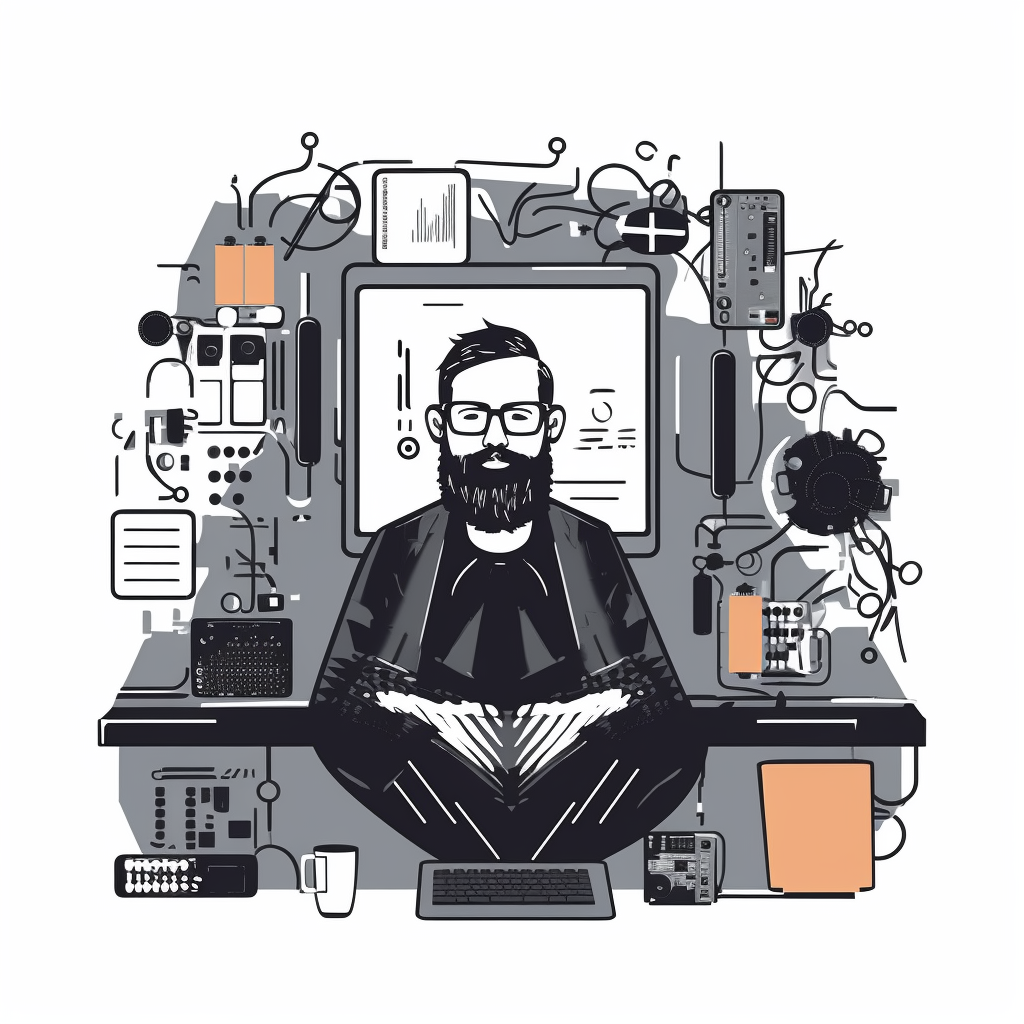
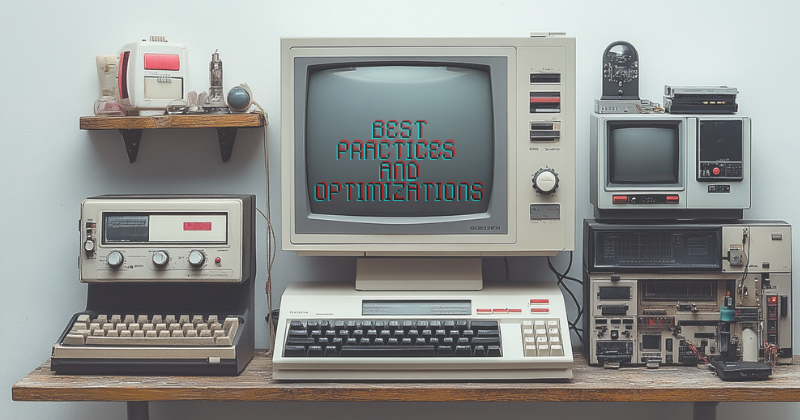
Efficient XPath queries are crucial for maintaining fast, reliable, and maintainable web automation scripts. This section covers best practices for writing XPath expressions and optimizing them for better performance.
