Mastering Node.js Streams with TypeScript: A Complete Guide

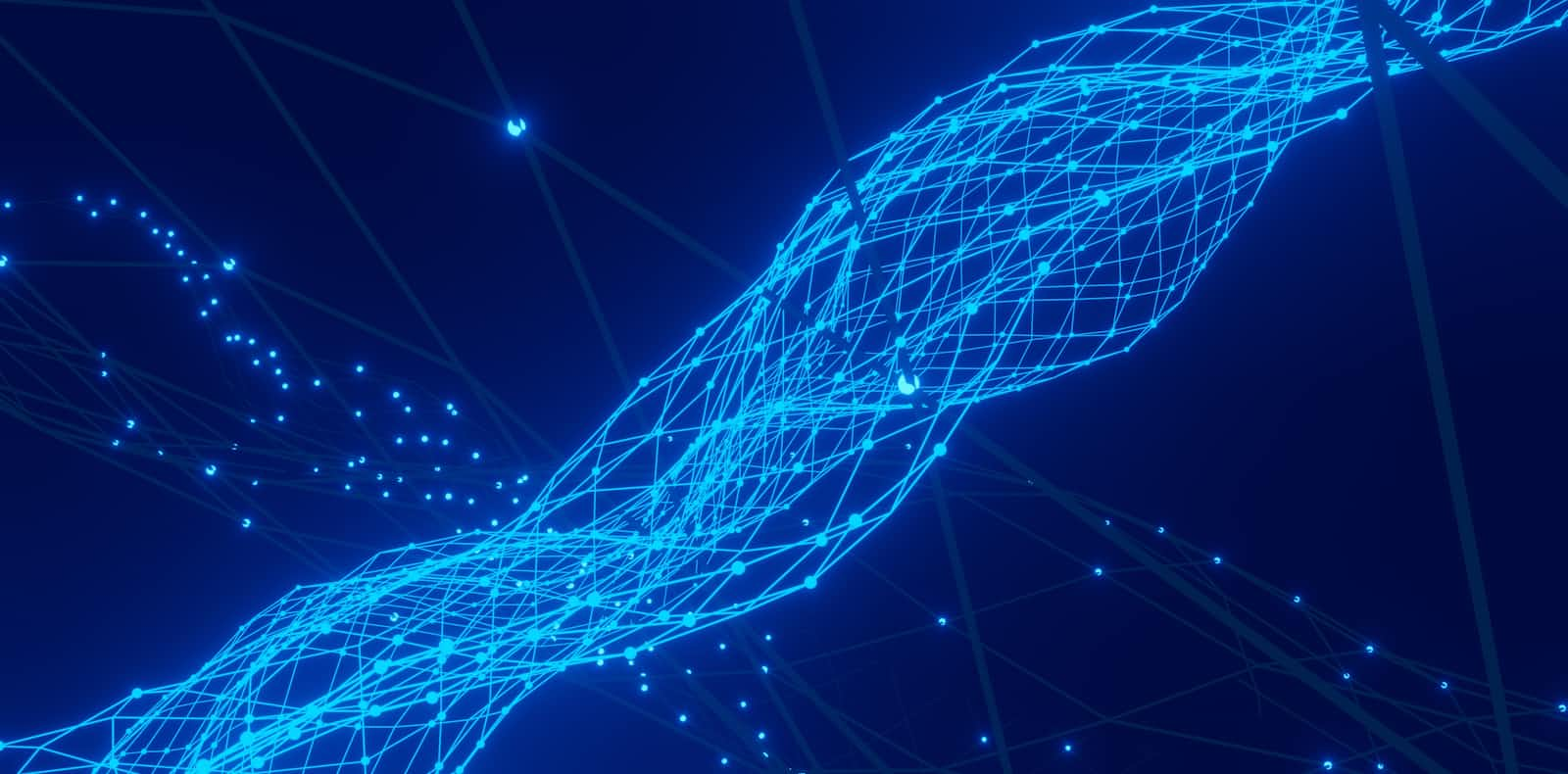
Streams are one of the core concepts in Node.js, enabling efficient reading and writing of large datasets. Instead of loading the entire content into memory, streams allow handling data chunk by chunk, making them especially useful for working with large files or network responses. In this article, we will explore the fundamentals of Node.js streams, and how to work with them using TypeScript.
What Are Streams?
Streams in Node.js are objects that facilitate the process of reading data from a source or writing data to a destination in a continuous fashion. They provide a powerful abstraction for handling data as it flows, rather than waiting for all of it to arrive.
Node.js has four types of streams:
Readable Streams: For reading data.
Writable Streams: For writing data.
Duplex Streams: Both readable and writable (e.g., a TCP socket).
Transform Streams: A special type of duplex stream where the output is a transformation of the input (e.g., file compression or encryption).
Why Use Streams?
Streams provide several advantages, especially when handling large amounts of data:
Memory Efficiency: By processing data chunk-by-chunk, streams reduce the memory footprint.
Time Efficiency: Streams process data as soon as it is available, reducing the time taken to process large datasets.
Pipelining: Streams can be piped together, which makes it easier to read from one stream and write to another.
Stream Basics in Node.js
Streams are instances of EventEmitter
, and they can emit several events like data
, end
, error
, and close
. Here’s a brief overview of these events:
data
: Emitted when a chunk of data is available.end
: Emitted when no more data is available.error
: Emitted in case of any error while processing the stream.close
: Emitted when the stream is closed.
Types of Streams with TypeScript Examples
Let's walk through examples for each stream type using TypeScript. TypeScript provides type safety and autocompletion, which can be very useful while working with streams.
