How to Open Chrome Extension's Side Panel: A Comprehensive Guide

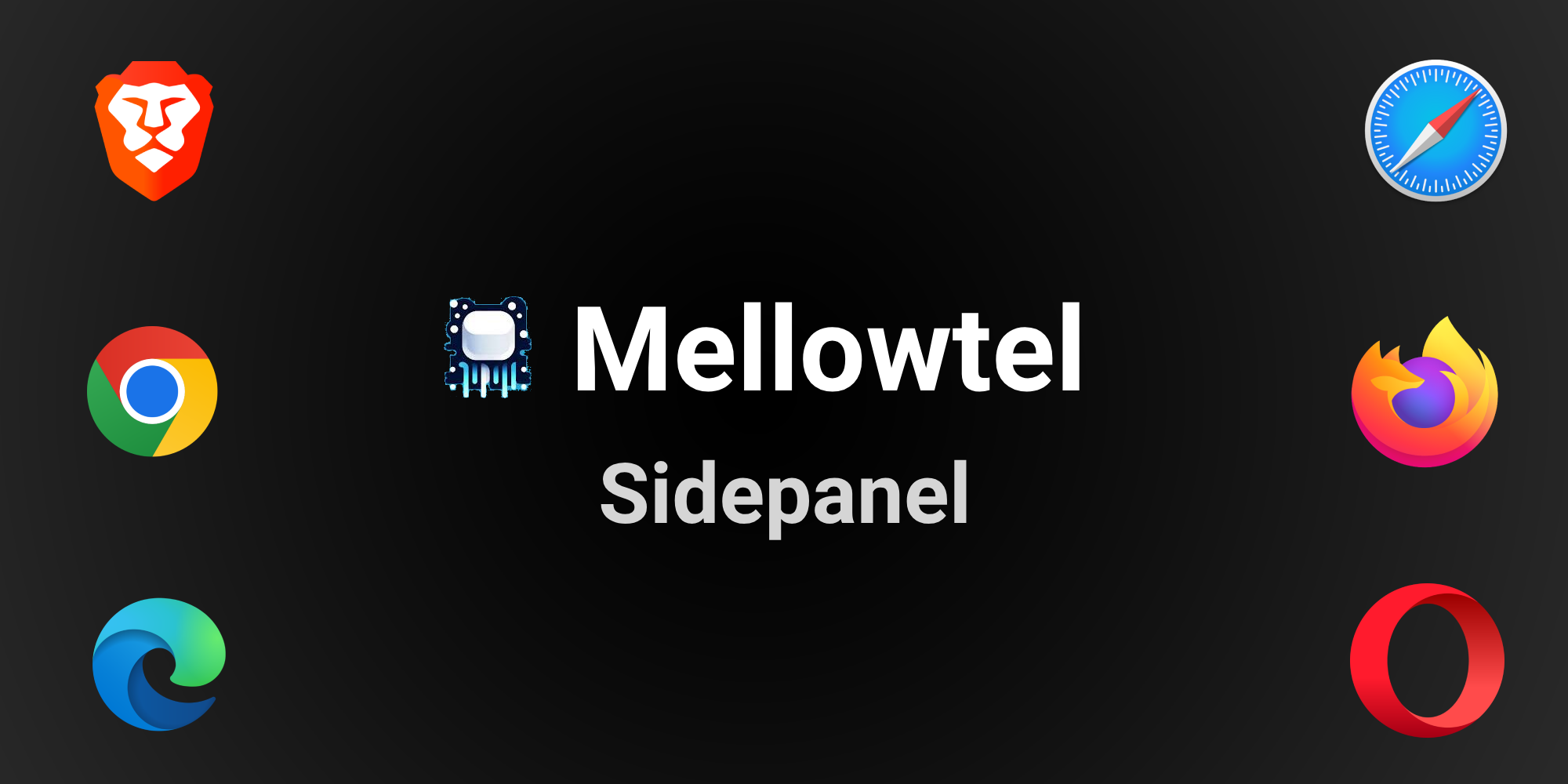
Chrome extensions can now utilize the chrome.sidePanel
API to create additional user interface elements. This guide explains how to open a Chrome extension's side panel using a button click inside the popup or directly from the extension icon.
Solution 1: Opening the Side Panel on Action Click
To open the side panel when the user clicks the extension icon in the toolbar:
- Update your
manifest.json
:
{
"permissions": ["sidePanel"],
"side_panel": {
"default_path": "sidepanel.html"
}
}
- In your
service-worker.js
:
chrome.sidePanel
.setPanelBehavior({ openPanelOnActionClick: true })
.catch((error) => console.error(error));
Solution 2: Opening the Side Panel from the Popup
To open the side panel when a user clicks a button inside the extension popup:
- Update your
manifest.json
:
{
"background": {
"service_worker": "service-worker.js"
},
"side_panel": {
"default_path": "sidepanel.html"
},
"action": {
"default_popup": "popup.html"
},
"permissions": ["sidePanel"]
}
- In your
popup.js
:
document.getElementById('openSidePanel').addEventListener('click', function() {
chrome.runtime.sendMessage({action: 'open_side_panel'});
});
- In your
service-worker.js
:
let windowId;
chrome.tabs.onActivated.addListener(function (activeInfo) {
windowId = activeInfo.windowId;
});
chrome.runtime.onMessage.addListener((message, sender) => {
if (message.action === 'open_side_panel') {
chrome.sidePanel.open({ windowId: windowId });
}
});
Alternative Solution: Direct API Call
For a simpler approach, call chrome.sidePanel.open
()
directly in your popup script:
const handleOpenSidePanel = () => {
chrome.windows.getCurrent({ populate: true }, (window) => {
chrome.sidePanel.open({ windowId: window.id });
});
}
Best Practices and Tips
Include necessary permissions in your
manifest.json
.Handle errors gracefully.
Use TypeScript for better type checking and autocomplete support.
Test your extension thoroughly in different scenarios.
By implementing one of these solutions, you can successfully open your Chrome extension's side panel using a button click inside the popup or directly from the extension icon.
Subscribe to my newsletter
Read articles from Mellowtel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mellowtel
Mellowtel
Easily monetize your browser extensions. Mellowtel is an open-source, ethical monetization engine that respects user privacy and maximizes your revenue.