Maximizing QuickNode for Solana Development: An African Developer's Guide
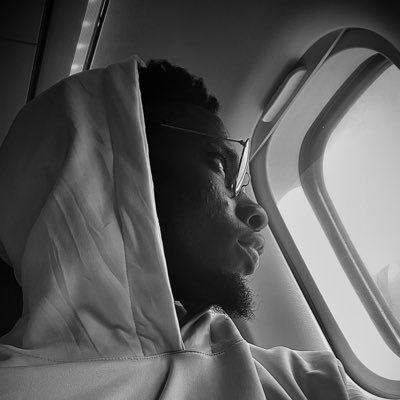
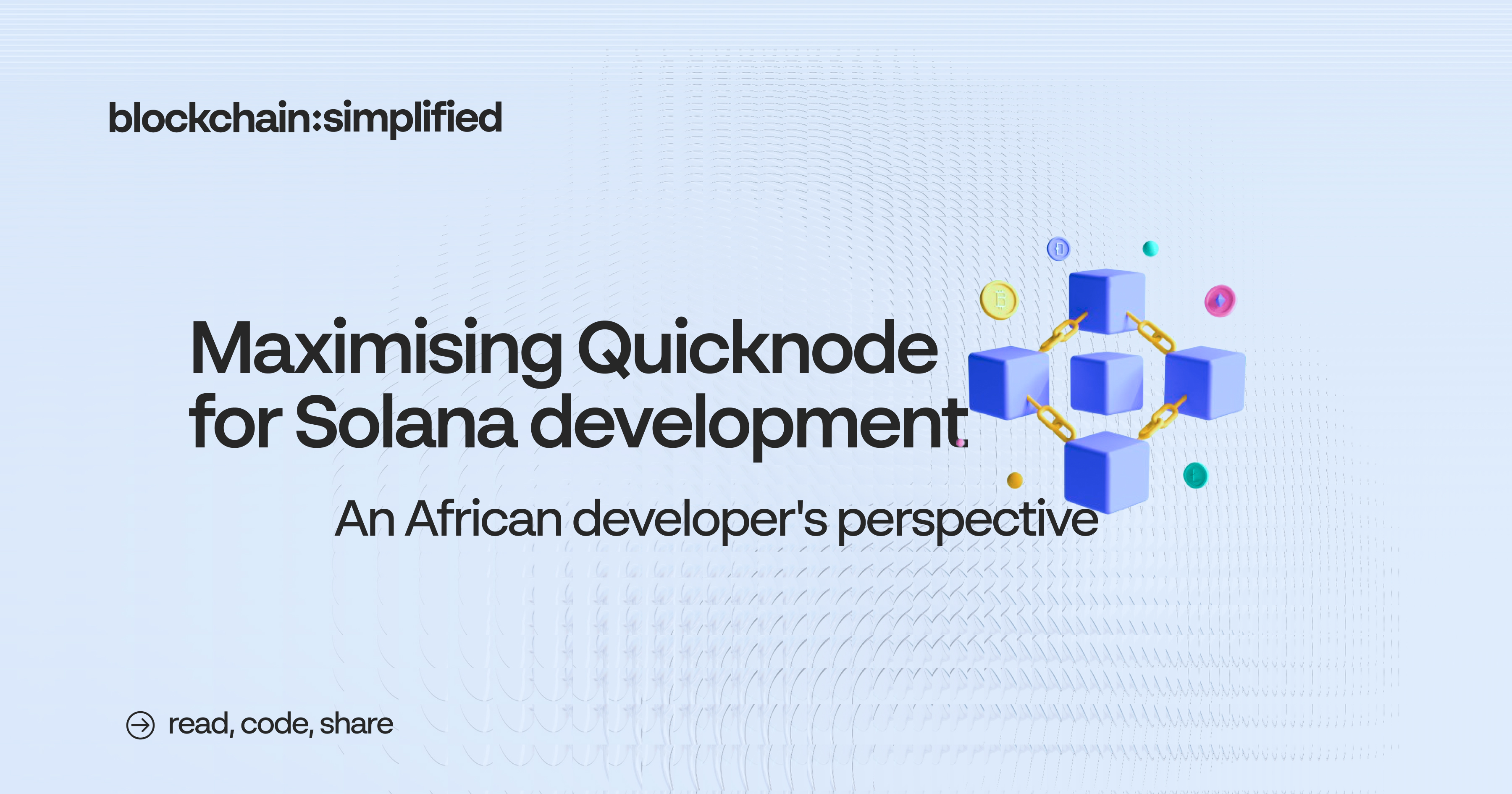
Table of Contents
Maximizing QuickNode Features 5.1 High-Performance RPC Endpoints 5.2 Add-ons and Analytics 5.3 WebSockets for Real-Time Data 5.4 NFT API Integration
1. Introduction
As an African developer venturing into the world of Solana blockchain development, you're part of a growing community that's shaping the future of decentralized finance and applications on the continent. This guide will help you maximize the use of QuickNode, a powerful blockchain infrastructure provider, to overcome unique challenges and build innovative Solana applications tailored to African needs.
