SCSS: A Complete Guide in Bangla (বাংলা) and English (With Examples)
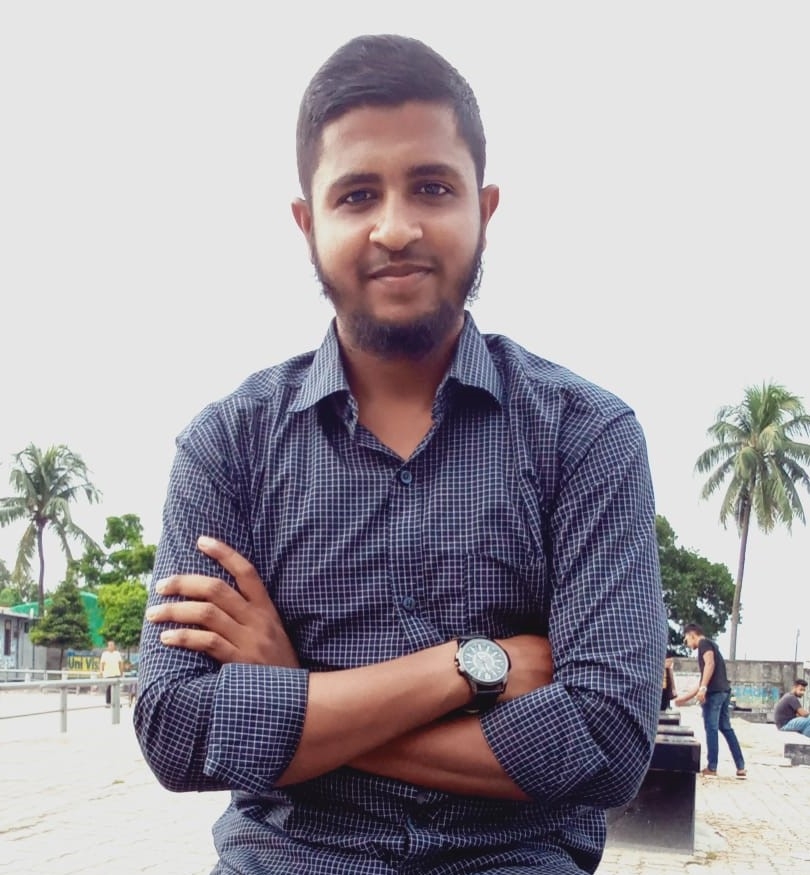
Table of contents
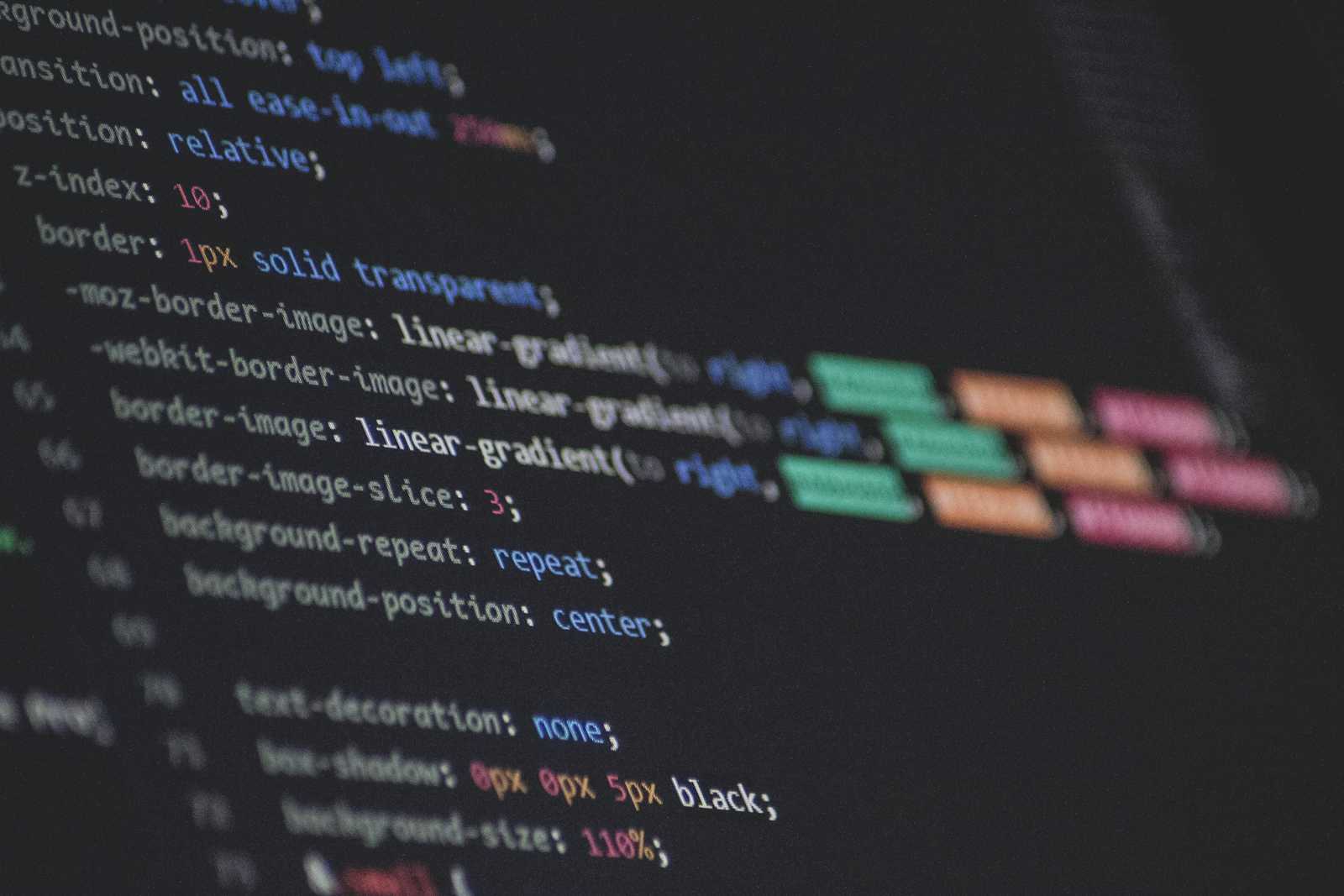
বাংলায় পড়তে স্ক্রল করে নিচে নামুন।
This guide will walk you through the basics of SCSS (Sassy CSS) to more advanced techniques, helping you understand how to write clean, organized, and scalable CSS using SCSS. Whether you're a beginner or looking to improve your skills, this series will provide real-world examples to make learning SCSS easy and practical.
1. What is SCSS and Why Use It?
What is SCSS?
- SCSS is a CSS preprocessor, meaning it extends the capabilities of CSS by adding features like variables, functions, and logic. It helps you write maintainable, modular, and reusable stylesheets.
Why Use SCSS?
Variables: Store values like colors and fonts and reuse them across your stylesheet.
Nesting: Write CSS in a way that matches the structure of your HTML.
Mixins: Create reusable blocks of code.
Inheritance: Share styles across multiple selectors.
Setting Up SCSS
To start using SCSS, you need to set it up in your development environment. You can install SCSS using npm
or yarn
:
npm install -g sass
Once installed, compile your SCSS files into CSS using the following command:
sass input.scss output.css
2. Using Variables in SCSS
What Are Variables?
In CSS, you often reuse the same values like colors or font sizes. SCSS allows you to store these values in variables, making it easier to maintain and update your stylesheets.
Declaring and Using Variables
// Declaring Variables
$primary-color: #3498db;
$secondary-color: #2ecc71;
$font-stack: 'Roboto', sans-serif;
// Using Variables
body {
font-family: $font-stack;
background-color: $primary-color;
color: $secondary-color;
}
Output: The variables store the colors and font so that you can use them throughout your styles. If you ever need to update the color, you only need to change it in one place.
3. Nesting in SCSS
What is Nesting?
Nesting in SCSS allows you to structure your CSS selectors in a way that mirrors the structure of your HTML. It helps make the code cleaner and easier to read, especially for complex layouts.
Nesting Example
Let's say you have the following HTML structure:
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
</ul>
</nav>
With SCSS, you can write the styles like this:
nav {
ul {
list-style: none;
padding: 0;
li {
display: inline-block;
margin-right: 10px;
a {
text-decoration: none;
&:hover {
color: red; // Nested pseudo-class
}
}
}
}
}
Output: SCSS nesting makes your stylesheets more organized and easier to maintain, avoiding long and repetitive selectors.
4. Mixins in SCSS
What is a Mixin?
