๐ก Handling API Requests in Flutter with HTTP and Dio ๐
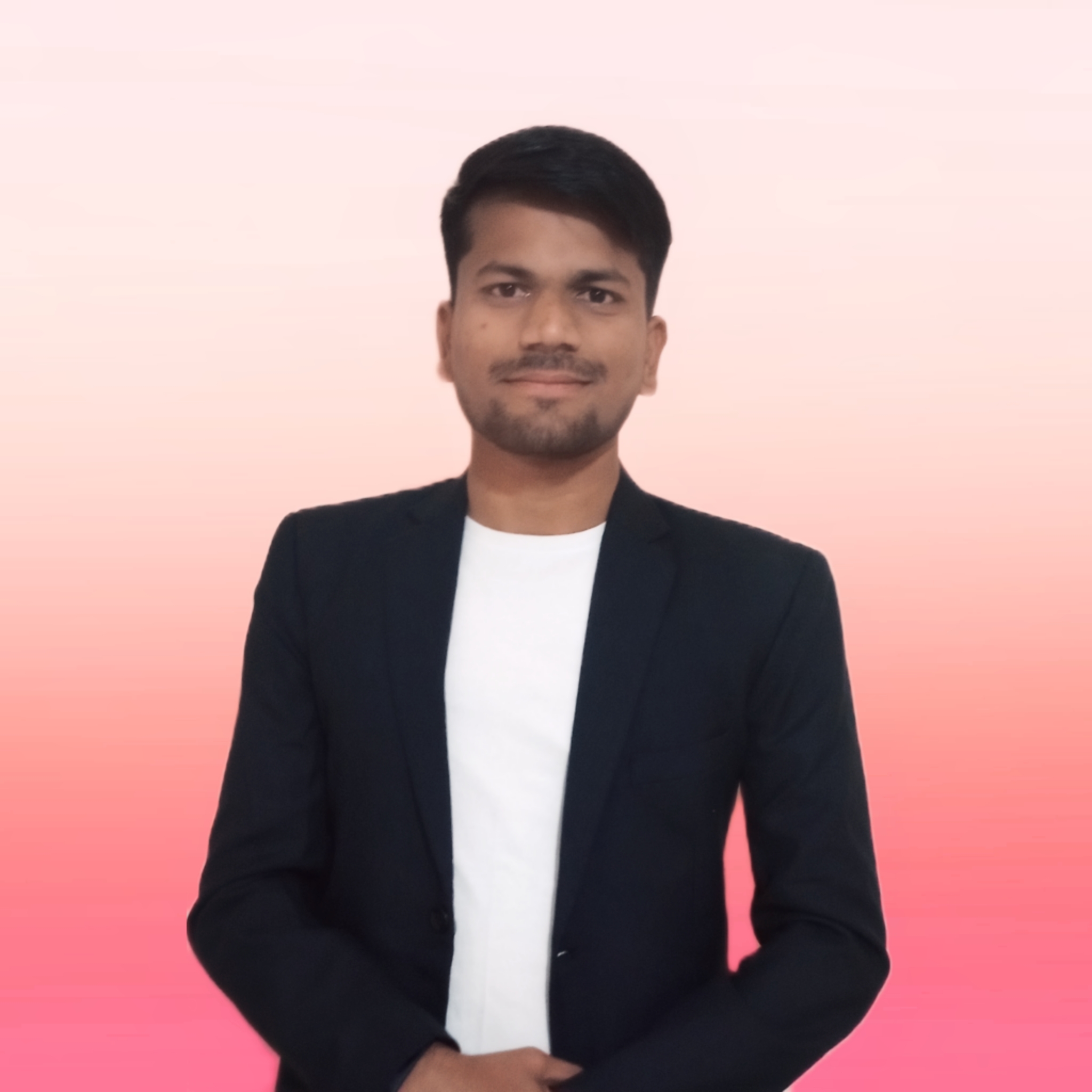
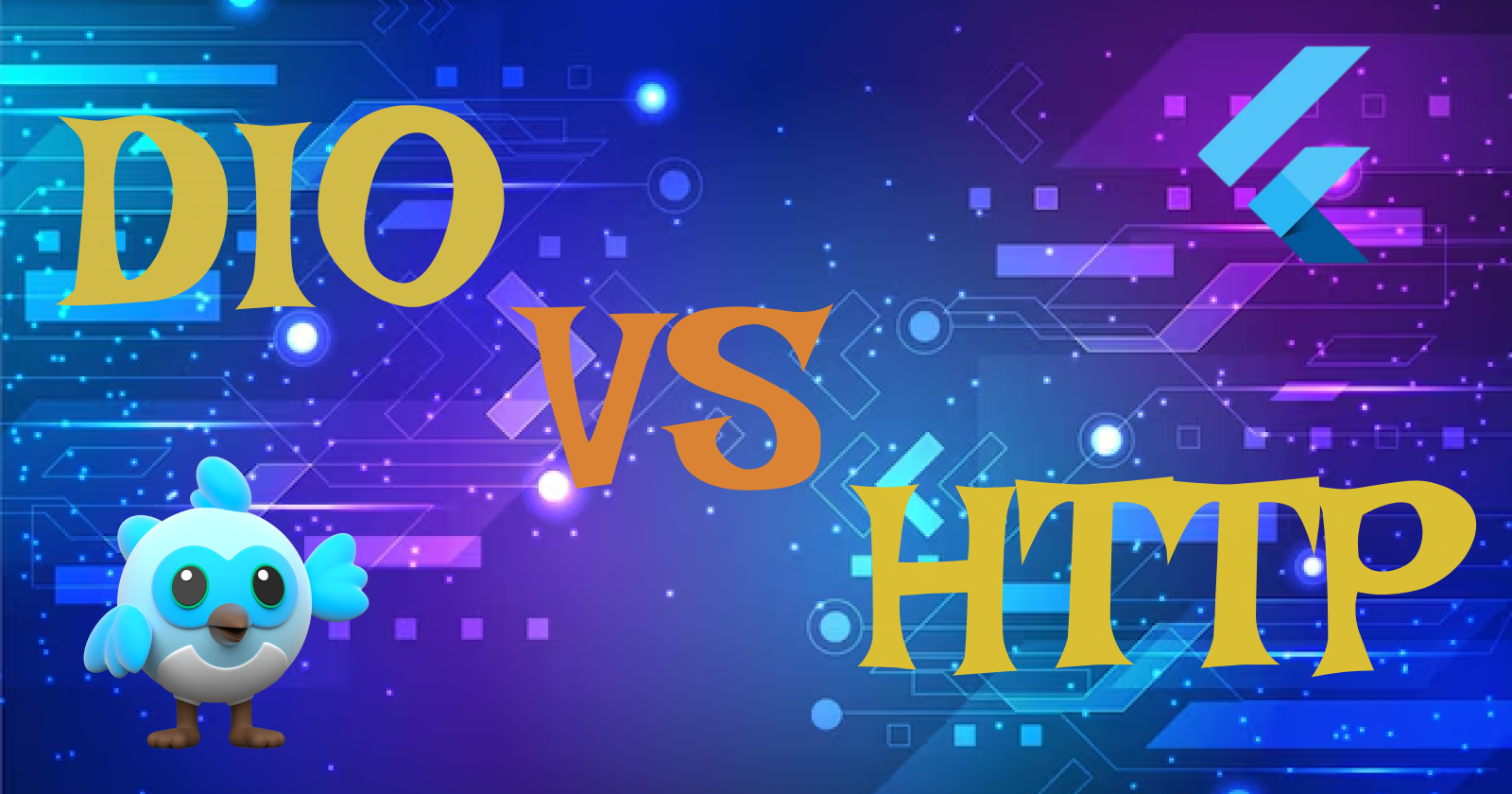
When building mobile applications, youโll often need to connect your app to a server to fetch or send data. In Flutter, handling API requests is made easy with libraries like HTTP
and Dio
. Both are powerful tools, but each has its own set of advantages depending on your needs.
In this article, we'll dive into how to handle API requests using these two popular packages.
๐ Why You Need API Requests
In many mobile apps, communicating with a server to retrieve data or send updates is essential. For example:
Fetching a list of products for an e-commerce app ๐.
Sending user information to a backend after login ๐.
Pulling real-time weather updates ๐ฆ๏ธ.
Flutter offers several tools to make working with APIs seamless. Let's explore two of the most common ones: HTTP
and Dio
.
