Unlocking the Power of K-Nearest Neighbors: A Deep Dive into NumPy Implementation
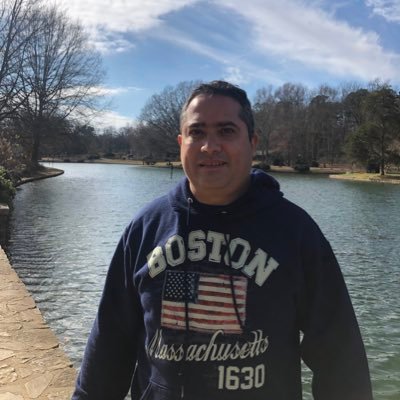
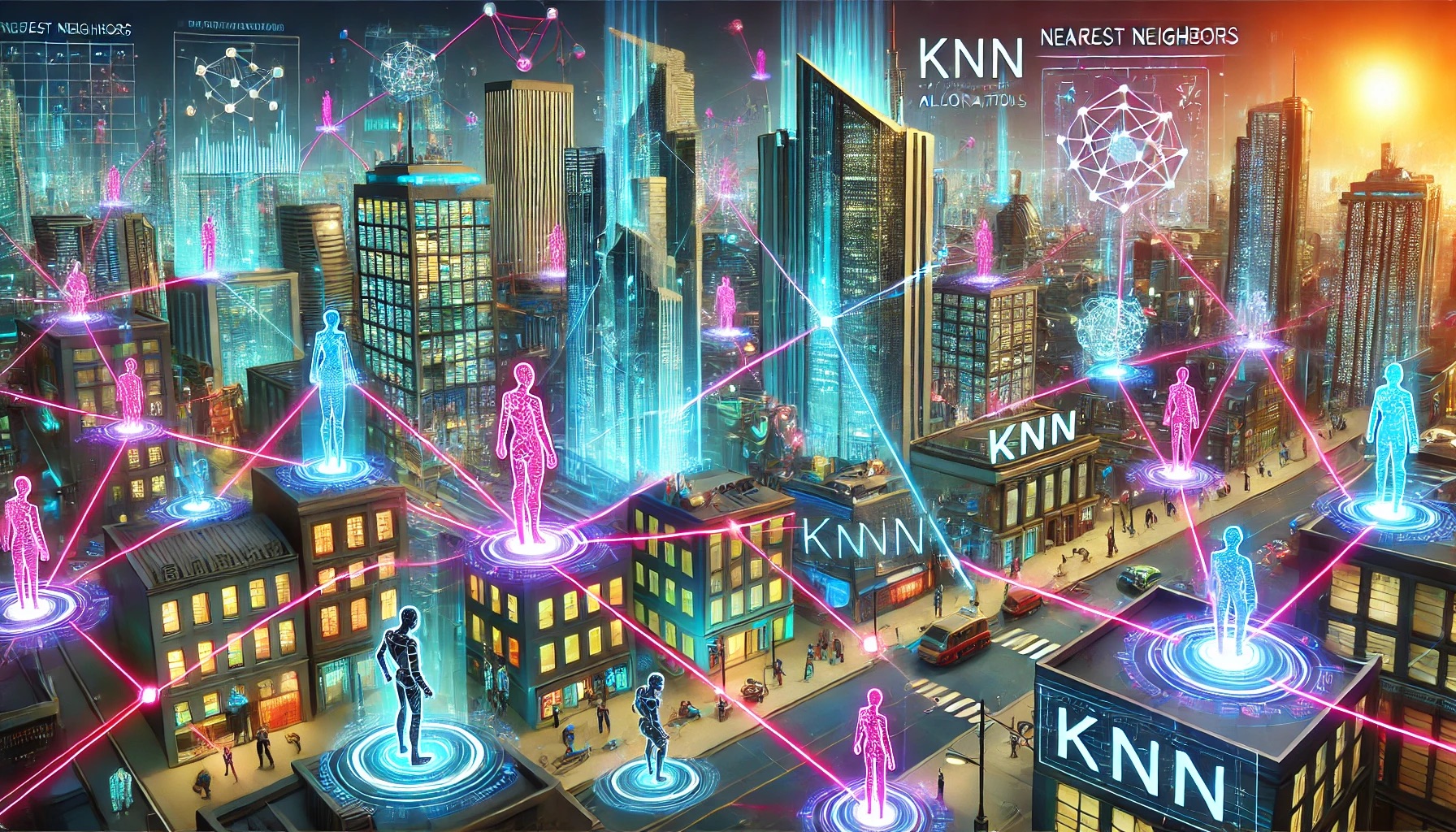
Welcome to the fascinating realm of K-Nearest Neighbors (KNN), a cornerstone algorithm in machine learning that's both elegantly simple and surprisingly powerful.
Its simplicity belies its powerful capabilities in both classification and regression tasks.
In this comprehensive guide, we'll unravel the mysteries of KNN and show you how to harness its potential using the numerical powerhouse of Python: NumPy.
Understanding K-Nearest Neighbors (KNN)
KNN is an instance-based learning algorithm, meaning it makes predictions based on the instances of the training data.
