#10.0 What XPath: XPath vs. CSS Selectors
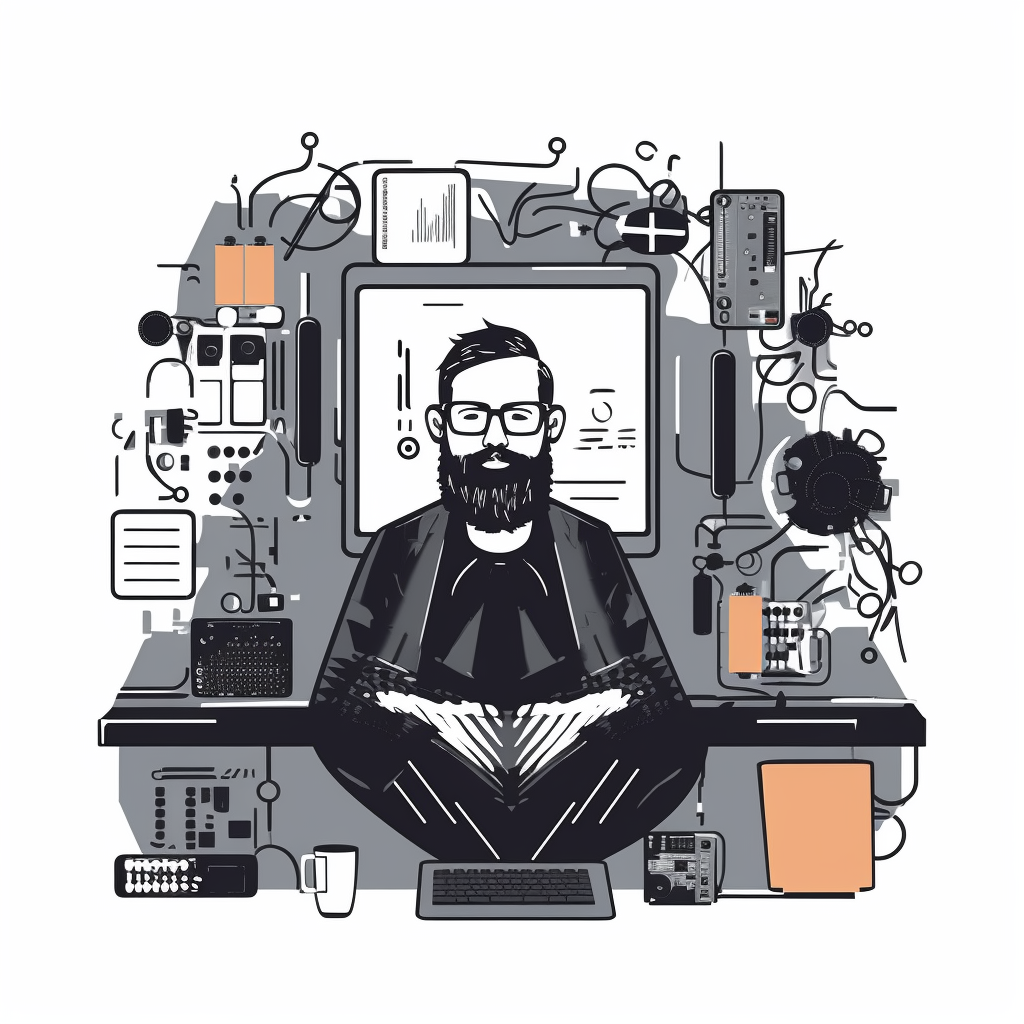
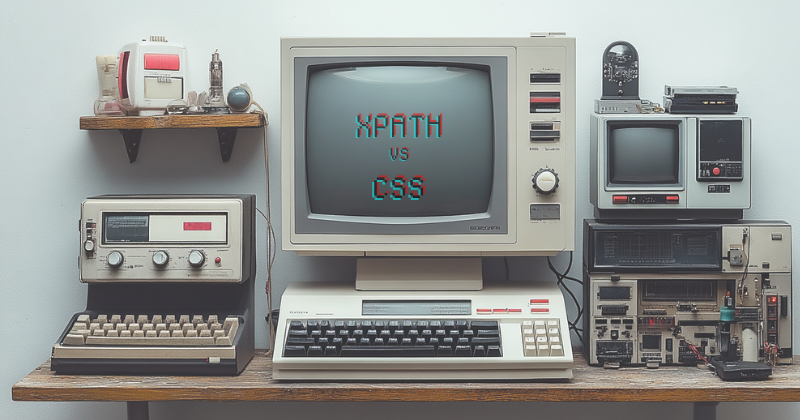
Introduction
When working with Selenium or other web automation tools, choosing between XPath and CSS selectors is a common dilemma. Both have their strengths and weaknesses, and understanding these can significantly impact the efficiency and maintainability of your automation scripts.