1. ๐ ๏ธ Using the HTTP Package
The http
package is the simpler and more lightweight of the two. Itโs great for quick and easy API calls without complex configurations.
๐ฆ Installing the HTTP Package
To start, add the http
package to your pubspec.yaml
file:
dependencies:
http: ^0.14.0
Then, run:
flutter pub get
๐ Making a GET Request
Here's how to make a simple GET request using http
:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<void> fetchData() async {
final url = Uri.parse('https://jsonplaceholder.typicode.com/posts');
final response = await http.get(url);
if (response.statusCode == 200) {
var data = jsonDecode(response.body);
print(data); // Process the data here
} else {
print('Failed to load data');
}
}
๐ง Explanation:
We use
http.get()
to make a GET request to an API.The
response.statusCode == 200
check ensures the request was successful.jsonDecode()
converts the JSON response to a Dart object.
โฉ Making a POST Request
Sending data to the server is also straightforward:
Future<void> sendData() async {
final url = Uri.parse('https://jsonplaceholder.typicode.com/posts');
final response = await http.post(
url,
headers: {'Content-Type': 'application/json'},
body: jsonEncode({'title': 'Flutter', 'body': 'Building apps', 'userId': 1}),
);
if (response.statusCode == 201) {
print('Data sent successfully!');
} else {
print('Failed to send data');
}
}
Pros of HTTP
:
Simple and lightweight ๐ชถ.
Easy to use for basic requests like GET and POST.
Cons of HTTP
:
- Limited features: lacks advanced functionality like interceptors, retries, or download/upload progress ๐.
2. โก Using the Dio Package
If you need more advanced features, Dio
is your go-to package. It supports:
Interceptors for modifying requests/responses ๐.
Retry mechanisms ๐.
Progress tracking for file uploads/downloads โฌ๏ธ.
๐ฆ Installing Dio
Add dio
to your pubspec.yaml
:
dependencies:
dio: ^5.0.0
Run the following command:
flutter pub get
โ๏ธ Making a GET Request with Dio
Hereโs how you can use Dio for a GET request:
import 'package:dio/dio.dart';
Future<void> fetchDataWithDio() async {
Dio dio = Dio();
final response = await dio.get('https://jsonplaceholder.typicode.com/posts');
if (response.statusCode == 200) {
print(response.data); // Process the data here
} else {
print('Error: ${response.statusCode}');
}
}
๐ Making a POST Request with Dio
Hereโs how to send data to a server using Dio:
Future<void> sendDataWithDio() async {
Dio dio = Dio();
final response = await dio.post(
'https://jsonplaceholder.typicode.com/posts',
data: {'title': 'Dio', 'body': 'Advanced features', 'userId': 1},
);
if (response.statusCode == 201) {
print('Data sent successfully!');
} else {
print('Error: ${response.statusCode}');
}
}
๐ Handling Errors and Timeouts
Dio makes it easier to handle errors and timeouts. Hereโs an example:
try {
final response = await dio.get('https://jsonplaceholder.typicode.com/posts');
} on DioError catch (e) {
if (e.type == DioErrorType.connectTimeout) {
print('Connection timeout');
} else if (e.type == DioErrorType.response) {
print('Error: ${e.response?.statusCode}');
}
}
๐ Advanced Features of Dio:
Interceptors: Modify requests and responses on the fly.
Timeouts: Customizable connection and response timeouts โฑ๏ธ.
Download/Upload Progress: Track the progress of file uploads or downloads ๐.
Pros of Dio
:
Full-featured, supports advanced use cases ๐๏ธโโ๏ธ.
Easier error handling, request/response manipulation.
Cons of Dio
:
- Slightly heavier and more complex to set up compared to
http
.
โ๏ธ HTTP vs. Dio: Which One to Use?
Go with
HTTP
if youโre building a simple app with basic API interactions. Itโs lightweight and gets the job done for simple use cases.Choose
Dio
if you need more control over requests, error handling, or plan to handle large files (like images or PDFs). Itโs feature-rich and perfect for advanced scenarios.
