#9.0 What XPath: Troubleshooting
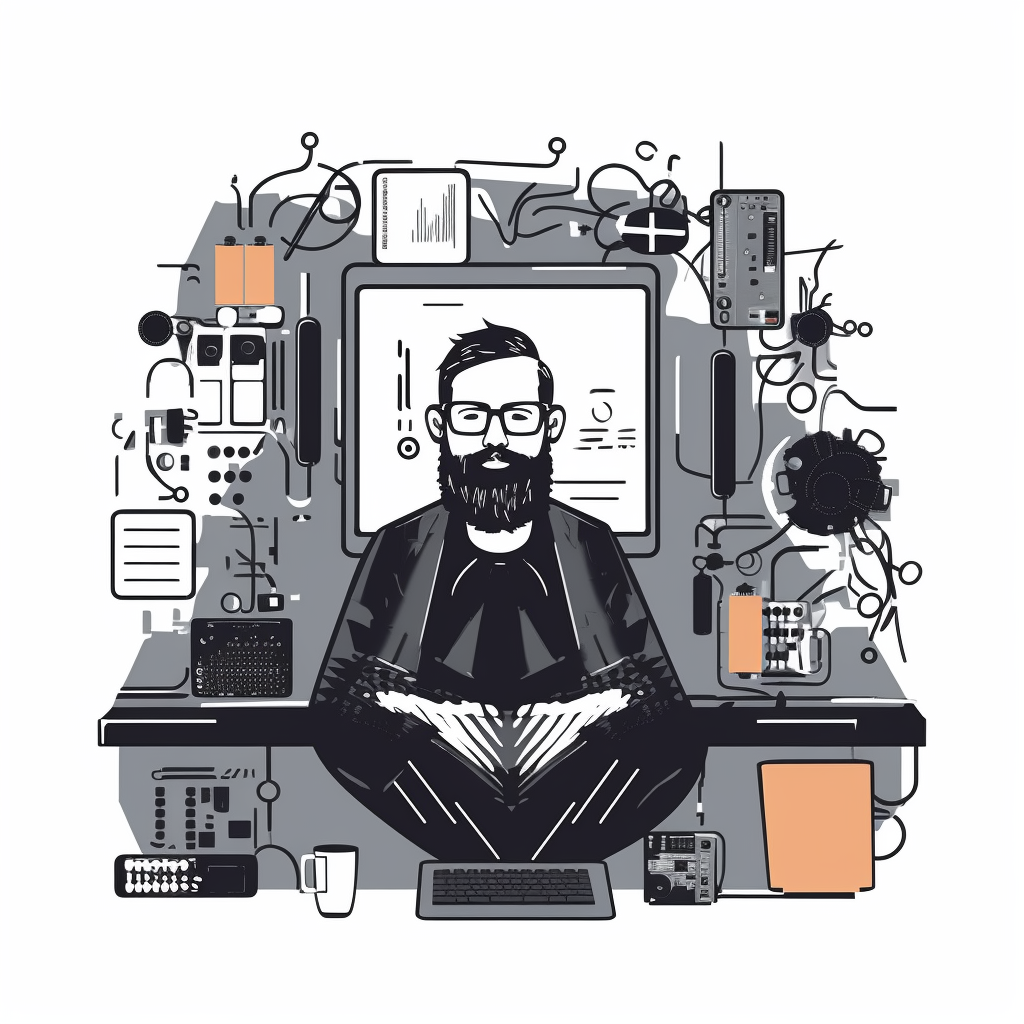
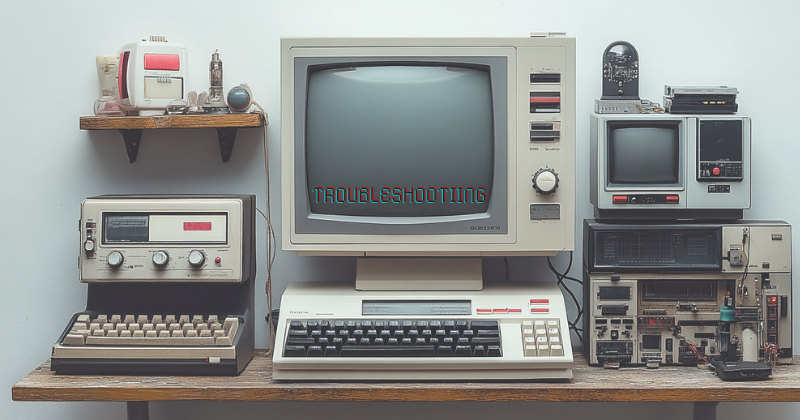
When working with XPath in Selenium or other web automation tools, you may encounter various issues. This section will help you identify, understand, and resolve common XPath-related problems.