2. Understanding QuickNode and Its Importance
QuickNode provides high-performance blockchain infrastructure, offering developers reliable and fast access to blockchain networks, including Solana. For African developers, QuickNode can be a game-changer, providing:
Reliable and fast access to the Solana network, crucial in regions with inconsistent internet connectivity.
Scalable infrastructure that can grow with your project, from a small startup to a continent-wide application.
Advanced features and APIs that can help build sophisticated applications addressing African-specific use cases.
3. Setting Up QuickNode for Solana
To get started with QuickNode for Solana development:
Sign up for a QuickNode account at https://www.quicknode.com.
Create a new Solana endpoint:
Choose Solana from the list of supported chains.
Select your preferred network (Mainnet, Testnet, or Devnet).
Choose a plan that fits your needs and budget.
Once your endpoint is created, you'll receive an HTTP and WSS URL. Keep these secure, as you'll use them to connect your application to the Solana network.
4. Overcoming African-Specific Challenges
African developers often face unique challenges. Here's how QuickNode can help address some of these:
Unreliable Internet Connectivity: QuickNode's globally distributed infrastructure can provide more stable connections compared to public nodes. Implement robust error handling and retry mechanisms in your code:
const { Connection, PublicKey } = require('@solana/web3.js'); const QuickNodeEndpoint = 'YOUR_QUICKNODE_URL'; async function getAccountInfo(publicKey) { const connection = new Connection(QuickNodeEndpoint); const maxRetries = 5; let retries = 0; while (retries < maxRetries) { try { const accountInfo = await connection.getAccountInfo(new PublicKey(publicKey)); return accountInfo; } catch (error) { console.log(`Attempt ${retries + 1} failed. Retrying...`); retries++; if (retries === maxRetries) { throw new Error('Max retries reached. Please check your internet connection.'); } // Wait for 2 seconds before retrying await new Promise(resolve => setTimeout(resolve, 2000)); } } }
Limited Access to Developer Resources: QuickNode provides extensive documentation and support. Utilize their learning resources, and join developer communities for knowledge sharing.
Power Outages: Implement state management and data persistence in your applications to handle unexpected disconnections:
const fs = require('fs'); function saveState(state) { fs.writeFileSync('app_state.json', JSON.stringify(state)); } function loadState() { if (fs.existsSync('app_state.json')) { return JSON.parse(fs.readFileSync('app_state.json', 'utf8')); } return null; } // Use these functions in your app to save and recover state
Limited Hardware Resources: Optimize your code for efficiency. Use QuickNode's powerful infrastructure to offload heavy computations:
// Instead of this (which may be heavy on local resources): const largeDataset = await processLargeDataLocally(); // Use QuickNode's RPC to offload computation: const largeDataset = await connection.getProgramAccounts( new PublicKey('YourProgramId'), { filters: [ { dataSize: 100 // Example filter }, { memcmp: { offset: 4, bytes: 'base58 encoded string' } } ] } );
5. Maximizing QuickNode Features
5.1 High-Performance RPC Endpoints
QuickNode's RPC endpoints offer superior performance. Here's how to make the most of them:
const { Connection, PublicKey } = require('@solana/web3.js');
const QuickNodeEndpoint = 'YOUR_QUICKNODE_URL';
const connection = new Connection(QuickNodeEndpoint);
async function getBalance(address) {
const publicKey = new PublicKey(address);
const balance = await connection.getBalance(publicKey);
console.log(`Balance: ${balance / 10**9} SOL`);
}
// Example usage
getBalance('AfzGtGEUkZtYq1s4xbgcteJ5bvLNsTeRJtE9qXzjUWW6');
5.2 Add-ons and Analytics
Leverage QuickNode's add-ons for enhanced functionality:
- Trace API: Useful for debugging transactions, especially in complex DeFi applications.
