Step-by-Step Guide to Making Image PDFs in Python with ReportLab and EasyGUI 🔥

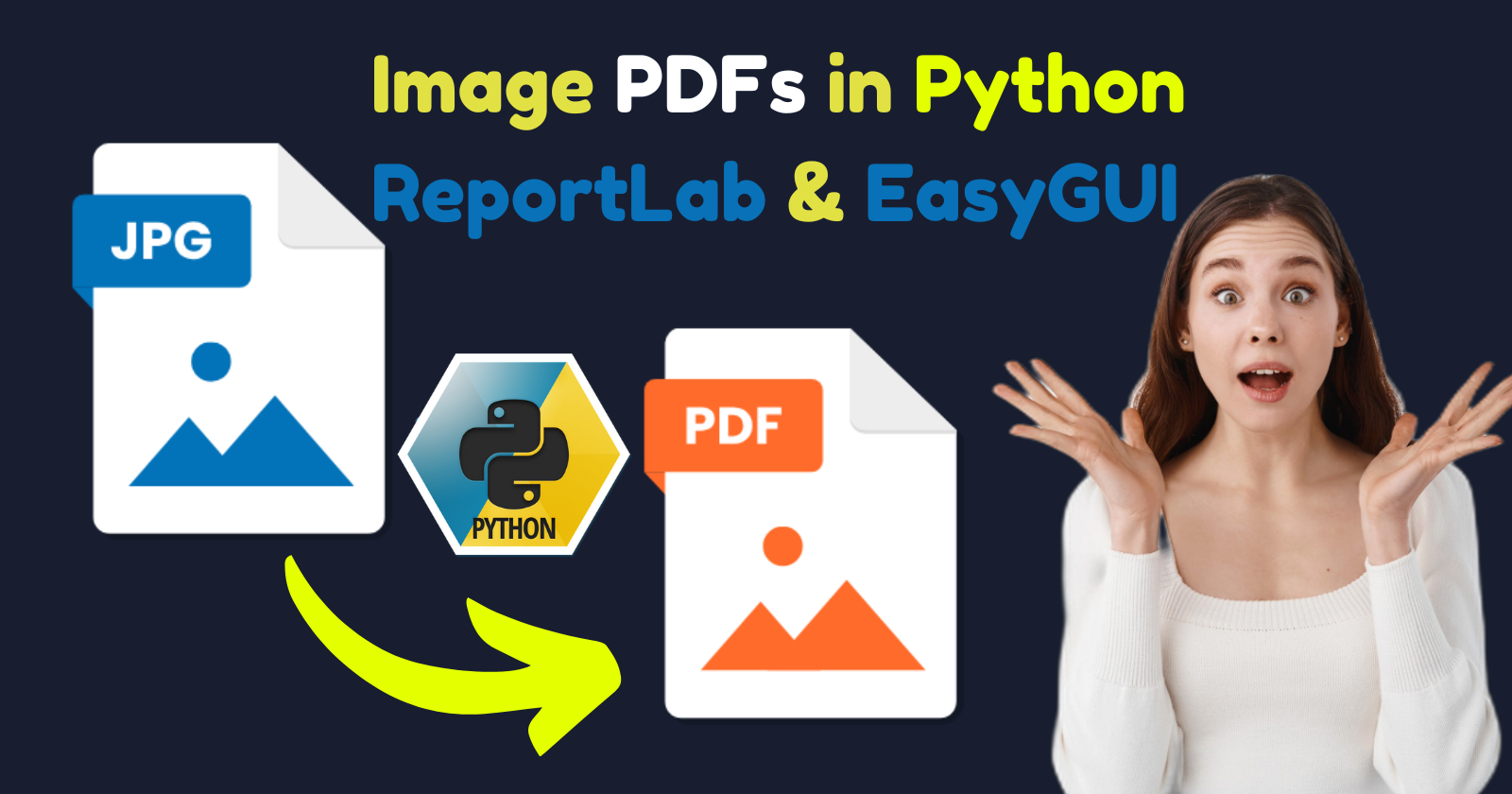
Creating PDF documents programmatically in Python is a powerful way to automate reporting or file generation. With the ReportLab
library, you can generate PDFs that include not only text but also images, shapes, and complex layouts. To make this process even more user-friendly, we can integrate EasyGUI
, a simple GUI tool that allows users to interact with the script using graphical dialogs instead of the command line. #2Articles1Week, #Hashnode.
In this article, we’ll explore two different Python scripts:
A basic image-to-PDF generator using
ReportLab
.A user-friendly version that allows image selection through a file dialog using
EasyGUI
.
1. Basic Script: Generating PDFs with Images Using ReportLab
The ReportLab
library provides powerful tools for creating PDFs from scratch in Python. In this example, we will generate a PDF with an image inserted into it.
Prerequisites
Before you start, make sure you have ReportLab
installed:
pip install easygui reportlab
Python Script
import easygui
from reportlab.pdfgen import canvas
from reportlab.lib.pagesizes import A4
from reportlab.lib.units import inch
# Open a file dialog to select multiple images using EasyGUI
image_paths = easygui.fileopenbox(
title="Select Images", filetypes=["*.png", "*.jpg", "*.jpeg", "*.gif"], multiple=True
)
if image_paths:
# Create a canvas for the PDF
pdf_file = 'multiple_images_pdf.pdf'
c = canvas.Canvas(pdf_file, pagesize=A4)
# Set initial coordinates for images
x = 100 # X-coordinate
y = 600 # Y-coordinate
# Loop through each selected image and add it to the PDF
for image_path in image_paths:
# Define the image size (you can modify this as needed)
width = 4 * inch # Width of the image
height = 3 * inch # Height of the image
# Add the image to the PDF
c.drawImage(image_path, x, y, width, height)
# Add the image file name as text below the image
c.drawString(x, y - 20, f"Image: {image_path}")
# Move to the next page for each image
c.showPage()
# Save the PDF
c.save()
easygui.msgbox(f"PDF '{pdf_file}' created successfully with multiple images!", title="Success")
else:
easygui.msgbox("No images selected. Exiting...", title="Cancelled")
🔥How It Works
Image Selection via EasyGUI:
easygui.fileopenbox()
opens a file dialog box that allows the user to browse and select an image. Thefiletypes
argument restricts the selection to common image formats like PNG and JPEG.
PDF Creation with ReportLab:
- Once an image is selected, it is inserted into a PDF in a similar fashion to the previous example.
User Feedback:
- If the PDF is created successfully, the script displays a success message using
easygui.msgbox()
. If no image is selected, the script notifies the user that the process has been cancelled.
User Experience
This script simplifies the interaction for non-technical users. They can simply run the script, select an image through a dialog box, and automatically generate a PDF with the chosen image.
Advantages
No Command Line Input: The user does not need to manually input file paths, which is especially useful in GUI-based environments.
Simplified Image Selection: EasyGUI provides an intuitive way to select files, making the process easier for non-programmers.
🎉Conclusion
By combining the strengths of ReportLab
and EasyGUI
, you can create powerful and user-friendly PDF generation tools in Python. The first script demonstrates the basics of PDF creation, while the second script takes it a step further by adding a graphical interface for file selection. Both are great starting points for more advanced projects where automated PDF generation is needed.
Whether you're generating reports, invoices, or certificates, these tools allow you to automate and simplify the PDF creation process while keeping it customizable and interactive for users.
Happy Coding ❤
Subscribe to my newsletter
Read articles from Shubham Sutar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Sutar
Shubham Sutar
"Tech enthusiast and blogger exploring the latest in gadgets, software, and innovation. Passionate about simplifying tech for everyday users and sharing insights on trends that shape the future."