7.1 Writing Efficient XPath Queries
7.1.1 Use Specific Attributes
When possible, use specific and unique attributes to locate elements. IDs are often the best choice as they are typically unique.
Good: //input[@id='username']
Avoid: //input[@type='text']
Explanation:
Good: Using the ID attribute provides a unique and stable reference to the element. IDs are designed to be unique within a page, making this selector highly specific and less prone to errors if the page structure changes.
Avoid: Selecting by input type is too broad. There may be multiple text inputs on a page, leading to potential ambiguity and unreliable element selection. This approach might select the wrong element if the page layout changes.
7.1.2 Avoid Using Indexes
Indexes can be fragile if the page structure changes. Use them sparingly and only when necessary.
Good: //table[@id='data']//tr[td[contains(text(), 'Specific Text')]]
Avoid: //table[@id='data']//tr[5]
Explanation:
Good: This approach selects a row based on its content, which is more robust to changes in the table structure. If rows are added or removed, this selector will still find the correct row as long as the specific text exists.
Avoid: Using a fixed index (5 in this case) is fragile. If the table structure changes (e.g., rows are added or removed), this selector will likely select the wrong row or fail to find any element.
7.1.3 Minimize the Use of Wildcards
While '//' and '*' are powerful, they can significantly slow down XPath evaluation, especially on large DOMs.
Good: //div[@id='content']//h2
Avoid: //*[@id='content']//*[name()='h2']
Explanation:
Good: This XPath is more specific and efficient. It directly looks for 'h2' elements within a div with a specific ID, reducing the search space and improving performance.
Avoid: Using '//*' forces the XPath engine to check every single element in the DOM, which is computationally expensive. The 'name()' function adds another layer of computation for each element, further slowing down the evaluation.
7.1.4 Use XPath Axes Judiciously
Axes like following-sibling
, preceding-sibling
, ancestor
, etc., can be powerful but may impact performance if overused.
Good: //label[@for='email']/following-sibling::input[1]
Avoid: //input[preceding::label[contains(text(), 'Email')]]
Explanation:
Good: This XPath efficiently finds the input element that immediately follows the label. It's precise and doesn't require scanning a large portion of the DOM.
Avoid: This approach scans all preceding elements for each input, which can be very inefficient, especially in large DOMs. It may also be less accurate if there are multiple labels containing 'Email'.
7.1.5 Leverage XPath Functions
Use XPath functions to create more precise and efficient queries.
Good: //button[normalize-space(text())='Submit']
Avoid: //button[contains(text(), ' Submit ')]
Explanation:
Good: The 'normalize-space()' function trims whitespace from the beginning and end of the text and replaces multiple spaces with a single space. This makes the selector more robust against inconsistent spacing in the button text.
Avoid: Using 'contains()' with spaces included can lead to false positives if there are variations in spacing. It's also less precise, potentially matching buttons that contain 'Submit' as part of a longer text.
7.2 Optimizing XPath Performance
7.2.1 Start with Specific Context
Begin your XPath with a specific, easily identifiable element to narrow the search scope.
Good: //div[@id='user-info']//span[@class='name']
Avoid: //span[@class='name']
Explanation:
Good: This XPath starts with a specific div, limiting the search area. It's faster because it only looks for spans within this specific context, reducing the number of elements to evaluate.