๐ Conclusion
Handling API requests in Flutter is straightforward with both HTTP
and Dio
. While HTTP
is perfect for lightweight apps and simpler tasks, Dio
offers powerful features that make it ideal for more complex applications. Choose the one that fits your project's needs and start building powerful, connected apps! ๐๐ป
Happy coding! ๐
#flutterindore #flutter developer #bestflutterdevloper
Subscribe to my newsletter
Read articles from Manoj Jaiswal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
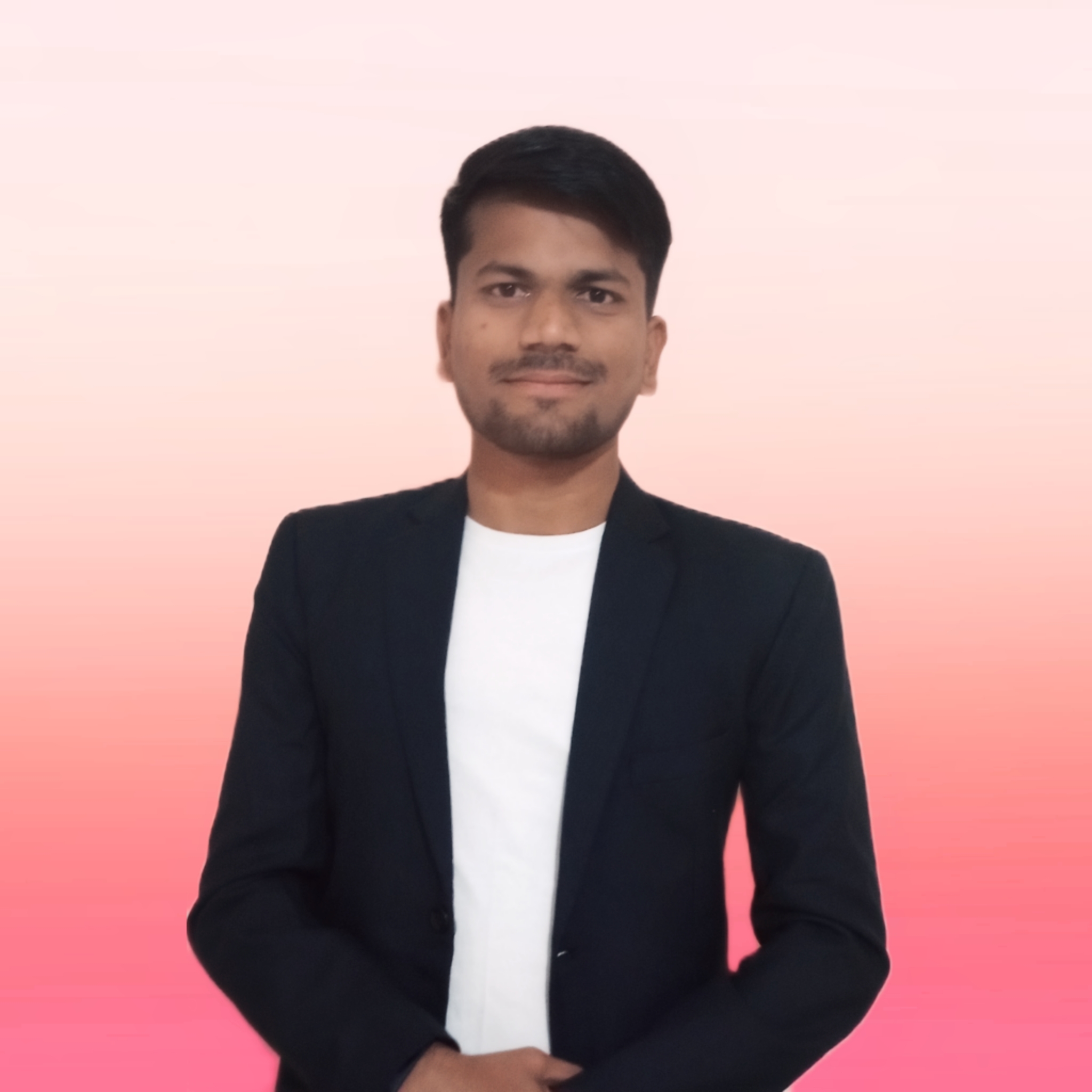
Manoj Jaiswal
Manoj Jaiswal
I am a Software Engineer with 3+ years of experience designing and developing mobile and web applications using flutter and python django. I specialization in flutter and have professional experience working with dart language. Also i have experience working with python django and node js.