bad API fetch VS good API fetch in JavaScript.
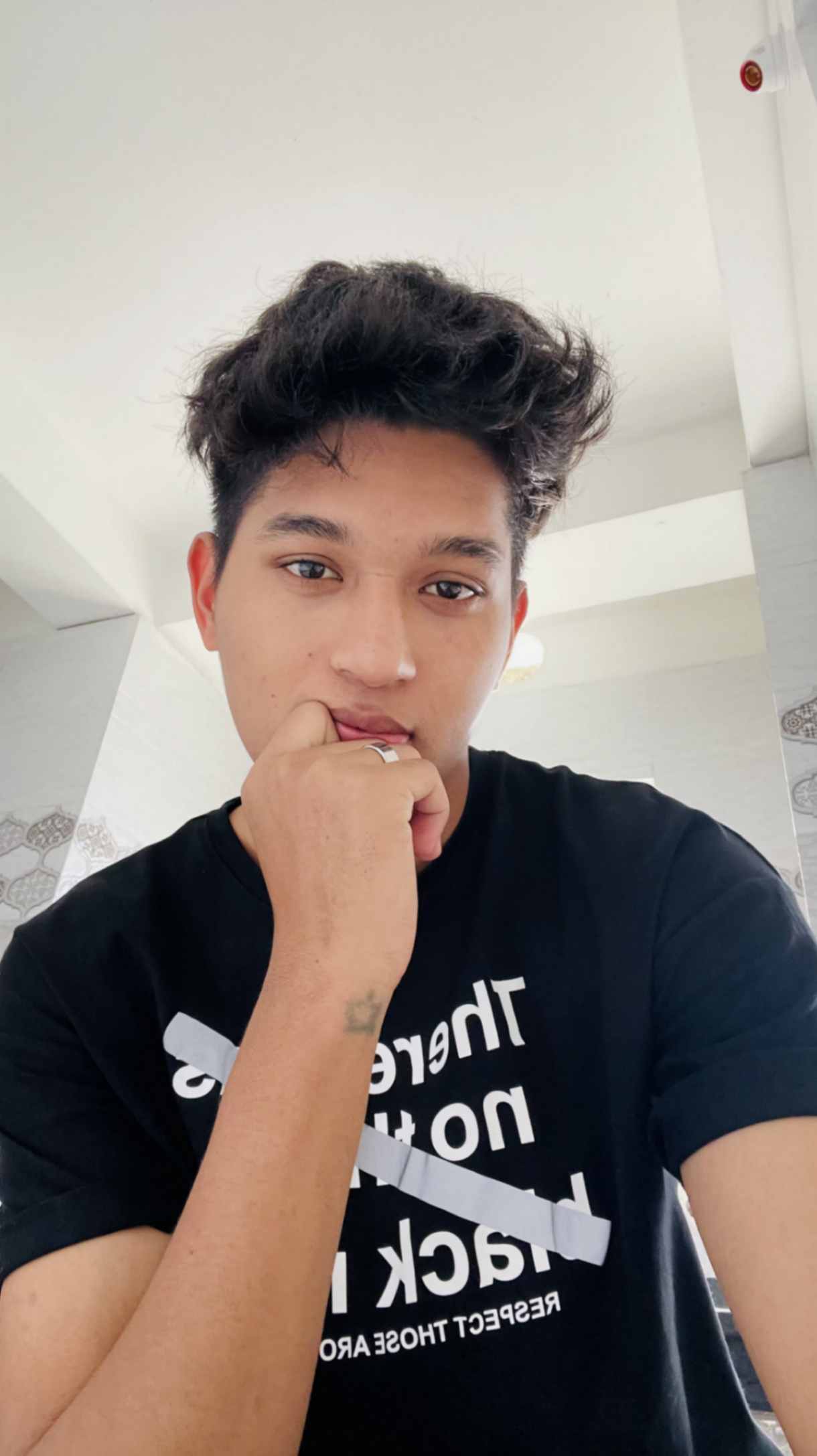
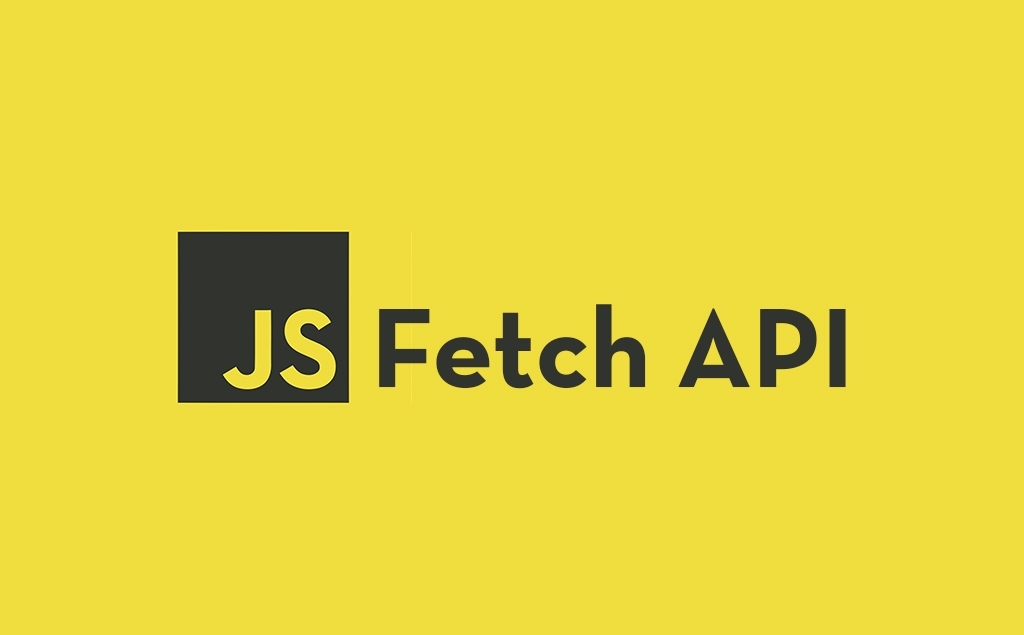
Bad API Fetch
No error handling
No timeout for long requests
Poor readability
No handling of response status codes
// Bad API fetch example
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log('Data:', data);
});
Issues with this code:
No error handling: If the network request fails, there is no way to catch and manage the error.
No status code handling: Even if the API responds, it might not be a
200
OK response (like404
or500
), but there's no way to handle that.No timeout mechanism: The request may hang indefinitely if there are connectivity issues.
Readability: If there are multiple
.then()
chains, the code can quickly become difficult to follow.
Good API Fetch
Error handling for network and status issues
Timeout mechanism for long requests
Improved readability using
async/await
// Good API fetch example with proper handling
async function fetchData(url) {
const controller = new AbortController();
const timeoutId = setTimeout(() => controller.abort(), 5000); // Set timeout of 5 seconds
try {
const response = await fetch(url, { signal: controller.signal });
clearTimeout(timeoutId);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log('Data:', data);
return data;
} catch (error) {
if (error.name === 'AbortError') {
console.error('Fetch aborted due to timeout');
} else {
console.error('Fetch error:', error.message);
}
}
}
// Usage
fetchData('https://api.example.com/data');
Improvements in this code:
Error handling: Catches network errors and logs them.
Status code handling: Checks for
response.ok
to ensure the request was successful, otherwise throws an error.Timeout mechanism: Uses
AbortController
to abort the request after 5 seconds if it takes too long.Readability: Using
async/await
makes the code more readable and easier to maintain.
