Arrays and it's methods
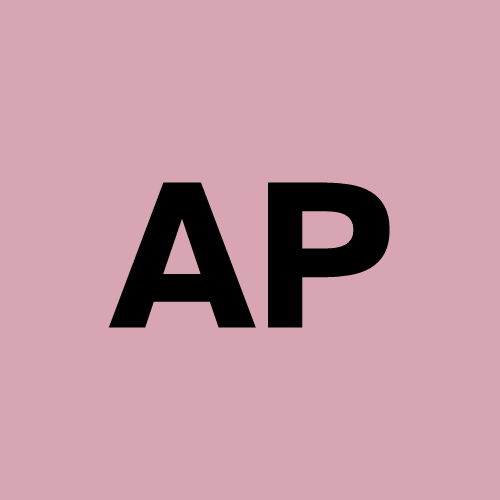
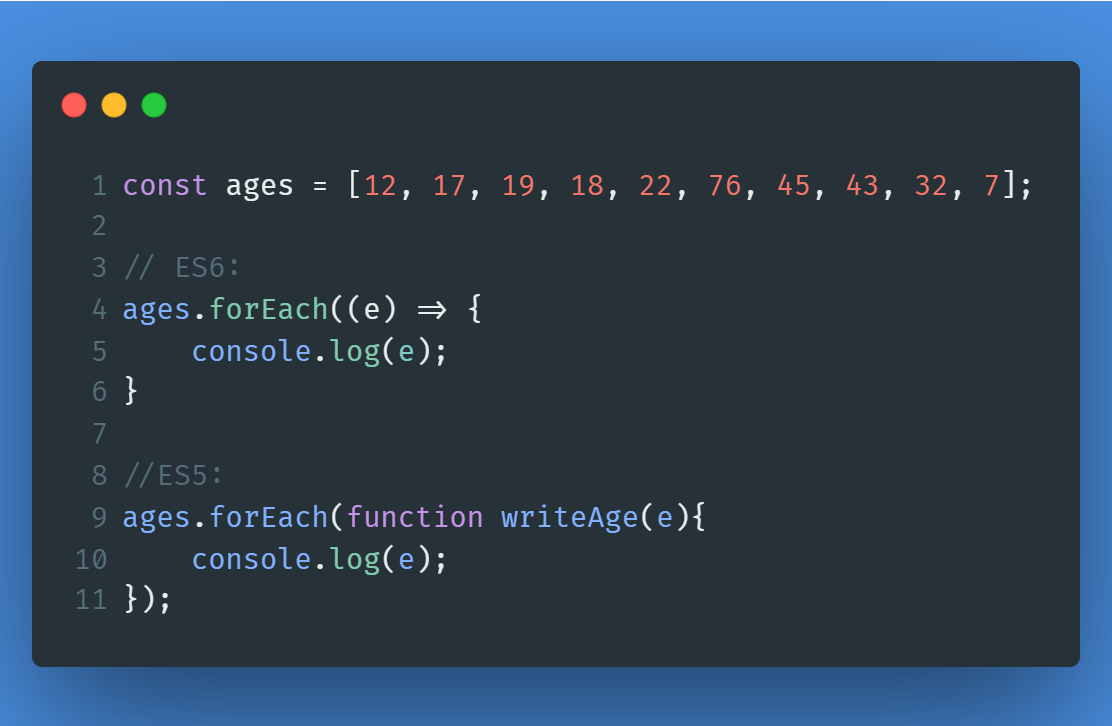
Arrays are a fundamental data structure in programming, enabling developers to store and manage collections of elements within a single variable. In this article, we’ll delve into the world of arrays, covering their definition, characteristics, types, and applications.
What is an Array?
An array is a data structure that holds a collection of elements, each identified by an index or key. Arrays store elements of the same data type, such as integers, strings, or objects, in contiguous memory locations. This makes arrays efficient for storing and accessing large amounts of data.
Characteristics of Arrays
Arrays possess several important characteristics:
Indexing: Each element in an array is identified by an index, starting from 0.
Fixed Size: Arrays have a fixed size, determined when they are declared.
Homogeneity: Typically, arrays store elements of the same data type.
Types of Arrays
There are various types of arrays, including:
One-Dimensional Arrays: These store elements in a single row or column.
Example: my_array = [1, 2, 3, 4, 5]Multi-Dimensional Arrays: These store elements in multiple rows and columns.
