Understanding Profile-Guided Optimization (PGO) in Go

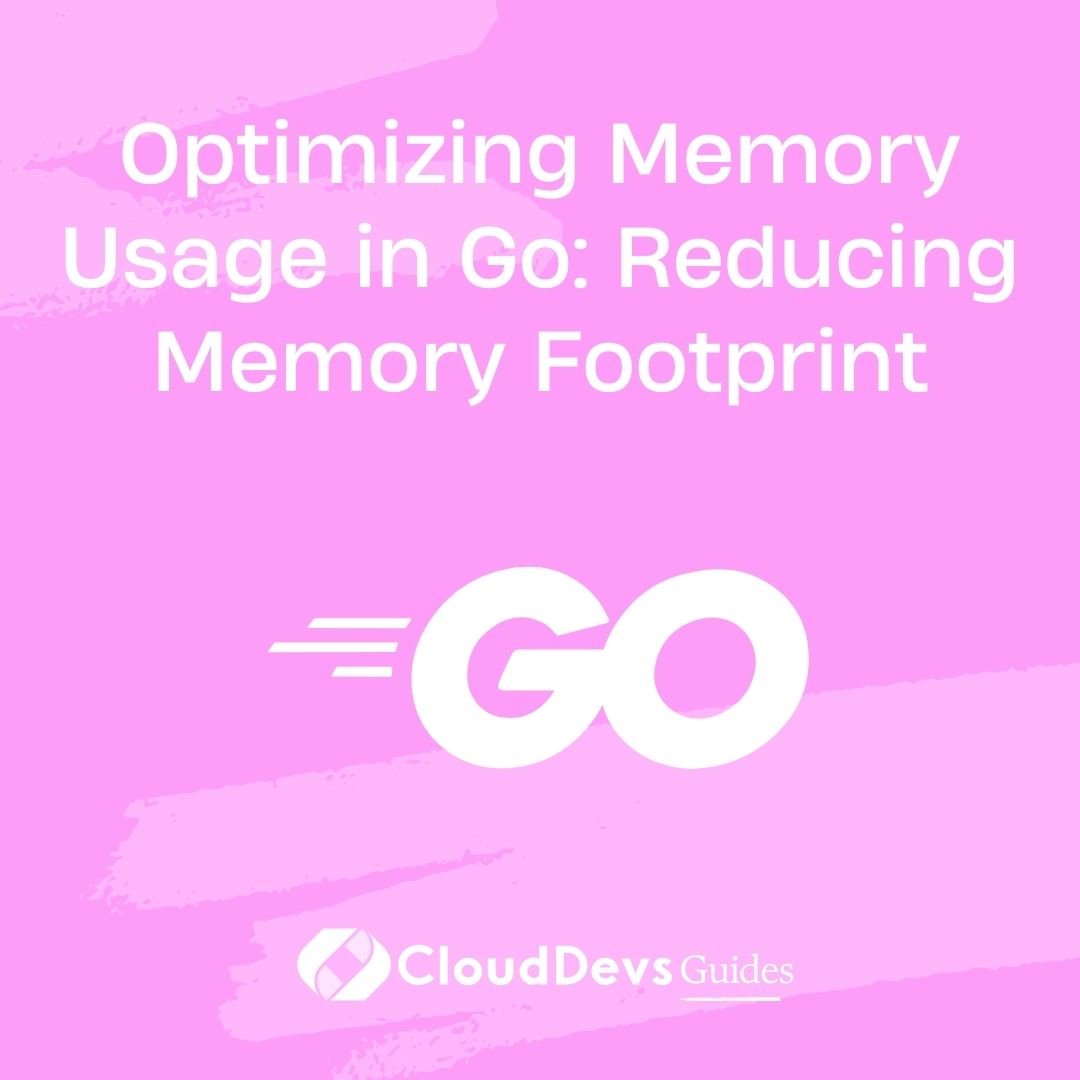
Profile-Guided Optimization (PGO), also known as Feedback-Directed Optimization (FDO), is a powerful compiler technique that enhances the performance of applications by using real-world data to inform optimization decisions during the build process. Starting from Go version 1.20, the Go compiler supports PGO to further optimize Go programs.
What is PGO?
PGO involves the following steps:
Collecting Profiling Data: Run your application to gather profiling information that reflects typical usage patterns. This data is usually collected from production environments to ensure it is representative.
Feeding Data Back to the Compiler: Use the collected profile during the next build of your application. The compiler utilizes this information to optimize the code more effectively.