Subscribe to my newsletter
Read articles from Sandesh Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
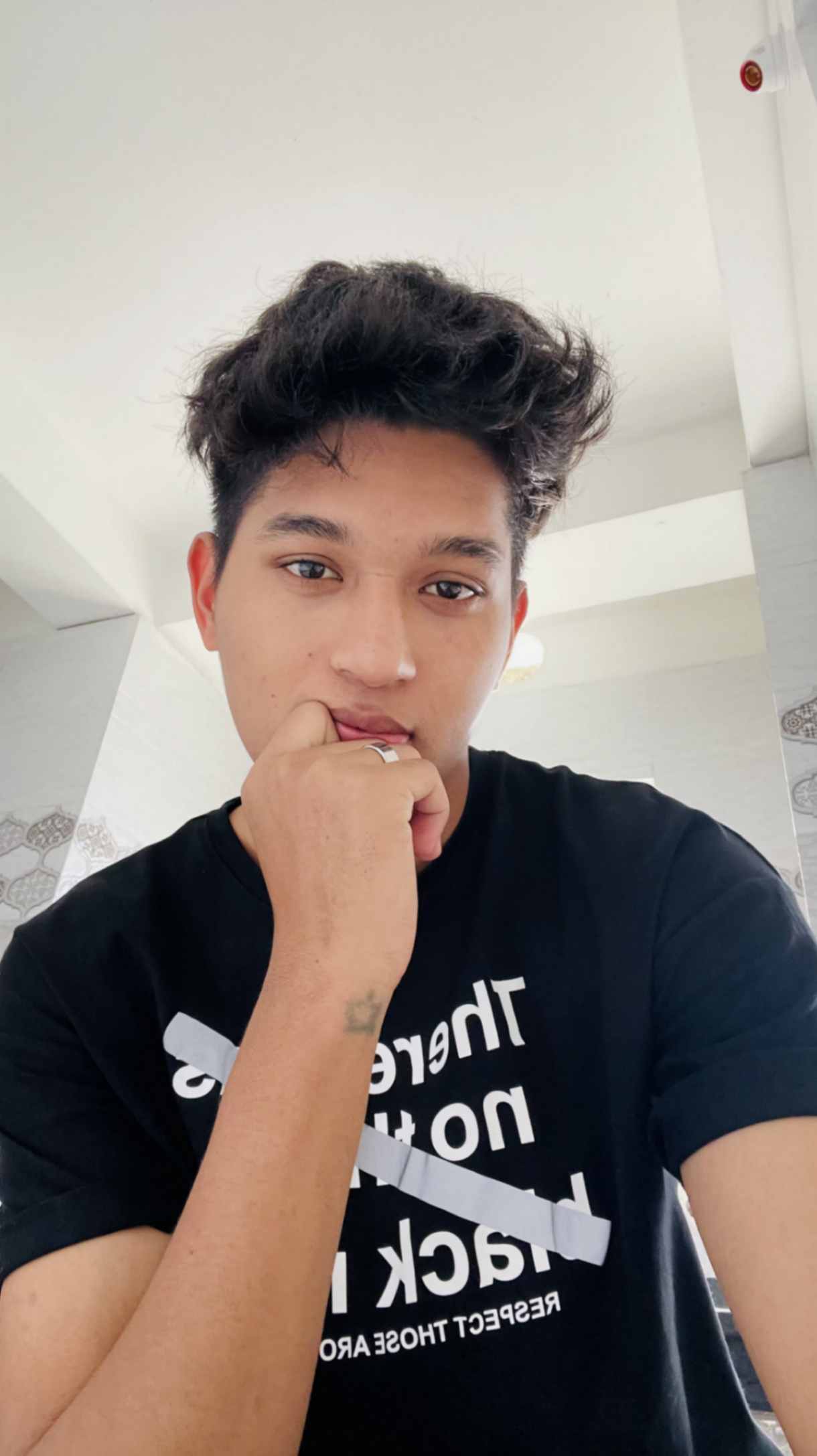
Sandesh Shrestha
Sandesh Shrestha
I'm Sandesh Shrestha, a passionate developer from India. Primarily interested in full-stack development and always exploring new technologies. Fascinated by science, space, technology, and ancient history. Love playing video games sometimes 🎮