Avoid: Searching for spans with a certain class across the entire document can be slow, especially on large pages. It may also return unwanted elements if the class is used elsewhere in the document.
7.2.2 Use Logical Operators Efficiently
When using 'and' or 'or', put the condition most likely to be false first in an 'and' operation, and the condition most likely to be true first in an 'or' operation.
Good: //input[@type='checkbox' and @checked]
Avoid: //input[@checked and @type='checkbox']
Explanation:
Good: This order is more efficient because it first filters for checkbox inputs (likely fewer elements) before checking if they're checked. If there are many checked inputs of different types, this approach evaluates fewer elements.
Avoid: This checks the 'checked' attribute first, potentially on many non-checkbox elements, before filtering for checkboxes. This can be less efficient, especially if there are many checked elements that aren't checkboxes.
7.2.3 Avoid Complex Calculations in Predicates
Minimize the use of functions that require complex calculations within predicates.
Good: //div[@class='price'][number(text()) > 100]
Avoid: //div[@class='price'][number(translate(text(), '$', '')) > 100]
Explanation:
Good: This XPath performs a simple numeric comparison. It assumes the text is already in a numeric format, which is often the case in well-structured HTML.
Avoid: The 'translate' function adds an extra layer of computation for each price element. While it's more flexible (handling prices with currency symbols), it's slower, especially when dealing with many elements.
7.2.4 Use Text Matching Carefully
Exact text matching can be fragile. Consider using contains() or starts-with() for more robust selectors.
Good: //button[contains(text(), 'Submit')]
Avoid: //button[text()='Submit']
Explanation:
Good: Using 'contains()' allows for partial text matching, making the selector more robust against small text changes or additional text in the button.
Avoid: Exact text matching with 'text()=' is fragile. It will fail if there's any deviation in the button text, such as added spaces, changed capitalization, or additional text.
7.2.5 Combine XPath with CSS Selectors
Some tools, like Selenium, allow you to combine CSS selectors with XPath for optimal performance.
# Selenium Python example
element = driver.find_element(By.CSS_SELECTOR, "#content")
sub_element = element.find_element(By.XPATH, ".//h2[contains(@class, 'title')]")
Explanation:
- This approach leverages the speed of CSS selectors for the initial broad selection (finding the #content element) and then uses XPath for more complex, specific selection within that context. It combines the strengths of both selector types for optimal performance.
7.3 Maintaining XPath-Based Test Scripts
7.3.1 Use Variables for Repeated XPaths
Store frequently used XPath expressions in variables or constants for easy maintenance.
USERNAME_INPUT = "//input[@id='username']"
PASSWORD_INPUT = "//input[@id='password']"
LOGIN_BUTTON = "//button[@type='submit']"
7.3.2 Create Custom XPath Functions
For complex or frequently used XPath patterns, create custom functions to encapsulate the logic.
def find_row_by_content(table_id, column_text):
return f"//table[@id='{table_id}']//tr[td[contains(text(), '{column_text}')]]"
7.3.3 Document Complex XPaths
For particularly complex XPath expressions, add comments explaining the logic and any assumptions made.
# Finds the price of a product in a dynamic list
# Assumes the price is in the second column of the row containing the product name
product_price_xpath = f"""
//table[@id='product-list']
//tr[td[1][contains(text(), '{product_name}')]]
/td[2]
"""
7.3.4 Regularly Review and Refactor
As web applications evolve, regularly review your XPath selectors to ensure they're still efficient and accurate. Refactor as needed to maintain performance and reliability.
7.3.5 Use Relative XPaths
When possible, use relative XPaths to make your selectors more robust to changes in the overall page structure.
Good: .//div[@class='content']//p
Avoid: /html/body/div[2]/div[1]/p
7.4 Common Pitfalls to Avoid
Overreliance on Position: Avoid using position-based selectors unless absolutely necessary, as they're prone to breaking when the page structure changes.
Ignoring Dynamic Content: Be aware of dynamically loaded content and use appropriate waits in your Selenium scripts.
