How to create infinite scrolling cards in react & next.js?
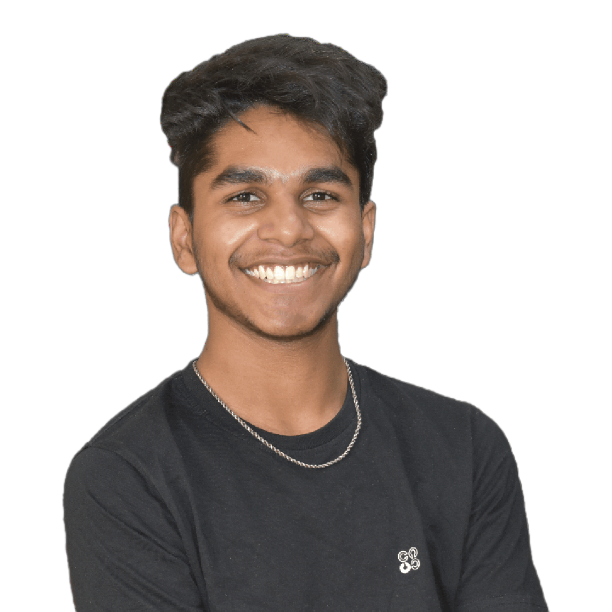
Recently, at my agency UNIOdessy, we decided to add a new element to showcase our work. We tried various components, but none of them matched our aesthetic. So, we created and re-designed a scroller, a component that does the job for us.
Step 1: Install MagicUI and add the Marquee element.
npx magicui-cli add marquee
Step 2: Create a file named Marquee.tsx/jsx in the components folder.
Step 3: Copy the provided code into your Marquee.tsx/jsx file.
import { cn } from "@/lib/utils";
import Marquee from "@/components/magicui/marquee";
const reviews = [
{
img: "./works/work1.png",
},
{
img: "./works/work2.png",
},
];
const firstRow = reviews;
const ReviewCard = ({ img }: { img: string }) => {
return (
<figure
className={cn(
"relative cursor-pointer overflow-hidden rounded-xl border p-4",
"border-gray-950/[.1] bg-gray-950/[.01] hover:bg-gray-950/[.05]",
"dark:border-gray-50/[.1] dark:bg-gray-50/[.10] dark:hover:bg-gray-50/[.15]"
)}
>
<img className="w-auto h-[500px] rounded md:h-[500px] object-top lg:border lg:border-white/5 " alt="" src={img} />
</figure>
);
};
export function Marquee() {
return (
<div className="relative flex h-[500px] w-full flex-col items-center justify-center overflow-hidden rounded-lg bg- md:shadow-xl">
<Marquee pauseOnHover className="[--duration:20s]">
{firstRow.map((review) => (
<ReviewCard key={review.img} {...review} />
))}
</Marquee>
</div>
);
}
Step 4: Import and use the Marquee component in your App.js file.
import React from 'react';
import Marquee from './components/Marquee';
function App() {
return (
<div className="App">
<header className="App-header">
<h1>Welcome to UNIOdessy</h1>
<Marquee />
</header>
</div>
);
}
export default App;
And voila! Add your own images, and you're done.
Follow me for more tips and tricks. Also you can connect with me at my portfolio.
Subscribe to my newsletter
Read articles from Roopesh Vetcha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
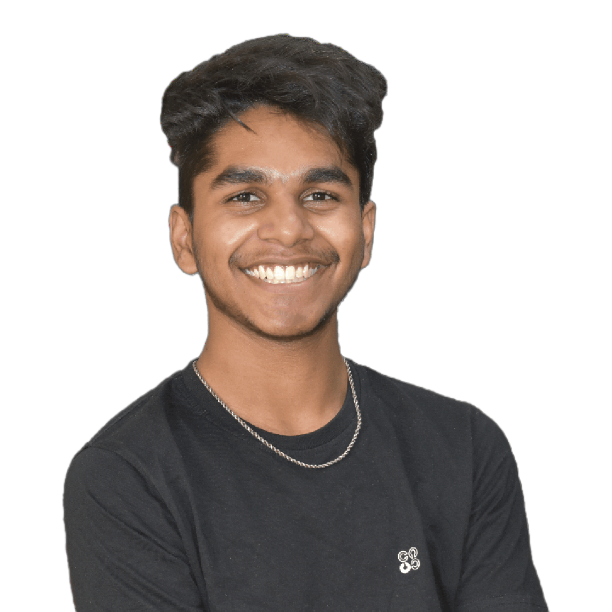
Roopesh Vetcha
Roopesh Vetcha
I'm just everything lol