9.1 Common XPath Errors and Their Solutions
9.1.1 NoSuchElementException
Problem: Selenium throws a NoSuchElementException
when trying to locate an element.
Possible Causes:
The XPath is incorrect or doesn't match any elements.
The element hasn't loaded yet when the XPath is evaluated.
The element is inside an iframe or shadow DOM.
Solutions:
Double-check your XPath using browser developer tools.
Implement explicit or implicit waits:
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.common.by import By
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.XPATH, "//your/xpath/here"))
)
- Switch to the correct iframe before locating the element:
driver.switch_to.frame("frame_name_or_id")
# Now locate your element
driver.switch_to.default_content() # Switch back to main content
9.1.2 StaleElementReferenceException
Problem: The element is no longer attached to the DOM.
Possible Causes:
The page has been refreshed after the element was located.
JavaScript has updated the DOM, removing or replacing the element.
Solutions:
Re-locate the element before interacting with it.
Use a try-except block to catch the exception and re-locate the element:
def click_element(driver, xpath):
max_attempts = 3
attempts = 0
while attempts < max_attempts:
try:
element = driver.find_element(By.XPATH, xpath)
element.click()
return
except StaleElementReferenceException:
attempts += 1
raise Exception("Element remained stale after multiple attempts")
9.1.3 InvalidSelectorException
Problem: The XPath syntax is invalid.
Possible Causes:
Typos in the XPath expression.
Using XPath 2.0 features in an XPath 1.0 environment.
Solutions:
Validate your XPath syntax using online tools or browser extensions.
Ensure you're using XPath 1.0 compatible syntax if working with older systems.
9.2 XPath Performance Issues
9.2.1 Slow XPath Queries
Problem: XPath queries are taking too long to execute.
Possible Causes:
Using // at the beginning of the XPath, forcing a full DOM traversal.
Overly complex XPath expressions.
Solutions:
Start with a specific path when possible:
/html/body/div/...
instead of//div/...
Use more efficient locators when available (ID, name, etc.)
Optimize your XPath:
Instead of
//div[contains(@class, 'example')]
, use//div[contains(concat(' ', normalize-space(@class), ' '), ' example ')]
Use indexing when possible:
(//div[@class='example'])[1]
instead of//div[@class='example'][1]
9.3 Browser-Specific XPath Issues
Problem: XPath works in one browser but not in another.
Possible Causes:
Different browsers may have slight differences in DOM structure.
Some XPath functions may not be supported in all browsers.
Solutions:
Use more robust XPath that doesn't rely on specific DOM structures.
Test your XPath in all target browsers.
Consider using CSS selectors for better cross-browser compatibility.
9.4 Dynamic Content and XPath
Problem: XPath fails to locate elements in dynamically loaded content.
Solutions:
Implement waits as mentioned in 9.1.1.
Use XPath that targets stable parts of the DOM and navigates to dynamic content:
# Instead of directly targeting a dynamic element
dynamic_element = driver.find_element(By.XPATH, "//div[@id='dynamic-content']/p")
# Target a stable parent and then find the dynamic child
stable_parent = driver.find_element(By.XPATH, "//div[@id='stable-parent']")
dynamic_element = stable_parent.find_element(By.XPATH, ".//div[@id='dynamic-content']/p")
9.5 Tools for Debugging XPath
Browser Developer Tools: Use the Console to test XPath expressions:
$x("//your/xpath/here")
XPath Helper: A Chrome extension for testing and debugging XPath.
FirePath: A Firefox add-on for XPath and CSS selector generation and testing.