Example: my_2d_array = [[1, 2, 3], [4, 5, 6]]
Advantages of Arrays
Arrays provide several key advantages:
Efficient Memory Usage: Elements are stored in contiguous memory, optimizing storage.
Fast Access: Arrays allow direct access to elements via their index.
Simple Implementation: Arrays are straightforward to implement and use.
Disadvantages of Arrays
While useful, arrays have certain limitations:
Fixed Size: The size of an array cannot change once declared.
Homogeneity: They usually hold elements of the same data type, limiting flexibility.
Applications of Arrays
Arrays are widely used in various fields:
Database Management: Storing and retrieving structured data.
Image Processing: Representing and manipulating pixel values.
Game Development: Managing objects and game states.
Best Practices When Using Arrays
Initialize Properly: Always initialize arrays with the correct size.
Use Indexes Correctly: Be careful to avoid out-of-bounds errors.
Document Usage: Clearly document how arrays are used in your code.v
let toyBox = ["car", "teddy", "ball"];
In this example, the toyBox is holding three toys: a "car", a "teddy", and a "ball". Each of these is called an element.
Accessing Elements in an Array
Each element in an array is identified by its index. The first element is at index 0, the second element is at index 1, and so on. To access an element, you use bracket notation.
How Do You Pick a Toy from the Toy Box?
Every toy in your box has a special number called its index. But in JavaScript, we start counting from 0, not 1. So:
The "car" is at index 0,
The "teddy" is at index 1,
The "ball" is at index 2.
If you want to take out the teddy, you can say:
let myToy = toyBox[1]; // This will give us "teddy"
console.log(myToy); // It will print "teddy"
Array Methods
JavaScript provides a variety of methods to manipulate arrays. Here are some of the most commonly used array methods, along with explanations and examples:
1. push()
Definition: Adds one or more elements to the end of the array.
Example:
Adding More Toys to the Box (Using push
)
Now, what if you get a new toy and want to put it in the box? You can push it into the array, just like adding a new toy to your toy box.
toyBox.push("robot");
console.log(toyBox); // ["car", "teddy", "ball", "robot"]
2. pop()
Definition: Removes and returns the last element of the array.
Example: Taking the Last Toy Out (Using
pop
)
If you’re done playing with the last toy you put in the box, you can use pop to take it out.
let lastToy = toyBox.pop();
console.log(lastToy); // "robot"
console.log(toyBox); // ["car", "teddy", "ball"]
3. length
Definition: Returns the number of elements in the array.
- Example: Counting All the Toys (Using
length
)
Sometimes, you want to know how many toys are in the box. You can use the length method to count them!
let numberOfToys = toyBox.length;
console.log(numberOfToys); // 3
More Fun Array Methods:
4. Joining Toys Together (Using join
)
Let’s say you want to put all the toy names together in one long string. You can use join to do this:
let toysList = toyBox.join(", ");
console.log(toysList); // "car, teddy, ball"
5. shift()
Definition: Removes and returns the first element of the array.
Example: Removing the First Toy (Using
shift
)
If you want to take out the first toy in the box (the "car"), you can use shift:
let firstToy = toyBox.shift();
console.log(firstToy); // "car"
console.log(toyBox); // ["teddy", "ball"]
5. unshift()
Definition: Adds one or more elements to the beginning of the array.
Example: Adding a Toy to the Front (Using
unshift
)
What if you want to add a toy to the beginning of the box? Use unshift:
toyBox.unshift("dinosaur");
console.log(toyBox); // ["dinosaur", "teddy", "ball"]
6 . indexOf()
Definition: Returns the index of the first occurrence of the specified element, or
-1
if the element is not found.Example:
Finding the Toy's Place (Using indexOf
)
You can also find out where a toy is inside the box using indexOf. Let’s see where the "teddy" is:
let teddyPosition = toyBox.indexOf("teddy");
console.log(teddyPosition); // 1
7. splice()
Definition: Adds/removes elements from the array. You specify the starting index, how many elements to remove (can be 0), and optionally, new elements to add.
Example:
Taking Out Toys and Adding New Ones (Using splice
)
Sometimes, you want to take out a toy and maybe add new ones. You can use splice for this. Let's take out "teddy" and add "plane" and "train" instead:
toyBox.splice(1, 1, "plane", "train");
console.log(toyBox); // ["dinosaur", "plane", "train", "ball"]
So here, lets create another array to explaining how other array methods work and to also give you a better perspective.