A mixin is a block of reusable code in SCSS. It allows you to create a set of styles that you can reuse throughout your stylesheet.
Creating and Using a Mixin
// Define a Mixin
@mixin border-radius($radius) {
border-radius: $radius;
}
// Use the Mixin
.button {
@include border-radius(10px);
}
Output: In this example, the mixin border-radius
is defined with a parameter $radius
. You can then include the mixin wherever you need to apply a border-radius
, keeping your code DRY (Don't Repeat Yourself).
5. Inheritance with @extend
What is Inheritance?
SCSS allows you to use the @extend
directive to share styles between selectors. This reduces redundancy and helps keep your stylesheet more modular.
Inheritance Example
%button-base {
padding: 10px 20px;
border-radius: 5px;
font-weight: bold;
}
.button-primary {
@extend %button-base;
background-color: #3498db;
}
.button-secondary {
@extend %button-base;
background-color: #2ecc71;
}
Output: Here, the %button-base
placeholder stores common styles for buttons. Both .button-primary
and .button-secondary
extend this base and apply their own background color, reducing the need to write duplicate code.
6. Partials and Importing
What is a Partial?
Partials are small SCSS files that you can split your code into, keeping your project organized and manageable, especially in large-scale applications. A partial SCSS file starts with an underscore (e.g., _variables.scss
).
Using Partials and Importing Them
For example, you could have a partial _variables.scss
for storing all your variables:
// _variables.scss
$primary-color: #3498db;
$secondary-color: #2ecc71;
In your main SCSS file, import the partial like this:
// style.scss
@import 'variables';
body {
background-color: $primary-color;
}
Output: Partials help you break down your styles into manageable sections, making your stylesheets more modular and easy to maintain.
Conclusion
In this SCSS series, we covered everything from the basics like variables and nesting to more advanced features like mixins and inheritance. SCSS makes writing CSS cleaner, reusable, and scalable. By mastering SCSS, you'll be able to maintain and scale even large web projects with ease.
For beginners, SCSS is a powerful tool that can significantly improve your workflow, making CSS more maintainable and efficient.
Special Note*: This blog post was written using AI.*
বাংলায় পড়তে এখানে ক্লিক করুন
এই সিরিজের মাধ্যমে আপনি SCSS (Sassy CSS)-এর বেসিক বিষয়গুলি থেকে শুরু করে আরও কিছু শিখবেন। SCSS হলো CSS-এর একটি এক্সটেনশন যা স্টাইলশিট লেখা সহজতর, সংগঠিত, এবং ব্যবস্থাপনাযোগ্য করে তোলে। এই সিরিজ শেষে আপনি বড় প্রজেক্ট এ SCSS সহজেই ব্যবহার করতে সক্ষম হবেন।
১। SCSS শুরু করার উপায়
SCSS কী?
SCSS (Sassy CSS) হলো একটি প্রিপ্রসেসর স্ক্রিপ্টিং ভাষা যা CSS-কে আরও ক্ষমতাশালী করে এবং এতে ভেরিয়েবল, নেস্টিং, মিক্সিনস, এবং ইনহেরিটেন্সের মতো বৈশিষ্ট্য যোগ করে। SCSS-এর মাধ্যমে আপনি আরও পরিষ্কার, মডিউলার এবং ব্যবস্থাপনাযোগ্য স্টাইল লিখতে পারেন, যা সাধারণ CSS-এ সম্ভব হয় না।
SCSS কেন ব্যবহার করবেন?