const traceResponse = await connection.sendRawTransaction(
rawTransaction,
{ maxRetries: 5, skipPreflight: true, preflightCommitment: 'confirmed' },
{ trace: true }
);
console.log('Transaction Trace:', traceResponse);
- Analytics: Monitor your dApp's performance and usage patterns.
Access analytics data through the QuickNode dashboard to optimize your application based on usage patterns specific to your African user base.
5.3 WebSockets for Real-Time Data
Implement WebSockets for real-time updates, crucial for applications like remittance services or market data:
const { Connection, PublicKey } = require('@solana/web3.js');
const QuickNodeWSSEndpoint = 'YOUR_QUICKNODE_WSS_URL';
const connection = new Connection(QuickNodeWSSEndpoint);
const programId = new PublicKey('YOUR_PROGRAM_ID');
connection.onProgramAccountChange(
programId,
(accountInfo, context) => {
console.log('Account changed:', accountInfo.accountId.toBase58());
console.log('New account data:', accountInfo.accountInfo.data);
},
'confirmed'
);
5.4 NFT API Integration
For projects involving digital art or tokenized assets (e.g., representing ownership of physical goods), use QuickNode's NFT API:
const axios = require('axios');
async function getNFTMetadata(mintAddress) {
const QuickNodeNFTAPI = 'https://YOUR_QUICKNODE_ENDPOINT/nft/v1/solana/nft/';
try {
const response = await axios.get(`${QuickNodeNFTAPI}${mintAddress}`);
return response.data;
} catch (error) {
console.error('Error fetching NFT metadata:', error);
}
}
// Example usage
getNFTMetadata('MINT_ADDRESS').then(metadata => console.log(metadata));
6. Building African-Centric Solana dApps with QuickNode
Let's explore how to build Solana dApps that address African needs using QuickNode:
6.1 Remittance dApp
Create a remittance service that leverages Solana's fast and low-cost transactions:
const { Connection, PublicKey, Transaction, SystemProgram } = require('@solana/web3.js');
const QuickNodeEndpoint = 'YOUR_QUICKNODE_URL';
const connection = new Connection(QuickNodeEndpoint);
async function sendRemittance(senderKeypair, recipientAddress, amount) {
const transaction = new Transaction().add(
SystemProgram.transfer({
fromPubkey: senderKeypair.publicKey,
toPubkey: new PublicKey(recipientAddress),
lamports: amount * 10**9 // Convert SOL to lamports
})
);
const signature = await connection.sendTransaction(transaction, [senderKeypair]);
await connection.confirmTransaction(signature);
console.log('Remittance sent successfully:', signature);
}
6.2 Agricultural Supply Chain Tracking
Develop a dApp to track agricultural products from farm to market:
const { Connection, PublicKey, Transaction } = require('@solana/web3.js');
const BufferLayout = require('buffer-layout');
const QuickNodeEndpoint = 'YOUR_QUICKNODE_URL';
const connection = new Connection(QuickNodeEndpoint);
const PRODUCT_ACCOUNT_DATA_LAYOUT = BufferLayout.struct([
BufferLayout.u8('isInitialized'),
BufferLayout.blob(32, 'productId'),
BufferLayout.u8('stage'), // 0: Farm, 1: Processing, 2: Distribution, 3: Retail
BufferLayout.blob(64, 'metadata')
]);
async function updateProductStage(productAccount, newStage, updateAuthority) {
const productInfo = await connection.getAccountInfo(new PublicKey(productAccount));
const productData = PRODUCT_ACCOUNT_DATA_LAYOUT.decode(productInfo.data);
productData.stage = newStage;
const instruction = new Transaction().add({
