How to run Python Flask
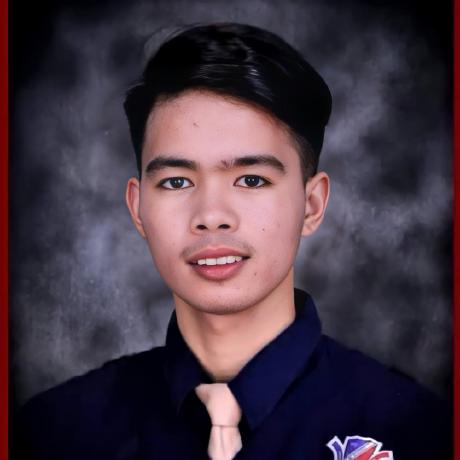
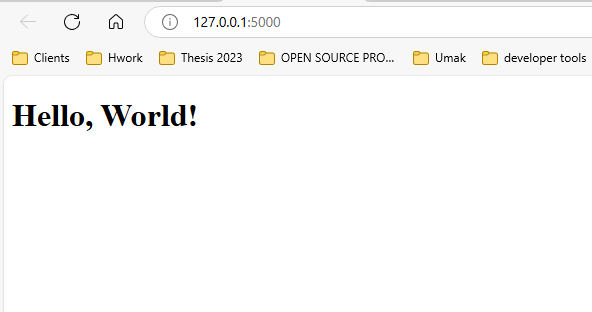
Flask is a lightweight, yet powerful web framework written in Python. It is designed to be easy to use and extend, making it a popular choice for web developers who want to build web applications quickly and efficiently. Flask provides the basic tools needed to get a web app running but gives developers the flexibility to add only the tools they need as their project grows.
Flask is ideal for small to medium-sized applications and provides useful features like routing, request handling, and template rendering. Its simplicity and modularity allow developers complete control over their applications while providing enough functionality to support complex web services.
This tutorial will teach you how to set up a Flask environment and run a basic Flask application.
Step 1: Create Folder
Step 2: Type CMD in the search bar:
Step 3: Check Python Version
Make sure to check your folder directory to ensure you are in your project folder. So in my case, I’m in the C:\Users\gayar\OneDrive\Desktop\python-list-flask
Step 4: Check Pip Version
Step 5: Create a Virtual Environment
A virtual environment in Python is a self-contained directory that contains a specific version of Python along with a set of installed packages. It helps developers manage dependencies for different projects, ensuring that the libraries and Python versions used in one project do not interfere with others.
The command python -m venv venv
creates a new virtual environment in a folder named "venv" inside your project directory. This environment will have its own Python interpreter and libraries.
Verify the venv folder to your project folder
Step 6: Activate Virtual Environment
venv\Scripts\activate
Once activated, the terminal prompt changes to show the name of the environment (in this case, (venv)
), indicating that you are now working within the virtual environment.
Step 7: Install Flask
pip install Flask
Step 8: add venv on gitignore
Adding the venv
directory to .gitignore
is essential to prevent the virtual environment files from being tracked by version control tools like Git. Since the venv
directory contains environment-specific files that are not necessary for the application’s source code, it is a good practice to exclude it from version control to keep the repository clean and reduce clutter.
Steps to add venv
to .gitignore
:
nul > .gitignore
Open the .gitignore
file with a text editor.
Add the following line:
venv/
Save the file.
By adding venv/
to the .gitignore
file, you ensure that the virtual environment folder is not included in the repository, keeping your Git history clean and only containing necessary code and dependencies.
Step 9: Open your Project in Pycharm
I recommend using PyCharm as your IDE (Integrated Development Environment) for Python projects. PyCharm is a powerful tool developed by JetBrains, designed specifically for Python development
Step 10: Create app.py
Step 11: Create Directory “templates”
Step 12: add index.html
in the templates folder
Step 13: In your app.py
, import Flask
and render_template
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def hello_world():
return render_template('index.html', message="Hello, World!")
if __name__ == '__main__':
app.run(debug=True)
Step 14: In your index.html
file, use the passed variable:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello Page</title>
</head>
<body>
<h1>{{ message }}</h1>
</body>
</html>
In this example, the string "Hello, World!"
is passed as a variable (message
) from the Python script to the HTML template. The template renders the variable inside the HTML content.
Step 14: Run app.py in cmd
python app.py
make sure the venv is activated
Access the Web Application:
- Open your browser and go to
http://127.0.0.1:5000/
to view your running application.
Github Repository
Subscribe to my newsletter
Read articles from Thirdy Gayares directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
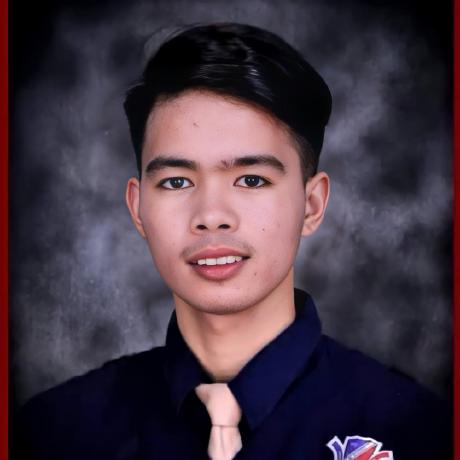
Thirdy Gayares
Thirdy Gayares
I am a dedicated and skilled Software Engineer specializing in mobile app development, backend systems, and creating secure APIs. With extensive experience in both SQL and NoSQL databases, I have a proven track record of delivering robust and scalable solutions. Key Expertise: Mobile App Development: I make high-quality apps for Android and iOS, ensuring they are easy to use and work well. Backend Development: Skilled in designing and implementing backend systems using various frameworks and languages to support web and mobile applications. Secure API Creation: Expertise in creating secure APIs, ensuring data integrity and protection across platforms. Database Management: Experienced with SQL databases such as MySQL, and NoSQL databases like Firebase, managing data effectively and efficiently. Technical Skills: Programming Languages: Java, Dart, Python, JavaScript, Kotlin, PHP Frameworks: Angular, CodeIgniter, Flutter, Flask, Django Database Systems: MySQL, Firebase Cloud Platforms: AWS, Google Cloud Console I love learning new things and taking on new challenges. I am always eager to work on projects that make a difference.