Let's start
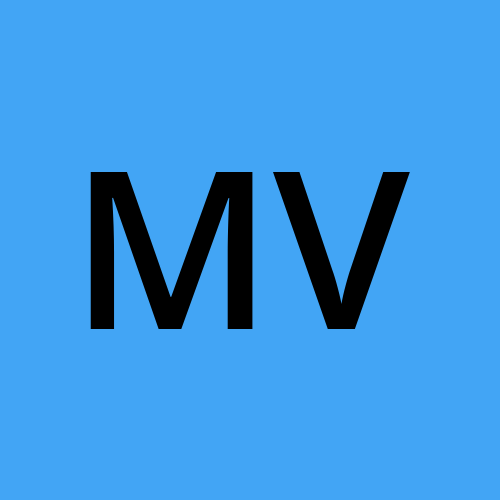
Since we have to start somewhere, beginning with the APIs seems like a good idea.
APIs are the heart of communication between the front-end and the back-end, and defining them at the beginning will help me establish a clear structure and ensure that all necessary functionalities are covered.
Let's start by identifying the functionalities that the CMS will need to support.
Functionalities
GET /contents: Retrieves all Contents.
POST /contents: Creates a new Content.
GET /contents/{id}: Retrieves a specific Content.
PUT /contents/{id}: Updates an existing Content.
DELETE /contents/{id}: Deletes a Content.
Starting a Kotlin project and testing it with JUnit involves several steps.
Here's a structured approach to set up the project:
The Set Up
(Initializing a brand new Kotlin Project)
I could use a tool like Gradle or Maven. Since I don't know nothing of both, I'll use Gradle, as it's widely used and, most of all is used by the backend developers I work with.
Then I moved into the build.gradle.kts file and I added the springboot dependencies
// Spring Boot Starter Web for building web applications.
implementation("org.springframework.boot:spring-boot-starter-web")
// Spring Boot Starter Data MongoDB for MongoDB support.
implementation("org.springframework.boot:spring-boot-starter-data-mongodb")
Spring and Spring Boot
Spring Boot is an open-source, microservice-based Java framework used to create stand-alone, Spring-based applications with minimal effort. It is part of the larger Spring ecosystem and simplifies the process of bootstrapping and developing Spring applications by providing a wide range of pre-configured templates and conventions.
Key Features:
Auto-Configuration: Spring Boot automatically configures your application based on the dependencies you have added to your project.
Embedded Servers: Spring Boot includes embedded servers such as Tomcat, Jetty, and Undertow, allowing you to run your application as a self-contained unit without the need for a separate server installation.
Spring Boot Starters: Starters are a set of convenient dependency descriptors you can include in your application. They simplify dependency management by aggregating commonly used libraries for specific functionalities, like web development, security, or data access.
Folder Structure
Inside the src.main.kotlin.com.cms.application package, I’m going to add these files:
.
├── CmsApplication.kt
├── controllers
│ └── ContentController.kt
└── model
└── Content.kt
Controllers
Here you place your controllers, such as ContentController.kt
. This folder is responsible for handling HTTP requests and responses.
this is the code of the ContentController class
package com.your_package.controller
import com.your_package.model.Content
import org.springframework.web.bind.annotation.*
@RestController
@RequestMapping("/contents")
class ContentController {
// Initialization of the mutable list of Content
private val contents: MutableList<Content> = mutableListOf()
@GetMapping
fun getContents(): List<Content> {
return contents
}
@PostMapping
fun addContent(@RequestBody content: Content) {
contents.add(content)
}
}
mutableListOf()
, you are creating a new empty mutable list. This is necessary to avoid compilation errors when Kotlin looks for an initial value for the property.Models
Here you place your data models, such as Content.kt
. This folder contains classes that represent the entities of your application.
this is the model
package com.your_package.model
data class Content(
val id: Long,
val title: String,
val description: String
)
Project Root
This folder can contain the main class of the application (for example, CmsApplication.kt
). It is the class where you start the Spring Boot application.
Subscribe to my newsletter
Read articles from Matteo Villa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
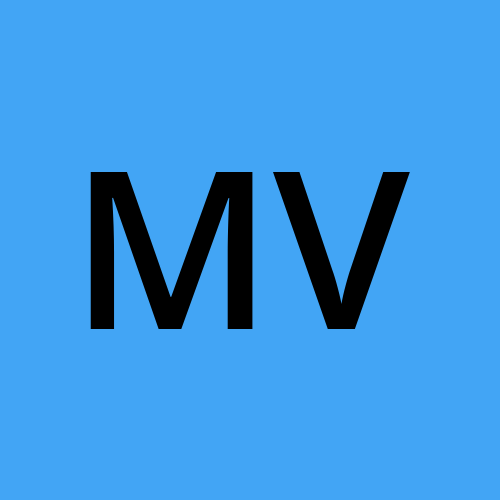