Syntax Comparison
XPath Syntax
XPath uses a path-like syntax to navigate through the XML structure of a document.
Example:
//div[@class='container']//p[contains(text(), 'Hello')]
CSS Selector Syntax
CSS selectors use a more concise syntax based on CSS rules.
Example:
div.container p:contains('Hello')
Key Differences
Traversing the DOM
XPath: Can traverse up, down, and sideways in the DOM.
CSS: Primarily traverses down the DOM, with limited abilities to select parent or sibling elements.
Text Content Selection
XPath: Can select elements based on their text content.
//button[text()='Submit']
CSS: Limited text content selection (varies by browser support).
button:contains('Submit') /* Not universally supported */
Attribute Handling
XPath: Flexible attribute selection, including partial matches and comparisons.
//input[@name='username' and @maxlength > 5]
CSS: Good attribute selection, but more limited in comparisons.
input[name='username'][maxlength] /* Can't compare values easily */
Index-based Selection
XPath: Supports index-based selection.
(//div[@class='item'])[3]
CSS: Limited index-based selection (nth-child, nth-of-type).
div.item:nth-of-type(3)
Axis Methods
XPath: Supports various axes like ancestor, following-sibling, etc.
//label[@for='email']/following-sibling::input
CSS: Limited axis support, mainly for direct relationships.
label[for='email'] + input
Performance
XPath: Generally slower, especially with complex queries.
CSS: Usually faster, as it's natively supported by browsers.
Readability
XPath: Can become complex and hard to read with nested conditions.
CSS: Often more concise and readable, especially for simpler selections.
When to Use XPath
When you need to select elements based on text content.
For complex structural relationships (e.g., "find the second td in the third row of the second table").
When working with dynamic attributes or IDs.
When you need to traverse up the DOM tree.
Example:
//table[2]//tr[3]/td[2]
When to Use CSS Selectors
For simpler, more straightforward element selection.
When performance is a critical factor.
When working with well-structured HTML with consistent classes and IDs.
For selecting elements based on multiple classes.
Example:
.container .btn-primary:not(.disabled)
Practical Comparison
Let's compare XPath and CSS selectors for a few common scenarios:
Selecting by ID
XPath:
//input[@id='username']
CSS:
#username
Selecting by Class
XPath:
//div[contains(@class, 'btn-primary')]
CSS:
.btn-primary
Selecting nth Child
XPath:
(//ul[@id='menu']/li)[3]
CSS:
#menu li:nth-child(3)
Selecting by Attribute
XPath:
//input[@name='password' and @type='text']
CSS:
input[name='password'][type='text']
Selecting Parent Element
XPath:
//input[@id='email']/parent::div
CSS: Not directly possible
Conclusion
Both XPath and CSS selectors have their place in web automation. XPath offers more power and flexibility, especially for complex queries and text-based selection. CSS selectors, on the other hand, are generally faster and more readable for simpler selections.