Unlike model-based algorithms, KNN doesn't assume any underlying distribution of the data.
Instead, it relies on the distance between data points to determine their similarity.
In classification tasks, KNN assigns the class most common among the k nearest neighbors of a data point.
For regression, it predicts the value based on the average of the k nearest neighbors.
Diving into the KNN Algorithm: A Step-by-Step Breakdown
Let's break down the KNN algorithm into its core components.
This step-by-step approach will give you a clear understanding of how KNN operates under the hood.
Step 1: Choose Your Neighbors
The first decision in implementing KNN is determining the value of K.
This number represents how many nearest neighbors we'll consider when making a prediction.
Choosing K is a balancing act:
Too small, and your model becomes sensitive to noise.
Too large, and you risk oversimplifying your decision boundary.
Step 2: Calculate Distances
For each prediction, KNN calculates the distance between the new data point and every single point in your training set.
This is where the "nearest" in K-Nearest Neighbors comes into play.
Common distance metrics include:
Euclidean distance (straight-line distance)
Manhattan distance (city block distance)
Minkowski distance (a generalization of Euclidean and Manhattan)
Step 3: Find the K Nearest Neighbors
Once we have all the distances, we identify the K training points closest to our new data point.
These are our K nearest neighbors.
Step 4: Make a Decision
For classification tasks, KNN uses a majority vote among the K neighbors.
The class that appears most frequently among the neighbors is assigned to the new data point.
For regression tasks, KNN typically uses the average of the K neighbors' target values.
Step 5: Evaluate and Iterate
Like any machine learning algorithm, KNN's performance should be evaluated on a separate test set.
Based on the results, you might adjust the value of K or experiment with different distance metrics to improve performance.
Implementing KNN with NumPy: A Practical Approach
Now that we understand the theory, let's roll up our sleeves and implement KNN using NumPy.
NumPy's efficient array operations make it an ideal choice for implementing KNN from scratch.
Setting Up Our Environment
First, let's import NumPy and set up our KNN class:
import numpy as np
class KNNClassifier:
def __init__(self, k=3):
self.k = k
self.X_train = None
self.y_train = None
This initialization sets up our KNN classifier with a default of 3 neighbors.
We also create placeholder attributes for our training data.
Training the Model: Memorization is Key
KNN is often called a lazy learner because it doesn't do much during the training phase.
Instead, it simply memorizes the training data:
def fit(self, X, y):
self.X_train = X
self.y_train = y
The Heart of KNN: Making Predictions
The prediction phase is where the real magic happens.
Let's break down the predict
method:
def predict(self, X):
predictions = []
# iterate through each test point `x`.
for x in X:
# calculate the distance between `x` and all training points
# using the Euclidean distance.
distances = np.sqrt(np.sum((self.X_train - x)**2, axis=1))
# get the indices of the K nearest neighbors.
k_indices = np.argsort(distances)[:self.k]
# get the labels of these neighbors.
k_nearest_labels = self.y_train[k_indices]
# find the most common label.
most_common = np.bincount(k_nearest_labels).argmax()
# append this label to our predictions.
predictions.append(most_common)
return np.array(predictions)
This is the whole code.
import numpy as np
class KNNClassifier:
def __init__(self, k=3):
self.k = k
self.X_train = None
self.y_train = None
def fit(self, X, y):
self.X_train = X
self.y_train = y
def predict(self, X):
predictions = []
for x in X:
# Calculate the distance between the test point
# and all training points using the Euclidean distance
distances = np.sqrt(np.sum((self.X_train - x)**2, axis=1))
# Get the indices of the k nearest neighbors
k_indices = np.argsort(distances)[:self.k]
# Get the labels of the k nearest neighbors
k_nearest_labels = self.y_train[k_indices]
# For classify task, get the most common label
most_common = np.bincount(k_nearest_labels).argmax()
# For regression task, get the average of the k nearest neighbors
# Instead of taking a majority vote, we simply average the values
# of the K nearest neighbors.
# k_nearest_values = self.y_train[k_indices]
# prediction = np.mean(k_nearest_values)
# Append the most common label to the predictions
predictions.append(most_common)
# Return the predictions as a numpy array
return np.array(predictions)
The Power of NumPy: Vectorized Operations
While the above implementation is straightforward, it can be optimized further using NumPy's vectorization capabilities.
Vectorization reduces the reliance on Python loops, leading to significant performance gains, especially with large datasets.
import numpy as np
class KNNClassifierOptimized:
def __init__(self, k=3):
self.k = k
self.X_train = None
self.y_train = None
def fit(self, X, y):
self.X_train = X
self.y_train = y
def predict(self, X):
# Calculate the Euclidean distances between each test point
# and all training points
distances = np.sqrt(((self.X_train - X[:, np.newaxis])**2)\
.sum(axis=2))
# Get the indices of the k nearest neighbors
k_indices = np.argsort(distances, axis=1)[:, :self.k]
# Get the labels of the k nearest neighbors
k_nearest_labels = self.y_train[k_indices]
# Determine the most common class for each test point
predictions = np.array([
np.bincount(labels).argmax()
for labels in k_nearest_labels
])
return predictions
Feature Scaling and Normalization
KNN's reliance on distance calculations makes it sensitive to feature scales.
