JavaFX: Creating Rich Desktop Applications
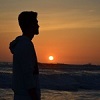
JavaFX is a powerful toolkit for building rich, interactive desktop applications using the Java programming language. It provides a comprehensive set of features for creating modern user interfaces, including graphics, controls, animations, and effects.
Key Features of JavaFX
Graphics: JavaFX offers a rich graphics API for drawing shapes, lines, and images. You can also create custom graphics components and apply various effects like shadows, blurs, and glows.
Controls: JavaFX provides a wide range of pre-built controls, such as buttons, labels, text fields, checkboxes, and more. You can customise these controls to match your application's style and behaviour.
Layouts: JavaFX offers flexible layout managers like AnchorPane, BorderPane, GridPane, and VBox to arrange controls within your application.
Animations: JavaFX makes it easy to create animations for various elements, including controls, shapes, and images. You can animate properties like position, size, color, and opacity.
Effects: JavaFX includes a variety of effects that can be applied to controls and graphics, such as dropshadow, blur, and lighting.
Getting Started with JavaFX
To get started with JavaFX, you'll need to have the Java Development Kit (JDK) installed on your system. Once you have the JDK, you can create a new JavaFX project using your preferred IDE or build tool.
Here's a basic JavaFX application that displays a simple label:
Java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class HelloWorld extends Application {
public void start(Stage primaryStage) {
Label label = new Label("Hello, World!");
VBox layout = new VBox(20);
layout.getChildren().add(label);
Scene scene = new Scene(layout, 400, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Hello, World!");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
This code creates a label with the text "Hello, World!" and places it inside a vertical layout. The layout is then added to a scene, which is set to the primary stage of the application. Finally, the stage is shown, making the application visible.
Creating a More Complex User Interface
Once you have the basics down, you can start creating more complex user interfaces. Here are some examples of what you can do with JavaFX:
Build custom controls: You can create your own custom controls by extending the Control class and overriding its methods.
Use FXML: FXML is a declarative language for defining user interfaces in JavaFX. It allows you to separate the UI from the application logic.
Integrate with other technologies: JavaFX can be integrated with other technologies, such as web services and databases.
Create animations and effects: You can use JavaFX to create stunning animations and effects that make your applications more engaging.
Advantages of Using JavaFX
There are several advantages to using JavaFX for building desktop applications:
Cross-platform compatibility: JavaFX applications can run on a variety of platforms, including Windows, macOS, and Linux.
Rich feature set: JavaFX provides a comprehensive set of features for creating modern user interfaces.
Integration with Java ecosystem: JavaFX integrates seamlessly with the Java ecosystem, allowing you to leverage existing Java libraries and frameworks.
Strong community support: JavaFX has a large and active community that provides support, resources, and contributions.
If you want to enhance your Java skills, many Java training course provider in Noida, Delhi, Pune, and other Indian cities offer comprehensive programs that include JavaFX training. These programs provide an excellent opportunity to deepen your understanding of this powerful toolkit.
In conclusion, JavaFX is a powerful and versatile toolkit for building rich desktop applications. With its rich feature set, cross-platform compatibility, and strong community support, JavaFX is an excellent choice for developers looking to create modern and engaging user interfaces.
Subscribe to my newsletter
Read articles from Sanjeet Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
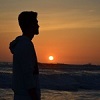
Sanjeet Singh
Sanjeet Singh
I am Sanjeet Singh, an IT professional with experience in the IT sector. I have a broad understanding of Data Analytics and proficiency across multiple layers of software development and testing, from the front end to the back end.