In practice, a combination of both is often the most effective approach. Use CSS selectors for simple, performance-critical selections, and reserve XPath for more complex queries that CSS can't handle efficiently.
Remember, the best choice often depends on the specific structure of your HTML and the requirements of your automation task. Familiarity with both will make you a more versatile and efficient automation engineer.
Subscribe to my newsletter
Read articles from Souvik Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
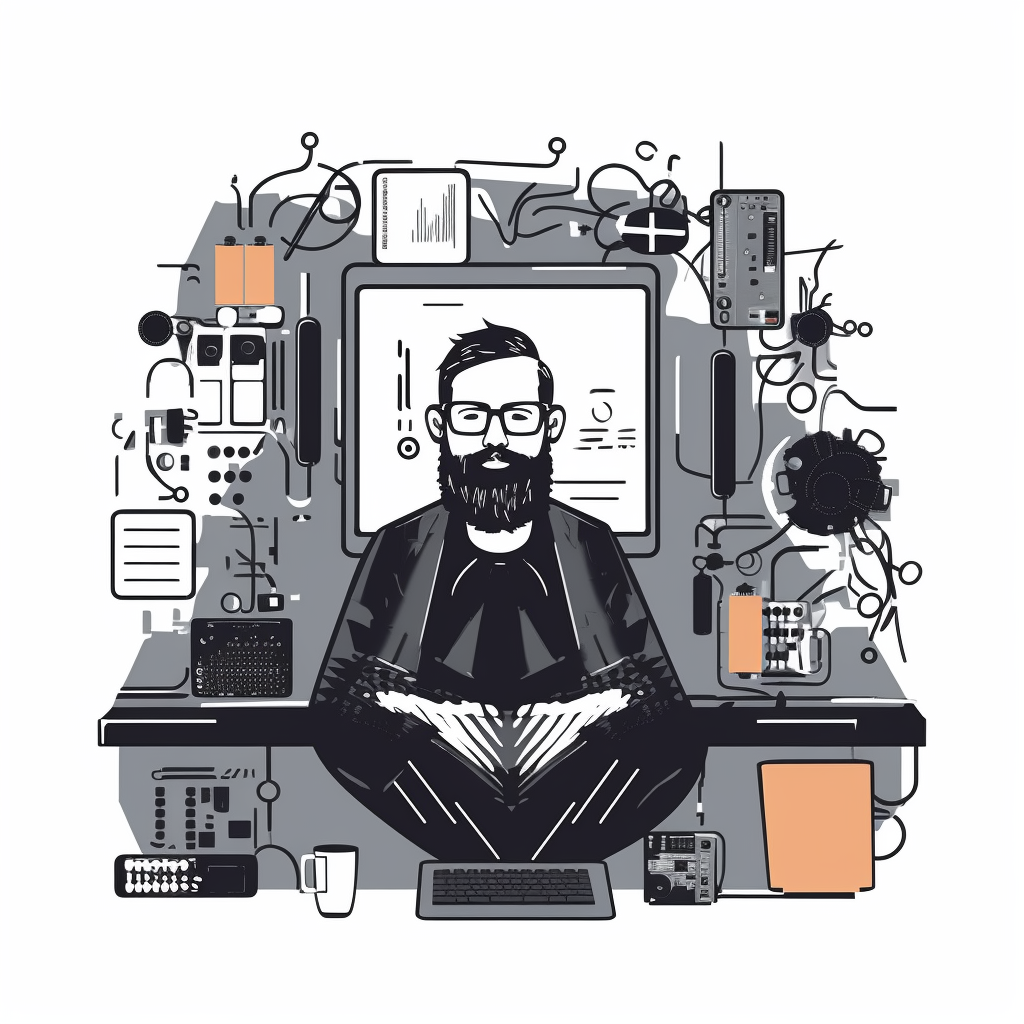
Souvik Dey
Souvik Dey
I design and develop programmatic solutions for Problem-Solving.