Neglecting Performance Testing: Regularly test the performance of your XPath selectors, especially on large or complex pages.
Hardcoding Text Values: Be cautious about hardcoding exact text values in your XPaths, as they can break with minor content changes or in multi-language sites.
Ignoring XPath Versions: Be aware of which XPath version your tool supports and stick to compatible functions and syntax.
By following these best practices and optimization techniques, you can create more efficient, reliable, and maintainable XPath-based selectors for your Selenium automation projects. Remember, the goal is to balance specificity, performance, and maintainability in your XPath expressions.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
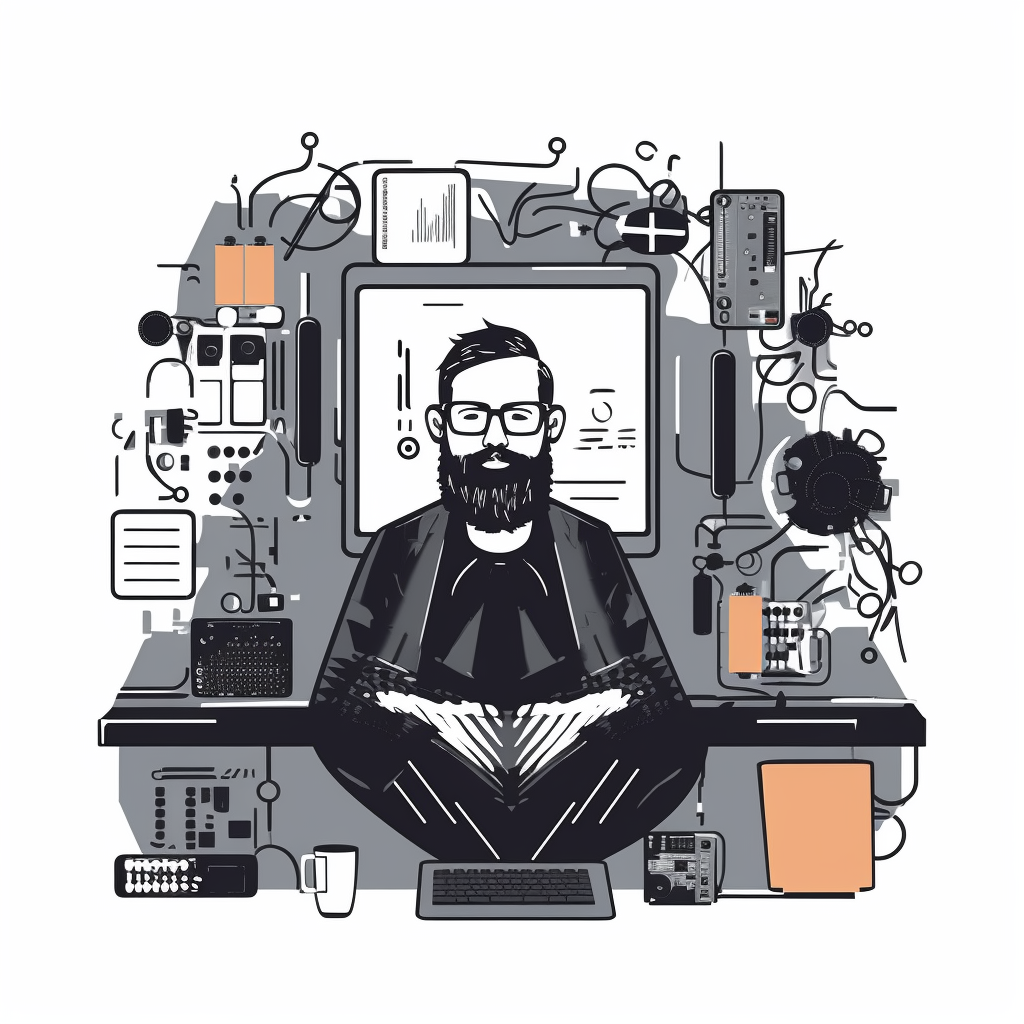
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.