Here's an example of an array:
javascriptCopy codelet fruits = ["apple", "banana", "cherry"];
In this example, fruits
is an array containing three string elements: "apple"
, "banana"
, and "cherry"
.
8. slice()
Definition: Returns a shallow copy of a portion of an array into a new array, without modifying the original array. The slice begins from the start index (inclusive) and ends before the end index (exclusive).
Example:
let newFruits = fruits.slice(1, 3);
console.log(newFruits); // ["mango", "grapes"]
console.log(fruits); // ["kiwi", "mango", "grapes", "cherry"]
9. reverse()
Definition: Reverses the order of the elements in the array.
Example:
fruits.reverse();
console.log(fruits); // ["cherry", "grapes", "mango", "kiwi"]
10. forEach()
Definition: Executes a provided function once for each array element.
Example:
fruits.forEach(function(fruit) {
console.log(fruit);
});
// Outputs:
// cherry
// grapes
// mango
// kiwi
11. map()
Definition: Creates a new array by applying a function to each element of the original array.
Example:
let uppercasedFruits = fruits.map(function(fruit) {
return fruit.toUpperCase();
});
console.log(uppercasedFruits); // ["CHERRY", "GRAPES", "MANGO", "KIWI"]
12. filter()
Definition: Creates a new array with all elements that pass the test implemented by the provided function.
Example:
let longFruits = fruits.filter(function(fruit) {
return fruit.length > 5;
});
console.log(longFruits); // ["cherry", "grapes"]
13. reduce()
Definition: Executes a reducer function on each element of the array, resulting in a single output value.
Example:
let numbers = [1, 2, 3, 4];
let sum = numbers.reduce(function(total, num) {
return total + num;
}, 0);
console.log(sum); // 10
Let’s Put It All Together
Here’s a full example showing how we use different array methods with our toy box:
let toyBox = ["car", "teddy", "ball"];
// Adding new toys
toyBox.push("robot"); // ["car", "teddy", "ball", "robot"]
// Removing the last toy
toyBox.pop(); // ["car", "teddy", "ball"]
// Counting toys
console.log(toyBox.length); // 3
// Taking out the first toy
toyBox.shift(); // ["teddy", "ball"]
// Adding a toy to the front
toyBox.unshift("dinosaur"); // ["dinosaur", "teddy", "ball"]
// Finding where "teddy" is
console.log(toyBox.indexOf("teddy")); // 1
// Replacing toys
toyBox.splice(1, 1, "plane", "train"); // ["dinosaur", "plane", "train", "ball"]
Summary
Arrays are fundamental in JavaScript as they allow you to store multiple values under a single variable. Whether you need to add, remove, or manipulate elements, JavaScript provides numerous array methods that make working with arrays efficient and flexible.
Here's a quick recap of the methods we covered:
push() / pop(): Add or remove elements from the end of the array.
shift() / unshift(): Add or remove elements from the beginning of the array.
splice(): Add or remove elements at any index.
slice(): Copy a portion of the array.
join(): Create a string from array elements.
forEach(): Loop through each element.
map(): Create a new array by modifying elements.
filter(): Create a new array with elements that pass a test.
reduce(): Reduce an array to a single value by applying a function.
Co-author: Ifeanyi Stanley .S.
Understanding these methods will help you manage and transform arrays effectively in your JavaScript code!
And boom 💥—you’ve just leveled up your array game like a coding boss! Go flex those JavaScript skills and make JavaScript bow to your skills! 💻⚡😎
Subscribe to my newsletter
Read articles from Aondona precious directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
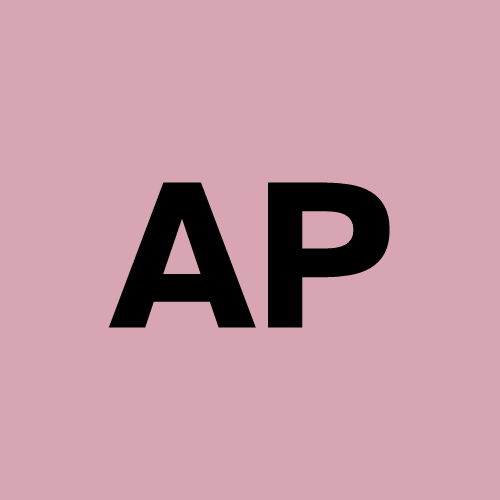