1. Readable Streams
A readable stream is used to read data. In Node.js, this might be reading from a file or an HTTP request body.
import { createReadStream, ReadStream } from 'fs';
import { join } from 'path';
const filePath = join(__dirname, 'large-file.txt');
// Create a readable stream
const readableStream: ReadStream = createReadStream(filePath, {
encoding: 'utf8',
highWaterMark: 1024 // Chunk size of 1KB
});
// Listen for 'data' event
readableStream.on('data', (chunk: string) => {
console.log('Received chunk:', chunk);
});
// Listen for 'end' event
readableStream.on('end', () => {
console.log('No more data to read');
});
// Listen for 'error' event
readableStream.on('error', (error: Error) => {
console.error('Error reading file:', error);
});
Here, we are using the createReadStream
method to create a stream to read data from a file. We handle the data in chunks (1KB in this example) using the data
event, and log when the stream has ended with the end
event.
2. Writable Streams
Writable streams allow writing data. An example could be writing data to a file or responding to an HTTP request.
import { createWriteStream, WriteStream } from 'fs';
import { join } from 'path';
const outputPath = join(__dirname, 'output.txt');
// Create a writable stream
const writableStream: WriteStream = createWriteStream(outputPath, {
encoding: 'utf8'
});
// Write data to stream
writableStream.write('This is the first chunk.\n');
writableStream.write('This is the second chunk.\n');
// Listen for 'finish' event when all data is written
writableStream.on('finish', () => {
console.log('All data has been written.');
});
// End the writable stream
writableStream.end();
In this example, we write data to output.txt
using a writable stream. We call end()
to signal the end of writing, which triggers the finish
event.
3. Duplex Streams
A duplex stream is both readable and writable. It is commonly used in network communication or data transformation tasks.
import { Duplex } from 'stream';
class SimpleDuplex extends Duplex {
private data: string[] = [];
constructor() {
super();
}
// Implement _read method to push data
_read(size: number): void {
if (this.data.length > 0) {
this.push(this.data.shift());
} else {
this.push(null); // No more data to read
}
}
// Implement _write method to handle writing
_write(chunk: Buffer, encoding: string, callback: () => void): void {
console.log('Writing:', chunk.toString());
this.data.push(chunk.toString());
callback();
}
}
const duplexStream = new SimpleDuplex();
// Write to the duplex stream
duplexStream.write('First write\n');
duplexStream.write('Second write\n');
// Read from the duplex stream
duplexStream.on('data', (chunk: string) => {
console.log('Read:', chunk);
});
// End the stream
duplexStream.end();
In this example, we create a custom SimpleDuplex
stream that both reads and writes. We override the _read
and _write
methods to define how data is processed.
4. Transform Streams
A transform stream modifies the data as it passes through. A common use case is compressing or encrypting data.
import { Transform } from 'stream';
class UpperCaseTransform extends Transform {
// Implement _transform method to modify data
_transform(chunk: Buffer, encoding: string, callback: () => void): void {
const upperChunk = chunk.toString().toUpperCase();
this.push(upperChunk);
callback();
}
}
const transformStream = new UpperCaseTransform();
// Write to the transform stream
transformStream.write('This is a test.\n');
transformStream.write('This will be uppercased.\n');
// Read from the transform stream
transformStream.on('data', (chunk: string) => {
console.log('Transformed:', chunk);
});
// End the stream
transformStream.end();
Here, we use a transform stream to convert the data into uppercase as it flows through the stream. This is useful for various real-world scenarios like data compression, encryption, or formatting.
Piping Streams
One of the most powerful features of streams is piping. You can connect streams to each other so that the output of one stream feeds into the input of another.
import { createReadStream, createWriteStream } from 'fs';
import { join } from 'path';
import { pipeline } from 'stream';
const inputFile = join(__dirname, 'large-file.txt');
const outputFile = join(__dirname, 'output.txt');
// Create read and write streams
const readable = createReadStream(inputFile);
const writable = createWriteStream(outputFile);
// Pipe the readable stream to the writable stream
pipeline(readable, writable, (error) => {
if (error) {
console.error('Pipeline failed:', error);
} else {
console.log('Pipeline succeeded');
}
});
In this example, we read from a file and directly pipe the data into another file, creating an efficient data flow.
Error Handling in Streams
Error handling is crucial when working with streams. Streams can fail for various reasons such as file read/write errors or network issues. You should always listen for the error
event.
readableStream.on('error', (err) => {
console.error('Stream Error:', err);
});
writableStream.on('error', (err) => {
console.error('Stream Error:', err);
});
Conclusion
Streams are an essential feature of Node.js, providing a powerful way to handle data efficiently by processing it in chunks rather than loading everything into memory. Whether you're dealing with file I/O, handling network traffic, or manipulating large datasets, mastering streams allows you to build applications that are both memory-efficient and highly performant. By leveraging readable, writable, duplex, and transform streams, you can streamline your workflows, handle large-scale data operations with ease, and ultimately create more scalable, responsive Node.js applications. With a deep understanding of streams, you'll be better equipped to tackle complex real-world challenges and optimize your application's resource usage.
In this article, we’ve covered:
Different types of streams: Readable, Writable, Duplex, and Transform.
How to work with streams in TypeScript, adding type safety and clarity.
How to handle common tasks such as piping and error handling.
With streams, you can efficiently manage data flow in Node.js applications, scaling your applications for larger and more complex tasks.
Subscribe to my newsletter
Read articles from Rohit Paul directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