CSS খুব শক্তিশালী হলেও, প্রকল্প বড় হলে সেটি পরিচালনা করা কঠিন হয়ে পড়ে। SCSS এর সীমাবদ্ধতা দূর করে নিচের সুবিধাগুলি প্রদান করে:
ভেরিয়েবলস: SCSS-এ আপনি মানগুলি সংরক্ষণ করতে এবং পুনরায় ব্যবহার করতে পারেন, যেমন রং, ফন্ট-সাইজ এবং অন্যান্য স্টাইল।
নেস্টিং: আপনি আপনার CSS সিলেক্টরগুলিকে HTML কাঠামোর সাথে মেলাতে পারেন, যা কোডটি সহজবোধ্য করে তোলে।
মিক্সিনস এবং ইনহেরিটেন্স: SCSS-এ পুনর্ব্যবহারযোগ্য কোড ব্লক তৈরি করা এবং বৈশিষ্ট্য উত্তরাধিকার নেওয়া সম্ভব।
ফাংশন এবং লজিক: SCSS-এ আপনি লজিক এবং গণনা করতে পারেন, যা রেসপন্সিভ ডিজাইন এবং জটিল লেআউট পরিচালনা করা সহজ করে তোলে।
SCSS সেটআপ করার উপায়
আপনার ডেভেলপমেন্ট পরিবেশে SCSS সেটআপ করার জন্য কিছু ধাপ অনুসরণ করতে হবে:
Node.js-এর মাধ্যমে SCSS ইনস্টল করা: SCSS ইনস্টল করা যায়
npm
বাyarn
এর মাধ্যমে:npm install -g sass
অথবা:
yarn global add sass
SCSS-কে CSS-এ কম্পাইল করা: SCSS ইনস্টল করার পর, আপনি নিচের কমান্ড ব্যবহার করে SCSS ফাইলগুলো CSS-এ কম্পাইল করতে পারবেন:
sass input.scss output.css
SCSS ফাইল যুক্ত করা: আপনার HTML প্রোজেক্টে SCSS ফাইল যুক্ত করতে:
<link rel="stylesheet" href="css/style.css">
২।SCSS-এ ভেরিয়েবলস ব্যবহার
SCSS-এ ভেরিয়েবলসের মাধ্যমে আপনি মানগুলি সংরক্ষণ করতে পারেন যা পরবর্তীতে বিভিন্ন স্থানে পুনরায় ব্যবহার করা যায়। এটি বিশেষত বৃহৎ প্রকল্পে কনসিস্টেন্সি বজায় রাখতে এবং কোড আপডেট সহজ করতে সহায়তা করে।
ভেরিয়েবল ডিক্লারেশন এবং ব্যবহার
SCSS-এ ভেরিয়েবল $
সাইন দিয়ে ডিক্লেয়ার করা হয়:
// ভেরিয়েবল ডিক্লারেশন
$primary-color: #3498db;
$secondary-color: #2ecc71;
$font-stack: 'Roboto', sans-serif;
// ভেরিয়েবল ব্যবহার
body {
font-family: $font-stack;
background-color: $primary-color;
color: $secondary-color;
}
গ্লোবাল এবং লোকাল ভেরিয়েবলস
ভেরিয়েবলস গ্লোবাল অথবা লোকালভাবে ব্যবহার করা যায়। গ্লোবাল ভেরিয়েবলস সব জায়গায় ব্যবহারযোগ্য আর লোকাল ভেরিয়েবলস কেবল নির্দিষ্ট ব্লকের মধ্যে ব্যবহার করা যায়।
৩। SCSS-এ নেস্টিং
SCSS-এর নেস্টিং সুবিধা CSS সিলেক্টরগুলিকে HTML কাঠামোর মতো করে গঠন করতে দেয়, যা কোডটি সহজে পড়া এবং বোঝা যায়।
নেস্টিং উদাহরণ
<nav>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
</ul>
</nav>
nav {
ul {
list-style: none;
padding: 0;
li {
display: inline-block;
margin-right: 10px;
a {
text-decoration: none;
&:hover {