keys: [{ pubkey: new PublicKey(productAccount), isSigner: false, isWritable: true }],
programId: new PublicKey('YOUR_PROGRAM_ID'),
data: Buffer.from([...PRODUCT_ACCOUNT_DATA_LAYOUT.encode(productData)])
});
const signature = await connection.sendTransaction(instruction, [updateAuthority]);
await connection.confirmTransaction(signature);
console.log('Product stage updated successfully:', signature);
}
7. Best Practices and Optimization Tips
Implement Caching: Cache frequently accessed data to reduce RPC calls and improve performance:
const NodeCache = require('node-cache'); const cache = new NodeCache({ stdTTL: 600 }); // Cache for 10 minutes async function getCachedAccountInfo(publicKey) { const cacheKey = `accountInfo_${publicKey}`; let accountInfo = cache.get(cacheKey); if (!accountInfo) { accountInfo = await connection.getAccountInfo(new PublicKey(publicKey)); cache.set(cacheKey, accountInfo); } return accountInfo; }
Batch Requests: Group multiple RPC requests to reduce network overhead:
const publicKeys = [ 'PublicKey1', 'PublicKey2', 'PublicKey3' ].map(key => new PublicKey(key)); const accountInfos = await connection.getMultipleAccountsInfo(publicKeys);
Error Handling and Logging: Implement robust error handling and logging for better debugging, especially important in environments with unstable internet:
const winston = require('winston'); const logger = winston.createLogger({ level: 'info', format: winston.format.json(), transports: [ new winston.transports.File({ filename: 'error.log', level: 'error' }), new winston.transports.File({ filename: 'combined.log' }) ] }); async function safeGetBalance(address) { try { const balance = await connection.getBalance(new PublicKey(address)); return balance; } catch (error) { logger.error('Error fetching balance', { address, error: error.message }); throw error; } }
Optimize for Mobile: Given the prevalence of mobile internet in Africa, optimize your dApps for mobile devices:
// Use responsive design in your frontend // Minimize data transfer // Implement offline capabilities where possible
Regular Monitoring: Utilize QuickNode's monitoring tools to keep track of your application's performance and resource usage.
8. Conclusion
By leveraging QuickNode's powerful infrastructure and following these best practices, African developers can build robust, efficient, and scalable Solana applications that address unique continental challenges. From improving financial inclusion through remittance dApps to enhancing agricultural supply chains, the possibilities are vast.
Remember to stay engaged with both the African and global Solana developer communities. Share your experiences, contribute to open-source projects, and continue pushing the boundaries of what's possible with blockchain technology in Africa.
As you embark on your Solana development journey with QuickNode, you're not just building applications; you're shaping the future of blockchain technology in Africa. Embrace the challenges, leverage the tools at your disposal, and create solutions that will drive the continent forward in the decentralized era.
Subscribe to my newsletter
Read articles from Udoka, AM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
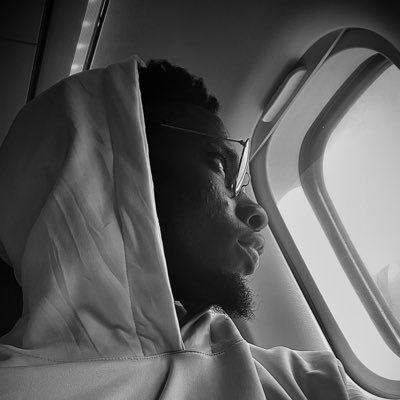
Udoka, AM
Udoka, AM
I'm a blockchain developer and developer relations engineer interested in growing the developer ecosystem and build amazing products with frontier technologies in the african community.