How to Solve CORS Issues When Calling Ollama API from a Chrome Extension

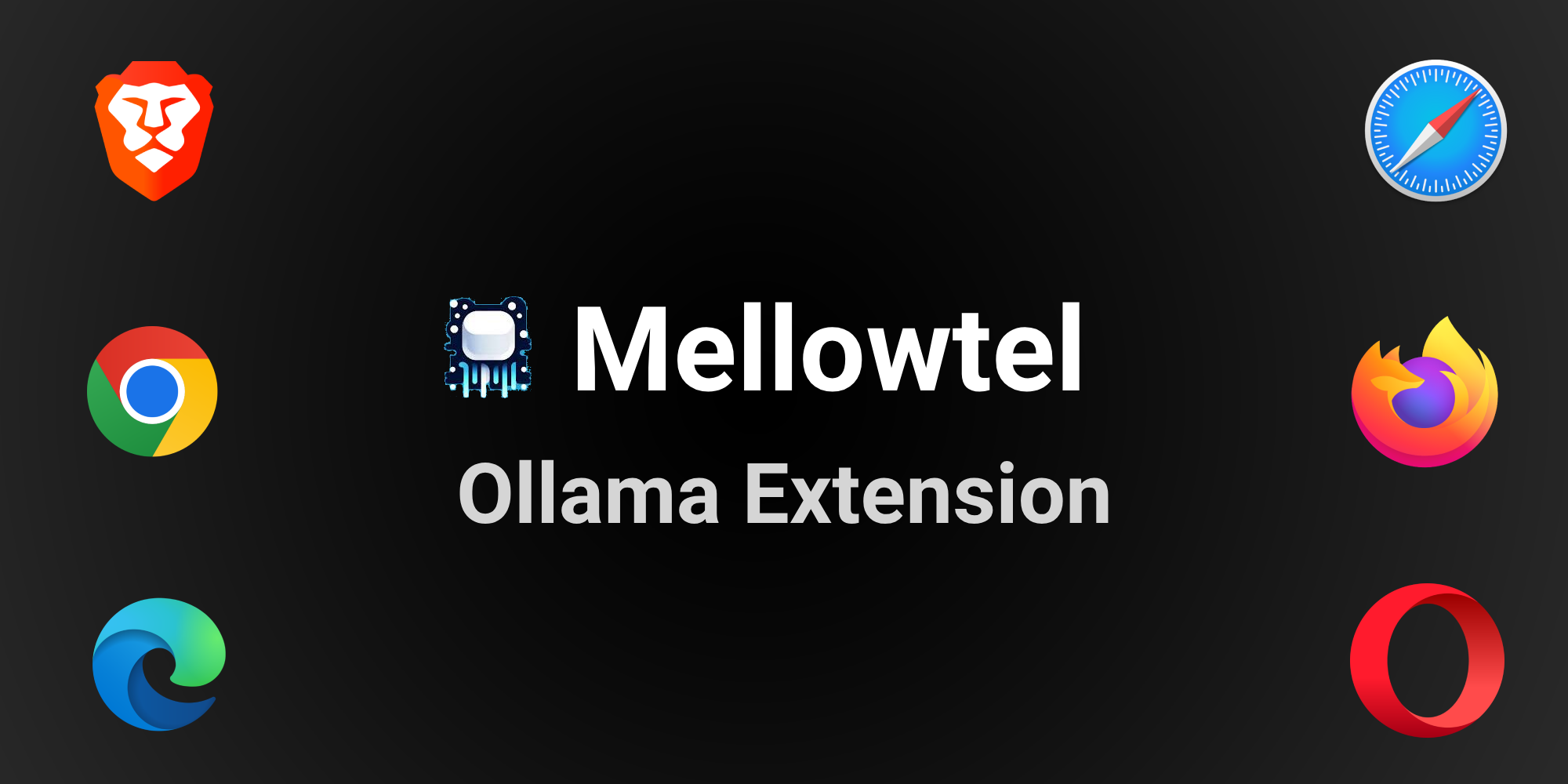
Integrating a local Ollama API with a Chrome Extension can be tricky due to CORS restrictions. This guide will walk you through the process of resolving these issues and successfully connecting your extension to Ollama.
The Challenge: 403 Forbidden Error
When attempting to call the Ollama API from a Chrome Extension, you may encounter a 403 Forbidden error. This occurs due to CORS (Cross-Origin Resource Sharing) restrictions, which prevent web applications from making requests to different domains.
Solution: Configuring Ollama and Chrome Extension
To resolve this issue, you need to make changes in both your Ollama configuration and your Chrome Extension's manifest file. Here's how:
1. Configure Ollama to Allow Chrome Extension Origins
Set the OLLAMA_ORIGIN
environment variable to allow Ollama to accept requests from Chrome Extension origins:
OLLAMA_ORIGINS=chrome-extension://* ollama serve
This command whitelists all Chrome Extension origins, enabling communication with Ollama.
2. Update Your Chrome Extension's Manifest File
In your manifest.json
file, set the permissions and host permissions correctly:
{
"permissions": [
"tabs",
"activeTab",
"http://localhost/*",
"scripting"
],
"host_permissions": ["http://localhost/*"]
}
This configuration grants your extension the necessary permissions to interact with localhost, where your Ollama instance is running.
Implementing the API Call
After making these changes, implement your API call function like this:
function generateCodeSuggestionFromOllama(prompt) {
const apiUrl = `http://localhost:11434/api/generate`;
const body = {
"model": "codellama",
"prompt": prompt,
"stream": false,
};
fetch(apiUrl, {
method: 'POST',
body: JSON.stringify(body),
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.text();
})
.then(data => {
const jsonData = JSON.parse(data);
const response = jsonData.response;
document.getElementById("codeSuggestion").innerHTML = response;
})
.catch(error => console.error('Error:', error));
}
Conclusion
By following these steps, you can successfully integrate Ollama with your Chrome Extension, overcoming CORS issues and enabling seamless API interactions. Always consider security implications when allowing cross-origin requests and only whitelist trusted sources.
Subscribe to my newsletter
Read articles from Mellowtel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mellowtel
Mellowtel
Easily monetize your browser extensions. Mellowtel is an open-source, ethical monetization engine that respects user privacy and maximizes your revenue.