color: red; // নেস্টেড সুডো-ক্লাস
}
}
}
}
}
নেস্টিং মিডিয়া কুয়েরি
SCSS-এ মিডিয়া কুয়েরি নেস্টিং করে রেসপন্সিভ স্টাইলগুলি সঠিকভাবে সংগঠিত রাখা সহজ।
.button {
background-color: #3498db;
@media (max-width: 600px) {
background-color: #2ecc71;
}
}
৪। পার্টিয়ালস এবং ইম্পোর্টিং
SCSS আপনাকে আপনার কোডকে ছোট ছোট ফাইলে ভাগ করার সুযোগ দেয়, যাকে পার্টিয়াল বলে। এটি আপনাকে বড় প্রকল্পে স্টাইলগুলিকে আরও সংগঠিতভাবে ব্যবস্থাপনা করতে সহায়তা করে।
পার্টিয়াল তৈরি করা
SCSS ফাইলের নামের আগে _
ব্যবহার করলে তা পার্টিয়াল হয়ে যায়। যেমন:
_variables.scss
_mixins.scss
@import
ব্যবহার করে পার্টিয়াল ইম্পোর্ট করা
@import 'variables';
@import 'mixins';
@import 'header';
৫।মিক্সিং (mixin)
Mixin হলো SCSS-এর এক বিশেষ সুবিধা যা পুনর্ব্যবহারযোগ্য কোড ব্লক তৈরি করতে দেয়। মিক্সিনের মাধ্যমে আপনি কোডের একটি ব্লক বিভিন্ন স্থানে ব্যবহার করতে পারেন।
@mixin border-radius($radius) {
border-radius: $radius;
}
.button {
@include border-radius(10px);
}
৩। এক্সটেন্ড/ইনহেরিটেন্স
@extend
নির্দেশ ব্যবহার করে আপনি বিভিন্ন সিলেক্টরের মধ্যে স্টাইল শেয়ার করতে পারেন।
%button-base {
padding: 10px 20px;
border-radius: 5px;
font-weight: bold;
}
.button-primary {
@extend %button-base;
background-color: #3498db;
}
উপসংহার
এই সিরিজে আমরা SCSS-এর মূল বিষয় থেকে শুরু করে ভেরিয়েবলস, নেস্টিং, মিক্সিনস এবং ইনহেরিটেন্সের মতো অ্যাডভান্সড ফিচার কভার করেছি। SCSS ব্যবহার করে আপনি আপনার CSS কোডকে আরও ক্লিন, রিইউজেবল, এবং স্কেলেবল করতে পারবেন।
Unlock the power of SCSS to write cleaner, organized, and scalable CSS. This guide covers everything from setting up SCSS to using its advanced features like variables, nesting, mixins, inheritance, partials, and more. Whether you're a beginner or looking to refine your skills, this series offers practical examples and tips to enhance your styling workflow. Start mastering SCSS today to make your CSS more maintainable and efficient. Read on to learn how SCSS can transform your web development process.
বিশেষ দ্রষ্টব্য: এই ব্লগ টি AI ব্যবহার করে লেখা হয়েছে।
Subscribe to my newsletter
Read articles from Noman Gazi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
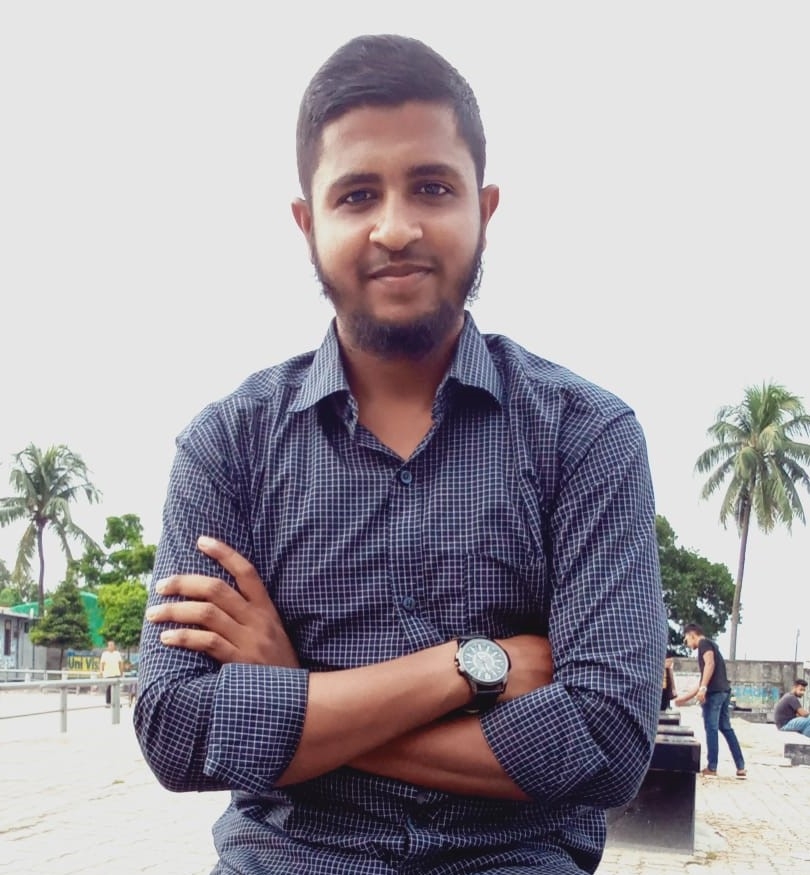