Optimizing Based on Actual Usage: The compiler may choose to inline frequently called functions, unroll loops, or make other optimizations that are beneficial based on the profiling data.
Benefits of Using PGO in Go
Performance Improvements: Benchmarks have shown that using PGO can improve the performance of Go applications by approximately 2-14%.
Smarter Optimizations: The compiler makes more informed decisions, leading to better-optimized binaries that perform well under real-world conditions.
Future Enhancements: As Go continues to evolve, more optimizations will be introduced, potentially increasing the benefits of PGO.
How to Collect Profiles
To use PGO effectively, you need to collect CPU profiles that represent your application's typical workload:
Use Go's Profiling Tools:
Employ the
runtime/pprof
ornet/http/pprof
packages to collect CPU profiles.These profiles record which parts of your code are most active during execution.
Collect from Production Environments:
Profiles should be gathered from production or environments that closely mimic production to ensure they are representative.
Unrepresentative profiles may not lead to performance gains and could even overlook critical optimizations.
Merge Multiple Profiles if Necessary:
If your application has varying workloads, consider collecting multiple profiles and merging them using the
pprof
tool:go tool pprof -proto profile1.pprof profile2.pprof > merged.pprof
Building with PGO
After collecting the profiles, you can build your Go application using PGO:
Save the Profile Appropriately:
- Place the profile file (e.g.,
default.pgo
) in the main package directory of your application.
- Place the profile file (e.g.,
Automatic Detection:
- By default,
go build
will detectdefault.pgo
and use it for PGO without additional flags.
- By default,
Using the -pgo Flag:
If you have profiles with different names or locations, specify the profile using the
-pgo
flag:go build -pgo=/path/to/your/profile.pprof
To disable PGO explicitly, use
-pgo=off
.
Handling Multiple Binaries:
- When building multiple binaries, run separate
go build
commands if you need to use different profiles for each.
- When building multiple binaries, run separate
Best Practices and Notes
Iterative Workflow:
Regularly update your profiles and rebuild your application to maintain optimal performance.
The typical workflow involves building, collecting profiles, and rebuilding with PGO.
Source Stability:
Go's PGO is designed to handle changes in your codebase gracefully.
Minor changes or refactoring usually do not significantly impact PGO effectiveness.
Performance of New Code:
- Newly added code won't benefit from PGO until new profiles are collected that include its execution.
Build Time and Binary Size:
Enabling PGO may increase build times due to additional compilation steps.
Binary size might slightly increase because of more aggressive inlining and optimizations.
Frequently Asked Questions
Q1: Can PGO optimize standard library packages and dependencies?
Yes, PGO in Go applies to the entire program, including standard library packages and any dependencies your application uses.
Q2: Will using an unrepresentative profile make my program slower?
Generally, no. While an unrepresentative profile might not provide performance gains, it should not degrade the performance of your application.
Q3: Can I use the same profile for different operating systems or architectures?
Yes, profiles are compatible across different GOOS/GOARCH
settings. However, platform-specific code may not benefit if it differs significantly between platforms.
Q4: How should I handle an application used for different workloads?
Option 1: Build separate binaries for each workload using profiles specific to each.
Option 2: Use profiles from the most critical workload.
Option 3: Merge profiles from all workloads to create a general-purpose optimized binary.
Q5: How does PGO affect build time and binary size?
Enabling PGO can increase build times due to the extra analysis required. Binary sizes may also increase slightly because of additional optimizations like function inlining.
Summary
Profile-Guided Optimization (PGO) in Go leverages real-world usage data to enhance the performance of Go applications. By collecting CPU profiles from representative runs and feeding them back into the compiler, you enable the compiler to make informed optimization decisions tailored to your application's actual behavior.
Steps to Implement PGO in Go:
Collect CPU Profiles: Use Go's profiling tools during typical application runs.
Prepare the Profile: Save the profile file in the main package directory or specify it with the
-pgo
flag.Build with PGO: Run
go build
, and the compiler will use the profile to optimize your application.
Key Takeaways:
PGO can significantly improve application performance.
Regularly updating profiles ensures ongoing optimization benefits.
PGO is designed to be robust against code changes and iterative builds.
Properly handling profiles when dealing with multiple workloads or platforms is essential for maximizing performance gains.
By incorporating PGO into your Go development workflow, you can achieve more efficient and faster applications that better utilize system resources based on actual usage patterns.
You can also learn more about it here
Subscribe to my newsletter
Read articles from Sundaram Kumar Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sundaram Kumar Jha
Sundaram Kumar Jha
I Like Building Cloud Native Stuff , the microservices, backends, distributed systems and cloud native tools using Golang