Features with larger scales can disproportionately influence the distance metrics, skewing predictions.
Scaling Techniques
Min-Max Scaling:
- Transforms features to a fixed range, typically [0, 1].
Standardization (Z-score Normalization):
- Centers features around the mean with a unit standard deviation.
Robust Scaling:
- Utilizes median and interquartile range, making it resilient to outliers.
Applying appropriate scaling ensures that all features contribute equally to the distance calculations, enhancing KNN's performance.
Optimizing for Large Datasets
KNN's prediction phase involves calculating distances to all training points, which can be computationally intensive for large datasets.
Strategies for Optimization
KD-Trees and Ball Trees:
- Data structures that partition the space to allow efficient nearest neighbor searches.
Approximate Nearest Neighbors:
- Employs algorithms that trade off some accuracy for significant speed gains.
Parallel Processing:
- Utilizes multi-threading or GPU acceleration to distribute distance computations.
Incorporating these strategies can substantially reduce prediction times, making KNN feasible for large-scale applications.
Real-World Applications: Where KNN Shines
KNN's simplicity belies its power in various real-world scenarios.
Let's explore some domains where KNN proves particularly effective.
Recommendation Systems: Finding Similar Users
KNN can power recommendation engines by identifying users or items with similar preferences.
For instance, in collaborative filtering, KNN can suggest products by finding users with comparable purchase histories.
Its instance-based nature ensures personalized and dynamic recommendations.
By finding users with similar preferences, we can recommend products or content:
def recommend(self, user_preferences):
distances = self.euclidean_distance(self.user_data, user_preferences)
k_indices = np.argsort(distances)[:self.k]
similar_users = self.user_data[k_indices]
recommendations = np.mean(similar_users, axis=0)
return recommendations
Image Recognition: Classifying Based on Pixel Similarity
In image classification tasks, KNN can categorize images based on feature similarities.
Features can include pixel values, color histograms, or more abstract representations from deep learning models.
Despite its simplicity, KNN can achieve competitive performance, especially when combined with dimensionality reduction techniques.
In computer vision, KNN can be used for simple image classification tasks:
def classify_image(self, image):
flattened_image = image.flatten()
distances = self.euclidean_distance(self.image_database, flattened_image)
k_indices = np.argsort(distances)[:self.k]
k_nearest_labels = self.image_labels[k_indices]
return np.bincount(k_nearest_labels).argmax()
Anomaly Detection: Identifying Outliers
KNN can identify outliers by measuring the distance of data points from their nearest neighbors.
Points with distances exceeding a threshold are flagged as anomalies.
This capability is valuable in fraud detection, network security, and quality control.
KNN can be adapted for anomaly detection by looking at the distance to the K-th nearest neighbor:
def detect_anomalies(self, X, threshold):
anomalies = []
for x in X:
distances = self.euclidean_distance(self.X_train, x)
k_distance = np.sort(distances)[self.k]
if k_distance > threshold:
anomalies.append(x)
return np.array(anomalies)
Conclusion
As we've journeyed through the world of K-Nearest Neighbors, from its fundamental principles to advanced implementations and real-world applications, one thing becomes clear: KNN's simplicity is its strength.
In an era of increasingly complex machine learning models, KNN serves as a reminder that sometimes, the most intuitive approaches can yield powerful results.
Whether you're building a recommendation system, tackling a classification problem, or exploring anomaly detection, KNN offers a versatile and interpretable solution.
Its implementation in NumPy, as we've explored, combines the algorithm's inherent simplicity with the computational efficiency of vectorized operations.
As you continue your machine learning journey, remember that understanding KNN is not just about mastering a single algorithm.
It's about grasping fundamental concepts like distance metrics, the importance of data representation, and the trade-offs between model complexity and interpretability.
These insights will serve you well across the entire spectrum of machine learning techniques.
So the next time you're faced with a new dataset or a challenging problem, consider turning to your nearest neighbors.
They might just have the answers you're looking for.
PS: If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
Subscribe to my newsletter
Read articles from Juan Carlos Olamendy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
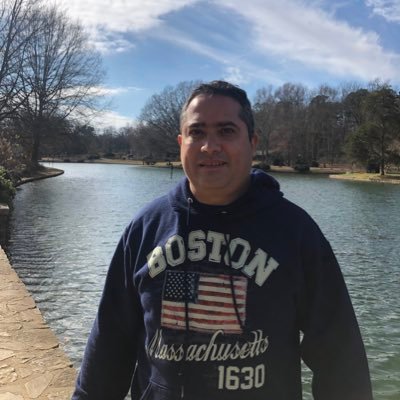
Juan Carlos Olamendy
Juan Carlos Olamendy
🤖 Talk about AI/ML · AI-preneur 🛠️ Build AI tools 🚀 Share my journey 𓀙 🔗 http://pixela.io