XPath Tester Websites: Online tools like XPathTester to validate and test your XPath expressions.
9.6 Best Practices for Avoiding XPath Issues
Use descriptive and specific XPath when possible.
Avoid using indexes in XPath unless absolutely necessary.
Prefer IDs and unique attributes over complex XPath expressions.
Regularly maintain and update your XPath selectors as the website changes.
Implement proper waits and handle exceptions gracefully.
Use XPath axes to create more robust selectors.
Comment your XPath expressions to explain complex logic.
By following these troubleshooting tips and best practices, you can significantly reduce XPath-related issues in your web automation projects and create more robust, maintainable test scripts.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
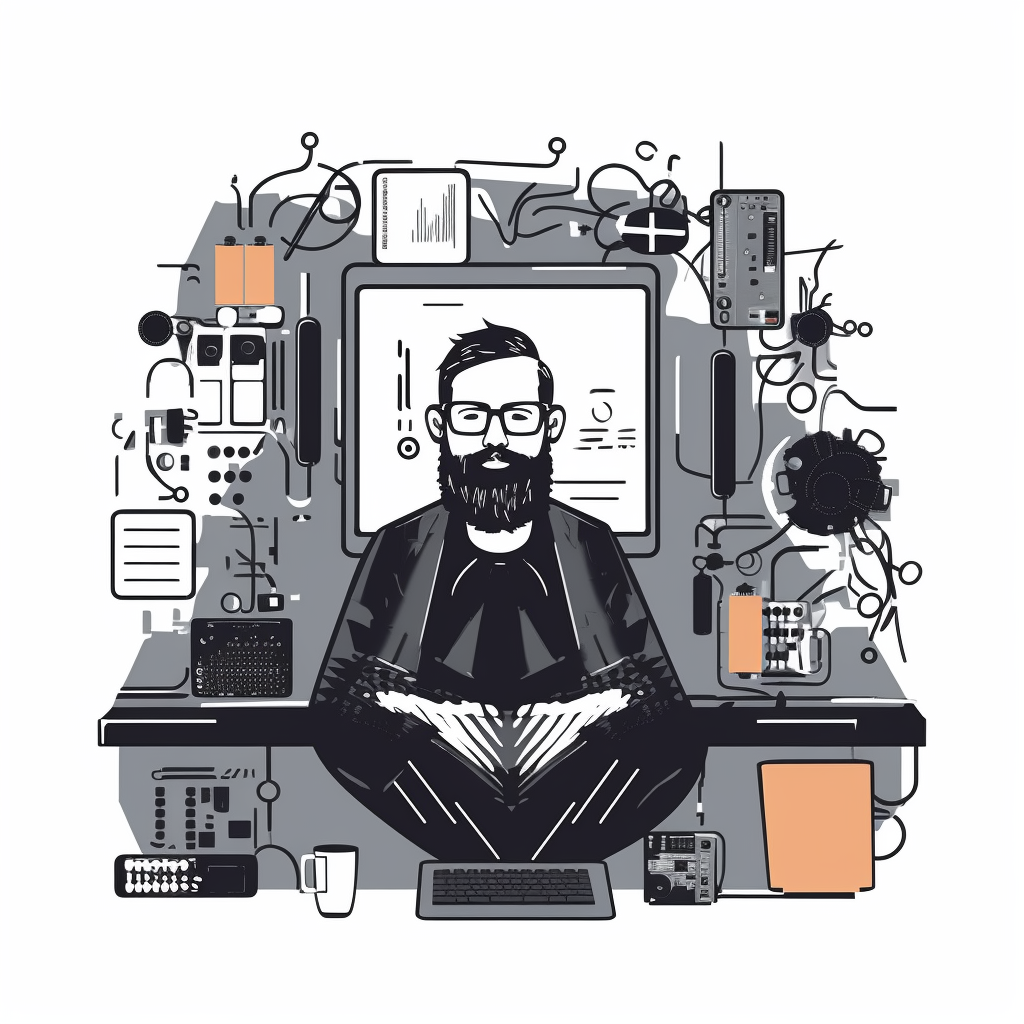
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.